Using the Stamped API to Create Customers in PHP
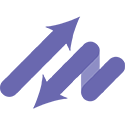
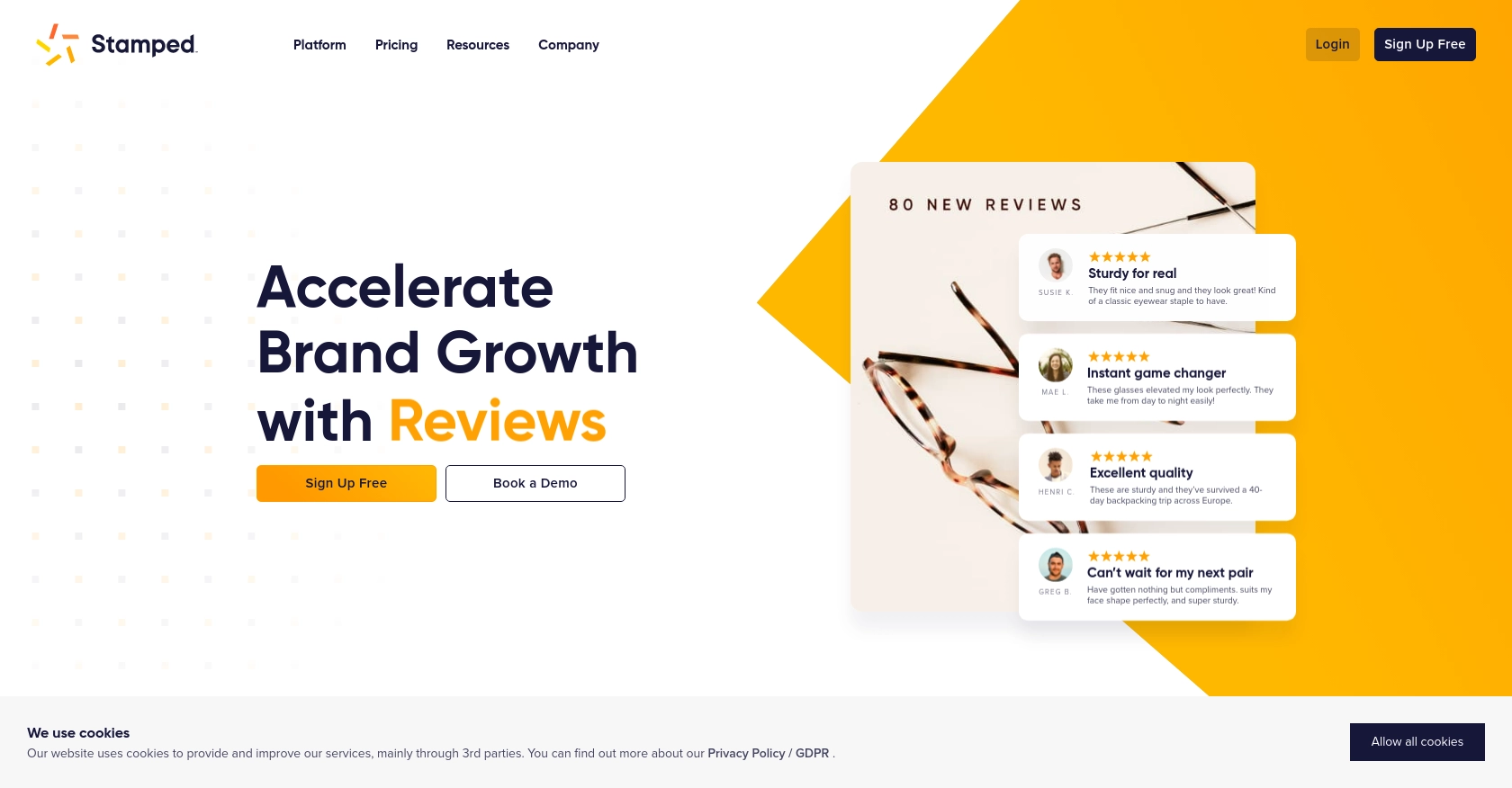
Introduction to Stamped API
Stamped is a powerful platform that empowers over 75,000 brands to enhance customer engagement and drive growth through reviews, loyalty programs, and more. With its robust suite of tools, Stamped helps businesses collect and showcase customer feedback, fostering trust and boosting sales.
Integrating with the Stamped API allows developers to seamlessly manage customer data and interactions. By leveraging the API, you can automate tasks such as creating new customer profiles, which can be particularly useful for syncing customer information from your e-commerce platform to Stamped. This integration ensures that your customer engagement strategies are always up-to-date and effective.
Setting Up Your Stamped Account for API Integration
Before you can start using the Stamped API to create customers, you'll need to set up your Stamped account and obtain the necessary API credentials. This involves creating an account, accessing the API Keys section, and configuring your authentication details.
Creating a Stamped Account
If you don't already have a Stamped account, you can sign up for a Professional or Enterprise plan on the Stamped website. These plans are required to access the API features. Follow the instructions provided during the sign-up process to create your account.
Accessing the API Keys
Once your account is set up, log in to the Stamped dashboard. Navigate to the API Keys section in the Control Panel. Here, you'll find your public and private API keys, which are essential for authenticating your API requests.
Configuring Authentication for Stamped API
The Stamped API uses HTTP Basic Auth for authentication. You'll need to use your public API key as the username and your private API key as the password. This ensures secure access to the API endpoints.
Here's a quick guide on how to set up your authentication:
- Locate your public API key and private API key in the API Keys section.
- Use these keys to authenticate your API requests by setting the public key as the username and the private key as the password.
For more detailed information, you can refer to the Stamped REST API documentation.
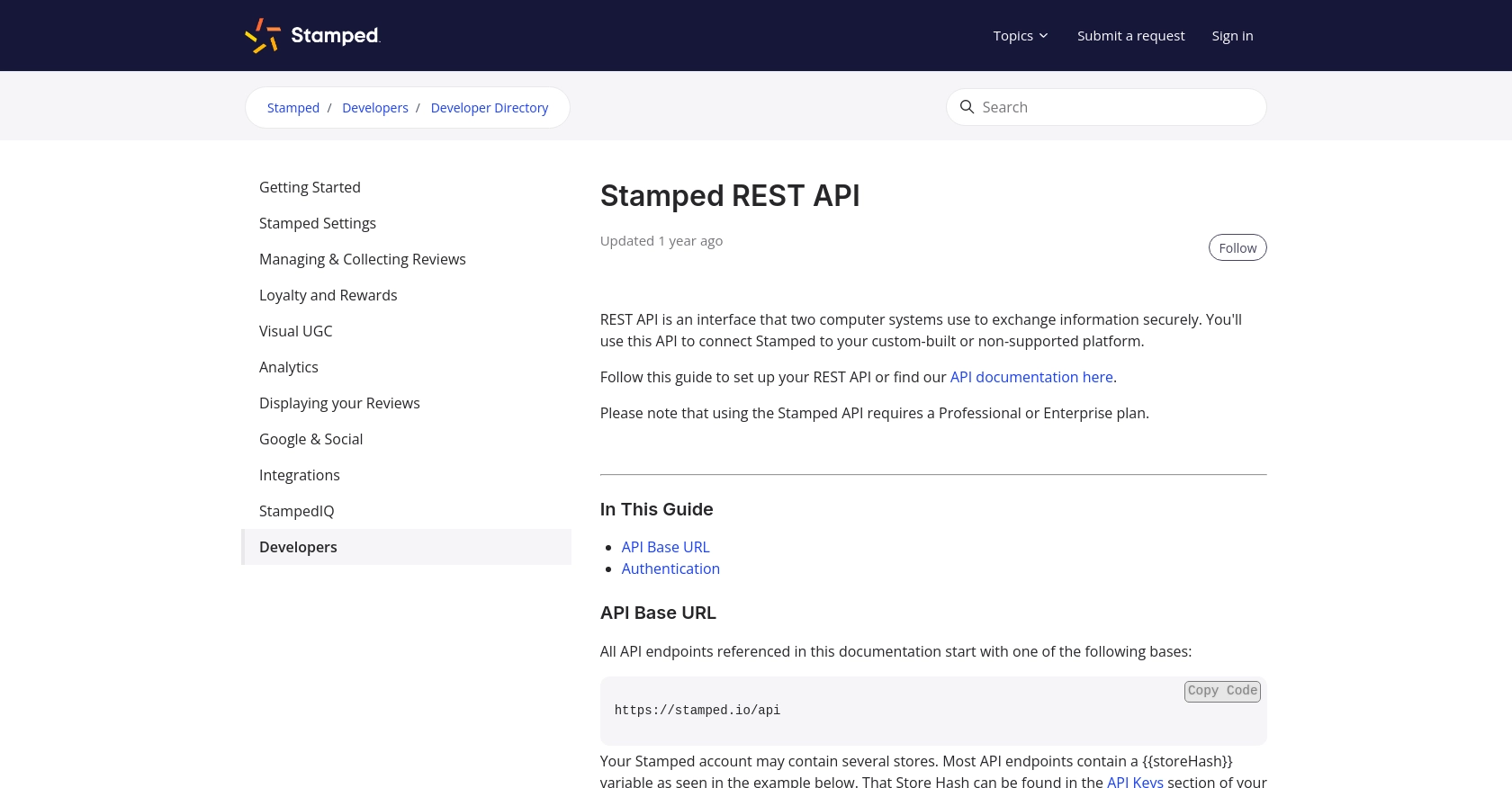
sbb-itb-96038d7
Making API Calls to Create Customers with Stamped API in PHP
To interact with the Stamped API and create customer profiles, you'll need to use PHP to make HTTP requests. This section will guide you through setting up your PHP environment, writing the code to make the API call, and handling the response effectively.
Setting Up Your PHP Environment for Stamped API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need PHP version 7.4 or later and the cURL
extension enabled to handle HTTP requests.
- Verify your PHP version by running
php -v
in your terminal. - Ensure the
cURL
extension is enabled by checking yourphp.ini
file or runningphp -m | grep curl
.
Writing PHP Code to Create a Customer with Stamped API
Now that your environment is ready, you can write the PHP code to create a customer using the Stamped API. Follow these steps:
<?php
// Stamped API credentials
$publicKey = 'your_public_api_key';
$privateKey = 'your_private_api_key';
// API endpoint for creating a customer
$shopId = 'your_shop_id';
$url = "https://stamped.io/api/v3/merchant/shops/{$shopId}/customers";
// Customer data
$data = [
'email' => 'customer@example.com',
'firstName' => 'John',
'lastName' => 'Doe'
];
// Initialize cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPAUTH, CURLAUTH_BASIC);
curl_setopt($ch, CURLOPT_USERPWD, "$publicKey:$privateKey");
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Content-Type: application/json'
]);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
// Decode the response
$responseData = json_decode($response, true);
echo 'Customer Created Successfully: ' . print_r($responseData, true);
}
// Close cURL session
curl_close($ch);
?>
Replace your_public_api_key
, your_private_api_key
, and your_shop_id
with your actual Stamped API credentials and shop ID.
Verifying the API Call and Handling Responses
After running the PHP script, check the response to ensure the customer was created successfully. The response should contain details of the newly created customer.
- If the request is successful, you'll see a confirmation message with customer details.
- If there's an error, the script will output the error message. Common issues include incorrect credentials or malformed data.
For more information on handling errors, refer to the Stamped API documentation.
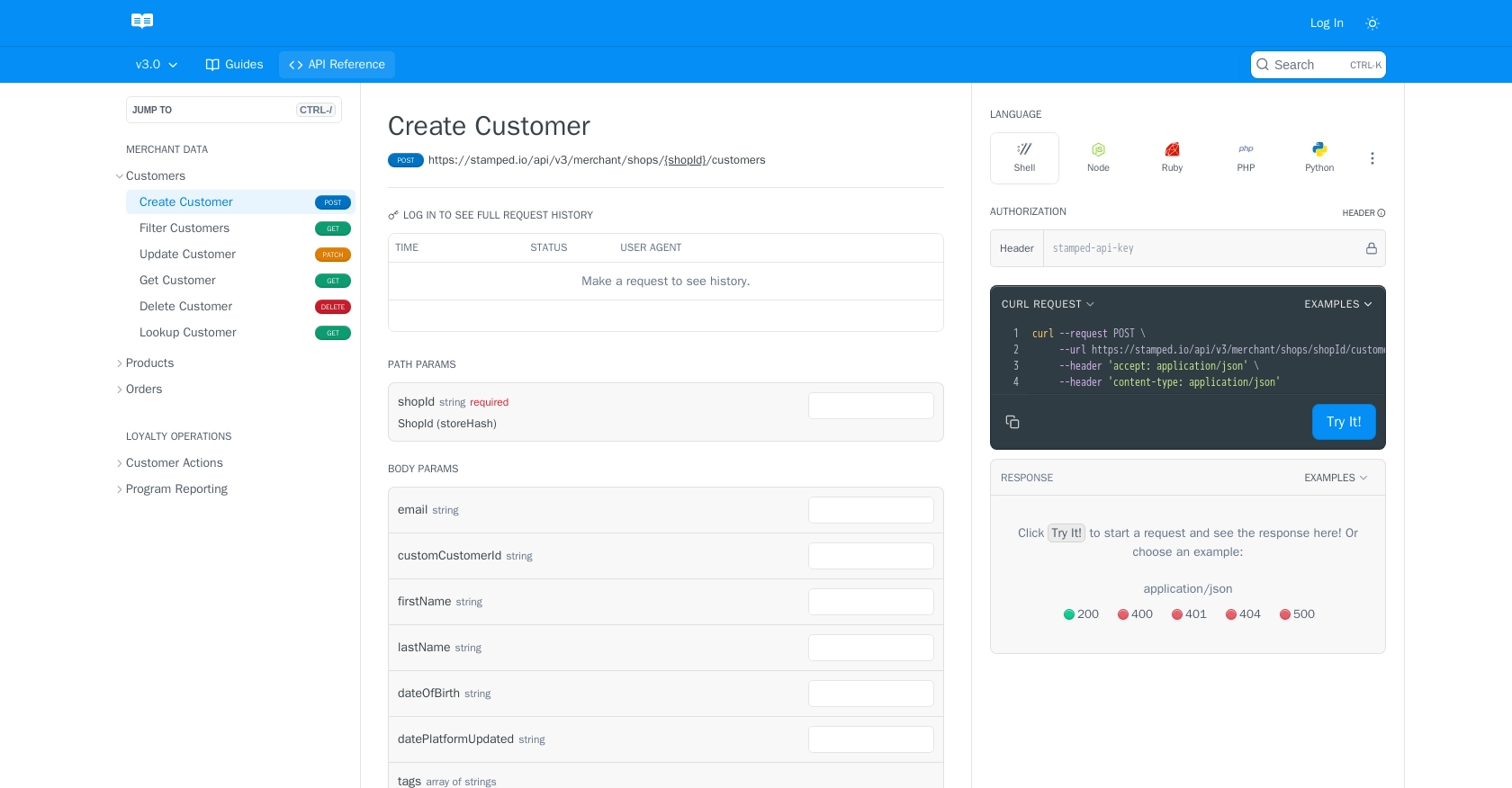
Best Practices for Using Stamped API in PHP
When integrating with the Stamped API, it's essential to follow best practices to ensure secure and efficient API interactions. Here are some recommendations:
- Securely Store API Credentials: Always store your public and private API keys securely. Avoid hardcoding them directly in your code. Instead, use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Stamped API. Implement logic to handle rate limit responses gracefully, such as retrying requests after a specified delay.
- Validate API Responses: Always check the API response status and data to ensure the request was successful. Implement error handling to manage unexpected responses or failures.
- Data Standardization: Ensure that customer data is standardized before sending it to the API. This includes validating email formats and ensuring required fields are populated.
Streamlining Integrations with Endgrate
Integrating multiple platforms can be complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including Stamped. This allows you to manage integrations more efficiently, saving time and resources.
With Endgrate, you can:
- Focus on Core Product Development: Outsource integration tasks to Endgrate, allowing your team to concentrate on enhancing your core product.
- Build Once, Use Everywhere: Create a single integration that works across multiple platforms, reducing the need for repetitive development work.
- Enhance Customer Experience: Provide your customers with a seamless and intuitive integration experience.
Explore how Endgrate can simplify your integration processes by visiting Endgrate.
Read More
Ready to get started?