How to Get Companies with the Cloze API in Python
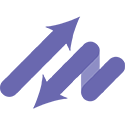
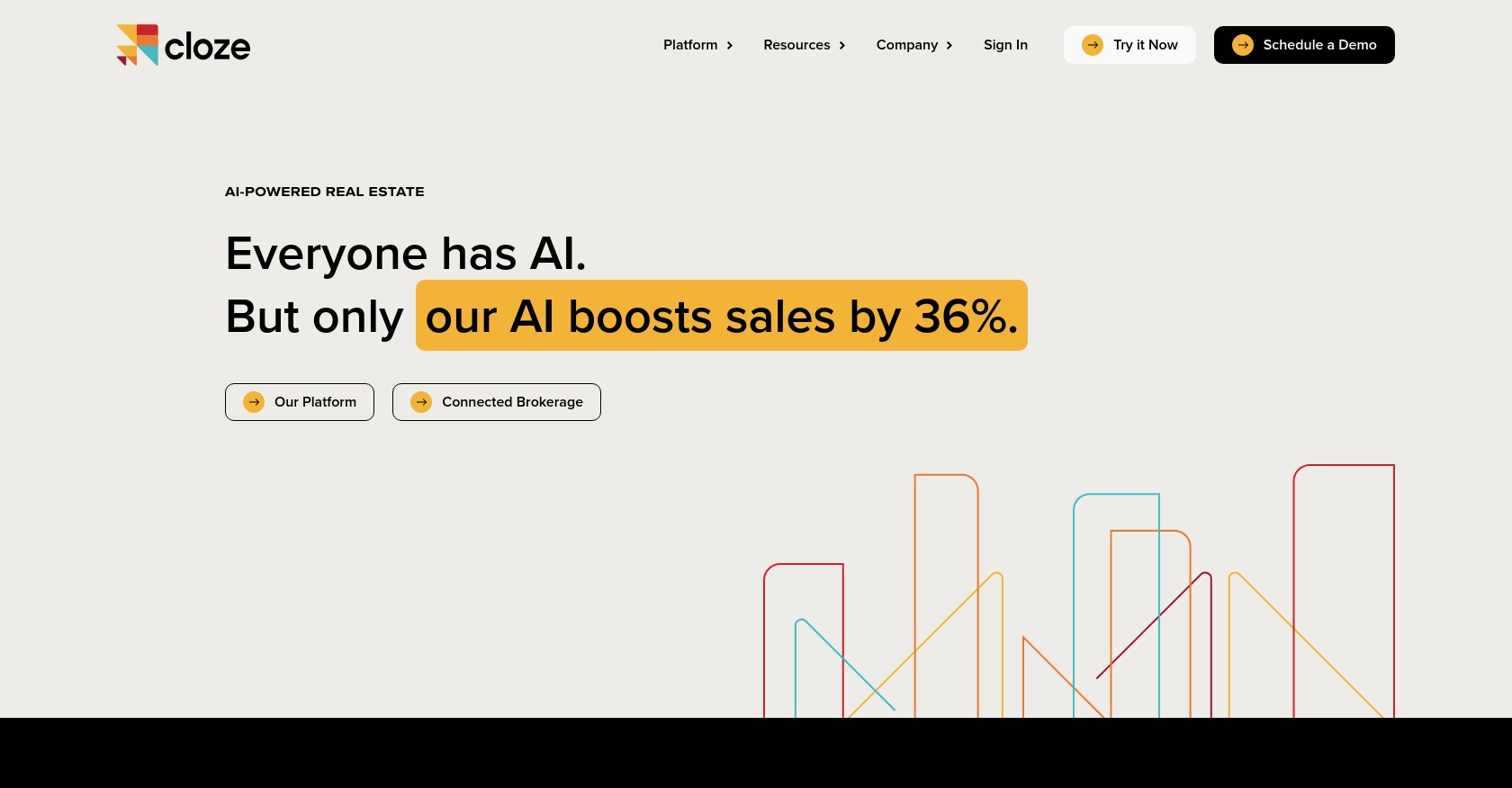
Introduction to Cloze API for Company Data Integration
Cloze is a powerful relationship management platform that helps businesses manage interactions with clients, partners, and prospects. It offers a comprehensive suite of tools designed to streamline communication and enhance productivity by organizing emails, calls, meetings, and social media interactions in one place.
Integrating with the Cloze API allows developers to access and manage company data efficiently. This can be particularly useful for automating tasks such as retrieving company information to enhance CRM systems or synchronizing data across platforms. For example, a developer might use the Cloze API to fetch company details and integrate them into a custom dashboard for sales teams, providing real-time insights and improving decision-making processes.
Setting Up Your Cloze API Test Account
Before you can start integrating with the Cloze API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Create a Cloze Account
- Visit the Cloze website and sign up for a free account.
- Follow the on-screen instructions to complete the registration process.
- Once your account is created, log in to access the Cloze dashboard.
Generate an API Key for Cloze Integration
To authenticate your API requests, you'll need an API key. Here's how to generate one:
- In the Cloze dashboard, navigate to the settings section.
- Look for the API settings or developer options.
- Follow the instructions to create a new API key. You may need to provide a name for the key and specify access permissions.
- Once generated, copy the API key and store it securely. You'll use this key to authenticate your API requests.
Configure API Key Authentication
With your API key ready, you can now configure your application to authenticate API requests:
- Set the
user
parameter to your Cloze account email address. - Set the
api_key
parameter to the API key you generated. - These parameters will be included in your API requests to authenticate with the Cloze API.
For more detailed instructions, refer to the Cloze API documentation.
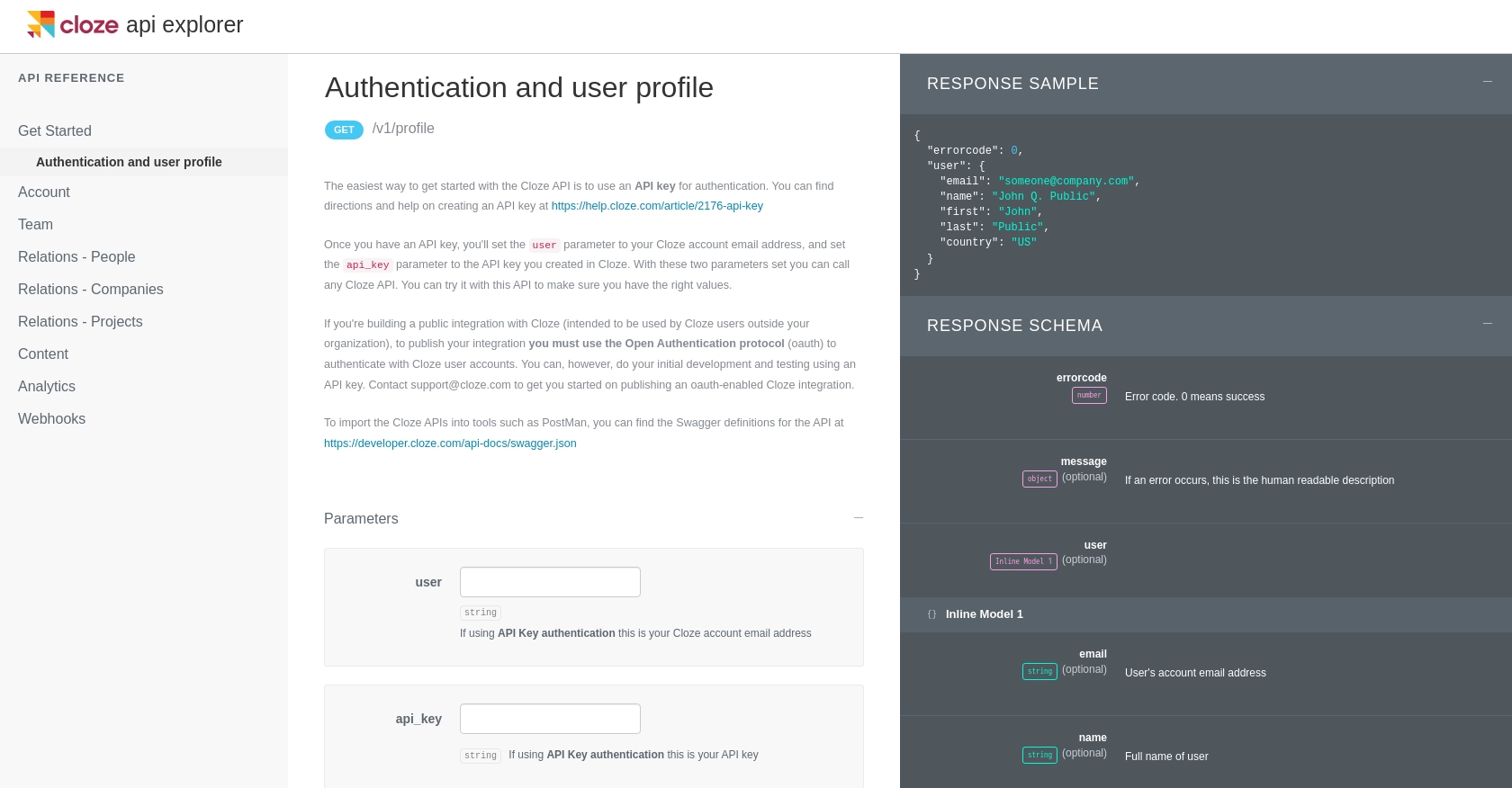
sbb-itb-96038d7
How to Make API Calls to Retrieve Company Data Using Cloze API in Python
In this section, we'll walk through the process of making API calls to the Cloze API using Python to retrieve company data. This will involve setting up your Python environment, writing the code to interact with the API, and handling the response effectively.
Setting Up Your Python Environment for Cloze API Integration
Before making API calls, ensure you have the necessary tools and libraries installed:
- Python 3.11.1 or later
- The Python package installer
pip
Install the required requests
library by running the following command in your terminal:
pip install requests
Writing Python Code to Fetch Company Data from Cloze API
Create a new Python file named get_cloze_companies.py
and add the following code:
import requests
# Define the API endpoint and parameters
endpoint = "https://api.cloze.com/v1/companies/find"
params = {
"user": "your_email@domain.com",
"api_key": "Your_API_Key",
"pagesize": 10,
"pagenumber": 1
}
# Make a GET request to the Cloze API
response = requests.get(endpoint, params=params)
# Check if the request was successful
if response.status_code == 200:
companies = response.json().get("companies", [])
for company in companies:
print(f"Company Name: {company.get('name')}")
else:
print(f"Failed to retrieve companies: {response.status_code} - {response.text}")
Replace Your_API_Key
and your_email@domain.com
with your actual API key and email address.
Understanding and Handling the API Response
When the API call is successful, the response will contain a list of companies. The code above prints the name of each company. If the request fails, it will print an error message with the status code and response text.
Verifying API Call Success in Cloze Sandbox
To ensure the API call was successful, you can verify the returned data against your Cloze sandbox account. The companies listed in the response should match those in your test environment.
Error Handling and Troubleshooting Cloze API Requests
It's important to handle potential errors when making API calls. Common error codes include:
- 400 Bad Request: Check your request parameters for errors.
- 401 Unauthorized: Verify your API key and email address.
- 500 Internal Server Error: Try the request again later.
For more detailed error information, refer to the Cloze API documentation.
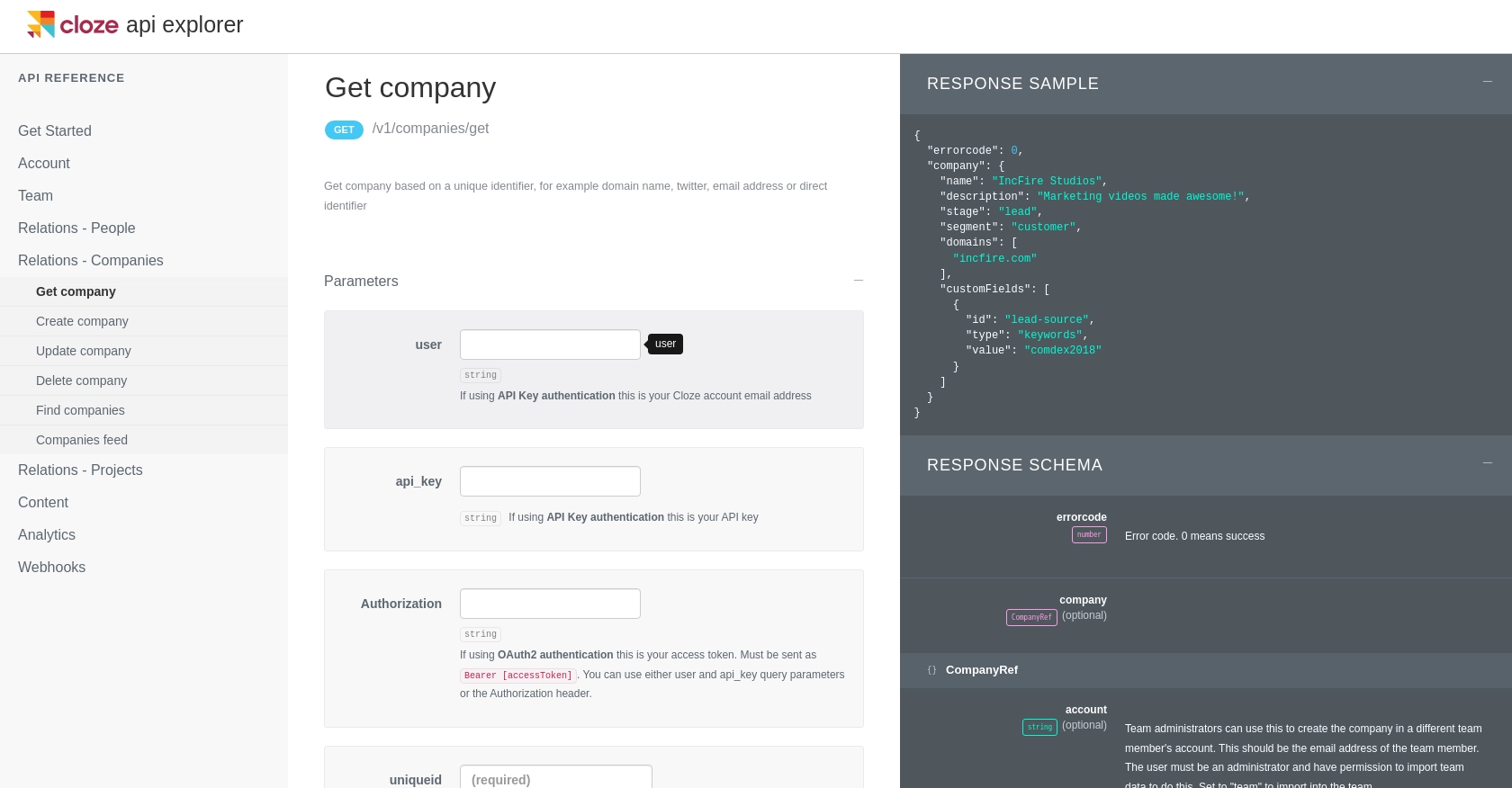
Conclusion and Best Practices for Using the Cloze API in Python
Integrating with the Cloze API provides a powerful way to manage company data efficiently, enhancing your CRM systems and automating data synchronization across platforms. By following the steps outlined in this guide, you can effectively retrieve company information using Python, ensuring seamless integration with your existing systems.
Best Practices for Secure and Efficient Cloze API Integration
- Secure API Key Storage: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data retrieved from the Cloze API is standardized and transformed as needed to fit your application's data model.
- Error Handling: Implement robust error handling to manage API call failures and provide meaningful feedback to users or logs for troubleshooting.
Streamlining Integration Development with Endgrate
For developers looking to simplify the integration process, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and leverage an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can streamline your integration development process.
Read More
Ready to get started?