Using the Active Campaign API to Get Contacts (with Javascript examples)
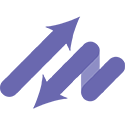
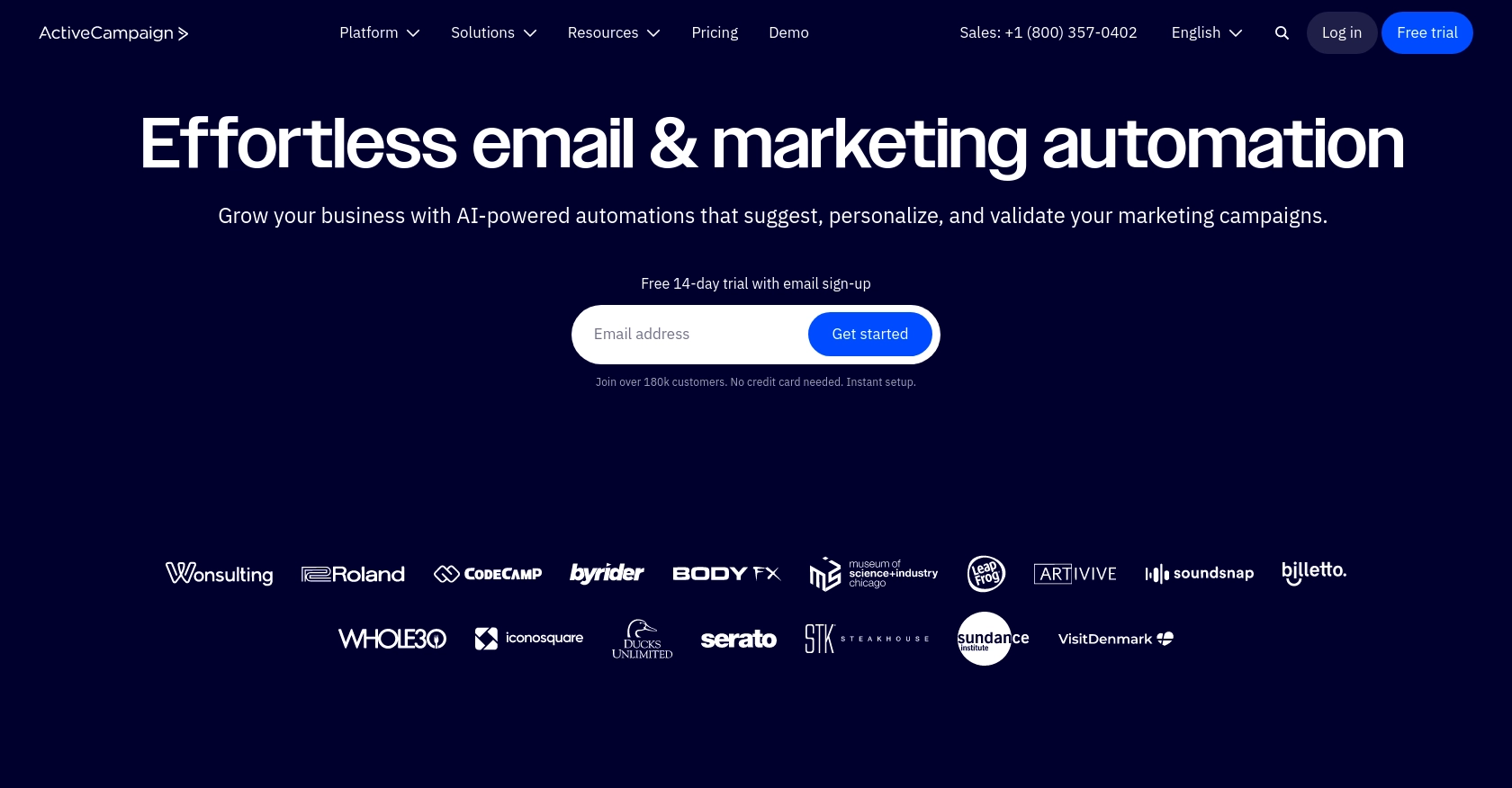
Introduction to Active Campaign API
Active Campaign is a powerful marketing automation platform that combines email marketing, CRM, and sales automation to help businesses grow and engage with their audience effectively. With its robust set of features, Active Campaign enables businesses to create personalized customer experiences and streamline their marketing efforts.
Developers may want to integrate with the Active Campaign API to access and manage contact data, automate marketing workflows, and enhance customer relationship management. For example, using the Active Campaign API, a developer can retrieve contact information to personalize email campaigns or sync contact data with other CRM systems.
This article will guide you through using JavaScript to interact with the Active Campaign API, specifically focusing on retrieving contact information. By following this tutorial, you'll learn how to efficiently access and manage contacts within the Active Campaign platform using JavaScript.
Setting Up Your Active Campaign Test Account
Before you can start interacting with the Active Campaign API using JavaScript, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Create an Active Campaign Account
If you don't already have an Active Campaign account, you can sign up for a free trial on their website. This trial will give you access to the necessary features to test API interactions.
- Visit the Active Campaign website and click on the "Start Your Free Trial" button.
- Fill out the registration form with your details and follow the instructions to complete the setup.
- Once your account is created, log in to access your dashboard.
Generate Your API Key
To authenticate your API requests, you'll need an API key. Follow these steps to obtain it:
- Navigate to the "Settings" section in your Active Campaign dashboard.
- Click on the "Developer" tab to find your API key.
- Copy the API key and keep it secure, as it will be used to authenticate your API requests.
For more details on authentication, refer to the Active Campaign API authentication documentation.
Configure Your Base URL
Each Active Campaign account has a unique base URL for API access. To find yours:
- Go to the "Developer" tab in the "Settings" section of your dashboard.
- Locate your API URL, which will be in the format:
https://
..api-us1.com/api/3/ - Ensure you use this URL for all API requests to access your account's data.
For more information, visit the Active Campaign API base URL documentation.
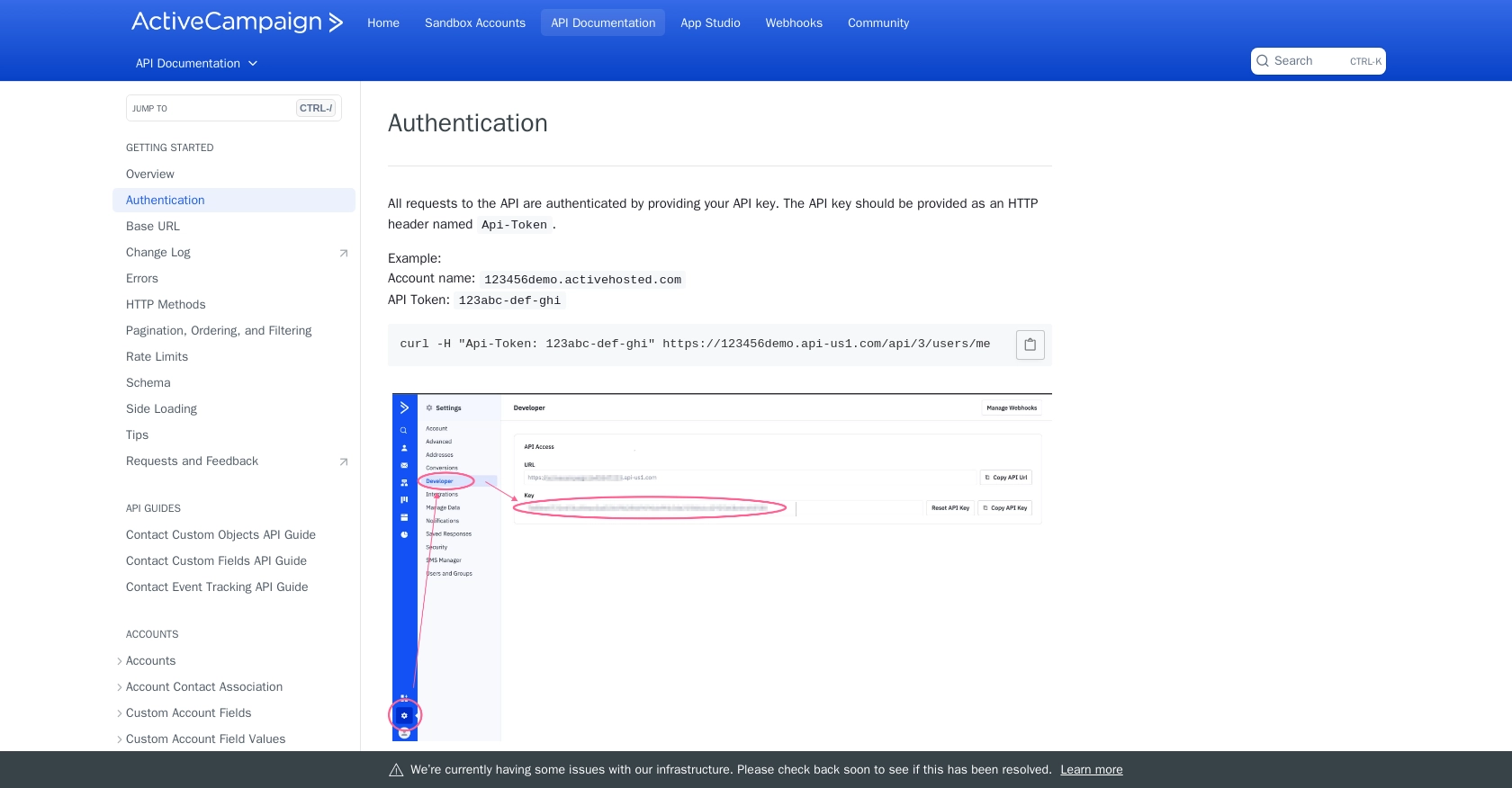
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Active Campaign Using JavaScript
To interact with the Active Campaign API using JavaScript, you'll need to make HTTP requests to the API endpoints. This section will guide you through the process of retrieving contact information using JavaScript, focusing on the necessary setup and code implementation.
Prerequisites for JavaScript Integration with Active Campaign API
Before proceeding, ensure you have the following:
- Node.js installed on your machine.
- A text editor or IDE for writing JavaScript code.
- Your Active Campaign API key and base URL, as described in the setup section.
Installing Required JavaScript Libraries
To make HTTP requests in JavaScript, you'll use the axios
library. Install it using npm:
npm install axios
Writing JavaScript Code to Retrieve Contacts from Active Campaign
Create a new JavaScript file, for example, getContacts.js
, and add the following code:
const axios = require('axios');
// Set your API key and base URL
const apiKey = 'Your_API_Key';
const baseUrl = 'https://your-account.api-us1.com/api/3/contacts';
// Configure headers for authentication
const headers = {
'Api-Token': apiKey,
'Accept': 'application/json'
};
// Function to retrieve contacts
async function getContacts() {
try {
const response = await axios.get(baseUrl, { headers });
const contacts = response.data.contacts;
console.log('Contacts retrieved successfully:', contacts);
} catch (error) {
console.error('Error retrieving contacts:', error.response ? error.response.data : error.message);
}
}
// Execute the function
getContacts();
Replace Your_API_Key
and your-account
with your actual API key and account name.
Running the JavaScript Code to Fetch Contacts
Execute the script using Node.js:
node getContacts.js
Upon successful execution, you should see the list of contacts retrieved from your Active Campaign account in the console output.
Handling Errors and Verifying API Call Success
To ensure the API call is successful, check the response status and data. The code above logs any errors encountered during the request. If the request is successful, the contacts data will be printed to the console.
For more details on error codes and handling, refer to the Active Campaign API documentation.
Optimizing API Requests with Pagination
Active Campaign API supports pagination to manage large datasets. Use the limit
and offset
query parameters to control the number of records returned:
const params = {
limit: 20, // Number of records per page
offset: 0 // Starting point for records
};
async function getPaginatedContacts() {
try {
const response = await axios.get(baseUrl, { headers, params });
const contacts = response.data.contacts;
console.log('Paginated contacts:', contacts);
} catch (error) {
console.error('Error retrieving paginated contacts:', error.response ? error.response.data : error.message);
}
}
getPaginatedContacts();
Adjust the limit
and offset
values as needed to retrieve additional pages of contacts.
For more information on pagination, visit the Active Campaign API pagination documentation.
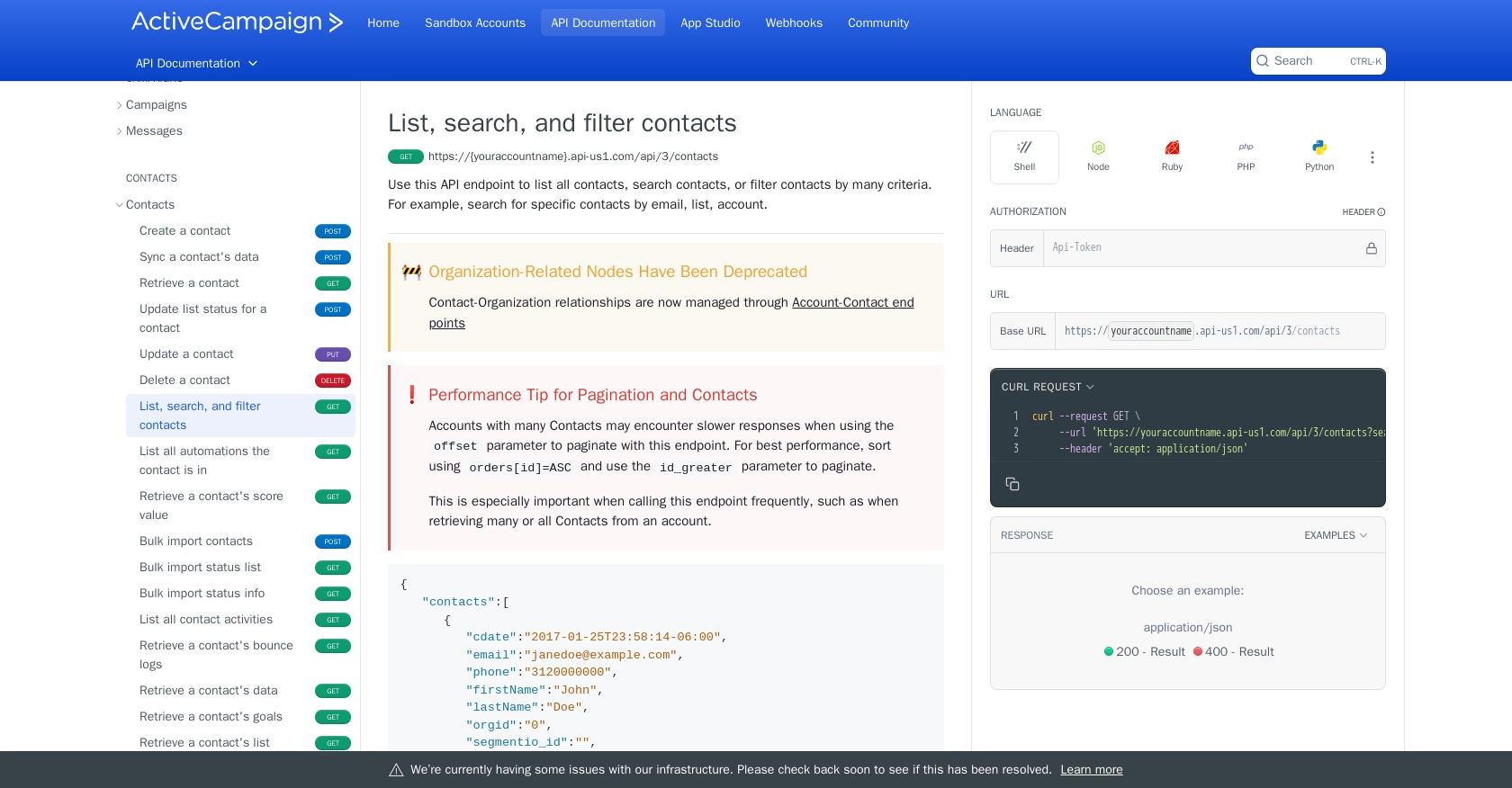
Conclusion and Best Practices for Using Active Campaign API with JavaScript
Integrating with the Active Campaign API using JavaScript provides a powerful way to manage and automate your marketing efforts. By following the steps outlined in this guide, you can efficiently retrieve and handle contact data, enhancing your CRM capabilities.
Best Practices for Secure and Efficient API Integration
- Secure API Key Storage: Always keep your API key confidential. Avoid hardcoding it in your scripts and consider using environment variables or secure vaults for storage.
- Handle Rate Limits: The Active Campaign API has a rate limit of 5 requests per second per account. Implement retry logic and exponential backoff to handle rate limit errors gracefully. For more details, refer to the Active Campaign API rate limits documentation.
- Optimize Data Retrieval: Use pagination to manage large datasets efficiently. Adjust the
limit
andoffset
parameters to retrieve data in manageable chunks. - Error Handling: Implement robust error handling to manage API call failures. Log errors for debugging and provide user-friendly messages where applicable.
- Data Transformation: Standardize and transform data fields as needed to ensure consistency across different systems and integrations.
Enhance Your Integration Strategy with Endgrate
While integrating with the Active Campaign API can significantly enhance your marketing automation, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes, allowing you to focus on your core product development.
With Endgrate, you can:
- Save time and resources by outsourcing integration tasks.
- Build once for each use case, avoiding redundant development efforts.
- Provide an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/activecampaign
- https://developers.activecampaign.com/reference/authentication
- https://developers.activecampaign.com/reference/url
- https://developers.activecampaign.com/reference/pagination
- https://developers.activecampaign.com/reference/rate-limits
- https://developers.activecampaign.com/reference/list-all-contacts
Ready to get started?