How to Create or Update Customers with the Zoho Books API in Javascript
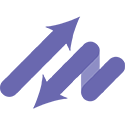
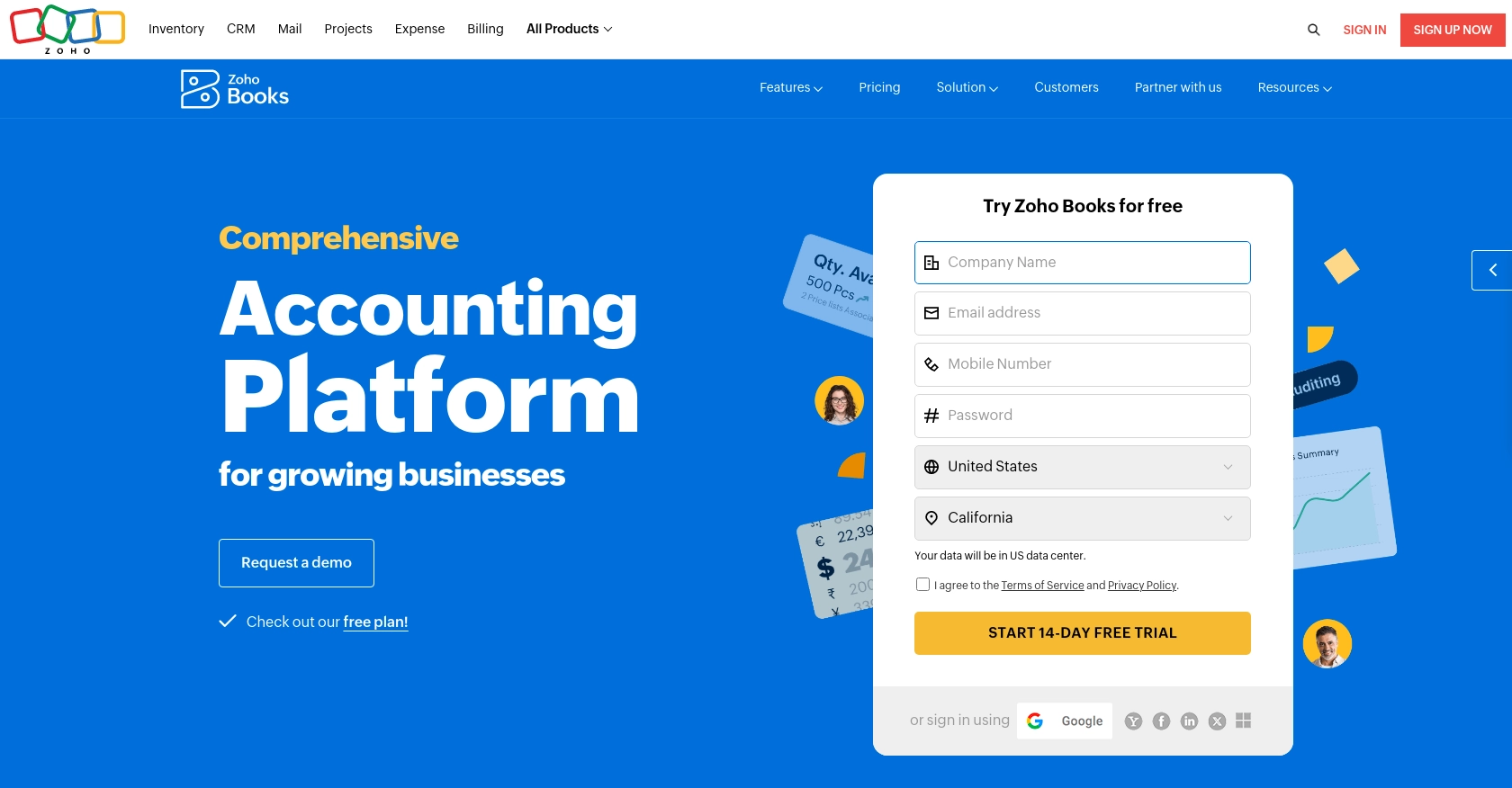
Introduction to Zoho Books API Integration
Zoho Books is a comprehensive online accounting software designed to manage your finances, automate business workflows, and help you work collectively across departments. It offers a wide range of features including invoicing, expense tracking, and inventory management, making it a popular choice for businesses of all sizes.
Integrating with the Zoho Books API allows developers to automate and streamline financial operations, such as creating or updating customer records. For example, you can automate the process of adding new customers from an e-commerce platform to Zoho Books, ensuring that your financial data is always up-to-date and accurate.
This article will guide you through the process of using JavaScript to interact with the Zoho Books API, specifically focusing on creating or updating customer records. By following this tutorial, you'll be able to efficiently manage customer data within Zoho Books, enhancing your business's financial operations.
Setting Up Your Zoho Books Test/Sandbox Account
Before you can start integrating with the Zoho Books API, you'll need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting your live data. Zoho Books offers a free trial that you can use for this purpose.
Step-by-Step Guide to Creating a Zoho Books Account
- Visit the Zoho Books website and sign up for a free trial account.
- Follow the on-screen instructions to complete the registration process. You'll need to provide basic information such as your name, email address, and company details.
- Once your account is created, log in to access the Zoho Books dashboard.
Setting Up OAuth for Zoho Books API Access
Zoho Books uses OAuth 2.0 for authentication, ensuring secure access to your data. Follow these steps to set up OAuth for your application:
- Navigate to the Zoho Developer Console.
- Click on Add Client ID to register your application.
- Fill in the required details, including the redirect URI, which should match the URL where you want Zoho to redirect after authentication.
- After registration, you'll receive a Client ID and Client Secret. Keep these credentials secure.
Generating OAuth Tokens for Zoho Books API
With your Client ID and Client Secret, you can generate OAuth tokens to authenticate API requests:
- Redirect users to the following URL for authorization:
- Upon user consent, Zoho will redirect to your specified URI with a code parameter.
- Exchange this code for an access token by making a POST request:
- Store the received access_token and refresh_token securely for future API calls.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.contacts.CREATE,ZohoBooks.contacts.UPDATE&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
For more detailed information on OAuth setup, refer to the Zoho Books OAuth documentation.
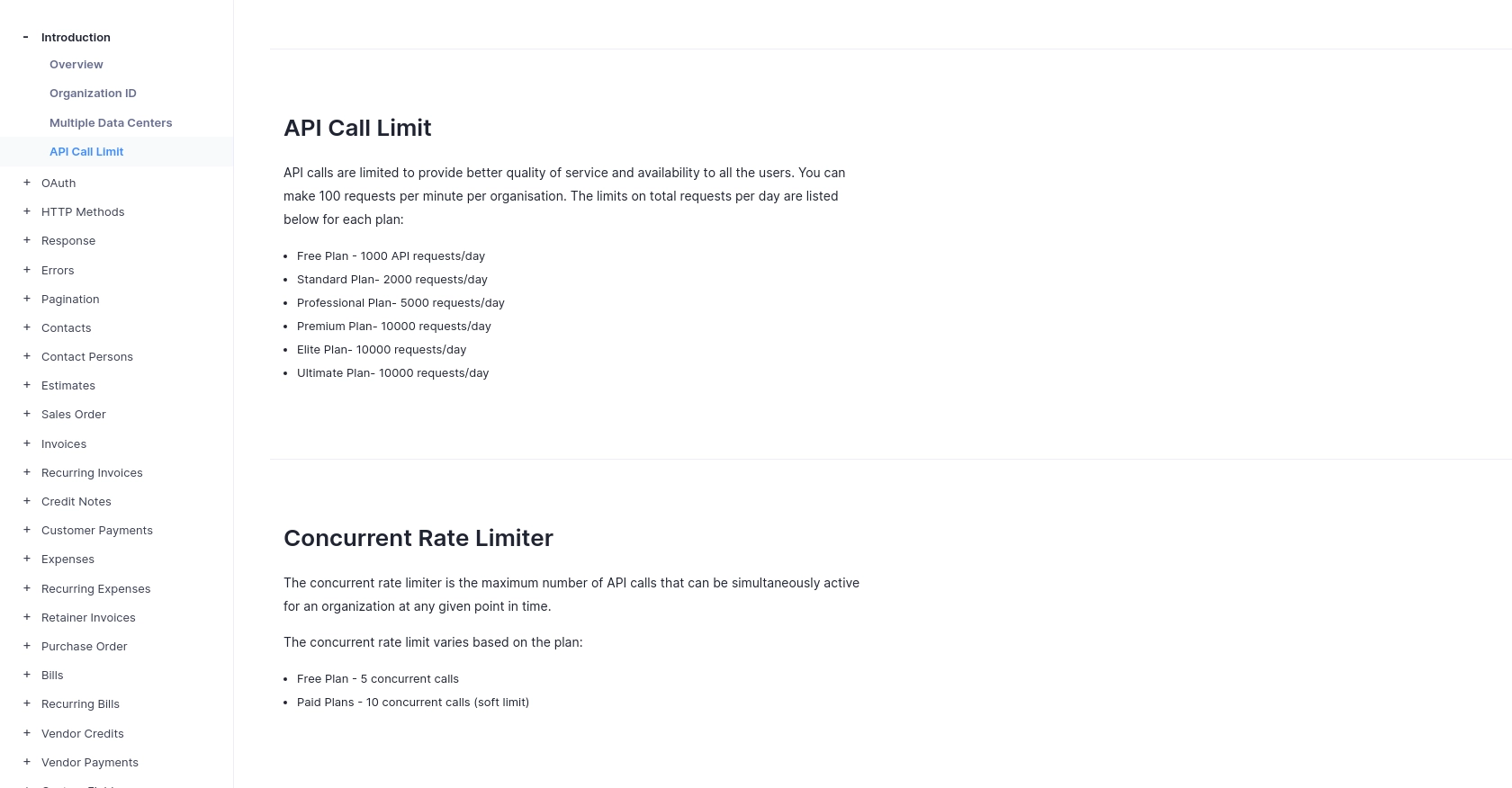
sbb-itb-96038d7
Making API Calls to Create or Update Customers in Zoho Books Using JavaScript
To interact with the Zoho Books API for creating or updating customer records, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process, including setting up your environment, writing the code, and handling responses.
Setting Up Your JavaScript Environment for Zoho Books API Integration
Before you begin, ensure you have Node.js installed on your machine. You can download it from the official Node.js website. Node.js will allow you to run JavaScript code outside of a browser.
You'll also need to install the node-fetch
package to make HTTP requests. Run the following command in your terminal:
npm install node-fetch
Writing JavaScript Code to Create a Customer in Zoho Books
To create a new customer in Zoho Books, you'll need to send a POST request to the API endpoint. Here's a sample code snippet to help you get started:
const fetch = require('node-fetch');
const createCustomer = async () => {
const url = 'https://www.zohoapis.com/books/v3/contacts?organization_id=YOUR_ORG_ID';
const headers = {
'Authorization': 'Zoho-oauthtoken YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
const body = JSON.stringify({
contact_name: 'John Doe',
company_name: 'Doe Enterprises',
contact_type: 'customer'
});
try {
const response = await fetch(url, {
method: 'POST',
headers: headers,
body: body
});
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error creating customer:', error);
}
};
createCustomer();
Replace YOUR_ORG_ID
and YOUR_ACCESS_TOKEN
with your actual organization ID and access token. The contact_name
and company_name
fields are required to create a customer.
Updating an Existing Customer in Zoho Books Using JavaScript
To update an existing customer, you'll need to send a PUT request. Here's how you can do it:
const updateCustomer = async (contactId) => {
const url = `https://www.zohoapis.com/books/v3/contacts/${contactId}?organization_id=YOUR_ORG_ID`;
const headers = {
'Authorization': 'Zoho-oauthtoken YOUR_ACCESS_TOKEN',
'Content-Type': 'application/json'
};
const body = JSON.stringify({
contact_name: 'John Doe Updated',
company_name: 'Doe Enterprises Updated'
});
try {
const response = await fetch(url, {
method: 'PUT',
headers: headers,
body: body
});
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error updating customer:', error);
}
};
updateCustomer('CONTACT_ID');
Replace CONTACT_ID
with the ID of the customer you wish to update. Ensure that the fields you want to update are included in the request body.
Handling API Responses and Errors in Zoho Books Integration
After making an API call, it's crucial to handle the response correctly. Check the status code to determine if the request was successful. Zoho Books uses standard HTTP status codes:
- 200 - OK
- 201 - Created
- 400 - Bad Request
- 401 - Unauthorized
- 404 - Not Found
- 429 - Rate Limit Exceeded
- 500 - Internal Server Error
For more details on error handling, refer to the Zoho Books Error Documentation.
Verifying API Call Success in Zoho Books
To verify that your API call was successful, check the response data for confirmation messages or the presence of the newly created or updated customer in your Zoho Books account. You can also use the Zoho Books dashboard to manually verify the changes.
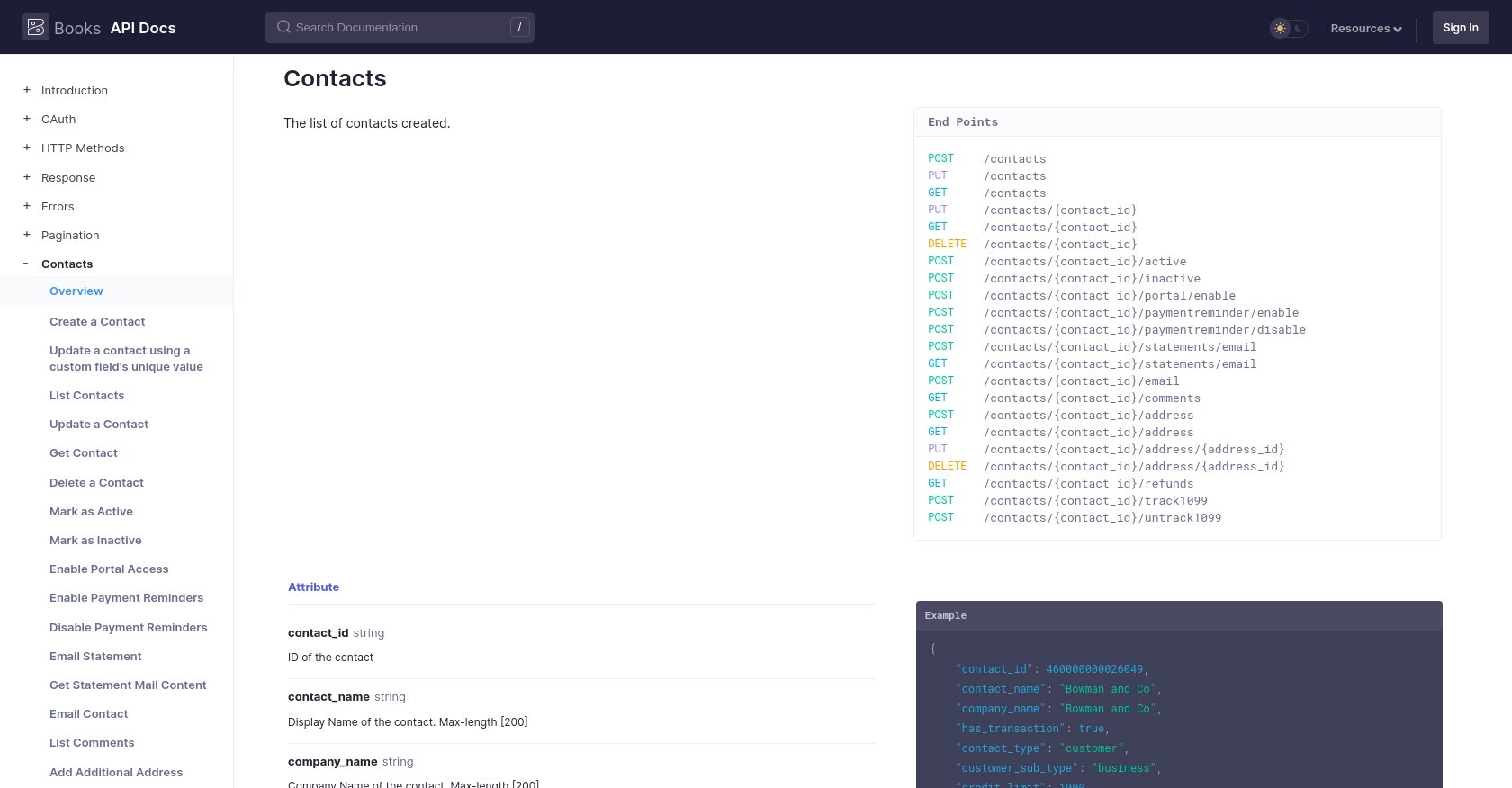
Conclusion and Best Practices for Zoho Books API Integration
Integrating with the Zoho Books API using JavaScript can significantly enhance your business's financial operations by automating customer management tasks. By following the steps outlined in this guide, you can efficiently create and update customer records, ensuring your financial data remains accurate and up-to-date.
Best Practices for Zoho Books API Integration
- Secure Storage of Credentials: Always store your OAuth tokens securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Zoho Books imposes a rate limit of 100 requests per minute per organization. Implement logic to handle rate limit errors (HTTP status code 429) by retrying requests after a delay. For more details, refer to the API Call Limit Documentation.
- Data Standardization: Ensure that the data you send to Zoho Books is standardized and validated to prevent errors. This includes formatting customer names, addresses, and other fields consistently.
- Error Handling: Implement robust error handling to manage different HTTP status codes. Refer to the Error Documentation for guidance on handling specific error codes.
Enhance Your Integration Experience with Endgrate
While integrating with Zoho Books API can streamline your operations, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product. With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers.
Explore how Endgrate can save you time and resources by visiting Endgrate's website today.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/contacts/#overview
Ready to get started?