Using the Quickbooks API to Create or Update Accounts (with Python examples)
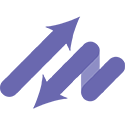
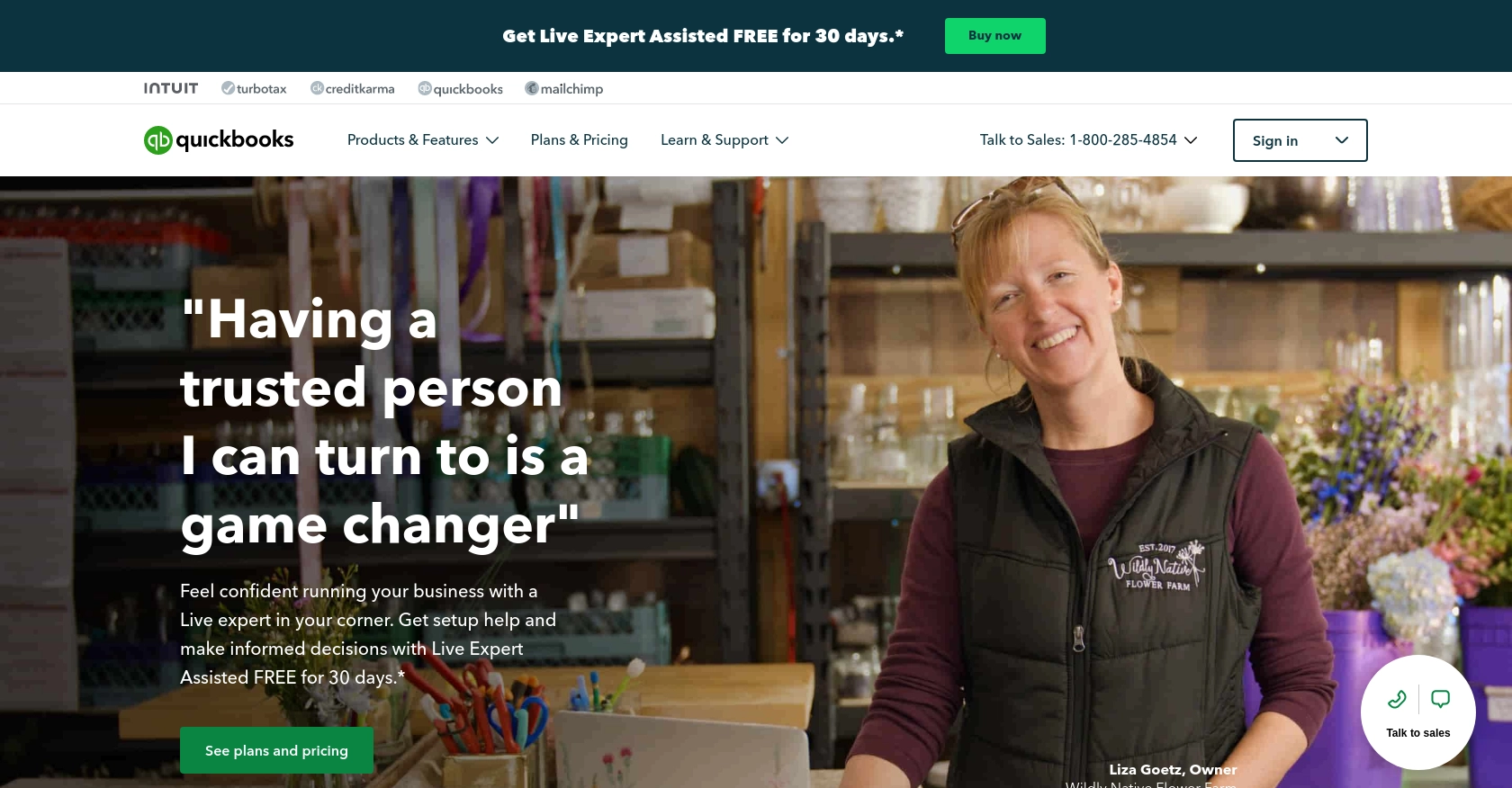
Introduction to QuickBooks API Integration
QuickBooks is a widely-used accounting software that offers a comprehensive suite of tools for managing business finances. It provides features such as invoicing, expense tracking, payroll management, and financial reporting, making it an essential tool for businesses of all sizes.
Integrating with QuickBooks API allows developers to automate and streamline financial processes, such as creating or updating accounts. For example, a developer might use the QuickBooks API to automatically update account information in response to changes in a company's financial system, ensuring that records remain accurate and up-to-date.
Setting Up Your QuickBooks Test/Sandbox Account
Before diving into the integration process, you'll need to set up a QuickBooks sandbox account. This environment allows you to test API interactions without affecting live data, ensuring a safe development process.
Creating a QuickBooks Developer Account
To begin, you'll need a QuickBooks Developer account. Follow these steps to create one:
- Visit the QuickBooks Developer Portal.
- Sign up for a free developer account or log in if you already have one.
- Once logged in, navigate to the dashboard to create a new app.
Generating OAuth Credentials for QuickBooks API
QuickBooks uses OAuth 2.0 for authentication. You'll need to create an app to obtain the necessary credentials:
- In your QuickBooks Developer dashboard, click on "Create an App."
- Select "QuickBooks Online and Payments" as the platform.
- Fill in the required details for your app and save the settings.
- Navigate to the "Keys & OAuth" section to find your Client ID and Client Secret.
- Ensure you note these credentials as they are essential for authenticating API requests.
For more detailed instructions, refer to the QuickBooks OAuth Documentation.
Configuring App Settings in QuickBooks Sandbox
After obtaining your OAuth credentials, configure your app settings to enable API access:
- Go to the "App Settings" section in your QuickBooks Developer dashboard.
- Set the redirect URI to a valid endpoint where QuickBooks can send authentication responses.
- Adjust any additional settings as needed for your development environment.
For further guidance, consult the QuickBooks App Settings Documentation.
With your QuickBooks sandbox account and OAuth credentials set up, you're ready to start making API calls to create or update accounts using Python.
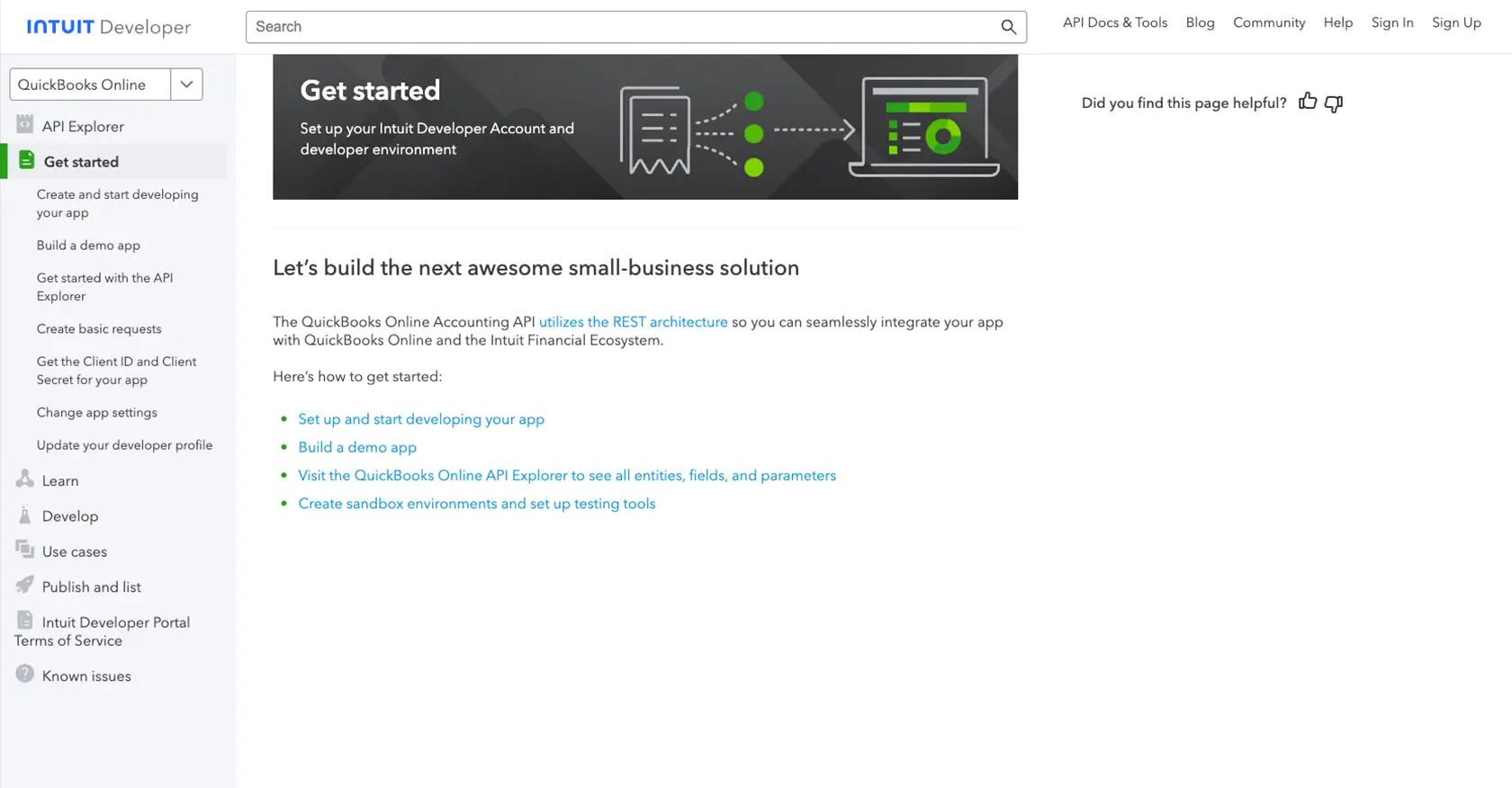
sbb-itb-96038d7
Making API Calls to QuickBooks for Creating or Updating Accounts with Python
To interact with the QuickBooks API using Python, you'll need to set up your development environment and write code to make API requests. This section will guide you through the necessary steps, including setting up Python, installing dependencies, and writing code to create or update accounts in QuickBooks.
Setting Up Your Python Environment for QuickBooks API Integration
Before you start coding, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Once these are installed, open your terminal or command prompt and install the requests
library, which will be used to make HTTP requests:
pip install requests
Writing Python Code to Create or Update Accounts in QuickBooks
Now, let's write the Python code to interact with the QuickBooks API. We'll demonstrate how to create or update an account using the API.
import requests
import json
# Set the API endpoint for creating or updating accounts
url = "https://sandbox-quickbooks.api.intuit.com/v3/company/YOUR_COMPANY_ID/account"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Accept": "application/json"
}
# Define the account data
account_data = {
"Name": "Sample Account",
"AccountType": "Expense"
}
# Convert the account data to JSON
account_json = json.dumps(account_data)
# Make a POST request to create or update the account
response = requests.post(url, headers=headers, data=account_json)
# Check if the request was successful
if response.status_code == 200 or response.status_code == 201:
print("Account created or updated successfully.")
print(response.json())
else:
print("Failed to create or update account.")
print("Error Code:", response.status_code)
print("Error Message:", response.json())
Replace YOUR_COMPANY_ID
and YOUR_ACCESS_TOKEN
with your actual QuickBooks company ID and access token. The code above sets up the API endpoint, headers, and account data, then makes a POST request to create or update an account. If successful, it prints the account details; otherwise, it prints the error code and message.
Verifying API Call Success in QuickBooks Sandbox
After running the code, you can verify the success of your API call by checking the QuickBooks sandbox account. Navigate to the accounts section in your QuickBooks dashboard to see if the account has been created or updated as expected.
Handling Errors and Error Codes in QuickBooks API
When making API calls, it's crucial to handle potential errors. The QuickBooks API returns various error codes that indicate different issues. For example, a 401 error indicates unauthorized access, while a 400 error suggests a bad request. Always check the response status code and handle errors appropriately in your code.
For more detailed information on error codes, refer to the QuickBooks API Documentation.
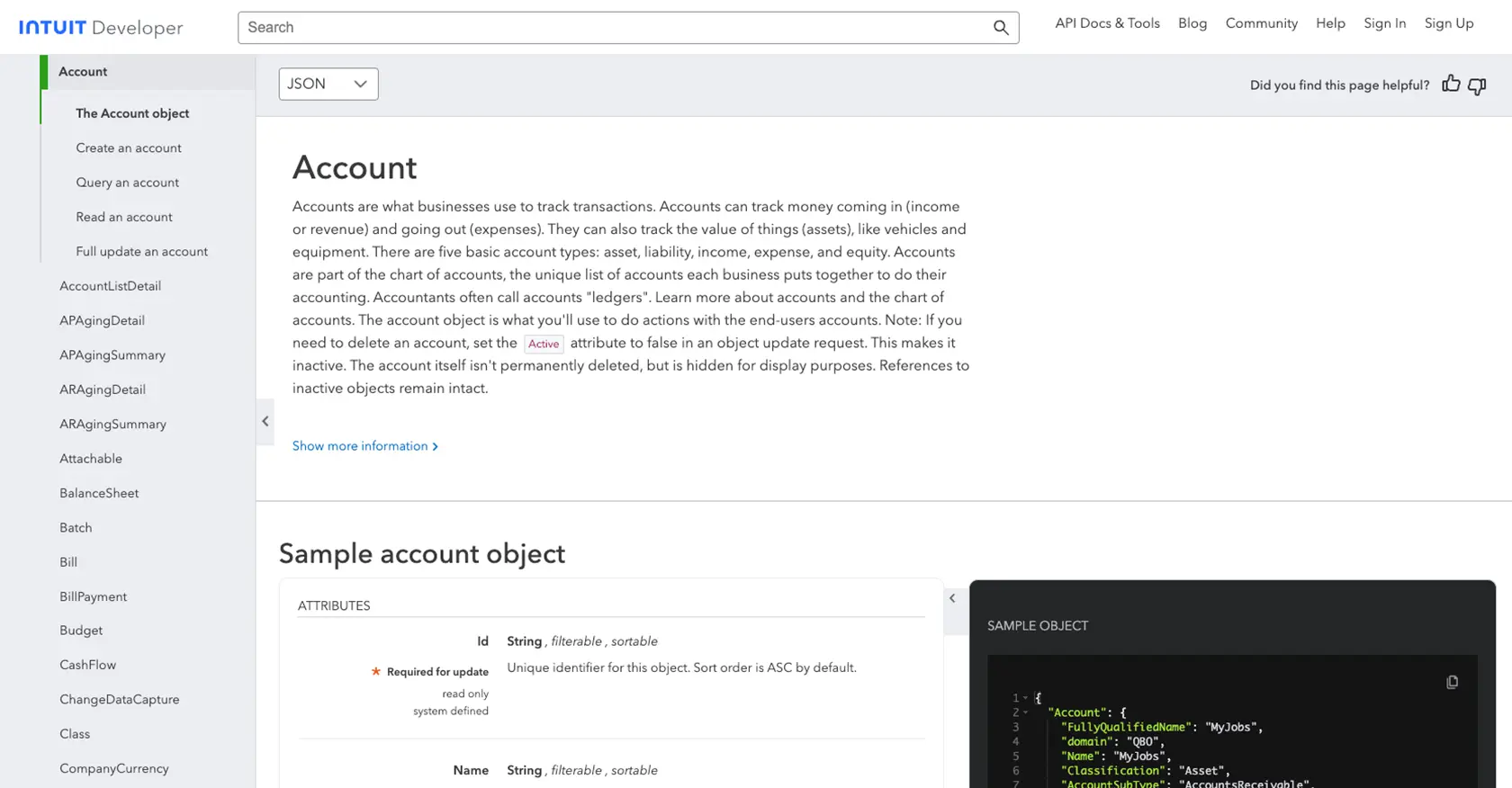
Conclusion and Best Practices for QuickBooks API Integration
Integrating with the QuickBooks API can significantly enhance your ability to manage financial data efficiently. By automating the creation and updating of accounts, you can ensure that your financial records are always accurate and up-to-date, reducing manual errors and saving valuable time.
Best Practices for Managing QuickBooks API Integrations
- Securely Store Credentials: Always store your OAuth credentials, such as the Client ID and Client Secret, securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: QuickBooks API has rate limits in place. Ensure your application gracefully handles rate limit responses by implementing retry logic and backoff strategies.
- Standardize Data Fields: When integrating with QuickBooks, ensure that your data fields are standardized to match QuickBooks' requirements. This will help prevent errors and ensure seamless data exchange.
- Error Handling: Implement robust error handling to manage different error codes returned by the API. This will help you identify and resolve issues quickly.
Streamline Your Integration Process with Endgrate
While integrating with QuickBooks API can be highly beneficial, it can also be complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that simplifies the integration process across multiple platforms, including QuickBooks.
By using Endgrate, you can save time and resources, allowing you to focus on your core product development. Build once for each use case instead of multiple times for different integrations, and provide an intuitive integration experience for your customers.
Explore how Endgrate can help you streamline your integration efforts by visiting Endgrate today.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/all-entities/account
Ready to get started?