Using the Hubspot API to Get Deals in Javascript
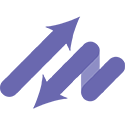
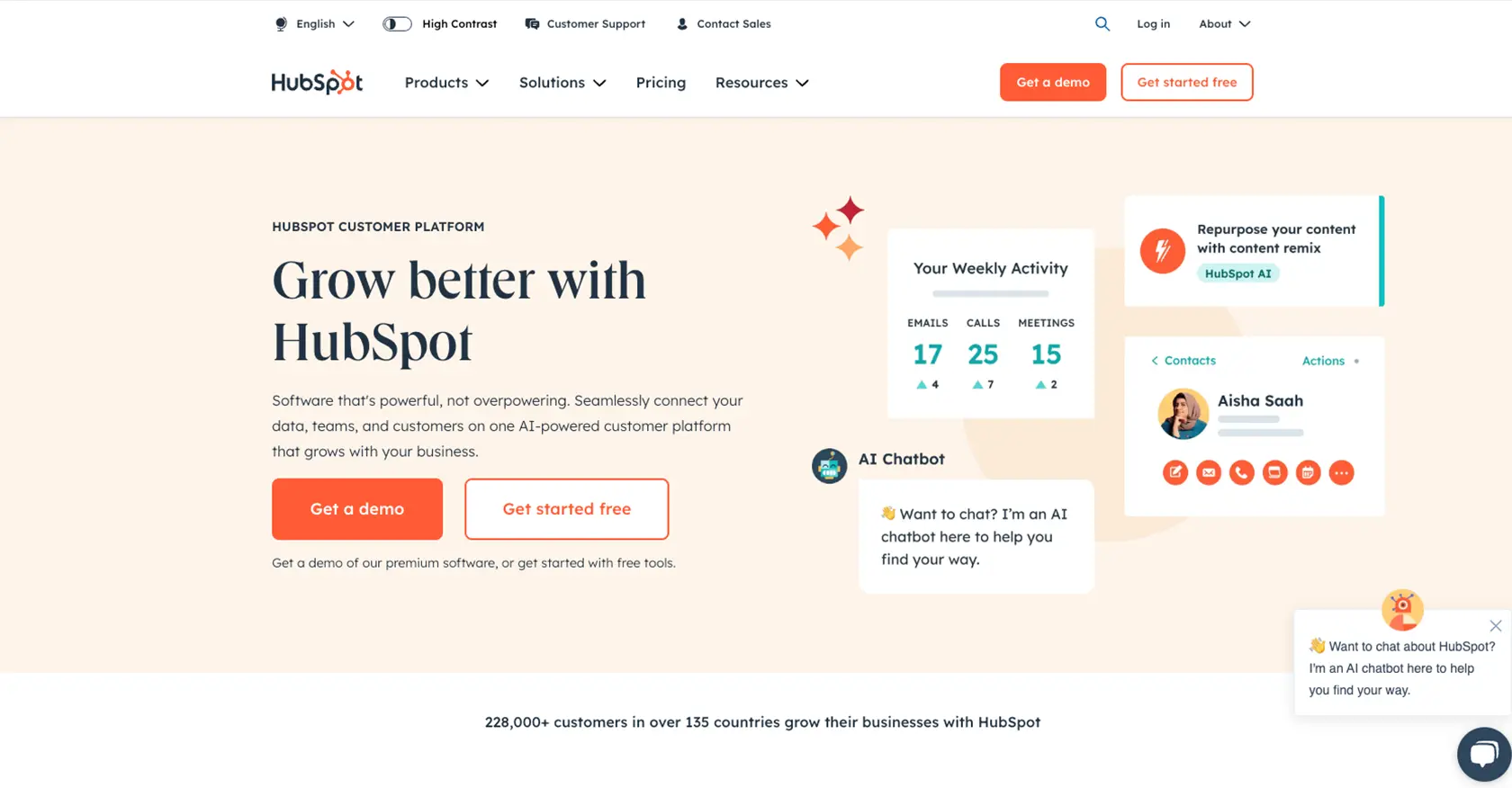
Introduction to HubSpot CRM and API Integration
HubSpot is a comprehensive CRM platform that offers a suite of tools to help businesses manage their marketing, sales, and customer service efforts. Known for its user-friendly interface and robust features, HubSpot is a popular choice for companies looking to streamline their customer relationship management processes.
Developers often integrate with HubSpot's API to access and manipulate data, such as deals, to enhance sales processes. For example, using the HubSpot API, a developer can retrieve deal information to analyze sales trends or automate reporting tasks, providing valuable insights into the sales pipeline.
Setting Up Your HubSpot Sandbox Account for API Integration
Before diving into the HubSpot API to retrieve deals using JavaScript, you'll need to set up a sandbox account. This environment allows you to test API calls without affecting live data, ensuring a safe and controlled development process.
Creating a HubSpot Developer Account
If you don't already have a HubSpot developer account, follow these steps to create one:
- Visit the HubSpot Developer Portal.
- Click on "Create a developer account" and fill in the required information.
- Once your account is created, log in to access the developer dashboard.
Setting Up a HubSpot Sandbox Environment
With your developer account ready, you can now set up a sandbox environment:
- Navigate to the "Test Accounts" section in your developer dashboard.
- Click on "Create a test account" to generate a sandbox environment.
- Follow the prompts to configure your sandbox with the necessary settings.
Configuring OAuth for HubSpot API Access
HubSpot uses OAuth for secure API access. Here's how to configure it:
- In your developer dashboard, go to "Apps" and click "Create a private app."
- Provide a name and description for your app.
- Under "Scopes," select the necessary permissions, such as
crm.objects.deals.read
for accessing deals. - Click "Create app" to generate your OAuth credentials.
- Copy the client ID and client secret for use in your API calls.
For more details on OAuth setup, refer to the HubSpot OAuth Documentation.
Generating an OAuth Access Token
To interact with the HubSpot API, you'll need an OAuth access token:
- Use the client ID and secret to request an access token from HubSpot's OAuth server.
- Include the access token in the authorization header of your API requests.
For a detailed guide on generating tokens, visit the HubSpot API Usage Details.
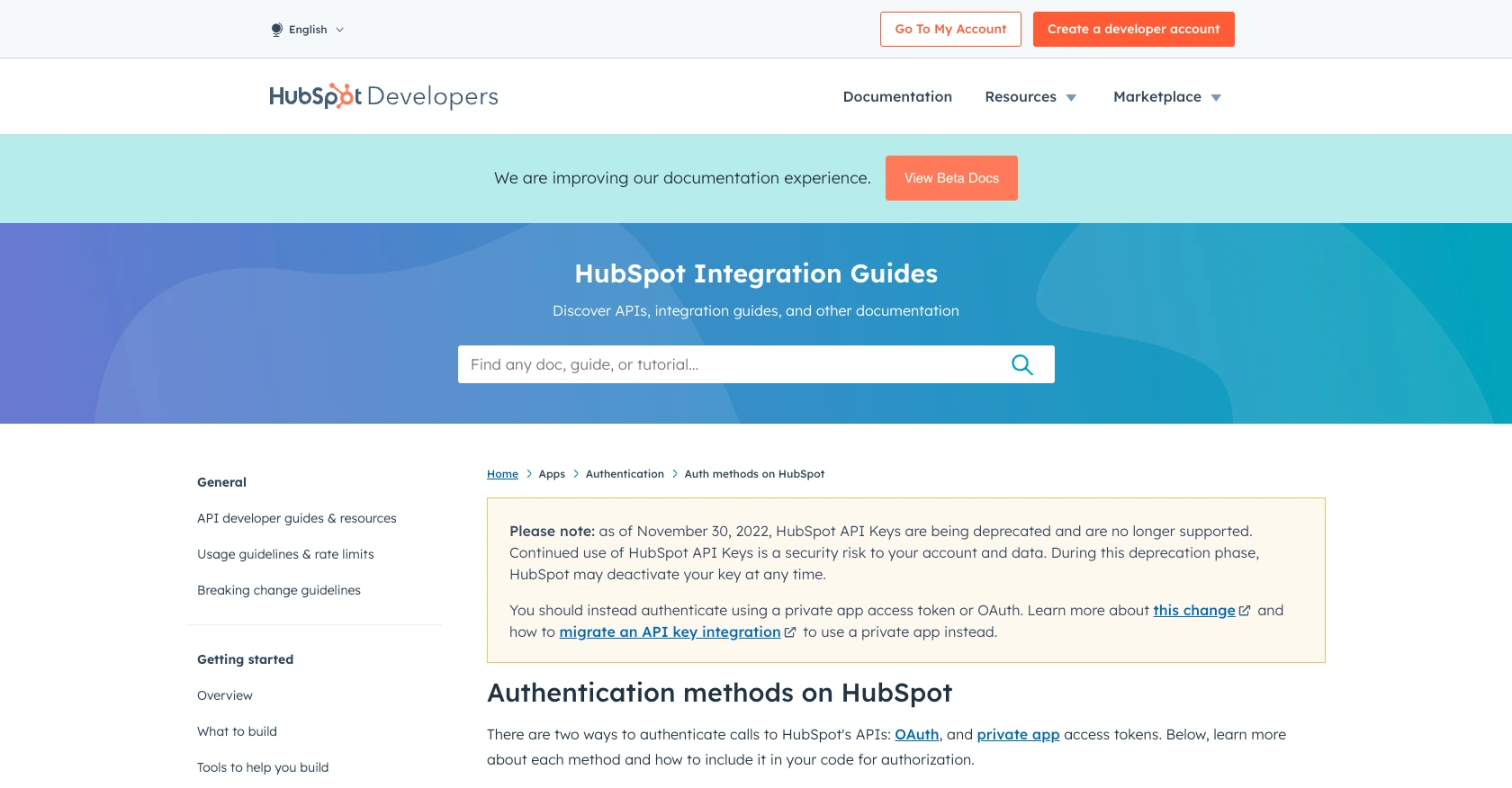
sbb-itb-96038d7
Making API Calls to Retrieve HubSpot Deals Using JavaScript
In this section, we'll explore how to use JavaScript to interact with the HubSpot API to retrieve deal information. This process involves setting up your development environment, writing the code to make API calls, and handling the responses effectively.
Setting Up Your JavaScript Development Environment
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A code editor such as Visual Studio Code.
- The
axios
library for making HTTP requests. Install it using the following command:
npm install axios
Writing JavaScript Code to Retrieve HubSpot Deals
With your environment ready, you can now write the JavaScript code to retrieve deals from HubSpot:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.hubapi.com/crm/v3/objects/deals';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
// Function to get deals
async function getDeals() {
try {
const response = await axios.get(endpoint, { headers });
const deals = response.data.results;
console.log('Retrieved Deals:', deals);
} catch (error) {
console.error('Error fetching deals:', error.response.data);
}
}
// Call the function
getDeals();
Replace YOUR_ACCESS_TOKEN
with the OAuth access token you generated earlier. This code uses the axios
library to make a GET request to the HubSpot API, retrieving deal data and logging it to the console.
Verifying API Call Success and Handling Errors
After running the code, you should see the retrieved deals in your console. To verify the success of your API call, check the response status code:
- A status code of
200
indicates a successful request. - If you encounter a
401
error, ensure your access token is valid and hasn't expired. - A
429
error indicates you've hit the rate limit. HubSpot allows 100 requests every 10 seconds for OAuth apps. Refer to the HubSpot API Usage Details for more information.
Checking Retrieved Data in HubSpot Sandbox
To ensure the data retrieved matches your expectations, log in to your HubSpot sandbox account and navigate to the Deals section. Compare the data displayed in your console with the deals listed in your sandbox environment.
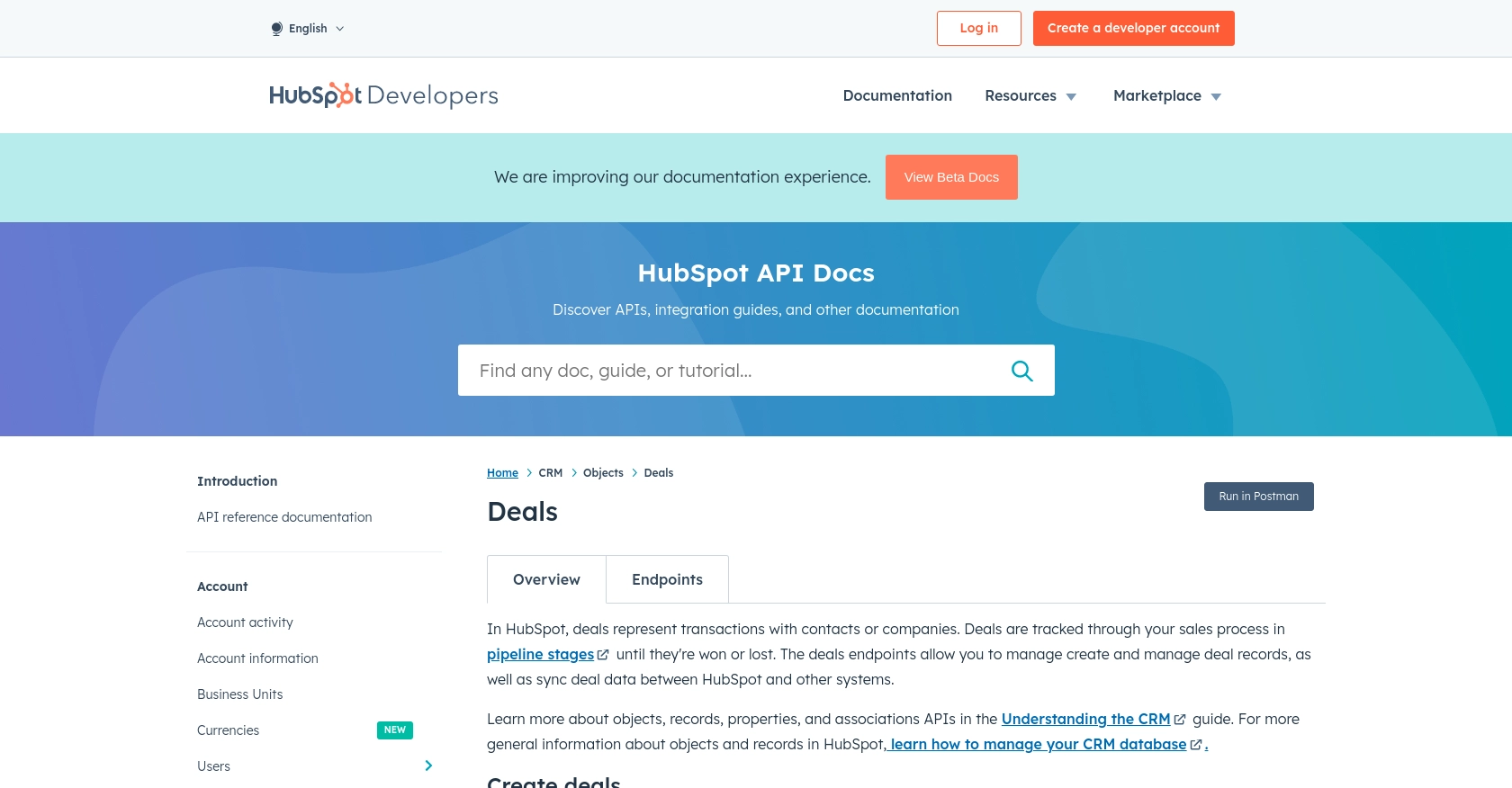
Best Practices for Using HubSpot API in JavaScript
When integrating with the HubSpot API using JavaScript, it's essential to follow best practices to ensure security, efficiency, and maintainability of your application. Here are some key recommendations:
Securely Storing OAuth Credentials
Always store your OAuth credentials securely. Avoid hardcoding them in your source code. Instead, use environment variables or a secure vault to manage sensitive information like client IDs, client secrets, and access tokens.
Handling HubSpot API Rate Limits
HubSpot enforces rate limits to ensure fair usage of its API. For OAuth apps, the limit is 100 requests every 10 seconds. Implement error handling to manage 429
errors gracefully by retrying requests after a delay. Consider using exponential backoff strategies to handle rate limiting efficiently.
Transforming and Standardizing Data Fields
When retrieving data from HubSpot, ensure that you transform and standardize data fields to match your application's requirements. This might involve converting date formats, normalizing text fields, or mapping HubSpot properties to your internal data structures.
Leveraging Endgrate for Seamless HubSpot Integrations
Building and maintaining integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including HubSpot. By using Endgrate, you can:
- Save time and resources by outsourcing integration development and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an easy and intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/deals
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?