Using the Outreach API to Get Accounts (with Python examples)
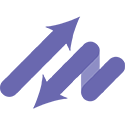
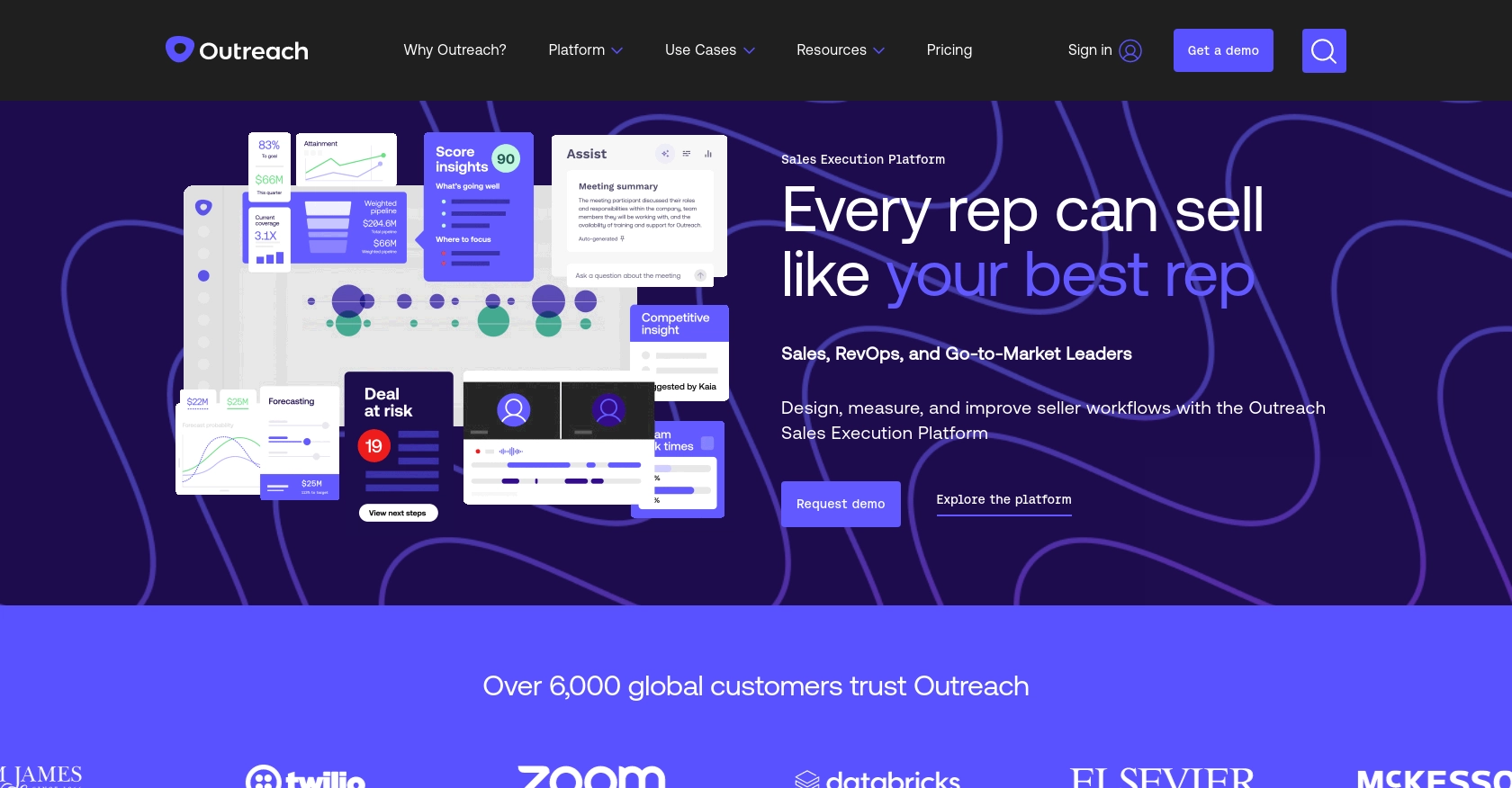
Introduction to Outreach API
Outreach is a leading sales engagement platform that empowers sales teams to drive efficient and effective communication with prospects. It offers a comprehensive suite of tools designed to streamline sales processes, enhance productivity, and improve customer relationships.
Integrating with the Outreach API allows developers to access and manage sales data, such as accounts, to automate and personalize sales strategies. For example, a developer might use the Outreach API to retrieve account information and use it to tailor sales pitches based on a company's industry and size.
Setting Up Your Outreach Test/Sandbox Account
Before you can start using the Outreach API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Outreach provides a development environment to help you get started.
Creating an Outreach Developer Account
If you don't have an Outreach account, you can sign up for a developer account on the Outreach website. This account will give you access to the necessary tools and resources to build and test your integrations.
- Visit the Outreach Developer Portal and sign up for a new account.
- Follow the on-screen instructions to complete the registration process.
- Once registered, log in to your Outreach developer account.
Setting Up OAuth for Outreach API Access
Outreach uses OAuth 2.0 for authentication, which is a secure protocol for authorizing API requests. Follow these steps to set up OAuth:
- Navigate to the "My Apps" section in the Outreach Developer Portal.
- Click on "Create New App" to start setting up your application.
- Fill in the required details, such as the app name and description.
- Specify the redirect URI(s) that Outreach will use to send the authorization code back to your application.
- Select the necessary OAuth scopes that your application will require. Ensure you include scopes related to accounts.
- Save your app settings to generate your client ID and client secret.
Note: Your OAuth client secret will only be displayed once, so make sure to store it securely.
Generating OAuth Tokens
With your app set up, you can now generate OAuth tokens to authenticate your API requests:
- Direct users to the following URL to obtain an authorization code:
- After the user authorizes your app, they will be redirected to your specified redirect URI with an authorization code.
- Exchange the authorization code for an access token by making a POST request to:
- Store the access token securely for making authenticated API requests.
https://api.outreach.io/oauth/authorize?client_id=<Your_Client_ID>&redirect_uri=<Your_Redirect_URI>&response_type=code&scope=<Your_Scopes>
curl https://api.outreach.io/oauth/token \
-X POST \
-d client_id=<Your_Client_ID> \
-d client_secret=<Your_Client_Secret> \
-d redirect_uri=<Your_Redirect_URI> \
-d grant_type=authorization_code \
-d code=<Authorization_Code>
For more details on OAuth setup, refer to the Outreach OAuth documentation.
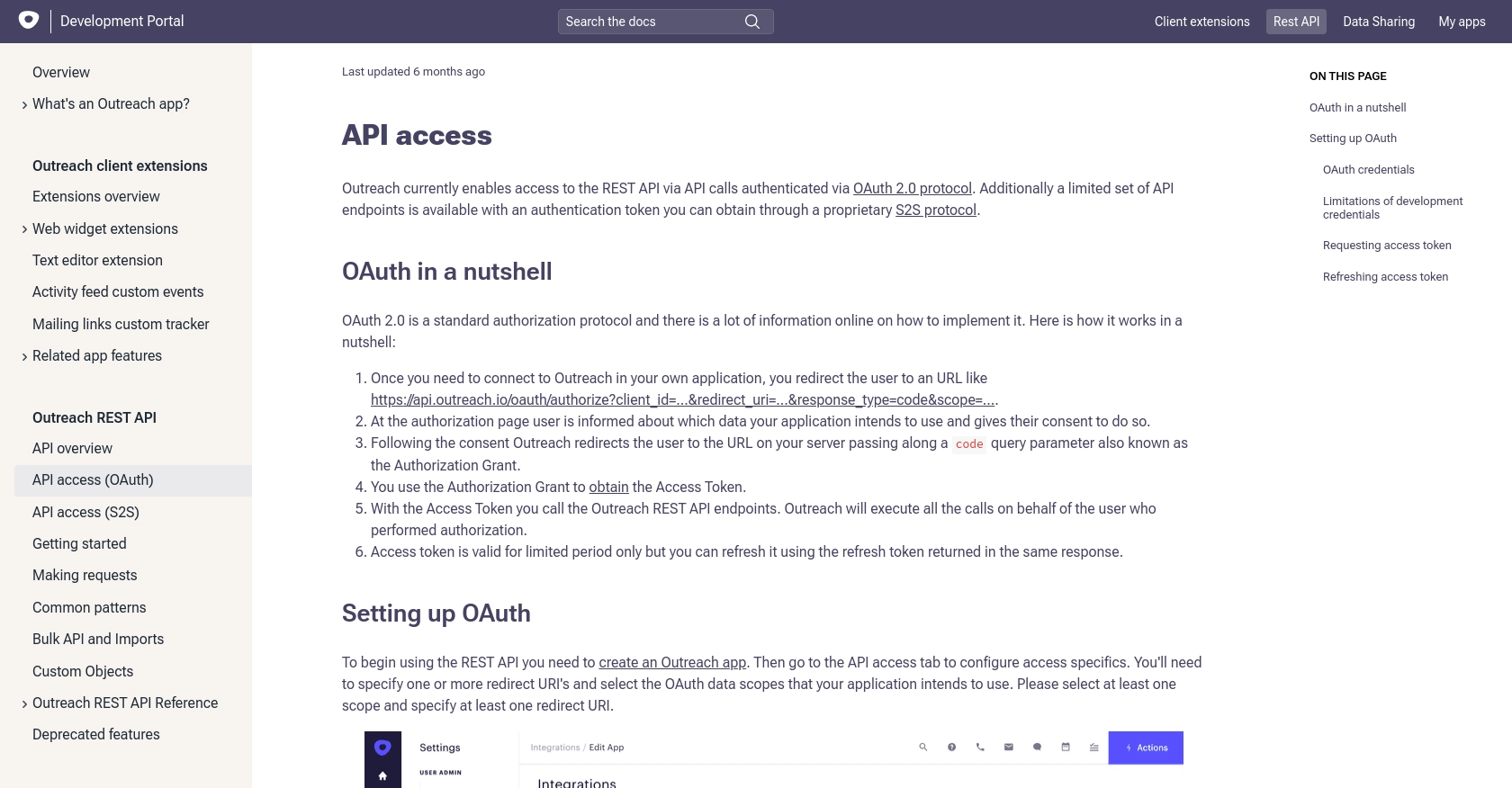
sbb-itb-96038d7
Making API Calls to Retrieve Accounts from Outreach Using Python
To interact with the Outreach API and retrieve account data, you'll need to make HTTP requests using Python. This section will guide you through the process, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for Outreach API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using pip:
pip install requests
Writing Python Code to Get Accounts from Outreach API
Once your environment is ready, you can write a Python script to fetch accounts from the Outreach API. Create a file named get_outreach_accounts.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://api.outreach.io/api/v2/accounts"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/vnd.api+json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
for account in data["data"]:
print(f"Account Name: {account['attributes']['name']}")
else:
print(f"Failed to retrieve accounts: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the access token obtained during the OAuth setup.
Understanding the Python Code for Outreach API Integration
In the code above, you start by importing the requests
library to handle HTTP requests. You then define the API endpoint and set the necessary headers, including the authorization token. The script makes a GET request to the Outreach API to retrieve account data. If successful, it prints the account names; otherwise, it outputs an error message.
Verifying API Call Success in the Outreach Sandbox
After running the script, verify the retrieved data by checking the accounts in your Outreach sandbox environment. The output should match the accounts available in your test setup.
Handling Errors and Understanding Outreach API Error Codes
When making API calls, it's crucial to handle potential errors. The Outreach API may return various HTTP status codes to indicate issues:
- 401 Unauthorized: Check your access token and ensure it's valid.
- 403 Forbidden: Verify that your OAuth scopes include the necessary permissions.
- 429 Too Many Requests: The API is rate-limited to 10,000 requests per hour. Implement retry logic with exponential backoff.
For more details on error handling, refer to the Outreach API documentation.
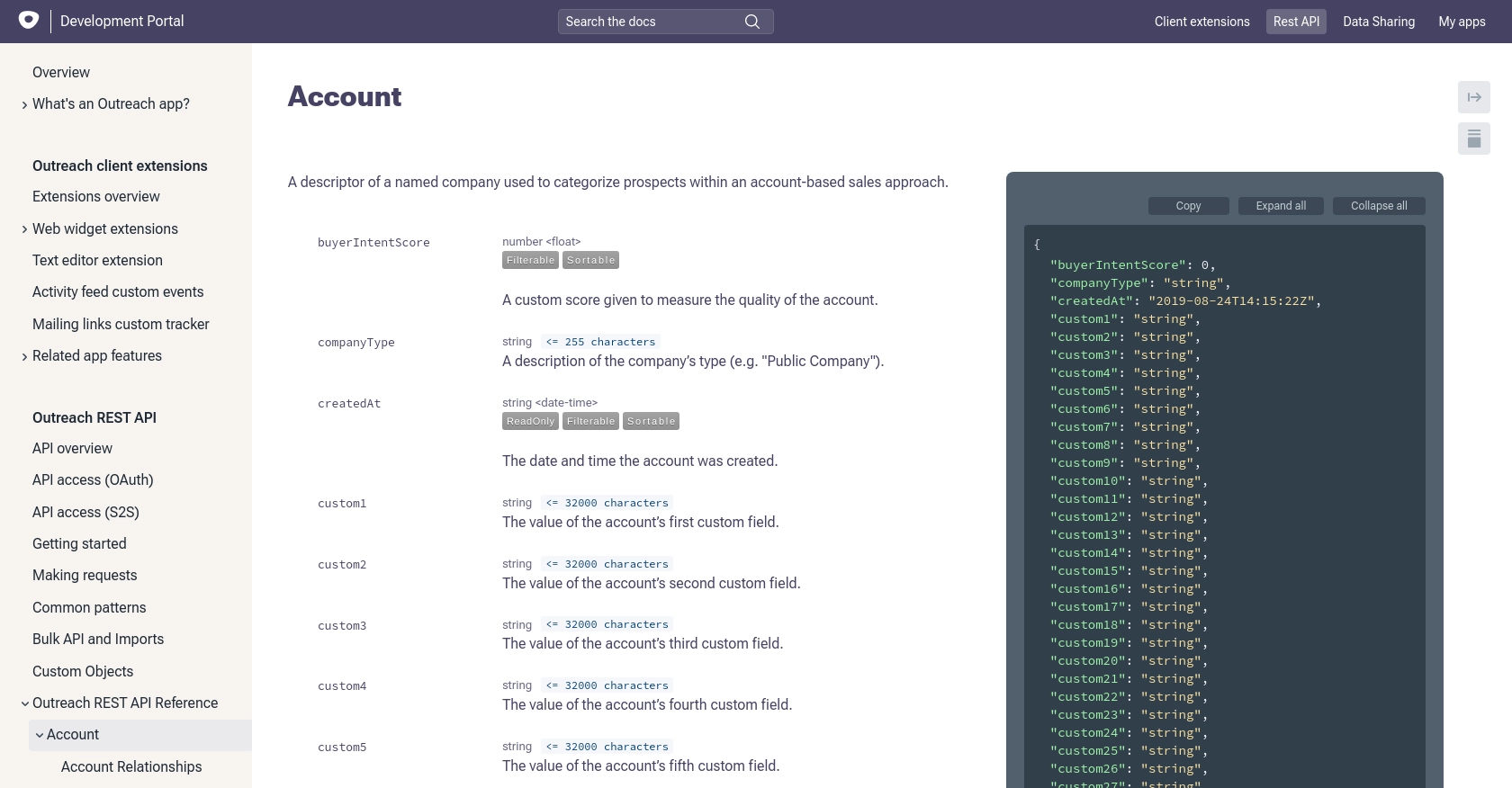
Conclusion and Best Practices for Using the Outreach API with Python
Integrating with the Outreach API using Python can significantly enhance your sales strategies by providing seamless access to account data. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate using OAuth, and retrieve account information.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth tokens and client secrets securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: The Outreach API enforces a rate limit of 10,000 requests per hour. Implement retry logic with exponential backoff to handle 429 errors gracefully.
- Optimize Data Handling: Transform and standardize data fields as needed to ensure consistency across your applications.
- Refresh Tokens Regularly: Access tokens are short-lived. Use refresh tokens to obtain new access tokens before they expire to maintain uninterrupted access.
Streamlining Integrations with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Outreach. By leveraging Endgrate, you can focus on your core product while ensuring a seamless integration experience for your customers.
Explore how Endgrate can help you save time and resources by visiting Endgrate today.
Read More
Ready to get started?