Using the PipelineCRM API to Get Deals in PHP
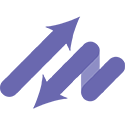
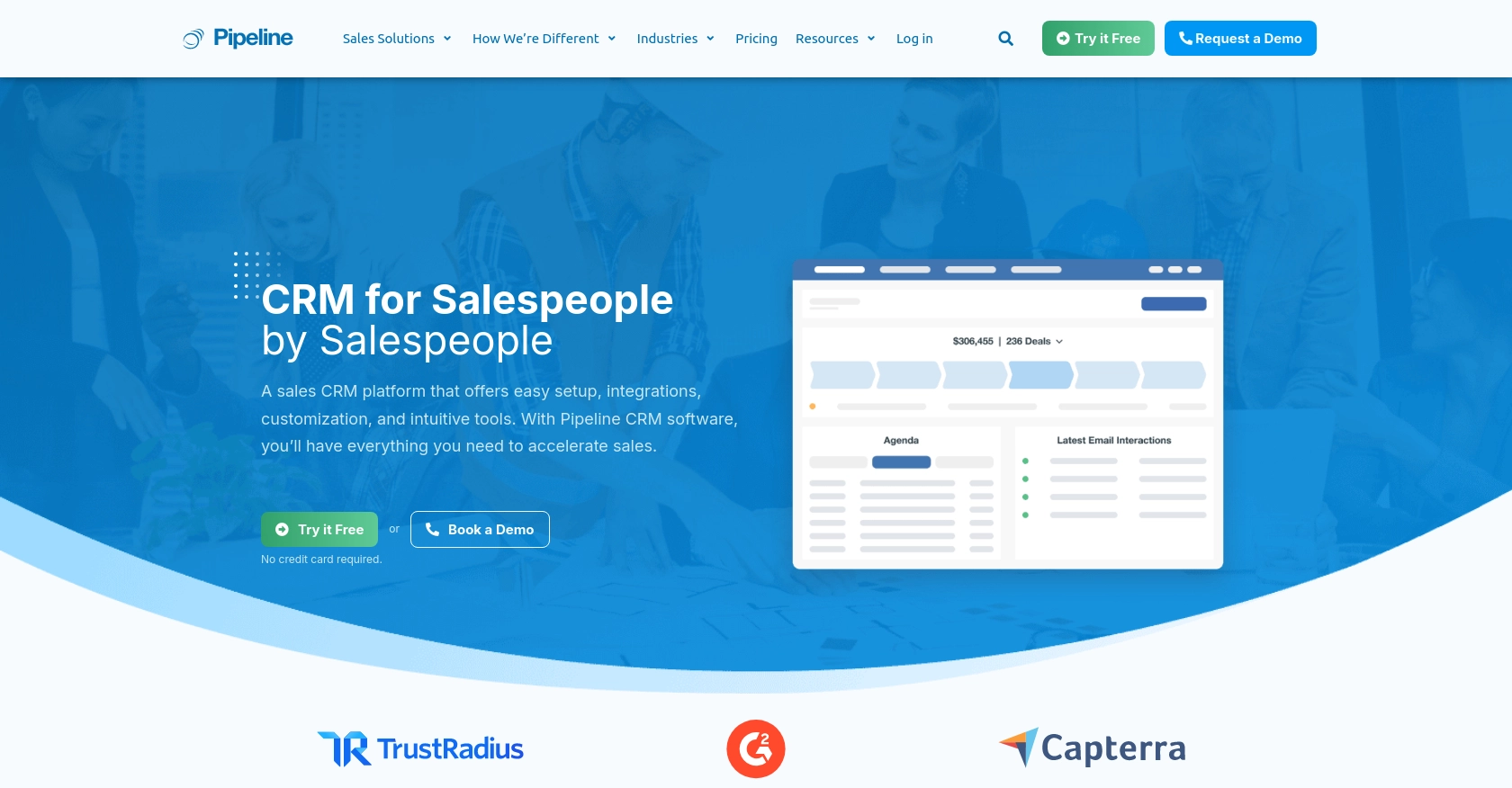
Introduction to PipelineCRM
PipelineCRM is a robust customer relationship management platform designed to help businesses manage their sales processes more effectively. With features like deal tracking, contact management, and sales forecasting, PipelineCRM empowers sales teams to close deals faster and more efficiently.
For developers, integrating with the PipelineCRM API offers the opportunity to automate and streamline sales operations. For example, you might want to retrieve deal information from PipelineCRM to generate real-time sales reports or to synchronize data with other business applications.
Setting Up Your PipelineCRM Test Account
Before you can start integrating with the PipelineCRM API, you need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Creating a PipelineCRM Account
If you don't already have a PipelineCRM account, you can sign up for a free trial on their website. This trial will give you access to the features necessary for testing API interactions.
- Visit the PipelineCRM website and click on the "Free Trial" button.
- Fill out the registration form with your details and submit it.
- Once your account is created, log in to access the dashboard.
Generating Your API Key for PipelineCRM
PipelineCRM uses API key-based authentication to secure API requests. Follow these steps to generate your API key:
- Log in to your PipelineCRM account and navigate to the "Settings" section.
- Under "API Access," click on "Generate API Key."
- Copy the generated API key and store it securely, as you'll need it to authenticate your API requests.
For more detailed information, you can refer to the PipelineCRM API documentation.
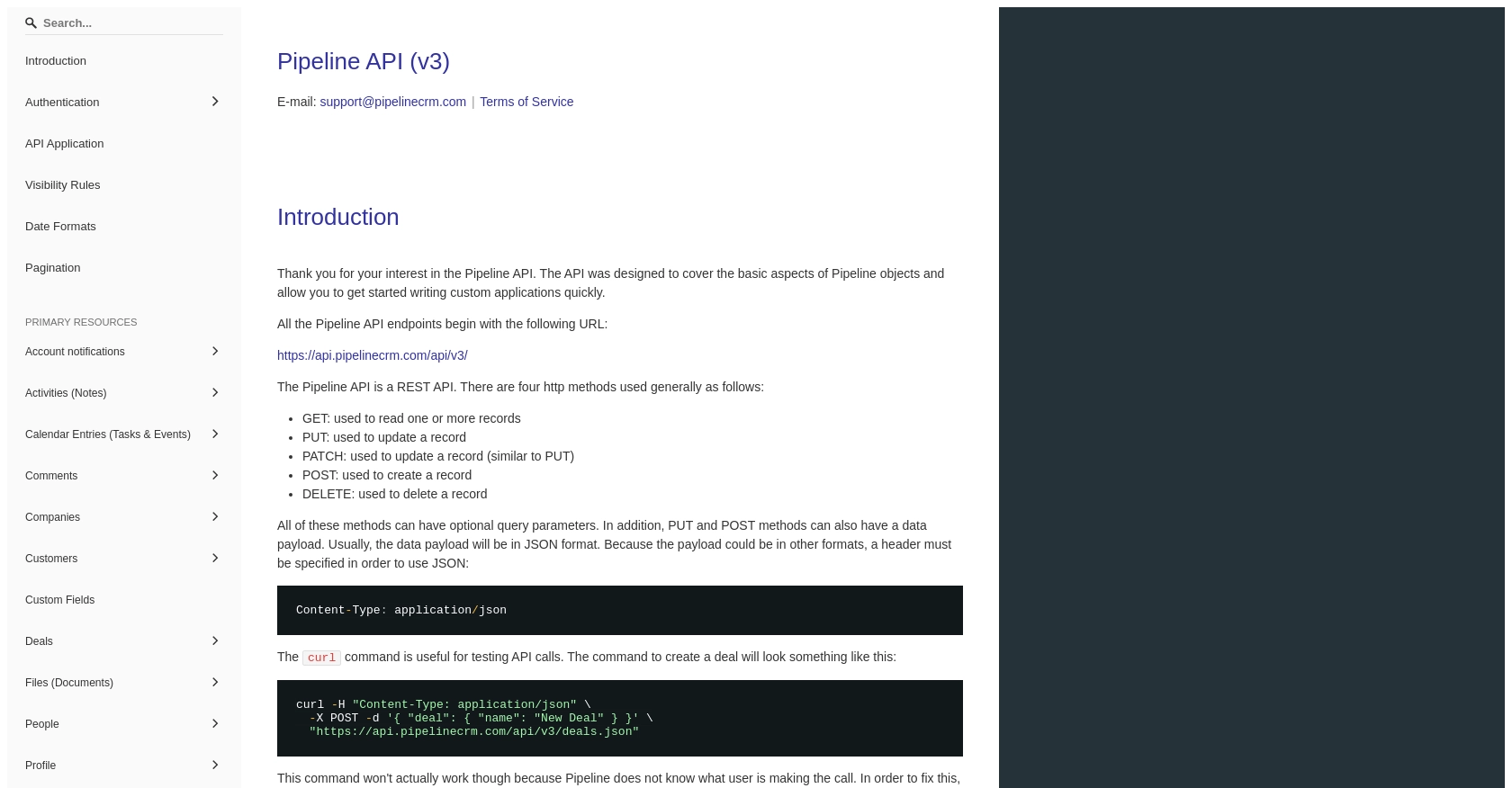
sbb-itb-96038d7
Making API Calls to Retrieve Deals from PipelineCRM Using PHP
To interact with the PipelineCRM API and retrieve deals, you'll need to use PHP, a popular server-side scripting language known for its ease of use and flexibility. This section will guide you through the process of setting up your PHP environment, making API calls, and handling responses.
Setting Up Your PHP Environment
Before you begin, ensure that you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer, the PHP package manager
You'll also need to install the Guzzle HTTP client, which simplifies making HTTP requests in PHP. Run the following command in your terminal:
composer require guzzlehttp/guzzle
Writing the PHP Code to Retrieve Deals
With your environment set up, you can now write the PHP code to make an API call to PipelineCRM and retrieve deals. Create a file named get_pipelinecrm_deals.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiKey = 'Your_API_Key'; // Replace with your actual API key
try {
$response = $client->request('GET', 'https://api.pipelinecrm.com/api/v3/deals', [
'headers' => [
'Authorization' => 'Bearer ' . $apiKey,
'Accept' => 'application/json',
]
]);
$deals = json_decode($response->getBody(), true);
foreach ($deals['data'] as $deal) {
echo 'Deal Name: ' . $deal['name'] . '<br>';
echo 'Deal Value: ' . $deal['value'] . '<br>';
echo 'Deal Stage: ' . $deal['stage'] . '<br><br>';
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace Your_API_Key
with the API key you generated earlier. This script uses Guzzle to send a GET request to the PipelineCRM API endpoint for deals. It then decodes the JSON response and prints out the deal details.
Running the PHP Script and Verifying Results
To execute the script, run the following command in your terminal:
php get_pipelinecrm_deals.php
If successful, you should see a list of deals printed in your terminal. Verify the results by comparing them with the data in your PipelineCRM test account.
Handling Errors and Troubleshooting
When making API calls, it's important to handle potential errors. The example code above includes a try-catch block to catch exceptions and print error messages. Common errors include:
- 401 Unauthorized: Check if your API key is correct and has the necessary permissions.
- 404 Not Found: Ensure the API endpoint URL is correct.
- 500 Internal Server Error: This might indicate an issue with the PipelineCRM server. Try again later.
For more detailed error handling, refer to the PipelineCRM API documentation.
Conclusion and Best Practices for Using PipelineCRM API in PHP
Integrating with the PipelineCRM API using PHP can significantly enhance your ability to manage and automate sales processes. By following the steps outlined in this article, you can efficiently retrieve deal information and integrate it with other business applications.
Best Practices for Secure and Efficient API Integration
- Securely Store API Keys: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by PipelineCRM. Implement logic to handle rate limit responses gracefully to avoid disruptions in service.
- Standardize Data Fields: Ensure that data retrieved from PipelineCRM is standardized to match your application's data structures for seamless integration.
- Error Handling: Implement comprehensive error handling to manage different HTTP status codes and exceptions, ensuring robust and reliable API interactions.
Streamlining Integrations with Endgrate
While integrating with individual APIs like PipelineCRM can be beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including PipelineCRM.
By using Endgrate, you can save time and resources, allowing your development team to focus on core product features. With a single API endpoint, you can manage multiple integrations efficiently, providing a seamless experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate and discover the benefits of a streamlined integration process.
Read More
Ready to get started?