How to Create or Update Customers with the Sage Accounting API in PHP
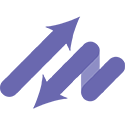
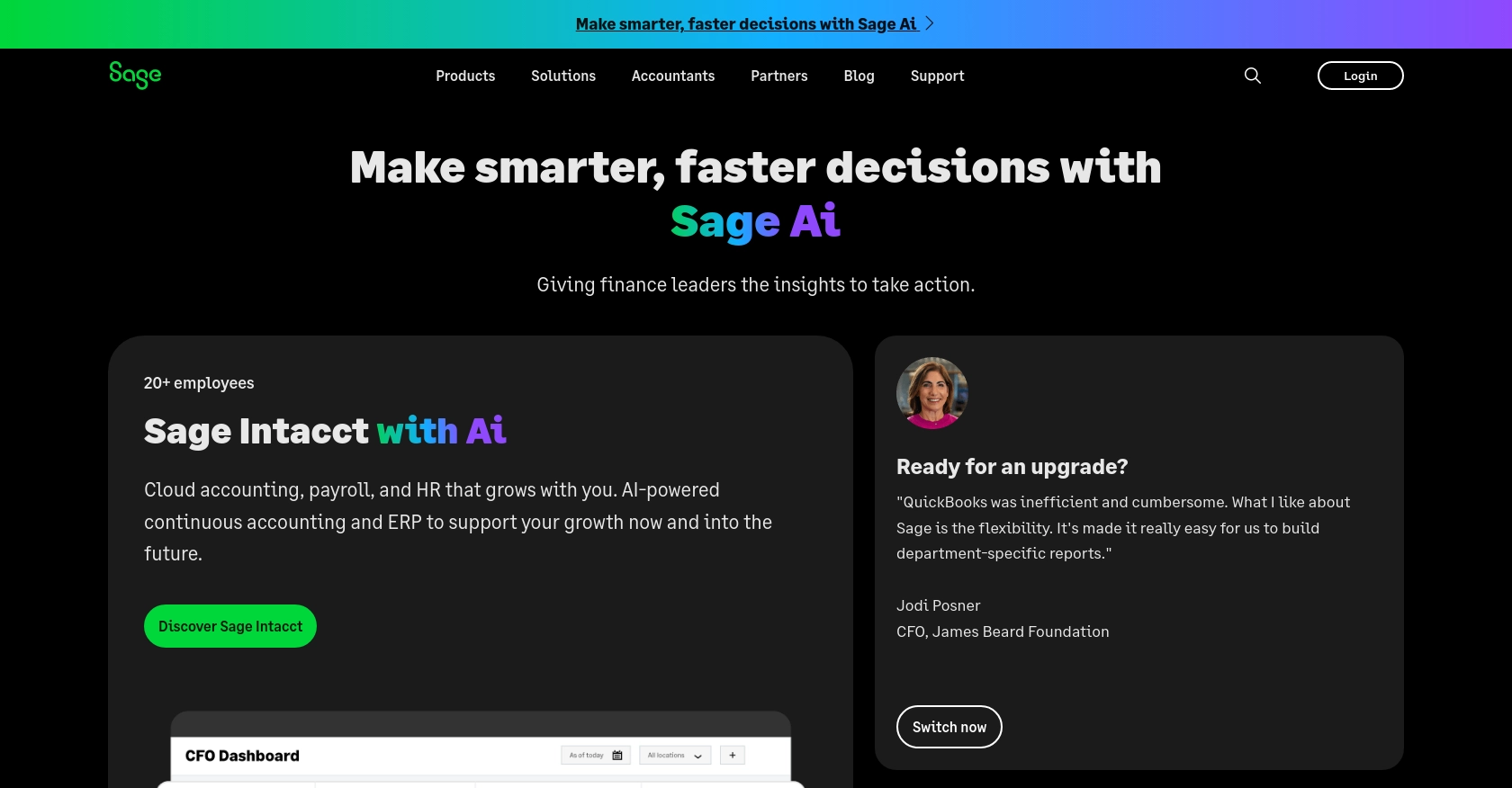
Introduction to Sage Accounting API
Sage Accounting is a robust cloud-based accounting solution tailored for small to medium-sized businesses. It offers a comprehensive suite of tools to manage financial transactions, invoicing, and customer relationships, making it an essential platform for businesses aiming to streamline their accounting processes.
Integrating with the Sage Accounting API allows developers to automate and enhance financial operations, such as creating or updating customer records. For example, a developer might use the Sage Accounting API to automatically update customer details from an external CRM system, ensuring that all financial data remains accurate and up-to-date.
Setting Up Your Sage Accounting Test/Sandbox Account
Before you can start integrating with the Sage Accounting API, it's essential to set up a test or sandbox account. This environment allows you to safely develop and test your integration without affecting live data.
Create a Sage Developer Account
To begin, you'll need a Sage Developer account. This account enables you to register and manage your applications, obtain client credentials, and configure essential settings like callback URLs.
- Visit the Sage Developer Portal.
- Sign up using your GitHub account or an email address.
- Follow the guide on creating an app to complete your registration.
Set Up a Trial Business for Development
Sage Accounting allows you to create trial businesses for development purposes. This setup is crucial for testing your integration across different subscription tiers and regions.
- Navigate to the trial business setup page.
- Select your region and the appropriate subscription tier (e.g., Accounting Start, Accounting Standard).
- Use an email service that supports aliasing to manage multiple environments efficiently.
Create a Sage App and Obtain OAuth Credentials
With your developer account and trial business ready, the next step is to create a Sage app to obtain OAuth credentials, which are necessary for authenticating API requests.
- Log in to your Sage Developer account.
- Click on "Create App" and provide a name and callback URL for your app.
- Save your app to generate a Client ID and Client Secret, which you'll use for OAuth authentication.
For more detailed instructions, refer to the Sage Developer documentation.
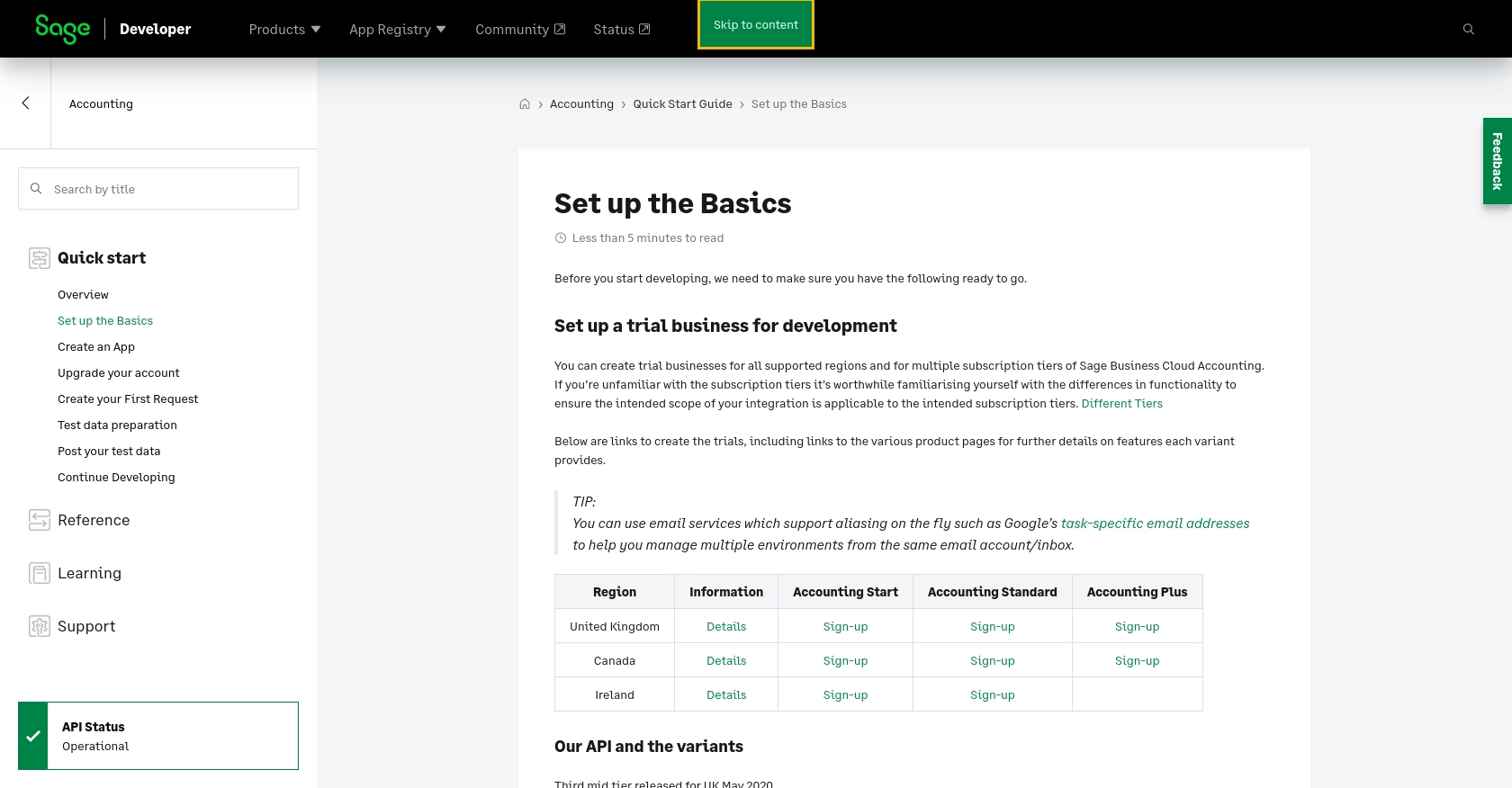
sbb-itb-96038d7
Making API Calls to Create or Update Customers with Sage Accounting API in PHP
To interact with the Sage Accounting API using PHP, you'll need to set up your environment and write code to handle API requests. This section will guide you through the process of making API calls to create or update customer records in Sage Accounting.
Setting Up Your PHP Environment
Before making API calls, ensure your PHP environment is correctly configured. You'll need:
- PHP 7.4 or later
- Composer for dependency management
- The
guzzlehttp/guzzle
library for making HTTP requests
Install the Guzzle library using Composer:
composer require guzzlehttp/guzzle
Creating or Updating Customers with Sage Accounting API
Now, let's write the PHP code to create or update a customer using the Sage Accounting API. You'll need your OAuth credentials (Client ID and Client Secret) for authentication.
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token'; // Replace with your OAuth access token
// Define the API endpoint
$endpoint = 'https://api.sage.com/accounting/contacts';
// Set the request headers
$headers = [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json'
];
// Define the customer data
$customerData = [
'contact' => [
'name' => 'John Doe',
'email' => 'john.doe@example.com',
'telephone' => '123-456-7890'
]
];
try {
// Make the POST request to create or update the customer
$response = $client->post($endpoint, [
'headers' => $headers,
'json' => $customerData
]);
// Check if the request was successful
if ($response->getStatusCode() == 201) {
echo "Customer created or updated successfully.";
} else {
echo "Failed to create or update customer.";
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace Your_Access_Token
with the OAuth token obtained during authentication. The above code uses Guzzle to send a POST request to the Sage Accounting API, creating or updating a customer record with the specified data.
Verifying API Call Success
To verify that the customer was created or updated successfully, check the response status code. A status code of 201 indicates success. You can also log into your Sage Accounting sandbox account to confirm the changes.
Handling Errors and Common Error Codes
When making API calls, it's crucial to handle potential errors. Common error codes include:
- 400 Bad Request: The request was invalid. Check your data and try again.
- 401 Unauthorized: Authentication failed. Verify your OAuth credentials.
- 404 Not Found: The specified resource could not be found.
- 500 Internal Server Error: An error occurred on the server. Try again later.
For more detailed error handling, refer to the Sage Developer documentation.
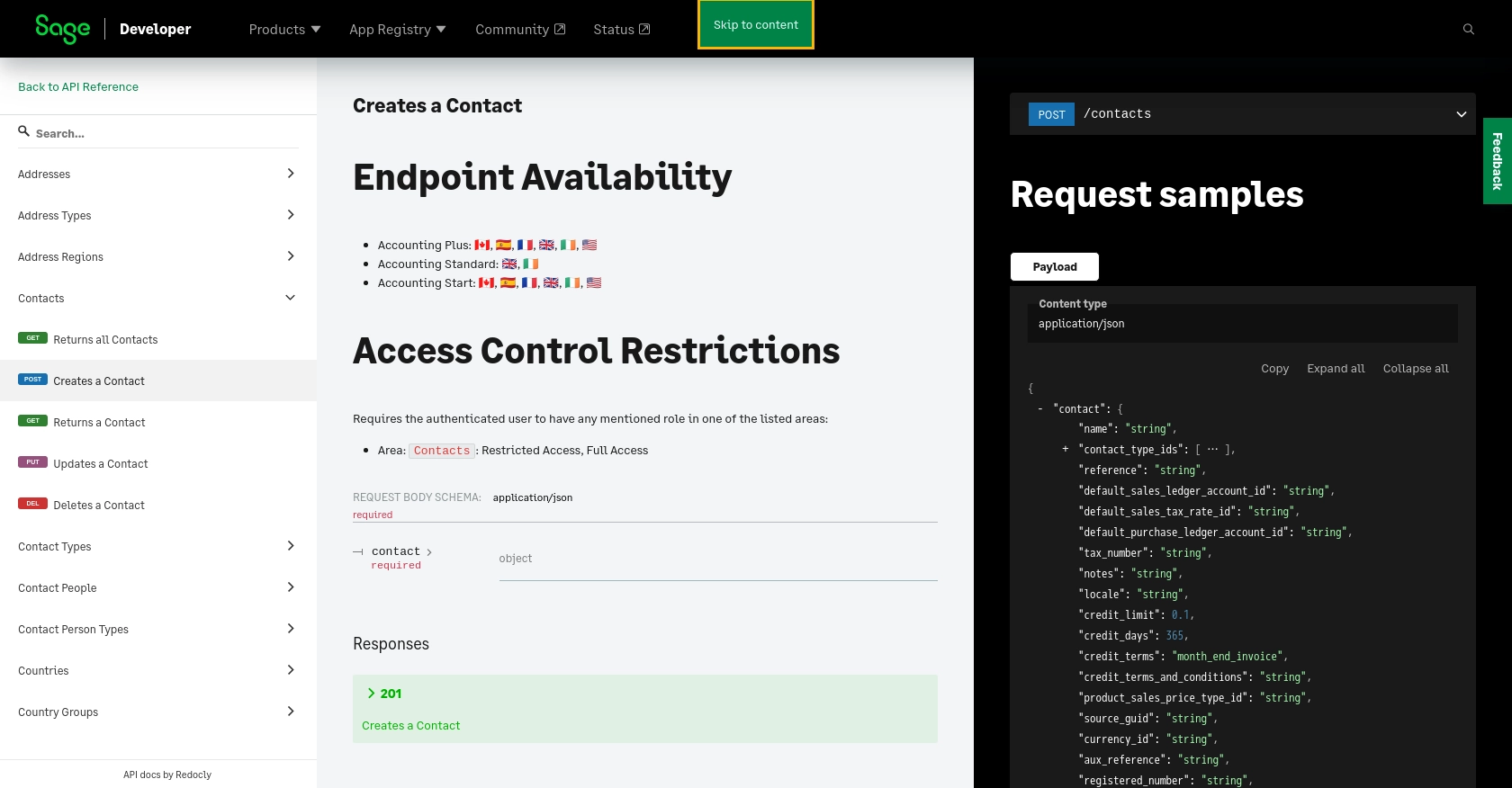
Conclusion and Best Practices for Using Sage Accounting API in PHP
Integrating with the Sage Accounting API using PHP can significantly enhance your financial operations by automating customer data management. By following the steps outlined in this guide, you can efficiently create or update customer records, ensuring your financial data remains accurate and up-to-date.
Best Practices for Secure and Efficient API Integration
- Securely Store OAuth Credentials: Always store your Client ID, Client Secret, and access tokens securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of API rate limits to avoid throttling. Implement exponential backoff strategies to handle retries gracefully.
- Standardize Data Formats: Ensure that customer data is consistently formatted before making API calls. This reduces errors and improves data integrity.
- Implement Robust Error Handling: Use try-catch blocks to manage exceptions and log errors for troubleshooting. Refer to the Sage Developer documentation for detailed error code explanations.
Streamline Your Integrations with Endgrate
While integrating with Sage Accounting API can be straightforward, managing multiple integrations can become complex. Endgrate simplifies this process by providing a unified API endpoint for various platforms, including Sage Accounting. By leveraging Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/sage
- https://developer.sage.com/accounting/quick-start/set-up-the-basics/
- https://developer.sage.com/accounting/quick-start/create-an-app/
- https://developer.sage.com/accounting/quick-start/upgrade-your-account/
- https://developer.sage.com/accounting/reference/contacts/#tag/Contacts/operation/postContacts
Ready to get started?