Using the Nimble API to Create or Update Companies (with PHP examples)
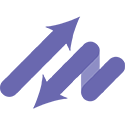
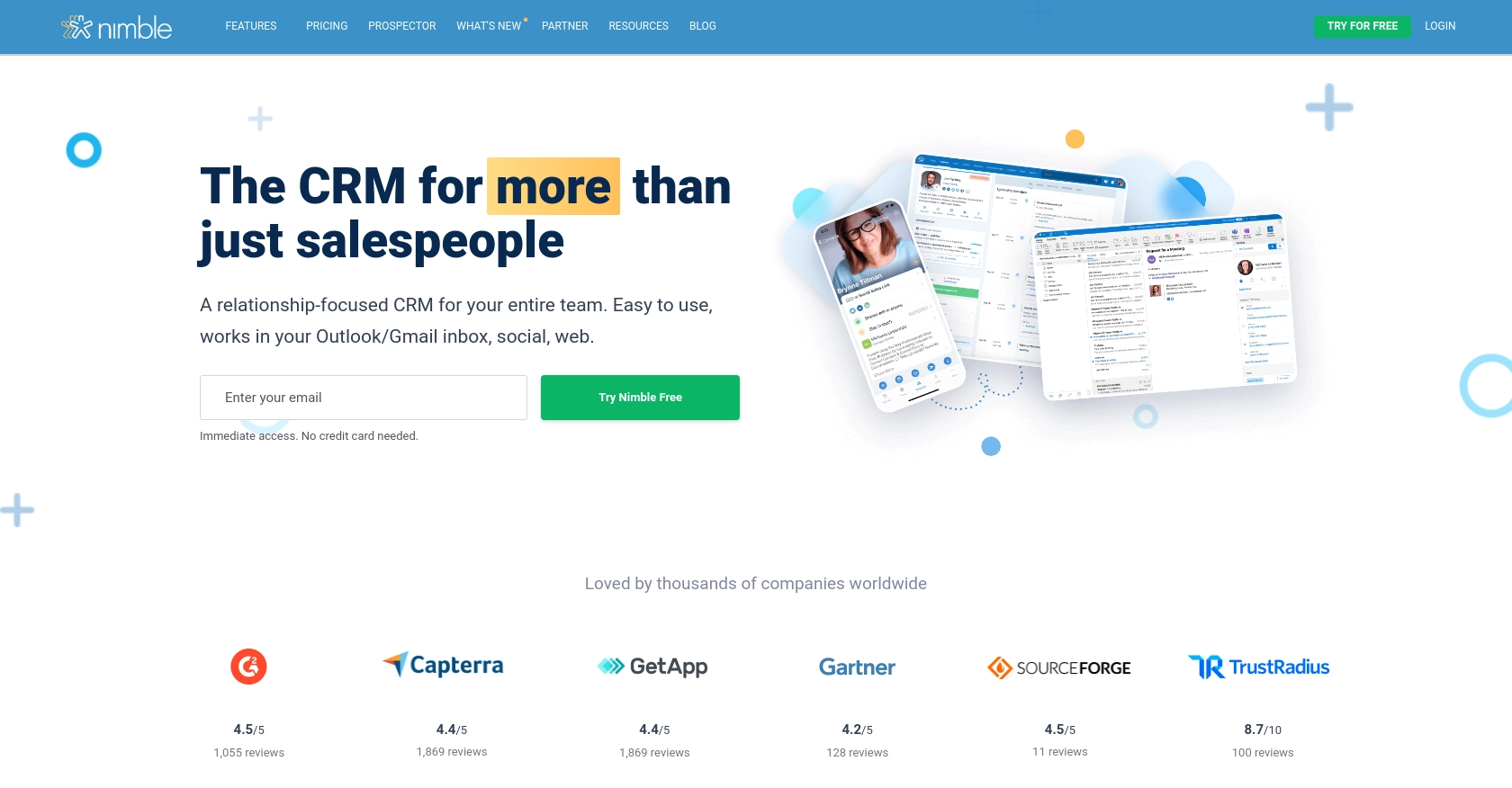
Introduction to Nimble CRM
Nimble is a relationship-focused CRM platform that integrates seamlessly with Microsoft 365 and Google Workspace. It offers a comprehensive suite of tools for managing contacts, tasks, and deals, making it an ideal choice for businesses looking to streamline their customer relationship management processes.
Developers might want to integrate with Nimble's API to automate and enhance their CRM functionalities. For example, using the Nimble API, developers can create or update company records programmatically, ensuring that the CRM data is always up-to-date and reducing manual data entry.
This article will guide you through using PHP to interact with the Nimble API, specifically focusing on creating or updating company records. By following this tutorial, you'll learn how to efficiently manage company data within Nimble, leveraging its API capabilities to enhance your business operations.
Setting Up Your Nimble Test Account and Generating an API Key
Before you can start integrating with the Nimble API, you'll need to set up a Nimble account and generate an API key. This key will allow you to authenticate your requests and interact with the API securely.
Step 1: Create a Nimble Account
If you don't already have a Nimble account, you can sign up for a free trial or use the free version available on the Nimble website. Follow the instructions to create your account and log in.
Step 2: Access API Settings
Once logged in, navigate to the Settings section of your Nimble dashboard. Here, you'll find the option to manage your API access.
Step 3: Generate an API Key
To generate an API key, go to Settings > API Token. Click on Generate New Token and provide a description for your token. When ready, click Generate.
You'll receive an API token, which you should copy and store securely. This token will be used to authenticate your API requests.
Step 4: Set API Permissions
Ensure that your API token has the necessary permissions to create or update company records. You can set these permissions during the token generation process by selecting the appropriate scopes.
Step 5: Verify API Access
To verify that your API key is working, you can make a simple test request to the Nimble API. Use the following PHP code to check your access:
Replace YOUR_API_KEY
with the API key you generated. Running this script should return your account details, confirming that your API access is correctly set up.
For more detailed information on generating and managing API keys, refer to the Nimble API documentation.
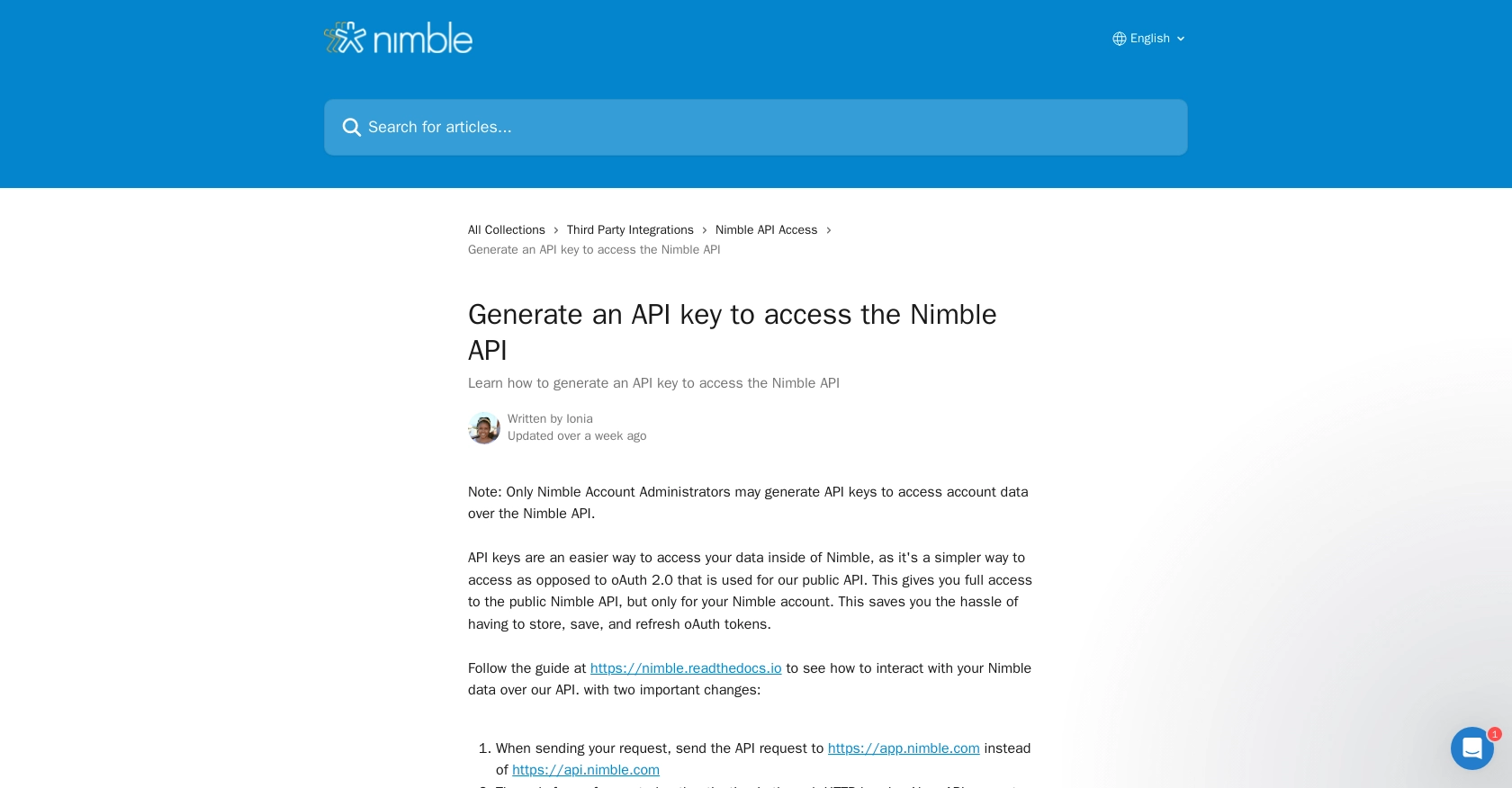
sbb-itb-96038d7
How to Make API Calls to Nimble for Creating or Updating Companies Using PHP
To interact with the Nimble API for creating or updating company records, you need to ensure your PHP environment is set up correctly. This section will guide you through the process of making API calls using PHP, including setting up dependencies and handling responses.
Setting Up PHP Environment for Nimble API Integration
Before making API calls, ensure you have PHP installed on your machine. You can download it from the official PHP website. Additionally, you will need the cURL extension enabled, which is typically included with PHP installations.
Installing Required PHP Dependencies
To make HTTP requests to the Nimble API, you will use the cURL library. Ensure it's enabled in your php.ini
file:
;extension=curl
Uncomment the line by removing the semicolon if it's commented out.
Creating a New Company with Nimble API Using PHP
To create a new company record in Nimble, use the following PHP code. This example demonstrates how to structure your API request:
[
'company name' => [['value' => 'Example Company']],
'description' => [['value' => 'A sample company description']]
],
'record_type' => 'company'
];
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, 'https://app.nimble.com/api/v1/contact');
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Bearer ' . $apiKey,
'Content-Type: application/json'
]);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($companyData));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
?>
Replace YOUR_API_KEY
with your actual API key. This script sends a POST request to create a new company with the specified fields.
Updating an Existing Company Record with Nimble API Using PHP
To update an existing company, you need the company's ID. Use the following PHP code to update a company record:
[
'description' => [['value' => 'Updated company description']]
]
];
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, 'https://app.nimble.com/api/v1/contact/' . $companyId);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Bearer ' . $apiKey,
'Content-Type: application/json'
]);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, 'PUT');
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($updateData));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
?>
Replace YOUR_API_KEY
and COMPANY_ID
with your actual API key and the ID of the company you wish to update. This script sends a PUT request to update the specified company fields.
Handling API Responses and Errors
After executing the API calls, it's crucial to handle the responses and potential errors. Check the HTTP status code and response body to ensure the request was successful:
This code snippet checks if the response code is 200 (success) and prints the response. Adjust your error handling based on the specific needs of your application.
For more detailed information on handling errors and response codes, refer to the Nimble API documentation.
Conclusion and Best Practices for Nimble API Integration
Integrating with the Nimble API using PHP allows developers to efficiently manage company records, enhancing CRM functionalities and automating data processes. By following the steps outlined in this guide, you can create and update company records seamlessly, ensuring your CRM data remains accurate and up-to-date.
Best Practices for Secure and Efficient Nimble API Usage
- Secure API Keys: Always store your API keys securely and avoid hardcoding them in your scripts. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of API rate limits to avoid throttling. Implement retry logic with exponential backoff to manage rate limits effectively.
- Data Standardization: Ensure that data fields are standardized across your application to maintain consistency and avoid errors during API interactions.
- Error Handling: Implement robust error handling to manage API response errors gracefully. Log errors for debugging and monitoring purposes.
Enhance Your Integration Strategy with Endgrate
While integrating with Nimble's API provides powerful capabilities, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes, allowing you to focus on your core product development.
By leveraging Endgrate, you can streamline your integration efforts, reduce development overhead, and provide an intuitive integration experience for your customers. Visit Endgrate to learn more about how you can optimize your integration strategy.
Read More
Ready to get started?