Using the Airtable API to Get Records (with PHP examples)
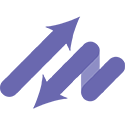
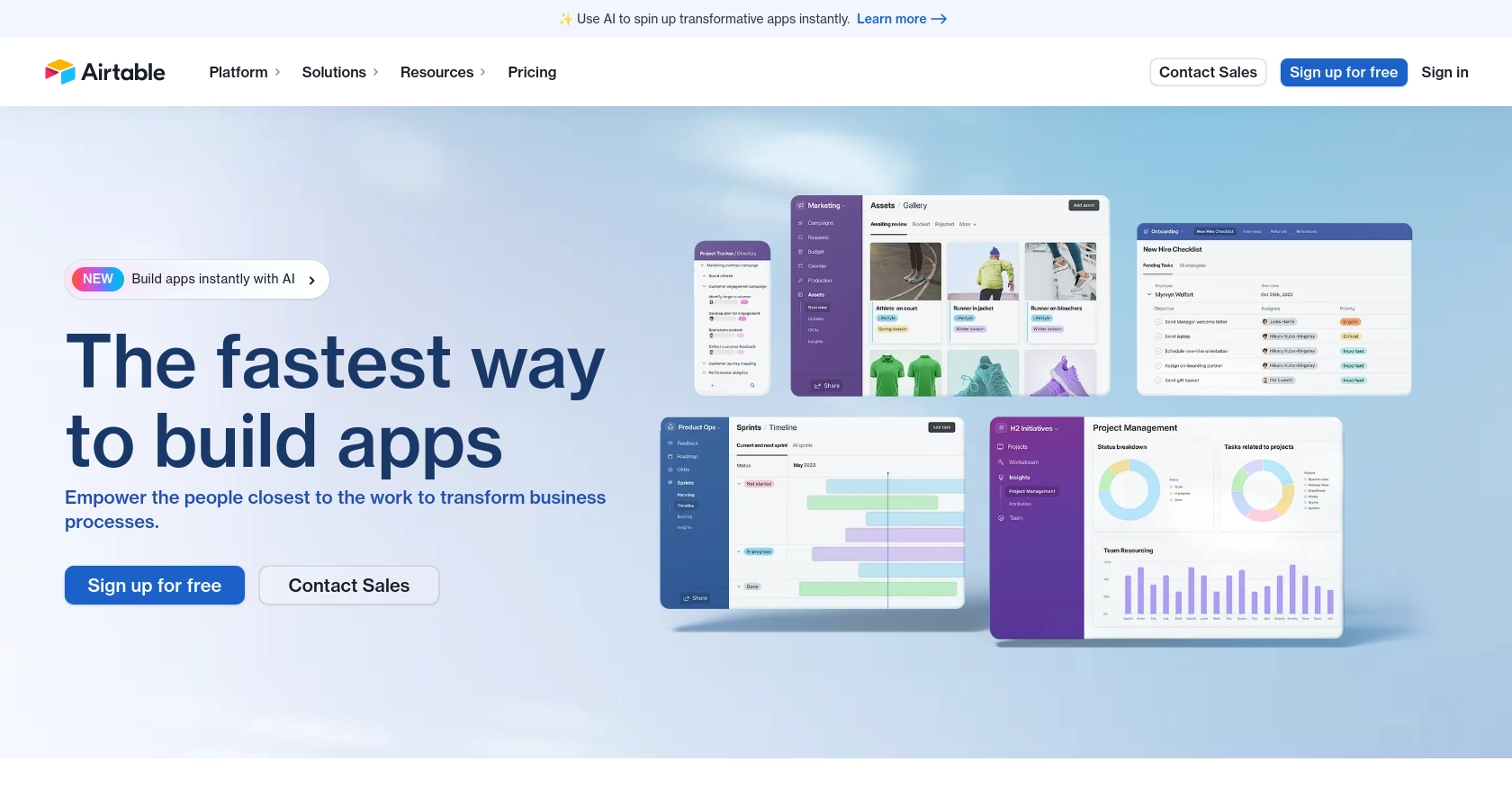
Introduction to Airtable API Integration
Airtable is a versatile platform that combines the simplicity of a spreadsheet with the power of a database, making it an ideal tool for organizing and managing data. Its user-friendly interface and robust API capabilities make it a popular choice for businesses looking to streamline their workflows and enhance productivity.
Developers often seek to integrate with Airtable's API to automate data management tasks, such as retrieving and updating records. For example, a developer might use the Airtable API to fetch records from a project management table and display them in a custom dashboard, providing real-time insights into project progress.
This article will guide you through the process of using PHP to interact with the Airtable API, specifically focusing on retrieving records. By following this tutorial, you'll learn how to efficiently access and manipulate data within Airtable, enabling you to build powerful integrations for your applications.
Setting Up Your Airtable Test/Sandbox Account for API Integration
Before diving into the Airtable API integration with PHP, it's essential to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Airtable offers a straightforward process to create a free account, which you can use for development and testing purposes.
Creating an Airtable Account
If you don't already have an Airtable account, follow these steps to create one:
- Visit the Airtable signup page and register for a free account using your email address.
- Once registered, log in to your Airtable account to access the dashboard.
Setting Up a Base for Testing
To interact with the Airtable API, you'll need a base (equivalent to a database) set up with tables and records. Follow these steps to create a test base:
- From the Airtable dashboard, click on "Add a base" and choose "Start from scratch" or use a template that suits your needs.
- Give your base a name and add tables with the necessary fields for your testing.
Generating an OAuth Access Token for Airtable API
Airtable uses OAuth-based authentication for API access. Here's how to generate an OAuth access token:
- Navigate to the Airtable OAuth integration page.
- Register your application by providing the required details, such as the application name, redirect URI, and scopes.
- Once registered, you'll receive a client ID and client secret. Use these to initiate the OAuth flow and obtain an access token.
- Ensure you request the
data.records:read
scope to fetch records from your base.
For more detailed instructions on OAuth setup, refer to the Airtable OAuth reference documentation.
Testing Your Airtable API Setup
With your test base and OAuth access token ready, you can now proceed to test API calls. Ensure your token has the necessary permissions and that you have access to the base you intend to interact with.
By setting up your Airtable test account and generating the required access token, you're now prepared to explore the Airtable API using PHP, as detailed in the following sections.
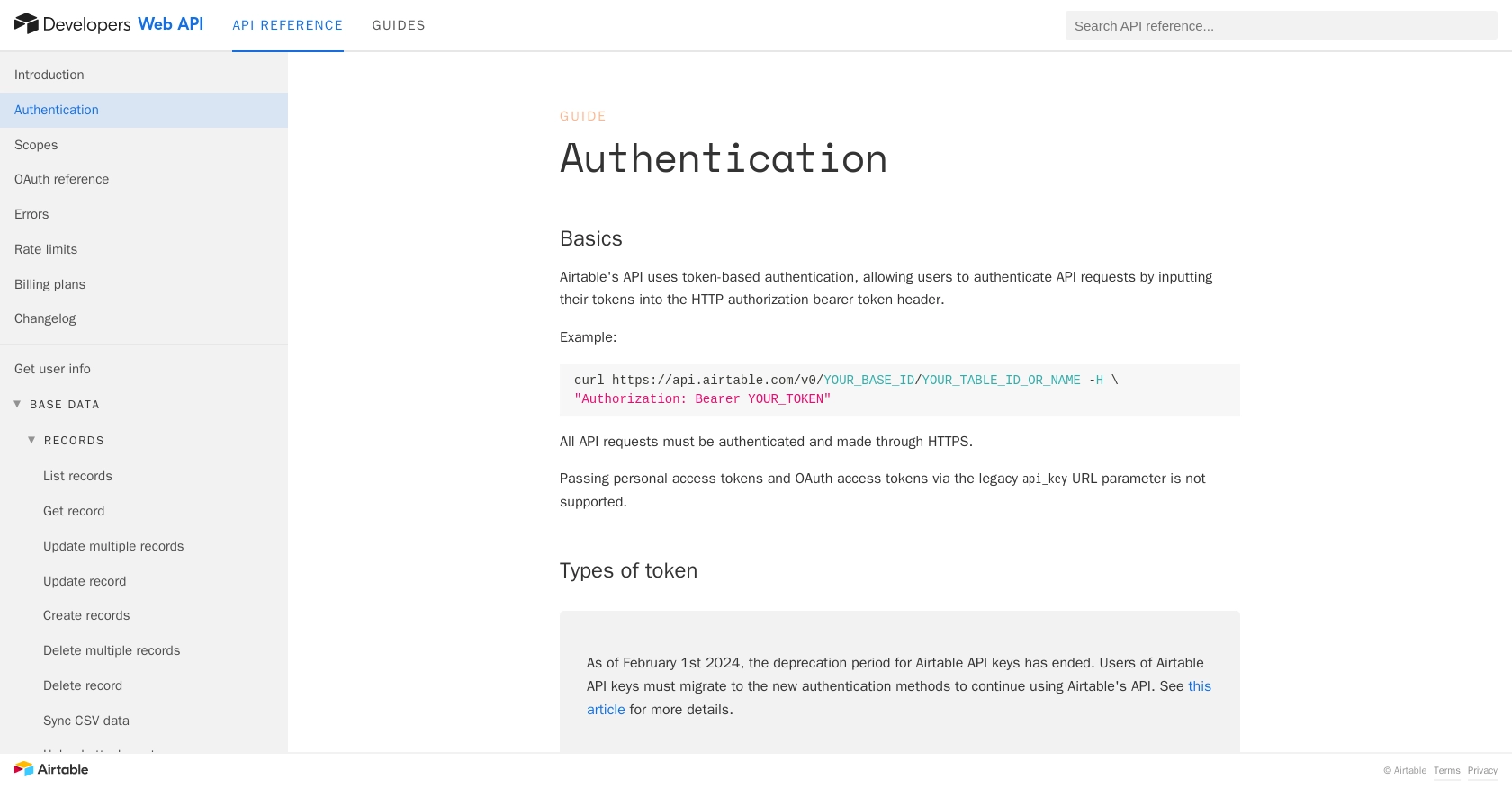
sbb-itb-96038d7
Making API Calls to Retrieve Records from Airtable Using PHP
To interact with Airtable's API using PHP, you'll need to set up your environment with the necessary tools and libraries. This section will guide you through the process of making API calls to retrieve records from Airtable, ensuring you have the right setup and understanding of the code involved.
Setting Up Your PHP Environment for Airtable API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need PHP installed on your machine, along with the cURL extension, which is commonly used for making HTTP requests.
- Ensure PHP is installed by running
php -v
in your terminal. - Verify that the cURL extension is enabled by checking your
php.ini
file or runningphp -m
to list installed modules.
Installing Required PHP Libraries for Airtable API
To simplify HTTP requests in PHP, you can use libraries like Guzzle. Install Guzzle using Composer, a dependency manager for PHP:
composer require guzzlehttp/guzzle
Example Code to Retrieve Records from Airtable
With your environment set up, you can now write PHP code to retrieve records from Airtable. Below is an example script that demonstrates how to make a GET request to the Airtable API:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$baseId = 'YOUR_BASE_ID';
$tableName = 'YOUR_TABLE_NAME';
$accessToken = 'YOUR_ACCESS_TOKEN';
$response = $client->request('GET', "https://api.airtable.com/v0/{$baseId}/{$tableName}", [
'headers' => [
'Authorization' => "Bearer {$accessToken}"
]
]);
$data = json_decode($response->getBody(), true);
foreach ($data['records'] as $record) {
echo 'Record ID: ' . $record['id'] . "\n";
echo 'Fields: ' . json_encode($record['fields']) . "\n\n";
}
Replace YOUR_BASE_ID
, YOUR_TABLE_NAME
, and YOUR_ACCESS_TOKEN
with your actual Airtable base ID, table name, and OAuth access token.
Understanding the PHP Code for Airtable API Calls
In the example above, we use Guzzle to make an HTTP GET request to the Airtable API. The request includes an authorization header with your access token. The response is then decoded from JSON format, and the records are iterated over to display their IDs and fields.
Verifying Successful API Requests and Handling Errors
After running the script, you should see the records from your Airtable base printed in the console. If the request fails, ensure your access token is valid and that you have the necessary permissions for the base.
Handle errors by checking the response status code. Airtable's API returns specific error codes for various issues, such as:
- 401 Unauthorized: Invalid or missing access token.
- 403 Forbidden: Insufficient permissions to access the resource.
- 429 Too Many Requests: Rate limit exceeded. Wait before retrying.
For more details on error handling, refer to the Airtable API error documentation.
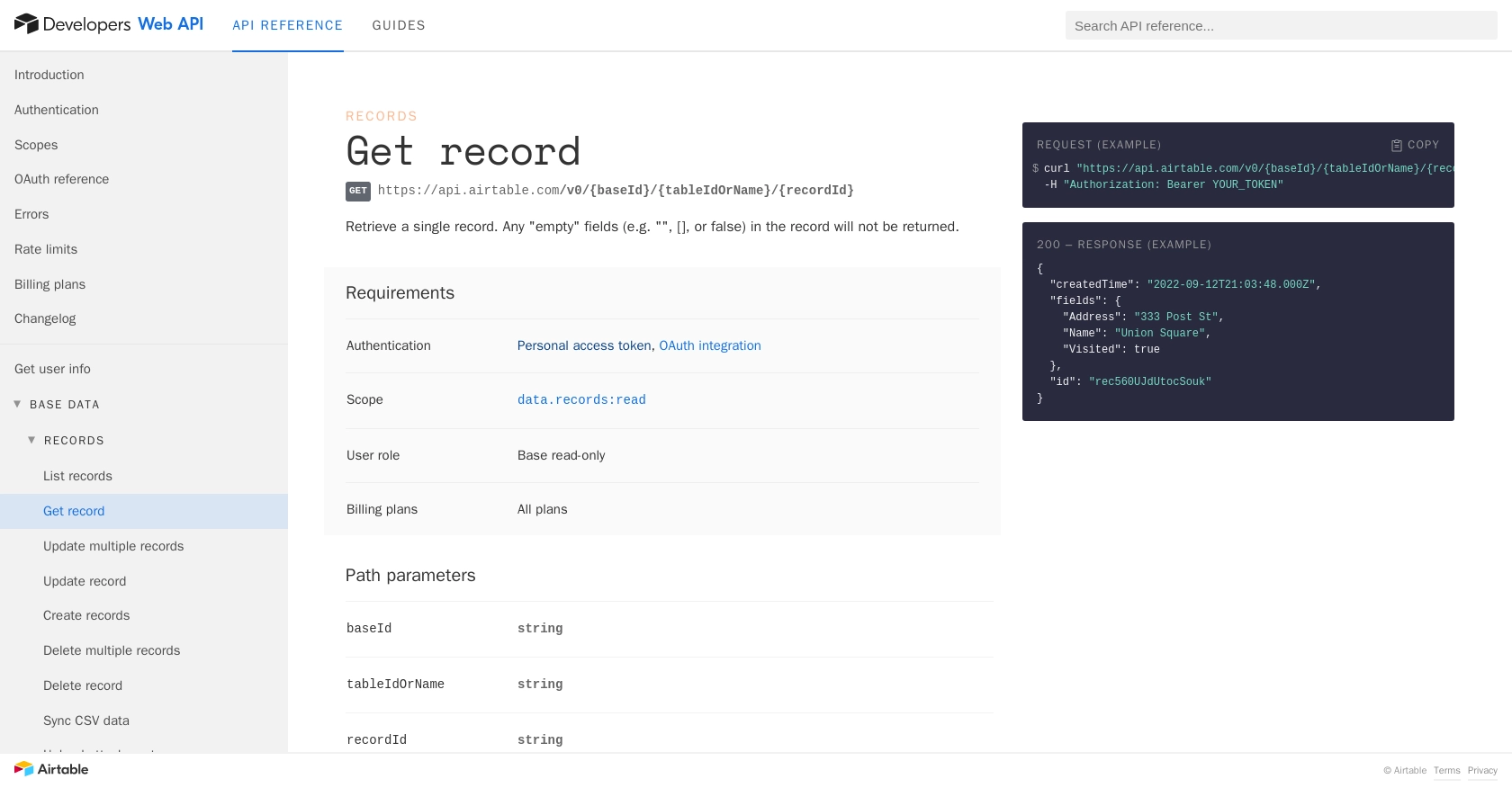
Conclusion and Best Practices for Using Airtable API with PHP
Integrating with the Airtable API using PHP can significantly enhance your application's data management capabilities, allowing for seamless automation and real-time data retrieval. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate using OAuth, and interact with Airtable's robust API to fetch records.
Best Practices for Secure and Efficient Airtable API Integration
- Securely Store Credentials: Always store your OAuth access tokens securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limits: Airtable's API enforces a rate limit of 5 requests per second per base. Implement back-off and retry logic to handle 429 status codes gracefully. For more details, refer to the Airtable API rate limits documentation.
- Optimize Data Retrieval: Use query parameters to filter and sort data efficiently, reducing the amount of data transferred and improving performance.
- Monitor API Usage: Regularly review your API usage to ensure compliance with Airtable's terms and to optimize your integration's performance.
Enhancing Your Integration with Endgrate
While integrating with Airtable's API directly is powerful, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Airtable. By using Endgrate, you can streamline your integration efforts, focus on your core product, and deliver an intuitive experience for your users.
Explore how Endgrate can transform your integration strategy by visiting Endgrate and discover how you can build once and deploy across multiple platforms effortlessly.
Read More
- https://endgrate.com/provider/airtable
- https://airtable.com/developers/web/api/authentication
- https://airtable.com/developers/web/api/scopes
- https://airtable.com/developers/web/api/oauth-reference
- https://airtable.com/developers/web/api/errors
- https://airtable.com/developers/web/api/rate-limits
- https://airtable.com/developers/web/api/get-record
Ready to get started?