Using the MongoDB API to Get Records in PHP
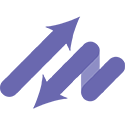
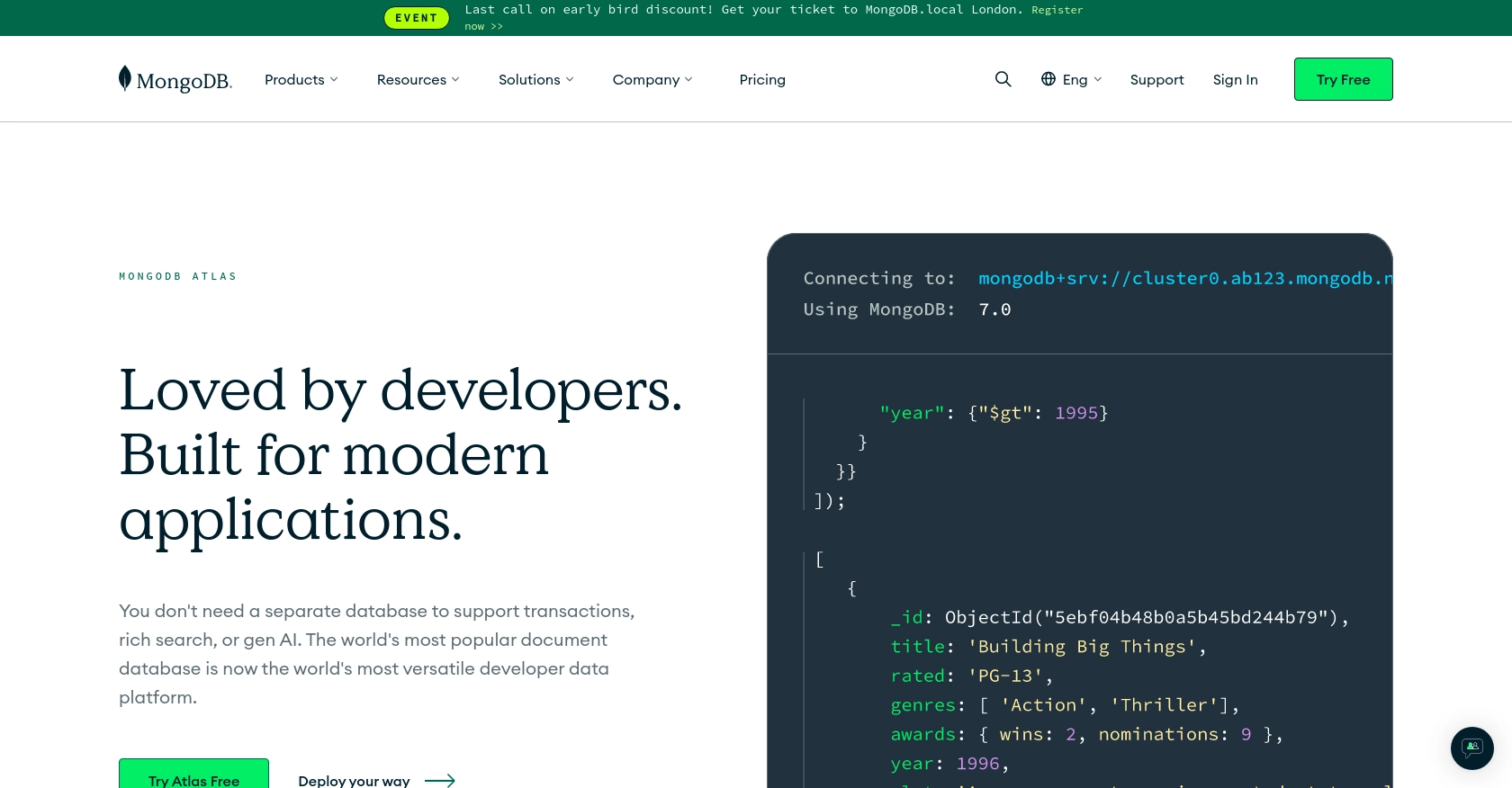
Introduction to MongoDB
MongoDB is a powerful, open-source NoSQL database that provides high performance, high availability, and easy scalability. It is designed to handle large volumes of data and is widely used by developers to build modern applications that require flexible data models and real-time analytics.
Integrating with MongoDB's API allows developers to efficiently manage and retrieve data stored in MongoDB databases. For example, a developer might use the MongoDB API to fetch records for a web application that displays real-time analytics dashboards, enabling users to gain insights from large datasets quickly.
In this article, we will explore how to use PHP to interact with the MongoDB API to retrieve records, providing a step-by-step guide to setting up your environment and executing API calls effectively.
Setting Up Your MongoDB Test Environment
Before you can start interacting with MongoDB using PHP, you'll need to set up a test environment. MongoDB offers several options for developers to get started, including MongoDB Atlas, a fully managed cloud database service, and the MongoDB Community Edition for local development.
Creating a MongoDB Atlas Account
MongoDB Atlas provides a free tier that allows you to create a cloud-based MongoDB database. Follow these steps to set up your account:
- Visit the MongoDB Atlas website and sign up for a free account.
- Once registered, log in to your Atlas account and create a new project.
- Within the project, click on "Build a Cluster" and select the free tier option to create your cluster.
- Follow the prompts to configure your cluster settings and deploy it.
Generating MongoDB API Credentials
To interact with your MongoDB database programmatically, you'll need to generate API credentials:
- Navigate to the "Database Access" section in your MongoDB Atlas dashboard.
- Create a new database user by clicking "Add New Database User."
- Set a username and password, and assign the necessary roles for accessing your database.
- Save the credentials securely, as you'll need them to authenticate your API requests.
Configuring Network Access
Ensure that your application can connect to the MongoDB cluster by configuring network access:
- Go to the "Network Access" section in your Atlas dashboard.
- Click "Add IP Address" and enter your IP address or allow access from anywhere (0.0.0.0/0) for development purposes.
- Save the changes to update your network access settings.
With your MongoDB Atlas account set up and API credentials generated, you are now ready to start making API calls using PHP to retrieve records from your MongoDB database.
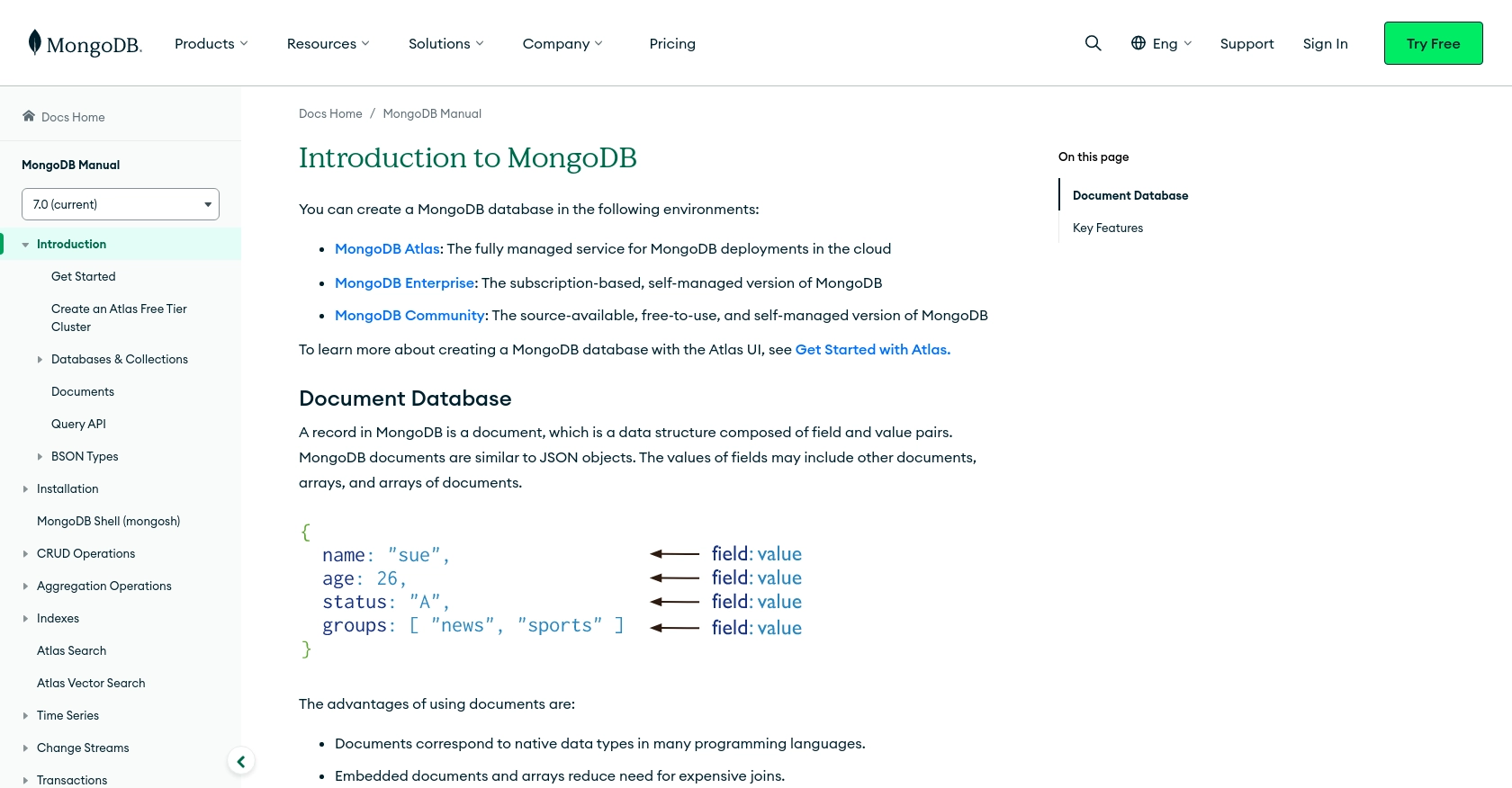
sbb-itb-96038d7
Making API Calls to Retrieve MongoDB Records Using PHP
To interact with MongoDB using PHP, you'll need to set up your environment with the necessary tools and libraries. This section will guide you through the process of making API calls to retrieve records from your MongoDB database.
Setting Up PHP Environment for MongoDB API Integration
Before making API calls, ensure your PHP environment is correctly configured:
- Install PHP 7.4 or later on your machine.
- Install Composer, the PHP dependency manager, if you haven't already.
- Use Composer to install the MongoDB PHP library by running the following command in your terminal:
composer require mongodb/mongodb
Connecting to MongoDB Database Using PHP
Once your environment is set up, you can connect to your MongoDB database using the MongoDB PHP library. Here's a sample script to establish a connection:
<?php
require 'vendor/autoload.php'; // Include Composer's autoloader
$client = new MongoDB\Client("mongodb+srv://<username>:<password>@cluster0.mongodb.net/test?retryWrites=true&w=majority");
$collection = $client->yourDatabaseName->yourCollectionName;
?>
Replace <username>
, <password>
, yourDatabaseName
, and yourCollectionName
with your actual MongoDB credentials and database details.
Fetching Records from MongoDB Collection
With the connection established, you can now fetch records from your MongoDB collection. Below is an example of how to retrieve and display records:
<?php
$cursor = $collection->find();
foreach ($cursor as $document) {
echo json_encode($document);
}
?>
This script uses the find()
method to retrieve all documents from the specified collection and outputs them in JSON format.
Handling Errors and Verifying API Call Success
It's crucial to handle potential errors and verify the success of your API calls. Here's how you can manage errors in your PHP script:
<?php
try {
$cursor = $collection->find();
foreach ($cursor as $document) {
echo json_encode($document);
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
?>
By wrapping the API call in a try-catch
block, you can catch exceptions and display error messages, ensuring your application handles issues gracefully.
After executing the script, verify the retrieved data by checking your MongoDB Atlas dashboard to ensure the records match those in your database.
Best Practices for MongoDB API Integration in PHP
When working with the MongoDB API in PHP, it's essential to follow best practices to ensure efficient and secure integration. Here are some recommendations:
- Securely Store Credentials: Always store your MongoDB credentials securely. Avoid hardcoding them in your scripts. Use environment variables or secure vaults to manage sensitive information.
- Implement Rate Limiting: Be mindful of any rate limits imposed by MongoDB Atlas. Implement logic to handle rate limit errors gracefully, such as retrying requests after a delay.
- Optimize Data Retrieval: Use MongoDB's powerful querying capabilities to fetch only the necessary data. This reduces the load on your database and improves application performance.
- Handle Errors Gracefully: Always use try-catch blocks to handle exceptions and provide meaningful error messages to users. This ensures a smooth user experience even when issues arise.
- Regularly Monitor Performance: Keep an eye on your application's performance and database usage. Use MongoDB Atlas's monitoring tools to identify and resolve potential bottlenecks.
Streamlining MongoDB Integrations with Endgrate
Building and maintaining integrations can be time-consuming and complex, especially when dealing with multiple platforms. Endgrate simplifies this process by providing a unified API endpoint that connects to various services, including MongoDB.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product development while Endgrate handles the intricacies of integration.
- Build Once, Deploy Anywhere: Create a single integration that works across multiple platforms, reducing redundancy and maintenance efforts.
- Enhance User Experience: Offer your customers a seamless and intuitive integration experience with minimal effort.
Explore how Endgrate can transform your integration strategy by visiting Endgrate and discover the benefits of a streamlined integration process.
Read More
Ready to get started?