How to Get Accounts with the Active Campaign API in PHP
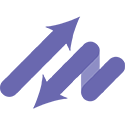
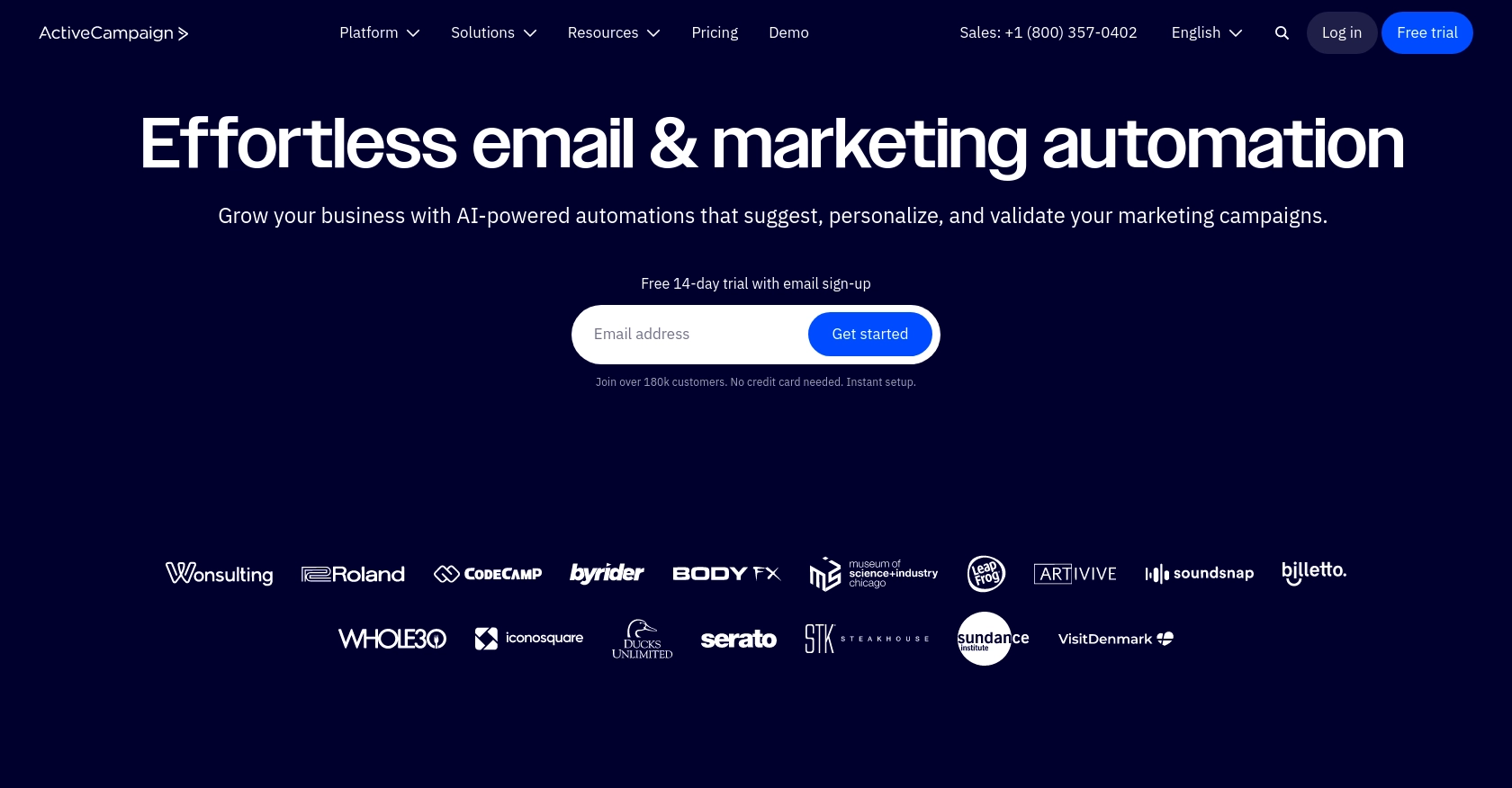
Introduction to Active Campaign API Integration
Active Campaign is a powerful marketing automation platform that combines email marketing, automation, and CRM tools to help businesses engage with their customers effectively. With its robust API, developers can seamlessly integrate Active Campaign's features into their own applications, enhancing customer relationship management and marketing efforts.
Connecting with the Active Campaign API allows developers to access and manage accounts, automate workflows, and retrieve valuable data. For example, a developer might want to list all accounts associated with their Active Campaign instance to analyze customer interactions and improve targeted marketing strategies.
Setting Up Your Active Campaign Test Account
Before you can start integrating with the Active Campaign API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data.
Creating an Active Campaign Account
If you don't already have an Active Campaign account, you can sign up for a free trial on their website. This trial will give you access to all the features you need to test the API integration.
- Visit the Active Campaign website.
- Click on "Start Your Free Trial" and follow the instructions to create your account.
- Once your account is set up, log in to access the dashboard.
Generating Your API Key
To authenticate API requests, you'll need an API key. Follow these steps to obtain it:
- Log in to your Active Campaign account.
- Navigate to the "Settings" page by clicking on your account name in the top right corner.
- Select the "Developer" tab.
- Here, you'll find your unique API key. Make sure to keep this key secure and do not share it publicly.
For more details, refer to the Active Campaign authentication documentation.
Configuring Your Base URL
The API base URL is specific to your account and is necessary for making API calls. To find your base URL:
- Go to the "Developer" tab in your account settings.
- Locate the base URL, which typically follows the format:
https://youraccountname.api-us1.com/api/3/
.
Ensure you use this URL for all API requests. More information can be found in the Active Campaign base URL documentation.
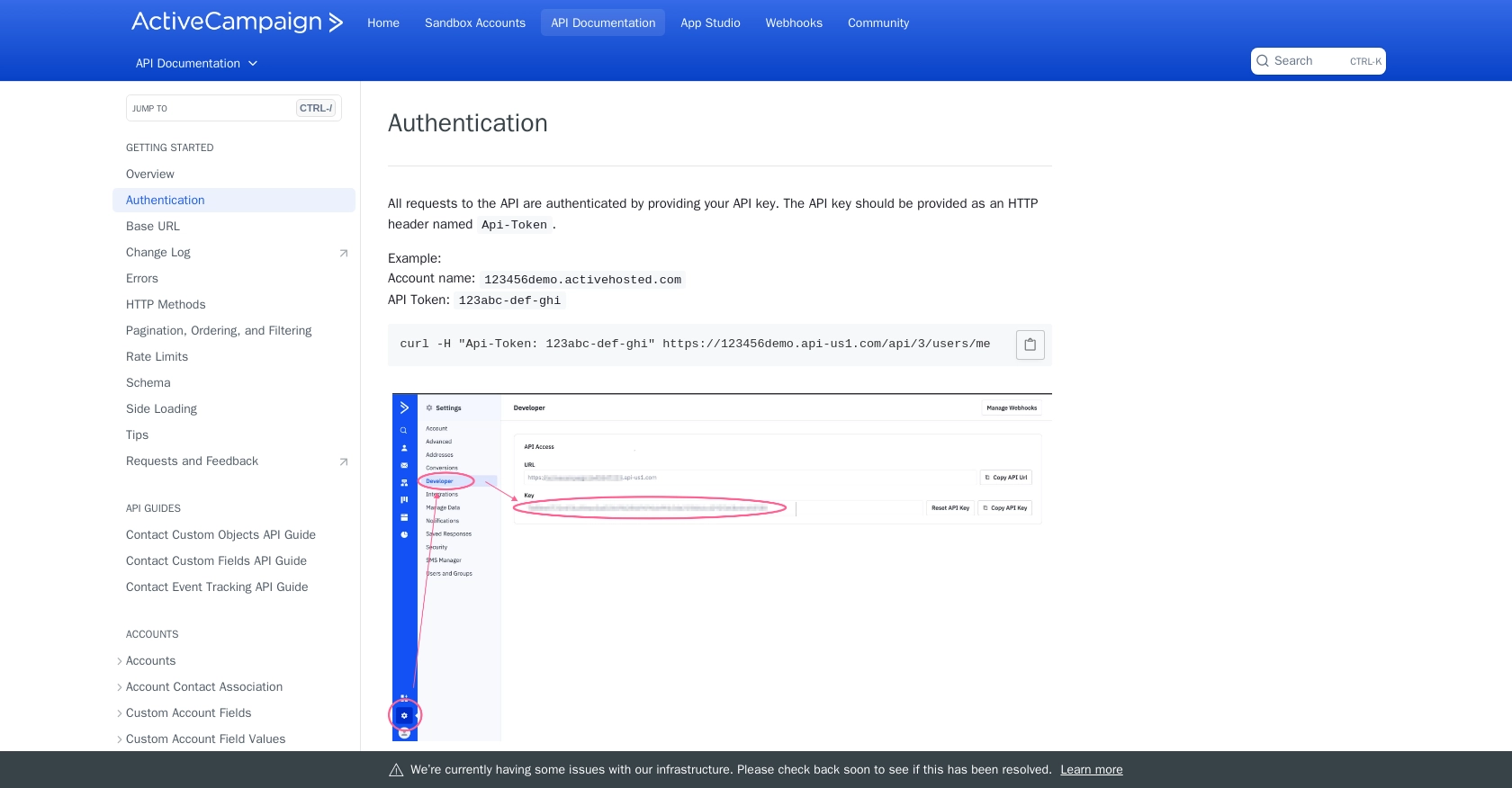
sbb-itb-96038d7
Making API Calls to List All Accounts with Active Campaign API in PHP
To interact with the Active Campaign API using PHP, you'll need to make HTTP requests to the API endpoints. In this section, we'll guide you through setting up your PHP environment and making a call to list all accounts associated with your Active Campaign instance.
Setting Up Your PHP Environment
Before making API calls, ensure you have the following prerequisites:
- PHP 7.4 or higher installed on your machine.
- Composer for managing dependencies.
To install the necessary HTTP client library, run the following command:
composer require guzzlehttp/guzzle
Writing the PHP Code to Retrieve Accounts
Now, let's write a PHP script to call the Active Campaign API and list all accounts. Create a file named list_accounts.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$apiKey = 'Your_API_Key';
$baseUrl = 'https://youraccountname.api-us1.com/api/3/';
$client = new Client([
'base_uri' => $baseUrl,
'headers' => [
'Api-Token' => $apiKey,
'Accept' => 'application/json',
],
]);
try {
$response = $client->get('accounts');
$data = json_decode($response->getBody(), true);
foreach ($data['accounts'] as $account) {
echo "Account Name: " . $account['name'] . "\n";
echo "Contact Count: " . $account['contactCount'] . "\n";
echo "Deal Count: " . $account['dealCount'] . "\n\n";
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace Your_API_Key
with the API key you obtained earlier. This script uses Guzzle, a PHP HTTP client, to send a GET request to the Active Campaign API endpoint for listing accounts.
Running the PHP Script and Verifying Results
To execute the script, run the following command in your terminal:
php list_accounts.php
If successful, you should see a list of accounts printed in your terminal, including their names, contact counts, and deal counts.
Handling Errors and Troubleshooting
If you encounter errors, ensure that:
- Your API key and base URL are correct.
- You have internet connectivity.
- The Guzzle library is properly installed.
For more detailed error information, refer to the Active Campaign API documentation.
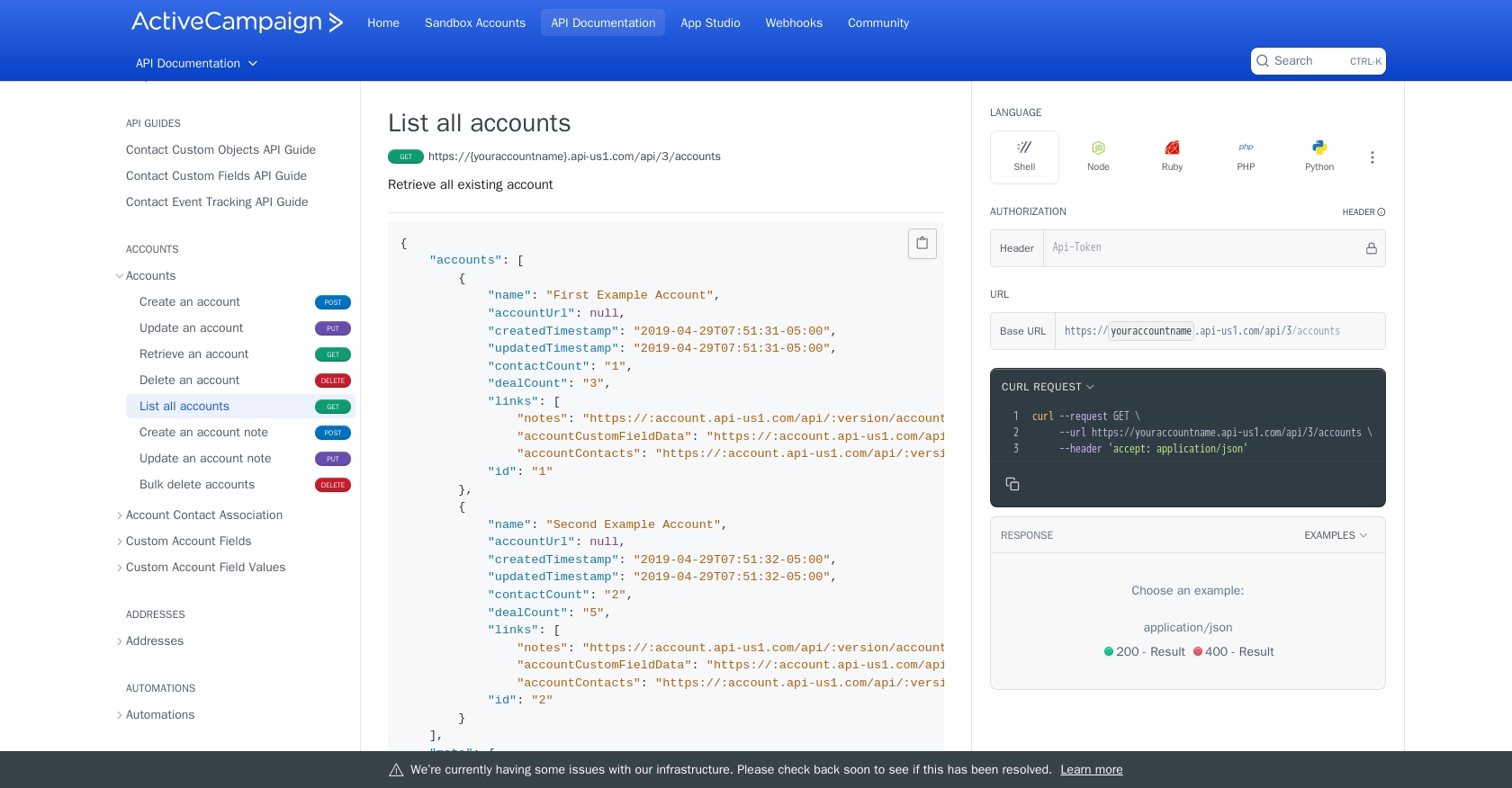
Conclusion and Best Practices for Active Campaign API Integration in PHP
Integrating with the Active Campaign API using PHP provides a powerful way to enhance your marketing automation and CRM capabilities. By following the steps outlined in this guide, you can efficiently retrieve account data and leverage it to improve your customer engagement strategies.
Best Practices for Secure and Efficient API Usage
- Secure API Key Storage: Always keep your API key confidential. Store it securely in environment variables or a secure vault, and avoid hardcoding it in your scripts.
- Handle Rate Limits: Active Campaign imposes a rate limit of 5 requests per second per account. Implement logic to handle rate limiting gracefully, such as retrying requests after a delay. For more details, refer to the Active Campaign rate limits documentation.
- Error Handling: Implement robust error handling to manage potential issues like network failures or invalid responses. Use try-catch blocks to capture exceptions and log errors for troubleshooting.
- Data Transformation and Standardization: When retrieving data, consider transforming and standardizing it to fit your application's requirements. This ensures consistency and ease of use across different systems.
Enhancing Your Integration Experience with Endgrate
While integrating with the Active Campaign API can be straightforward, managing multiple integrations can become complex and time-consuming. This is where Endgrate can make a significant difference.
Endgrate offers a unified API endpoint that simplifies integration with various platforms, including Active Campaign. By using Endgrate, you can:
- Save time and resources by outsourcing integration tasks and focusing on your core product development.
- Build once for each use case, reducing the need for multiple integrations.
- Provide an intuitive and seamless integration experience for your customers.
Explore how Endgrate can streamline your integration processes by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/activecampaign
- https://developers.activecampaign.com/reference/authentication
- https://developers.activecampaign.com/reference/url
- https://developers.activecampaign.com/reference/pagination
- https://developers.activecampaign.com/reference/rate-limits
- https://developers.activecampaign.com/reference/list-all-accounts
Ready to get started?