Using the Intercom API to Get Users in Javascript
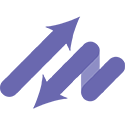
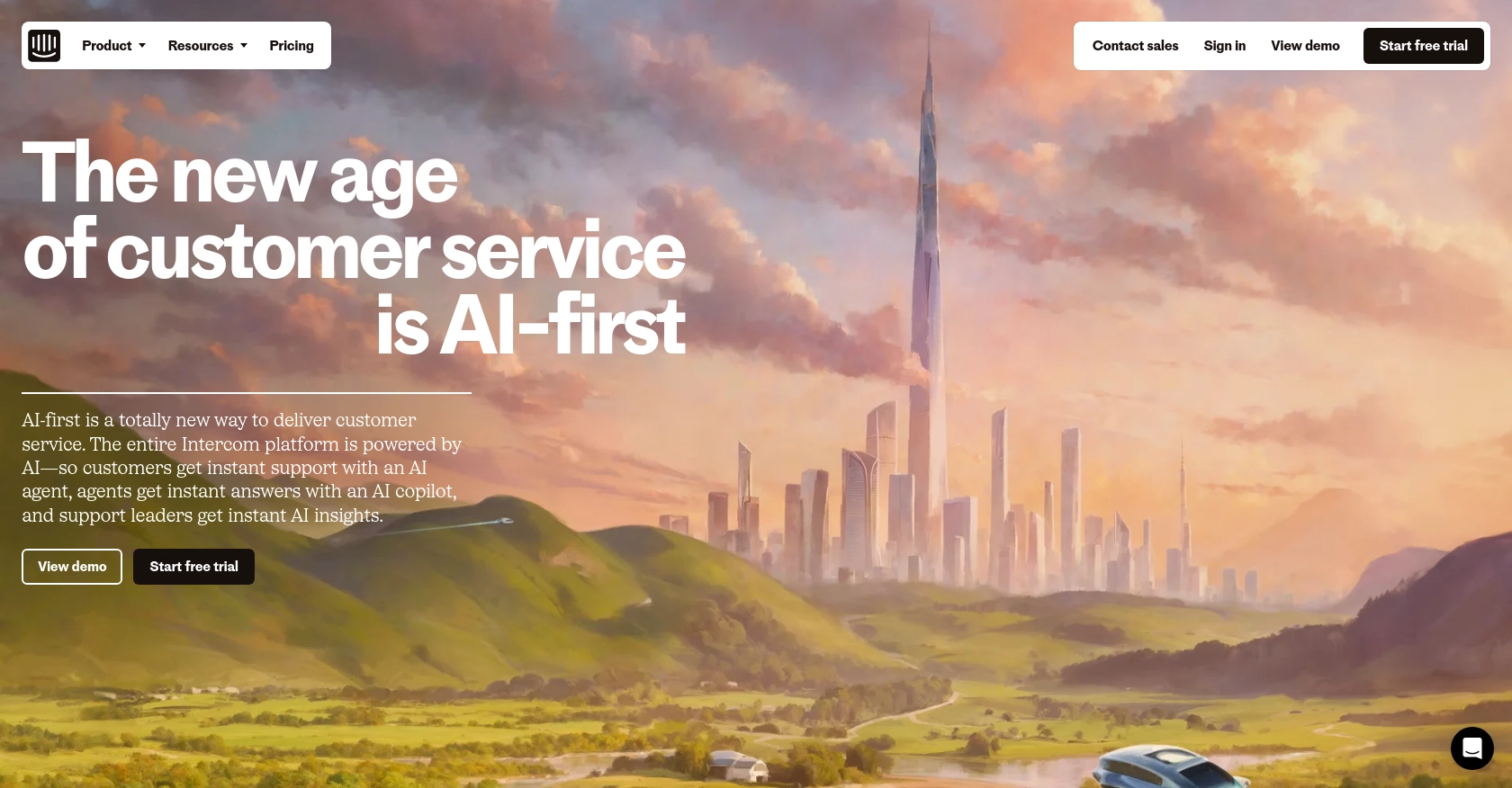
Introduction to Intercom API Integration
Intercom is a powerful customer communication platform that enables businesses to engage with their customers through personalized messaging and support. It offers a suite of tools for managing customer interactions, including chat, email, and automated messaging, making it a popular choice for businesses looking to enhance their customer engagement strategies.
Developers often integrate with Intercom's API to access and manage user data, enabling them to create customized experiences and automate workflows. For example, a developer might use the Intercom API to retrieve user information and tailor marketing campaigns based on user behavior and preferences.
This article will guide you through using JavaScript to interact with the Intercom API, specifically focusing on retrieving user data. By following this tutorial, you'll learn how to efficiently access and manage user information within the Intercom platform using JavaScript.
Setting Up Your Intercom Developer Account and OAuth Authentication
Before you can start interacting with the Intercom API using JavaScript, you'll need to set up a developer account and configure OAuth authentication. This process ensures that your application can securely access user data within the Intercom platform.
Create an Intercom Developer Account
If you don't already have an Intercom account, you'll need to create one. Follow these steps to set up your developer account:
- Visit the Intercom Developer Hub and sign up for a free account.
- Once registered, log in to your account and navigate to the Developer Hub.
- Create a new development workspace. This workspace will allow you to build and test your integration.
Configure OAuth for Intercom API Access
Intercom uses OAuth 2.0 for authentication, which allows your application to access user data securely. Follow these steps to set up OAuth:
- In the Developer Hub, go to the "Authentication" section and enable the "Use OAuth" option.
- Provide the necessary information, including redirect URLs. Ensure these URLs use HTTPS, as Intercom requires secure connections.
- Select the permissions your application needs. For retrieving user data, ensure you have the "Read and list users and companies" scope enabled.
- Save your changes and note down the client ID and client secret provided by Intercom. You'll use these credentials to obtain an access token.
For more detailed information on setting up OAuth, refer to the Intercom OAuth documentation.
Generate an Access Token
With your OAuth configuration in place, you can now generate an access token to authenticate API requests:
- Redirect users to the following URL to obtain an authorization code:
https://app.intercom.com/oauth?client_id=YOUR_CLIENT_ID&state=YOUR_STATE
- Once users authorize your app, they'll be redirected to your specified URL with a
code
parameter. - Exchange this authorization code for an access token by making a POST request to:
Include the following parameters in the request body:https://api.intercom.io/auth/eagle/token
code
: The authorization code received.client_id
: Your app's client ID.client_secret
: Your app's client secret.
Upon successful exchange, you'll receive an access token, which you can use to authenticate your API requests. For further details, consult the Intercom API documentation.
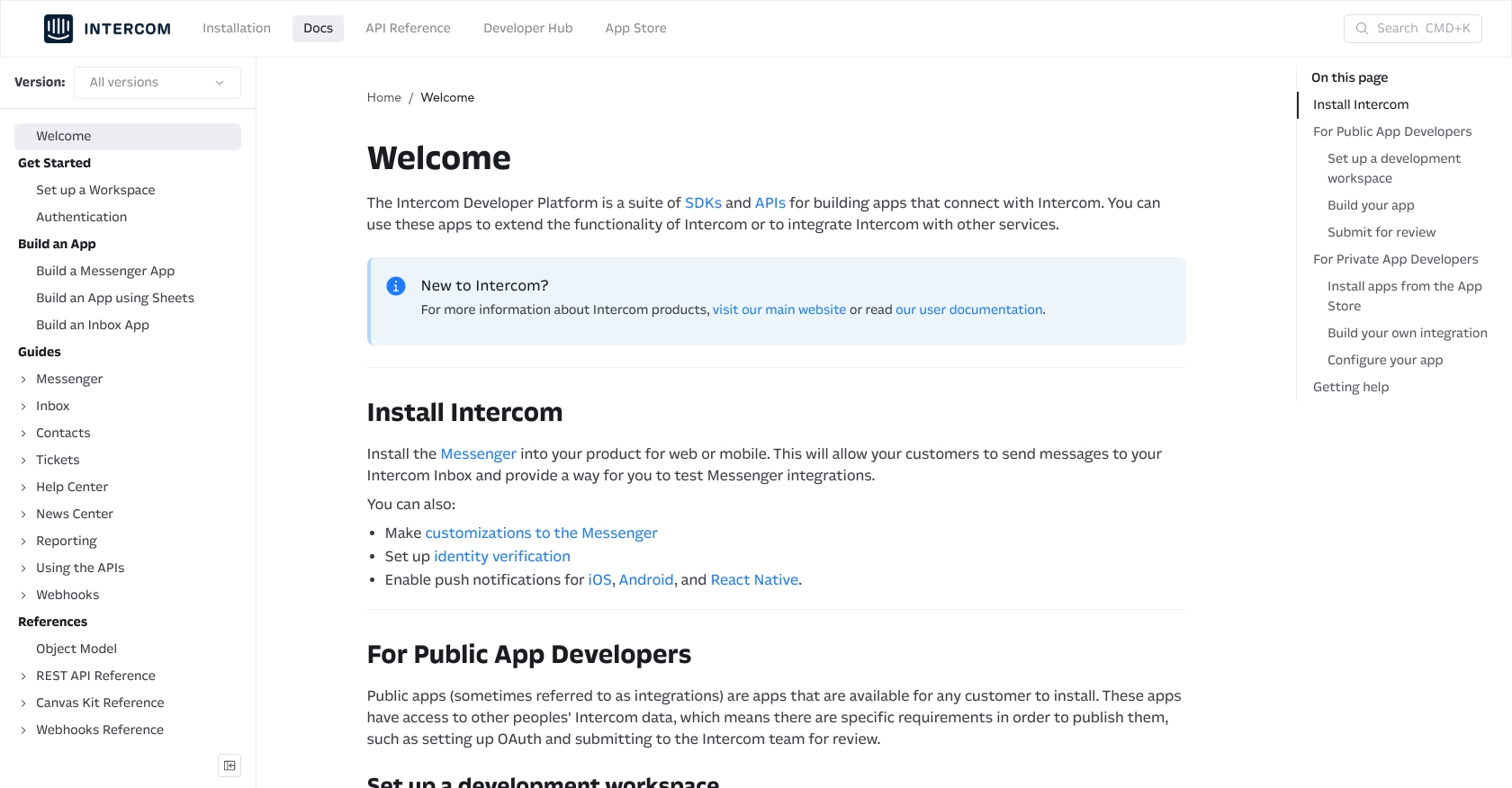
sbb-itb-96038d7
Making API Calls to Retrieve Users with Intercom API in JavaScript
Once you have set up your Intercom developer account and configured OAuth, you can proceed to make API calls to retrieve user data using JavaScript. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for Intercom API
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A code editor, such as Visual Studio Code, to write and execute your JavaScript code.
- The
axios
library for making HTTP requests. Install it using npm:
npm install axios
Writing JavaScript Code to Retrieve Users from Intercom
With your environment set up, you can now write the JavaScript code to interact with the Intercom API. Create a new file named getIntercomUsers.js
and add the following code:
const axios = require('axios');
// Set your access token
const accessToken = 'YOUR_ACCESS_TOKEN';
// Define the API endpoint and headers
const endpoint = 'https://api.intercom.io/users';
const headers = {
'Authorization': `Bearer ${accessToken}`,
'Accept': 'application/json',
'Intercom-Version': '2.11'
};
// Make a GET request to the Intercom API
axios.get(endpoint, { headers })
.then(response => {
// Handle successful response
console.log('Users retrieved:', response.data);
})
.catch(error => {
// Handle errors
console.error('Error retrieving users:', error.response ? error.response.data : error.message);
});
Replace YOUR_ACCESS_TOKEN
with the access token you obtained during the OAuth setup.
Executing the JavaScript Code and Verifying Results
Run the code from your terminal using the following command:
node getIntercomUsers.js
If successful, you should see a list of users retrieved from your Intercom workspace. Verify the results by checking the user data in your Intercom dashboard.
Handling Errors and Common Issues with Intercom API
When making API calls, you may encounter errors. Here are some common issues and how to handle them:
- 401 Unauthorized: Ensure your access token is correct and has not expired.
- Rate Limiting: Intercom's API has rate limits. If you exceed them, you'll receive a 429 error. Implement retry logic or backoff strategies to handle this.
- Network Errors: Check your internet connection and ensure the API endpoint is correct.
For more detailed information on error handling, refer to the Intercom API documentation.
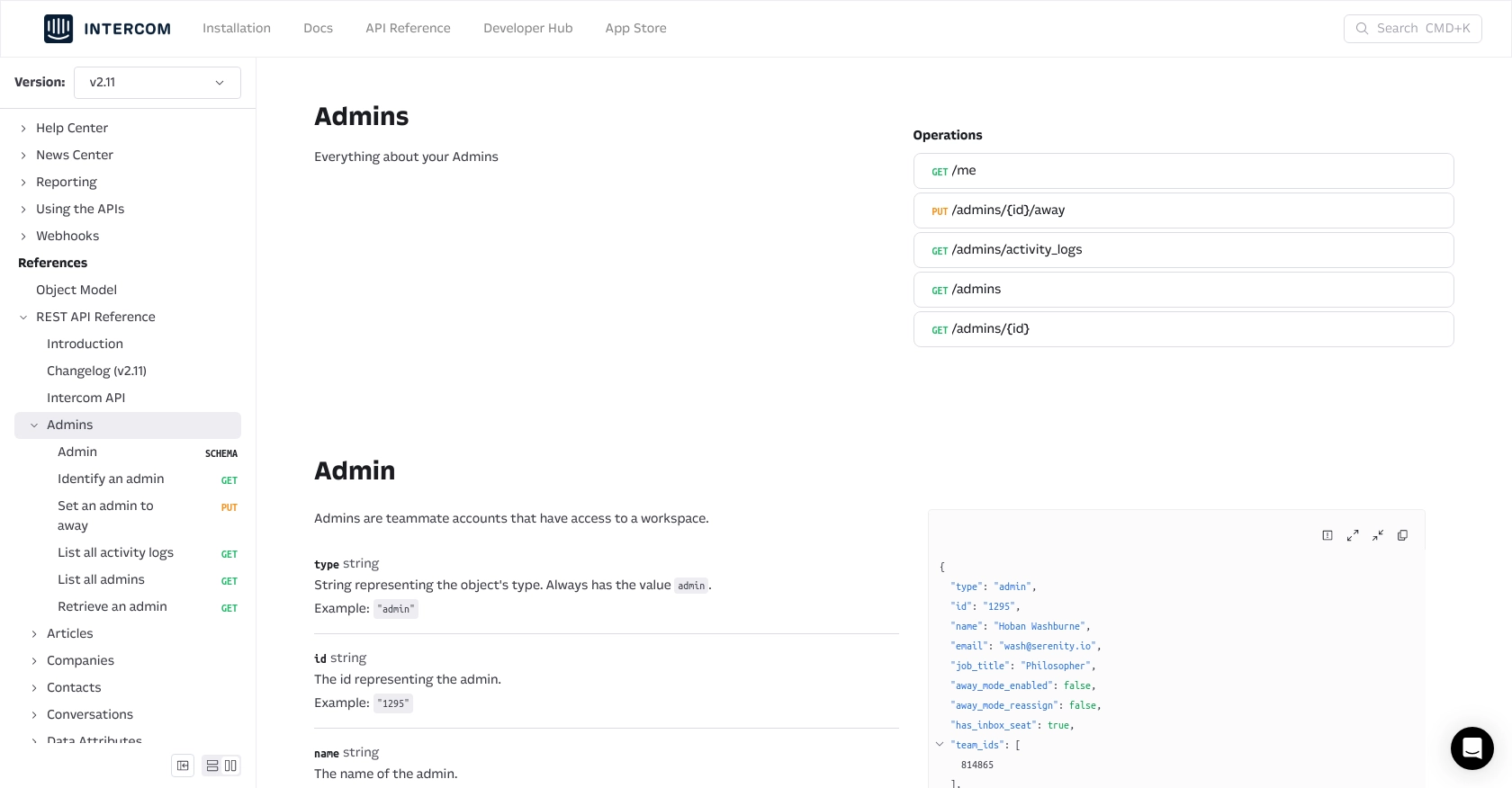
Best Practices for Using Intercom API in JavaScript
When working with the Intercom API, it's essential to follow best practices to ensure efficient and secure integration. Here are some recommendations:
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Implement Rate Limiting: Intercom's API has rate limits, so it's crucial to implement retry logic or exponential backoff strategies to handle 429 errors gracefully. This ensures your application remains responsive even when limits are reached.
- Standardize Data Fields: When retrieving user data, consider transforming and standardizing fields to match your application's data model. This will help maintain consistency across different integrations.
Leveraging Endgrate for Seamless Intercom Integrations
Building and maintaining integrations can be time-consuming and complex, especially when dealing with multiple platforms. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Intercom.
With Endgrate, you can:
- Focus on Core Product Development: Save time and resources by outsourcing integrations, allowing your team to concentrate on enhancing your core product.
- Build Once, Use Everywhere: Develop a single integration for each use case and apply it across multiple platforms, reducing redundancy and effort.
- Enhance Customer Experience: Provide your customers with a seamless and intuitive integration experience, improving satisfaction and engagement.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website and discover the benefits of a unified integration solution.
Read More
Ready to get started?