Using the BigCommerce API to Create Or Update Products in Python
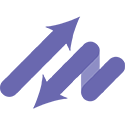
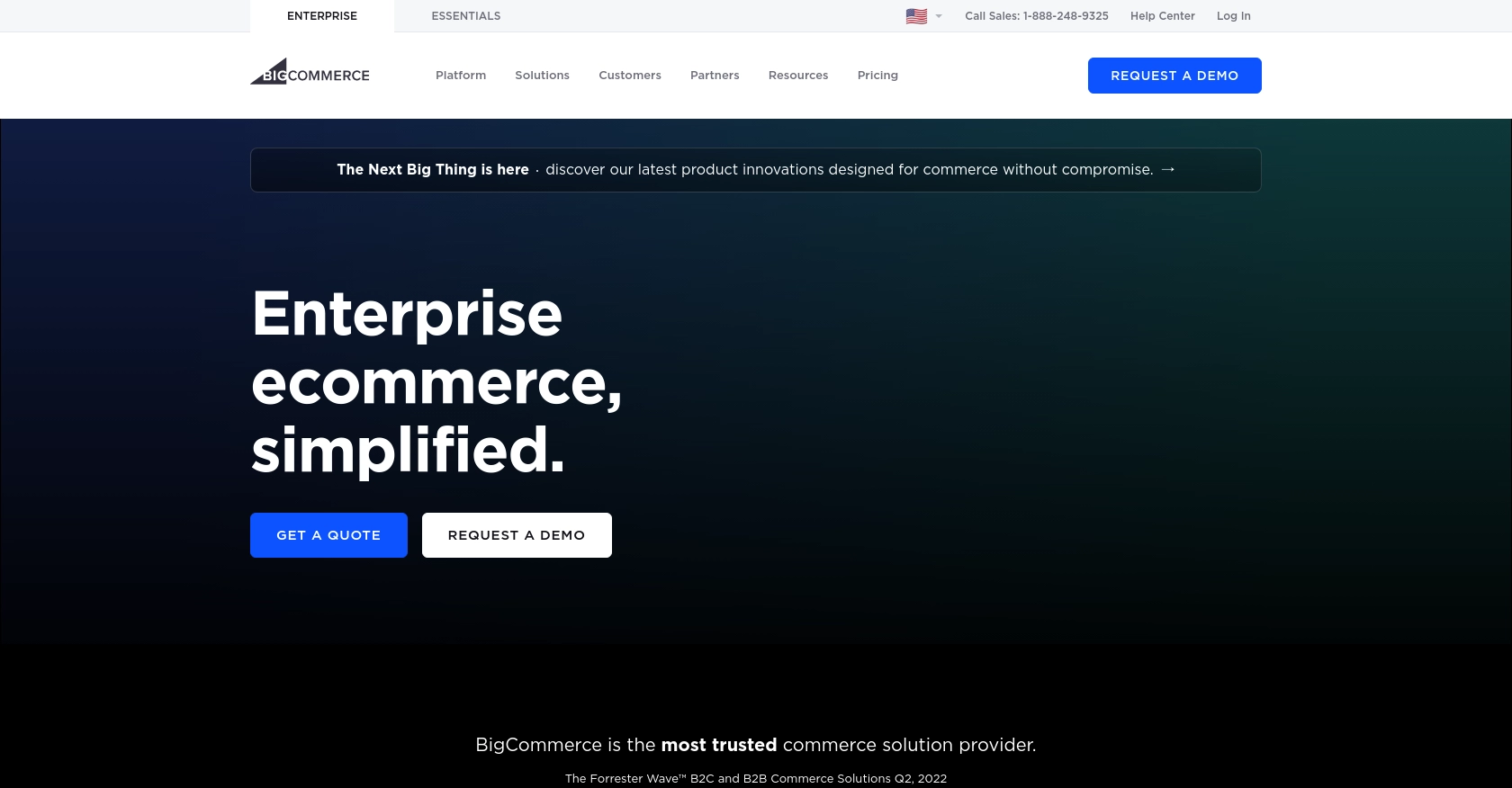
Introduction to BigCommerce API Integration
BigCommerce is a robust e-commerce platform that empowers businesses to create, manage, and scale their online stores. With a wide array of features, including customizable templates, SEO tools, and multi-channel selling capabilities, BigCommerce is a preferred choice for businesses aiming to enhance their online presence.
Integrating with the BigCommerce API allows developers to automate and streamline various e-commerce operations, such as managing product listings. For example, you can use the BigCommerce API to create or update product details directly from your application, ensuring that your store's inventory is always up-to-date and accurate.
This article will guide you through using Python to interact with the BigCommerce API, focusing on creating and updating products efficiently.
Setting Up Your BigCommerce Test or Sandbox Account
Before you can start integrating with the BigCommerce API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your live store data.
Creating a BigCommerce Sandbox Account
To begin, you'll need to create a BigCommerce sandbox account. Follow these steps:
- Visit the BigCommerce Sandbox page and sign up for a free account.
- Fill in the required information and complete the registration process.
- Once registered, you will have access to a sandbox store where you can test API integrations.
Generating API Credentials for BigCommerce
After setting up your sandbox account, you need to generate API credentials to authenticate your requests. Here's how:
- Log in to your BigCommerce control panel.
- Navigate to Advanced Settings > API Accounts.
- Click on Create API Account and select V2/V3 API Token.
- Provide a name for your API account and set the necessary OAuth scopes for product management.
- Click Save to generate your Client ID, Client Secret, and Access Token.
- Store these credentials securely as you will need them to authenticate your API requests.
Understanding BigCommerce API Authentication
BigCommerce uses OAuth-based authentication for API requests. You will need to include the X-Auth-Token
header with your access token in each request. For more details, refer to the BigCommerce Authentication Documentation.
With your sandbox account and API credentials set up, you're ready to start making API calls to create or update products in BigCommerce using Python.
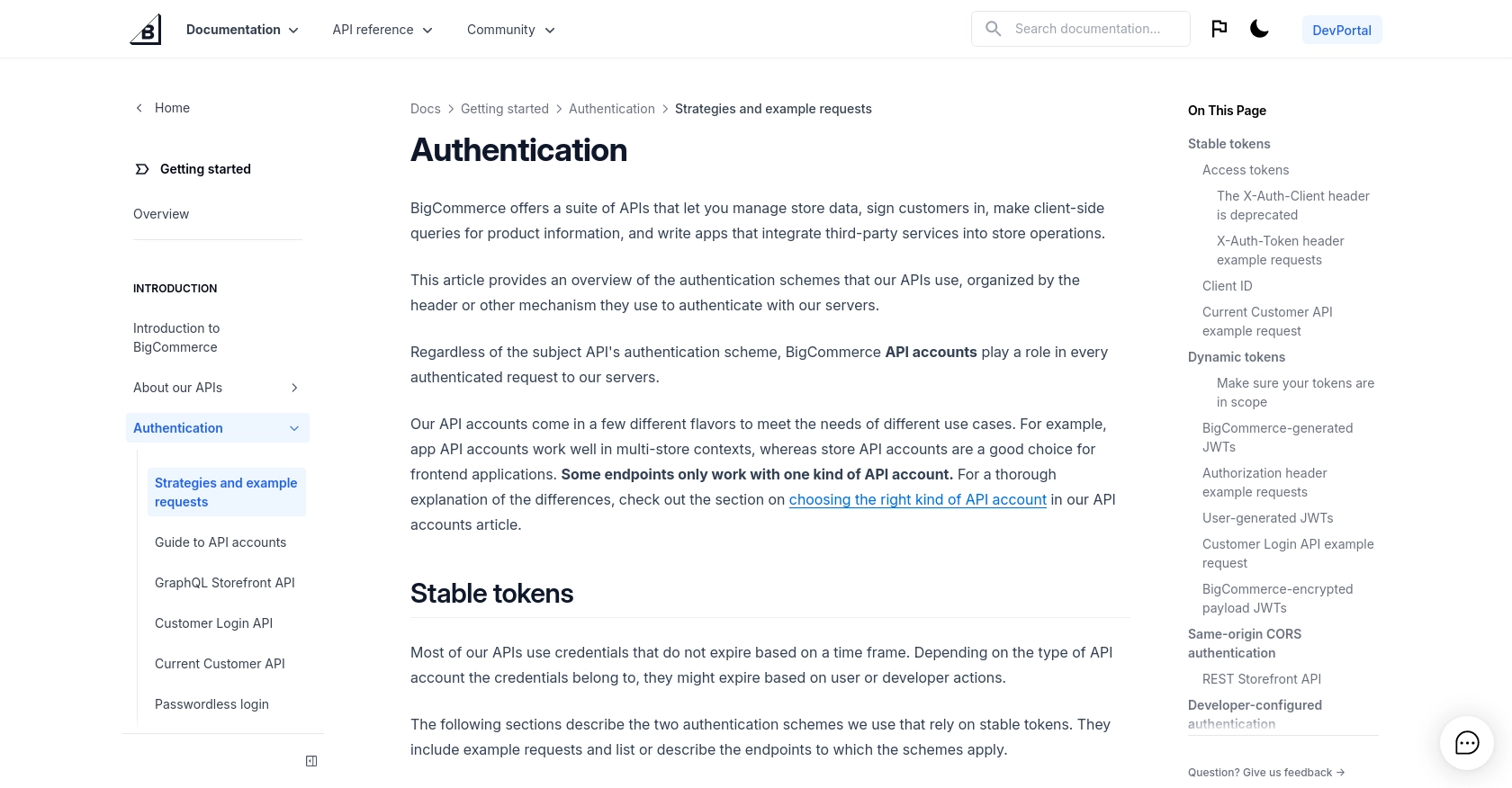
sbb-itb-96038d7
How to Make API Calls to Create or Update Products in BigCommerce Using Python
To interact with the BigCommerce API for creating or updating products, you'll need to use Python. This section will guide you through setting up your environment, writing the necessary code, and executing API requests effectively.
Setting Up Your Python Environment for BigCommerce API Integration
Before making API calls, ensure your Python environment is ready. You'll need Python 3.x and the requests
library to handle HTTP requests. Follow these steps to set up your environment:
- Ensure Python 3.x is installed on your machine. You can download it from the official Python website.
- Install the
requests
library by running the following command in your terminal or command prompt:
pip install requests
Creating a Product in BigCommerce Using Python
To create a product in BigCommerce, you'll need to send a POST request to the BigCommerce API endpoint. Here's a step-by-step guide:
import requests
import json
# Define the API endpoint and headers
url = "https://api.bigcommerce.com/stores/{store_hash}/v3/catalog/products"
headers = {
"X-Auth-Token": "Your_Access_Token",
"Content-Type": "application/json",
"Accept": "application/json"
}
# Define the product data
product_data = {
"name": "New Product",
"type": "physical",
"sku": "NP-001",
"description": "A new product description.",
"weight": 1.0,
"price": 19.99
}
# Send the POST request
response = requests.post(url, headers=headers, data=json.dumps(product_data))
# Check the response
if response.status_code == 201:
print("Product created successfully:", response.json())
else:
print("Failed to create product:", response.status_code, response.json())
Replace Your_Access_Token
and {store_hash}
with your actual access token and store hash. The response will confirm the product creation or provide error details.
Updating a Product in BigCommerce Using Python
To update an existing product, use a PUT request. Here's how you can update a product's details:
# Define the API endpoint for updating a product
product_id = "123" # Replace with your product ID
url = f"https://api.bigcommerce.com/stores/{{store_hash}}/v3/catalog/products/{product_id}"
# Define the updated product data
updated_product_data = {
"name": "Updated Product Name",
"price": 24.99
}
# Send the PUT request
response = requests.put(url, headers=headers, data=json.dumps(updated_product_data))
# Check the response
if response.status_code == 200:
print("Product updated successfully:", response.json())
else:
print("Failed to update product:", response.status_code, response.json())
Ensure you replace product_id
with the ID of the product you wish to update. The response will indicate success or provide error information.
Handling Errors and Verifying API Requests in BigCommerce
When making API calls, it's crucial to handle potential errors and verify the success of your requests. Common HTTP status codes include:
- 201 Created: The product was successfully created.
- 200 OK: The product was successfully updated.
- 404 Not Found: The specified product ID does not exist.
- 422 Unprocessable Entity: The request was well-formed but could not be processed due to semantic errors.
Always check the response status code and handle errors appropriately to ensure smooth integration with the BigCommerce API.
For more detailed information on error codes, refer to the BigCommerce API documentation.
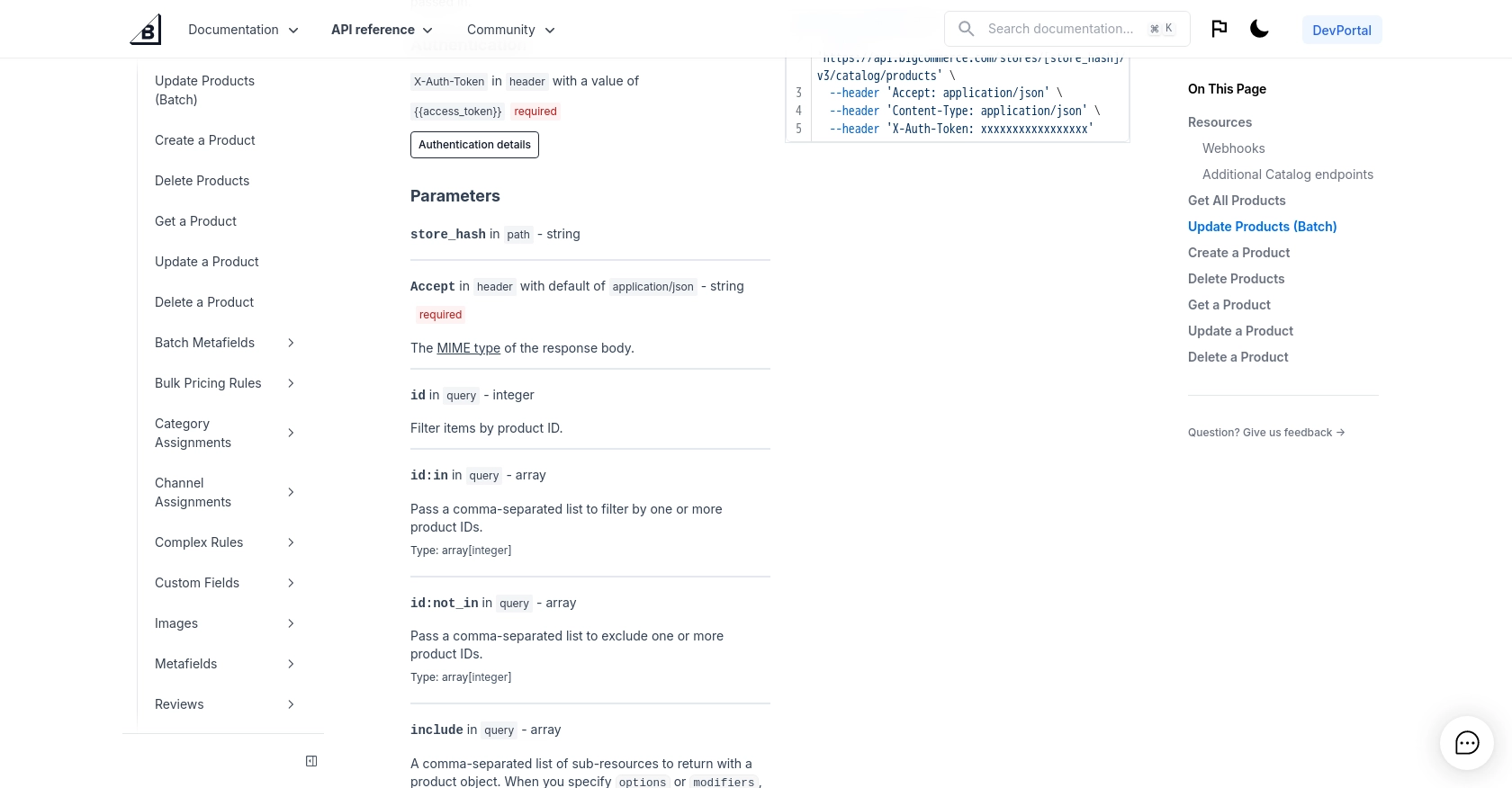
Conclusion and Best Practices for BigCommerce API Integration
Integrating with the BigCommerce API using Python provides a powerful way to automate and manage your e-commerce operations efficiently. By following the steps outlined in this guide, you can create and update products seamlessly, ensuring your online store remains current and competitive.
Best Practices for Secure and Efficient BigCommerce API Usage
- Securely Store Credentials: Always store your API credentials, such as the access token, securely. Consider using environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of API rate limits to avoid throttling. Implement retry logic with exponential backoff to manage requests efficiently.
- Validate API Responses: Always check the response status codes and handle errors gracefully. This ensures your application can recover from issues without disrupting user experience.
- Optimize Data Handling: When dealing with large datasets, consider paginating requests to manage data efficiently and reduce load times.
Leverage Endgrate for Streamlined Integration
While integrating with the BigCommerce API can be straightforward, managing multiple integrations can become complex. Endgrate offers a unified API solution that simplifies integration processes, allowing you to focus on your core product development. By using Endgrate, you can build once and deploy across multiple platforms, saving time and resources.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover a more efficient way to manage your e-commerce integrations.
Read More
Ready to get started?