Using the Salesflare API to Get Contacts (with Javascript examples)
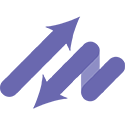
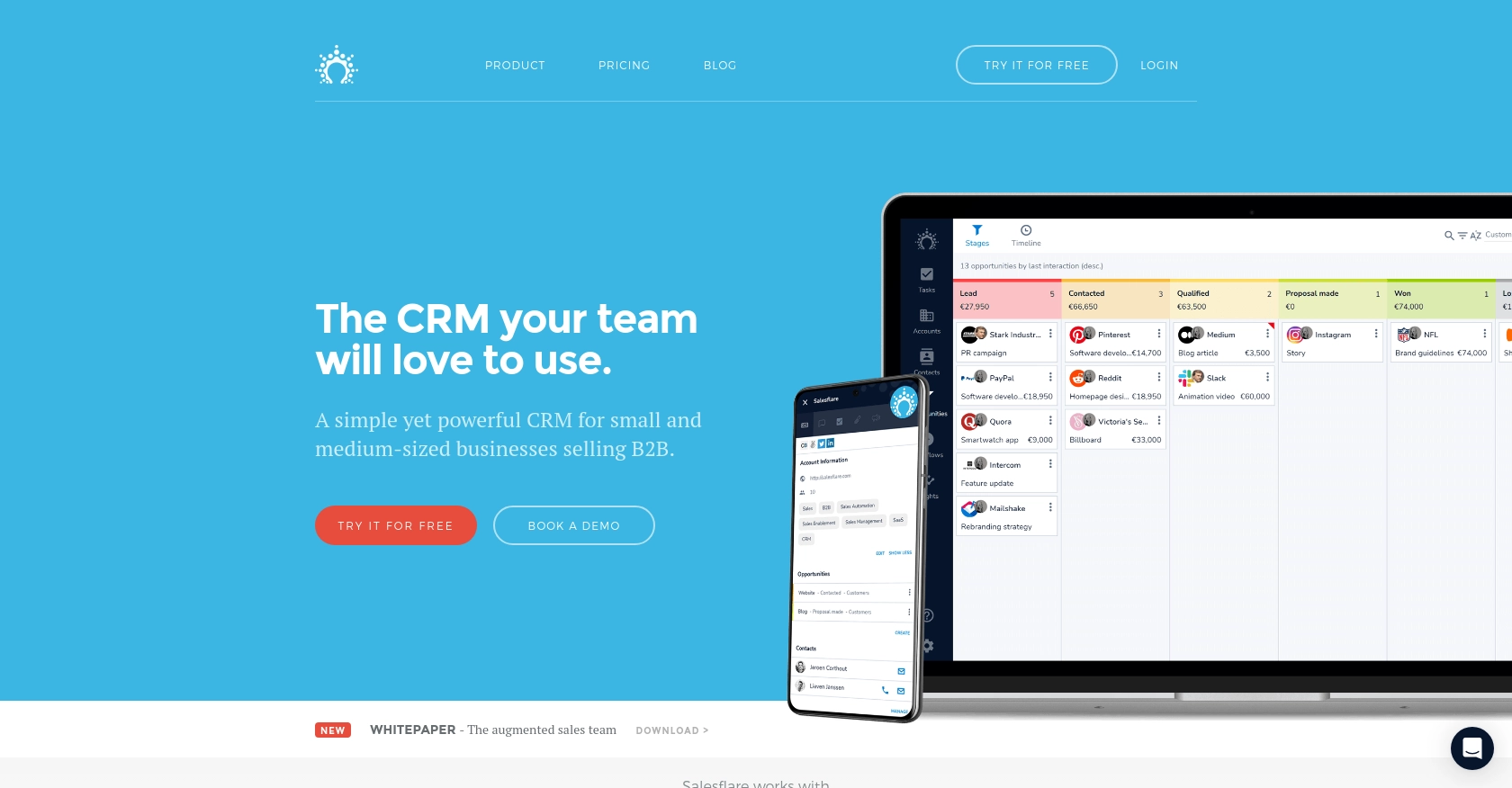
Introduction to Salesflare CRM
Salesflare is a powerful CRM platform designed to simplify and enhance the sales process for small and medium-sized businesses. With its intuitive interface and automation capabilities, Salesflare helps sales teams manage leads, track customer interactions, and close deals more efficiently.
Integrating with Salesflare's API allows developers to access and manage customer data seamlessly. For example, you might want to retrieve contact information from Salesflare to synchronize it with another application, ensuring that your sales and marketing efforts are aligned and up-to-date.
Setting Up Your Salesflare Test Account and API Key
Before you can start interacting with the Salesflare API, you need to set up a test account and obtain an API key. This will allow you to authenticate your requests and access the necessary data.
Create a Salesflare Account
If you don't already have a Salesflare account, you can sign up for a free trial on the Salesflare website. This will give you access to the platform and allow you to explore its features.
- Visit the Salesflare website and click on the "Sign Up" button.
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the Salesflare dashboard.
Generate Your Salesflare API Key
Salesflare uses API key-based authentication to secure its API. Follow these steps to generate your API key:
- Log in to your Salesflare account and navigate to the settings page.
- Look for the "API" or "Integrations" section in the settings menu.
- Click on "Generate API Key" to create a new key.
- Copy the generated API key and store it securely, as you'll need it to authenticate your API requests.
For more detailed information, refer to the Salesflare API documentation.
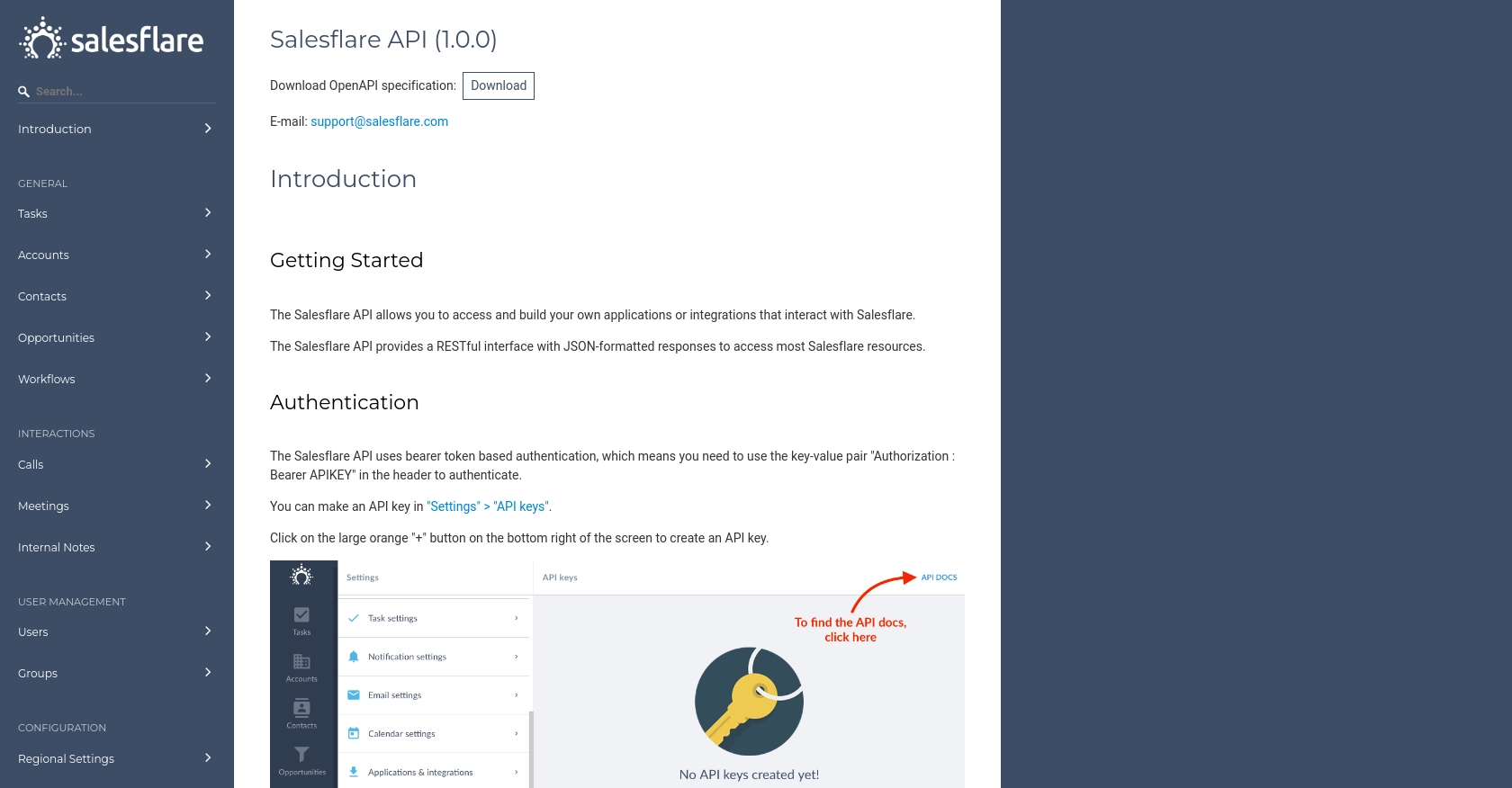
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Salesflare Using JavaScript
To interact with the Salesflare API and retrieve contact information, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment
Before you begin, ensure you have the following prerequisites installed on your machine:
- Node.js (version 14 or later)
- NPM (Node Package Manager)
Once you have these installed, open your terminal and create a new project directory:
mkdir salesflare-api-integration
cd salesflare-api-integration
Initialize a new Node.js project:
npm init -y
Install the Axios library to make HTTP requests:
npm install axios
Writing the JavaScript Code to Fetch Contacts
Create a new file named getContacts.js
and add the following code:
const axios = require('axios');
// Replace 'Your_API_Key' with your actual Salesflare API key
const apiKey = 'Your_API_Key';
// Set the API endpoint
const endpoint = 'https://api.salesflare.com/v1/contacts';
// Configure the request headers
const headers = {
'Authorization': `Bearer ${apiKey}`,
'Content-Type': 'application/json'
};
// Function to fetch contacts
async function fetchContacts() {
try {
const response = await axios.get(endpoint, { headers });
const contacts = response.data;
console.log('Contacts retrieved successfully:', contacts);
} catch (error) {
console.error('Error fetching contacts:', error.response ? error.response.data : error.message);
}
}
// Call the function
fetchContacts();
In this code, you use Axios to send a GET request to the Salesflare API endpoint for contacts. Make sure to replace Your_API_Key
with the API key you generated earlier. The response data is logged to the console, and any errors are caught and displayed.
Running the JavaScript Code
To execute the code, run the following command in your terminal:
node getContacts.js
If successful, you should see the list of contacts retrieved from Salesflare displayed in your terminal.
Handling Errors and Verifying API Requests
When making API calls, it's important to handle potential errors gracefully. The code above checks for errors and logs them to the console. Common error codes include:
- 401 Unauthorized: Check if your API key is correct.
- 404 Not Found: Verify the endpoint URL.
- 500 Internal Server Error: Try the request again later.
To verify that your request succeeded, you can log into your Salesflare account and check the contacts section to ensure the data matches the output from your API call.
Conclusion and Best Practices for Using Salesflare API with JavaScript
Integrating with the Salesflare API using JavaScript can significantly enhance your ability to manage and synchronize contact data across platforms. By following the steps outlined in this guide, you can efficiently retrieve contacts and handle any potential errors that may arise during the process.
Best Practices for Secure and Efficient API Integration
- Secure API Key Storage: Always store your API keys securely and avoid hardcoding them directly into your source code. Consider using environment variables or a secure vault.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the Salesflare API. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from Salesflare is transformed and standardized to fit your application's requirements, maintaining consistency across systems.
Streamlining Integrations with Endgrate
While integrating with Salesflare's API can be straightforward, managing multiple integrations across different platforms can become complex and time-consuming. This is where Endgrate can be a valuable asset.
Endgrate offers a unified API endpoint that connects to various platforms, including Salesflare. By using Endgrate, you can streamline your integration processes, reduce development time, and focus on your core product. With Endgrate, you build once for each use case and leverage an intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration needs by visiting Endgrate today.
Read More
Ready to get started?