Using the Quickbooks API to Create Or Update Purchase Orders (with PHP examples)
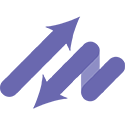
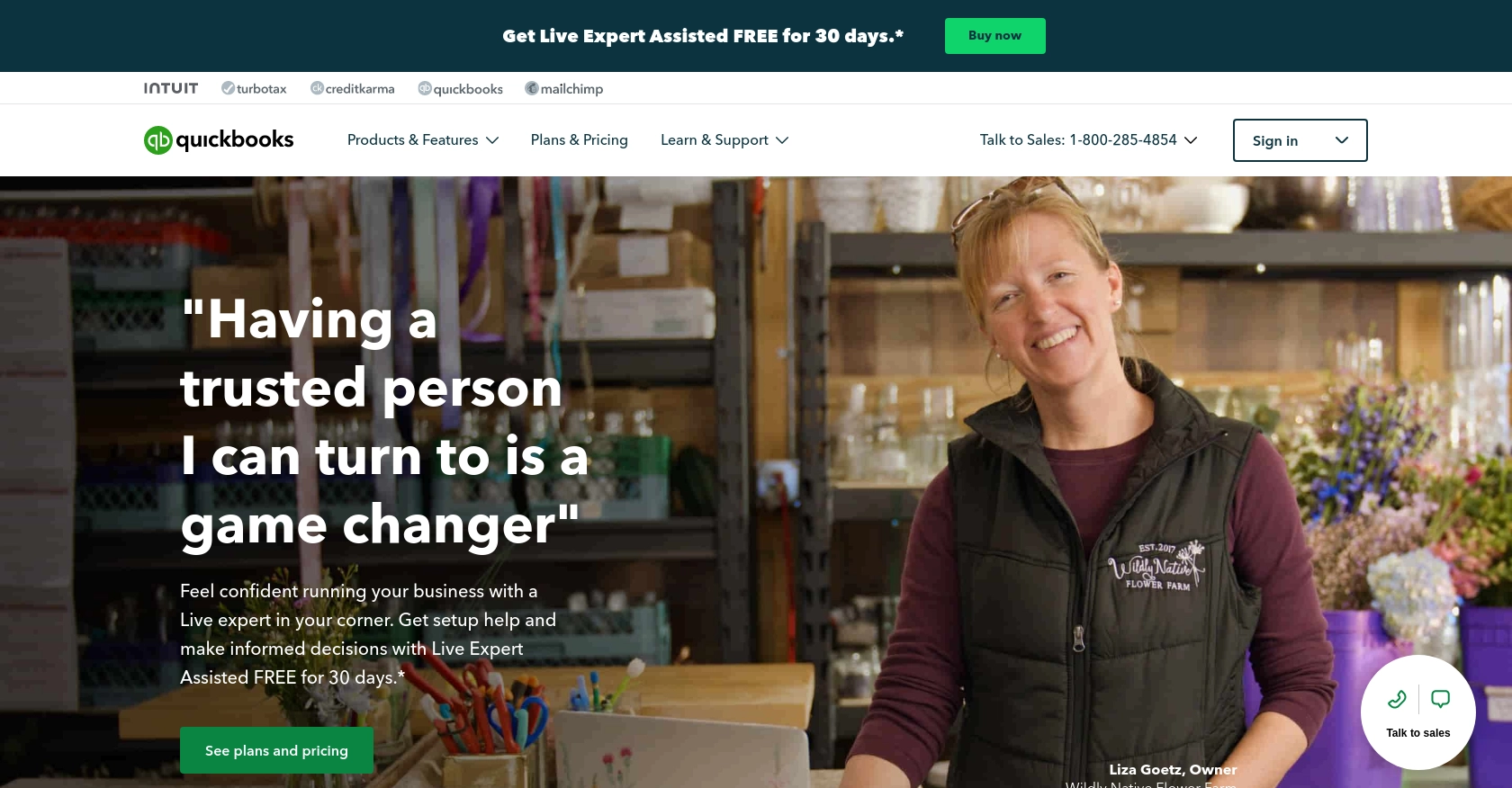
Introduction to QuickBooks API for Purchase Orders
QuickBooks is a widely-used accounting software that offers comprehensive solutions for managing business finances. It provides tools for invoicing, expense tracking, payroll, and more, making it a preferred choice for small to medium-sized businesses.
Integrating with the QuickBooks API allows developers to automate financial processes, such as creating or updating purchase orders. This can streamline operations by ensuring that purchase orders are accurately recorded and managed within the QuickBooks system. For example, a developer might use the QuickBooks API to automatically generate purchase orders from an e-commerce platform, reducing manual data entry and minimizing errors.
Setting Up a QuickBooks Test/Sandbox Account for API Integration
Before you can begin integrating with the QuickBooks API, you need to set up a test or sandbox account. This environment allows you to safely develop and test your application without affecting live data. QuickBooks provides a sandbox account that mimics the live environment, enabling you to experiment with API calls and workflows.
Creating a QuickBooks Developer Account
To get started, you need to create a QuickBooks Developer account. Follow these steps:
- Visit the QuickBooks Developer Portal.
- Click on "Sign Up" to create a new account or "Log In" if you already have one.
- Fill in the required information and verify your email address to activate your account.
Setting Up a QuickBooks Sandbox Company
Once your developer account is ready, you can set up a sandbox company:
- Log in to your QuickBooks Developer account.
- Navigate to the "Sandbox" section in the dashboard.
- Click on "Add Sandbox" to create a new sandbox company.
- Select the type of company you want to simulate and complete the setup process.
Creating an App for OAuth Authentication
QuickBooks API uses OAuth 2.0 for authentication. You need to create an app to obtain the necessary credentials:
- Go to the App Management section in your developer account.
- Click on "Create an App" and select "QuickBooks Online and Payments" as the platform.
- Fill in the app details, such as name and description.
- Once the app is created, navigate to the "Keys & OAuth" section to obtain your Client ID and Client Secret.
Configuring OAuth Redirect URIs
To ensure proper OAuth flow, configure your redirect URIs:
- In the app settings, locate the "Redirect URIs" section.
- Add the URIs where you want QuickBooks to redirect after authentication.
- Ensure these URIs match the ones used in your application code.
With your sandbox account and app set up, you're ready to start making API calls to QuickBooks. This setup allows you to test creating and updating purchase orders without impacting real data.
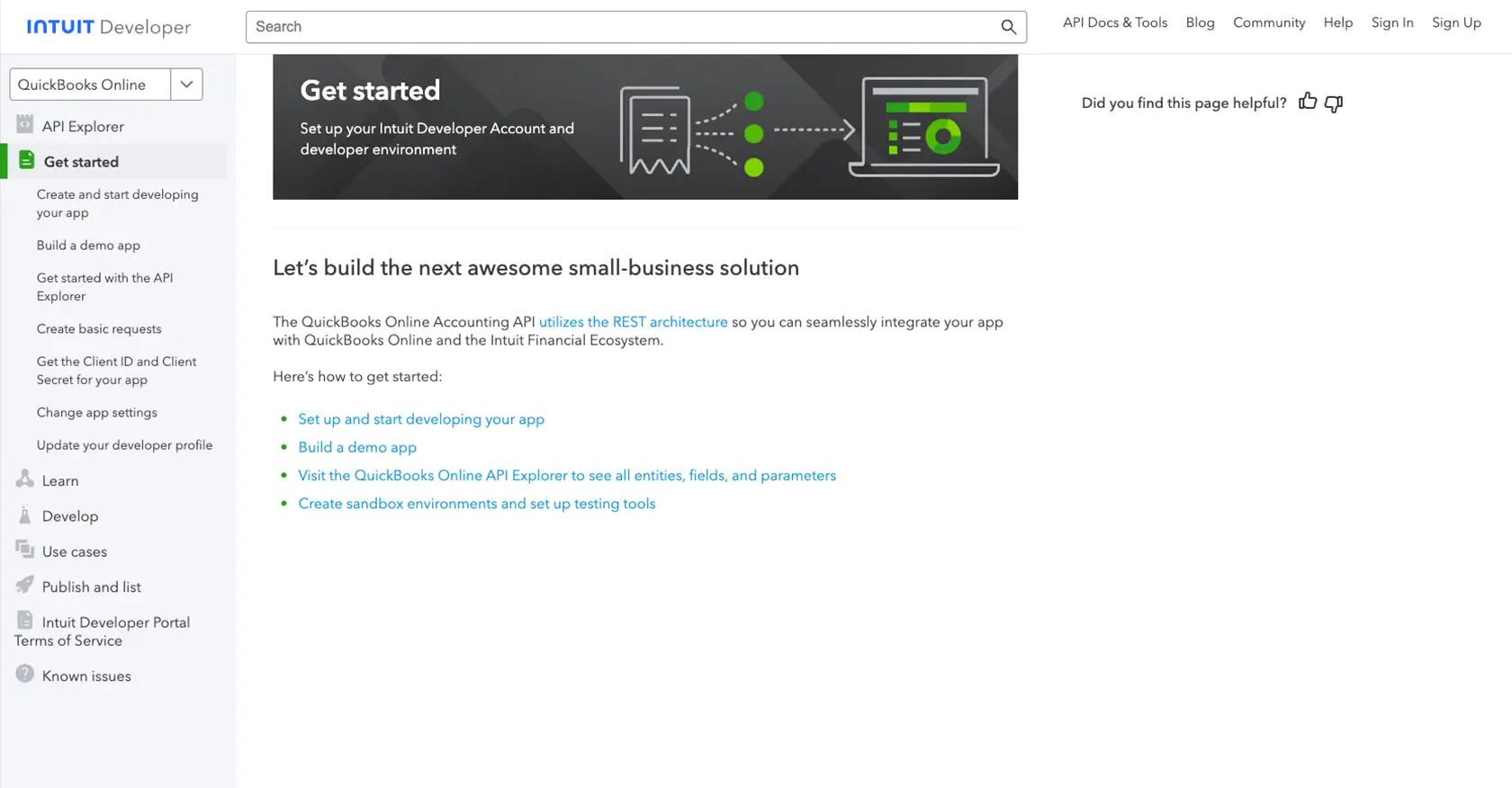
sbb-itb-96038d7
Making API Calls to QuickBooks for Creating or Updating Purchase Orders Using PHP
To interact with the QuickBooks API for creating or updating purchase orders, you'll need to use PHP. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses and errors.
Setting Up Your PHP Environment for QuickBooks API Integration
Before making API calls, ensure your PHP environment is correctly set up. You'll need:
- PHP 7.4 or later
- Composer for dependency management
- The
guzzlehttp/guzzle
library for making HTTP requests
Install the Guzzle library using Composer with the following command:
composer require guzzlehttp/guzzle
Creating a Purchase Order with QuickBooks API Using PHP
Here's how to create a purchase order using the QuickBooks API:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token';
$realmId = 'Your_Realm_ID';
$url = "https://sandbox-quickbooks.api.intuit.com/v3/company/$realmId/purchaseorder";
$headers = [
'Authorization' => "Bearer $accessToken",
'Accept' => 'application/json',
'Content-Type' => 'application/json'
];
$purchaseOrderData = [
'VendorRef' => [
'value' => '123'
],
'Line' => [
[
'Amount' => 100.00,
'DetailType' => 'ItemBasedExpenseLineDetail',
'ItemBasedExpenseLineDetail' => [
'ItemRef' => [
'value' => '1'
]
]
]
]
];
$response = $client->post($url, [
'headers' => $headers,
'json' => $purchaseOrderData
]);
if ($response->getStatusCode() == 200) {
echo "Purchase Order Created Successfully";
} else {
echo "Failed to Create Purchase Order";
}
Replace Your_Access_Token
and Your_Realm_ID
with your actual access token and realm ID. This code sends a POST request to the QuickBooks API to create a purchase order.
Updating a Purchase Order with QuickBooks API Using PHP
To update an existing purchase order, modify the request as follows:
$purchaseOrderId = 'Your_Purchase_Order_ID';
$url = "https://sandbox-quickbooks.api.intuit.com/v3/company/$realmId/purchaseorder/$purchaseOrderId";
$purchaseOrderData['Id'] = $purchaseOrderId;
$purchaseOrderData['SyncToken'] = '0'; // Update with the current sync token
$response = $client->post($url, [
'headers' => $headers,
'json' => $purchaseOrderData
]);
if ($response->getStatusCode() == 200) {
echo "Purchase Order Updated Successfully";
} else {
echo "Failed to Update Purchase Order";
}
Ensure you replace Your_Purchase_Order_ID
with the ID of the purchase order you wish to update and provide the correct sync token.
Handling QuickBooks API Responses and Errors
After making an API call, it's crucial to handle responses and errors appropriately. QuickBooks API responses include status codes that indicate success or failure:
- 200: Success
- 400: Bad Request
- 401: Unauthorized
- 403: Forbidden
- 404: Not Found
- 500: Internal Server Error
Check the response status code and handle errors by logging them or displaying user-friendly messages. For more details, refer to the QuickBooks API documentation.
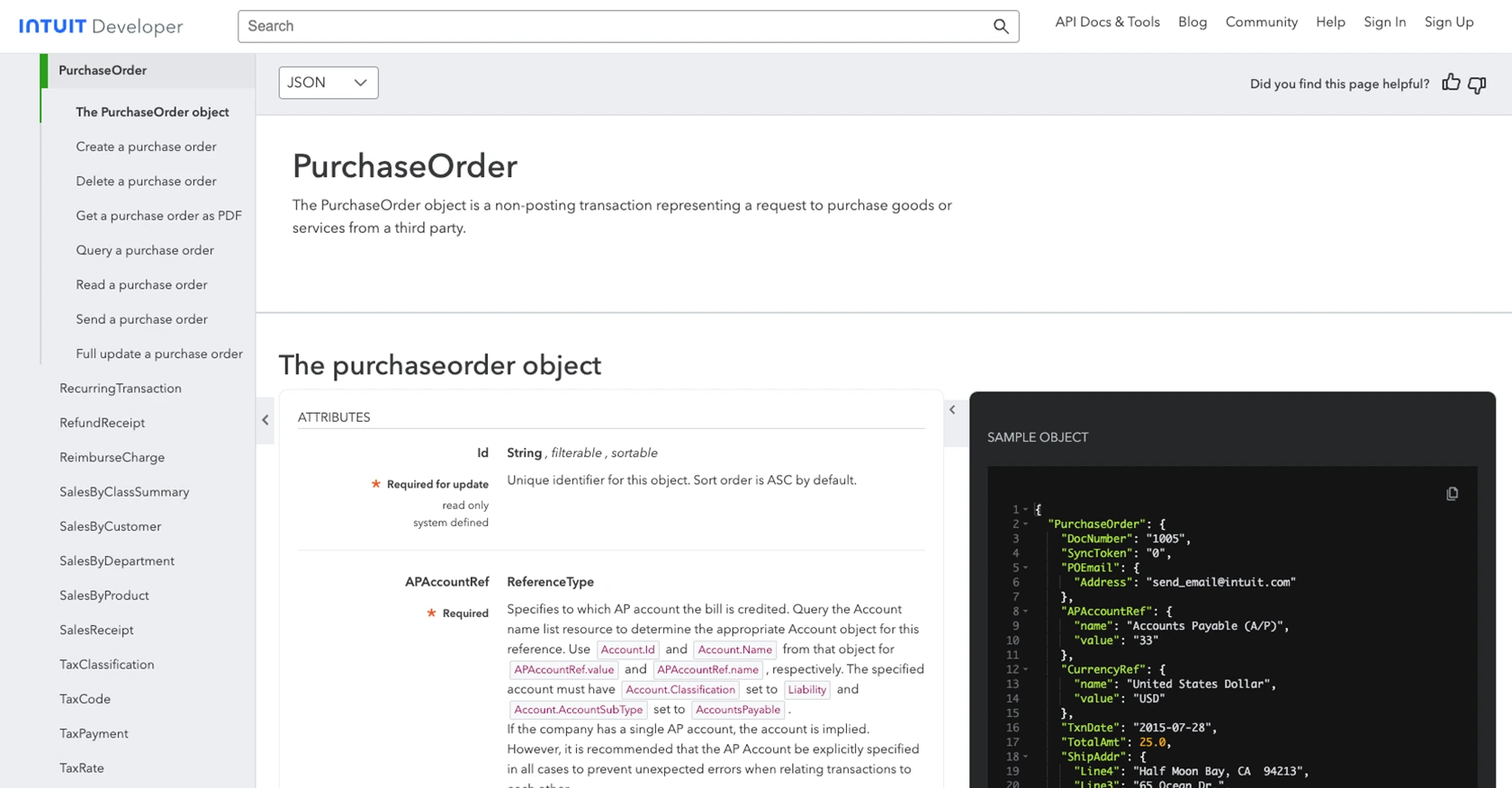
Conclusion and Best Practices for QuickBooks API Integration
Integrating with the QuickBooks API to create or update purchase orders using PHP can significantly enhance your business's financial management processes. By automating these tasks, you reduce manual errors and improve efficiency, allowing your team to focus on more strategic activities.
Best Practices for Secure and Efficient QuickBooks API Usage
- Securely Store Credentials: Always store your Client ID, Client Secret, and access tokens securely. Use environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: QuickBooks API has rate limits to ensure fair usage. Implement retry logic and exponential backoff to handle rate limit errors gracefully. Refer to the QuickBooks documentation for specific rate limit details.
- Data Standardization: Ensure that data fields are standardized and validated before sending them to the API. This helps in maintaining data integrity and consistency across systems.
- Error Handling: Implement robust error handling to manage API response codes effectively. Log errors and provide user-friendly messages to enhance the user experience.
Streamline Your Integration Process with Endgrate
While integrating with QuickBooks API can be powerful, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies this process by providing a single endpoint for all your integration needs.
By leveraging Endgrate, you can save time and resources, allowing your development team to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can transform your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/all-entities/purchaseorder
Ready to get started?