Using the Teamleader API to Create Or Update Contacts in PHP
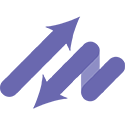
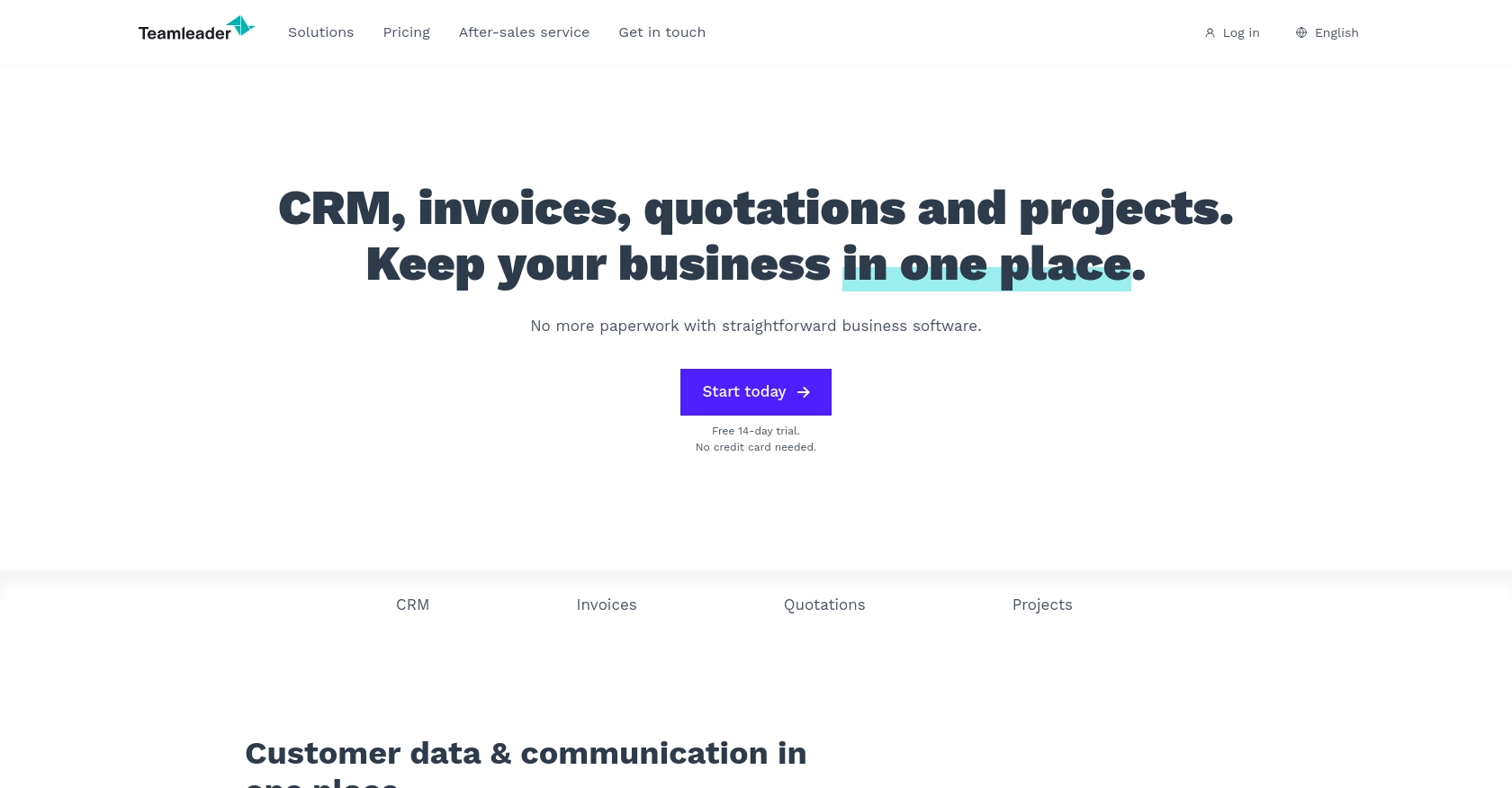
Introduction to Teamleader API
Teamleader is a comprehensive business management tool that combines CRM, project management, and invoicing functionalities into a single platform. It is designed to help small to medium-sized businesses streamline their operations and improve productivity.
Integrating with the Teamleader API allows developers to automate and enhance various business processes, such as managing customer contacts. For example, a developer might use the Teamleader API to create or update contact information directly from an external application, ensuring that customer data is always up-to-date and accessible.
Setting Up Your Teamleader Test/Sandbox Account
Before you can start integrating with the Teamleader API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting any live data.
Creating a Teamleader Account
If you don't already have a Teamleader account, you can sign up for a free trial on the Teamleader website. This trial will give you access to the necessary features to test the API integration.
- Visit the Teamleader website and click on the "Try for free" button.
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the Teamleader dashboard.
Setting Up OAuth Authentication for Teamleader API
The Teamleader API uses OAuth 2.0 for authentication, which requires you to create an app within your Teamleader account. This app will provide you with the client ID and client secret needed for API access.
- Navigate to the "Integrations" section in your Teamleader dashboard.
- Select "API & Webhooks" and then click on "Create a new app."
- Fill in the necessary details for your app, such as the name and description.
- After creating the app, you'll receive a client ID and client secret. Make sure to store these securely as you'll need them for authentication.
Generating Access Tokens
With your client ID and client secret, you can now generate access tokens to authenticate API requests.
- Use the OAuth 2.0 authorization flow to obtain an access token. This typically involves redirecting users to a Teamleader authorization URL where they can grant access to your app.
- Once the user authorizes the app, you'll receive an authorization code, which you can exchange for an access token using the client ID and client secret.
- Store the access token securely, as it will be used to authenticate your API requests.
For more detailed information on setting up OAuth authentication, refer to the Teamleader API Documentation.
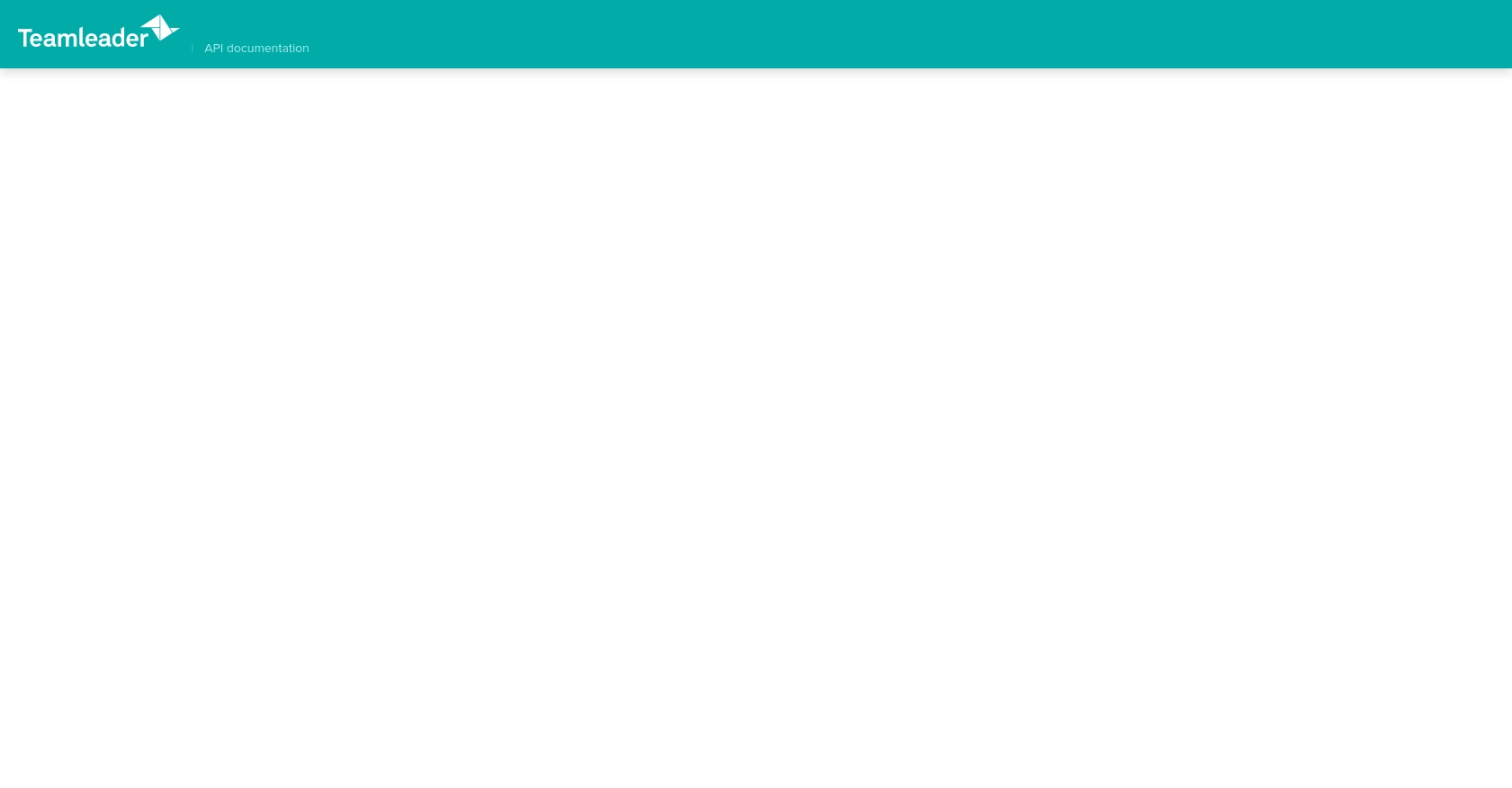
sbb-itb-96038d7
Making API Calls to Teamleader Using PHP
To interact with the Teamleader API using PHP, you'll need to set up your environment and write code to make HTTP requests. This section will guide you through the process of creating or updating contacts using the Teamleader API.
Setting Up Your PHP Environment
Before you begin, ensure you have PHP installed on your machine. You can download it from the official PHP website. Additionally, you'll need the cURL
extension enabled, as it is commonly used for making HTTP requests in PHP.
Installing Required PHP Dependencies
To make HTTP requests, we'll use the cURL
library, which is typically included with PHP. Ensure it's enabled in your php.ini
file:
extension=curl
Creating or Updating Contacts with Teamleader API
Now, let's write a PHP script to create or update contacts using the Teamleader API. Replace Your_Access_Token
with the access token you obtained during the OAuth setup.
<?php
// Set the API endpoint for creating or updating contacts
$endpoint = "https://api.teamleader.eu/contacts.createOrUpdate";
// Set the headers for the request
$headers = [
"Authorization: Bearer Your_Access_Token",
"Content-Type: application/json"
];
// Define the contact data
$data = [
"first_name" => "John",
"last_name" => "Doe",
"emails" => [
["type" => "primary", "email" => "john.doe@example.com"]
]
];
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Parse the response
$responseData = json_decode($response, true);
echo "Contact created or updated successfully: " . print_r($responseData, true);
}
// Close cURL session
curl_close($ch);
?>
Verifying the API Request Success
After running the script, you should see a response indicating that the contact was successfully created or updated. To verify, log into your Teamleader sandbox account and check the contacts section to ensure the data reflects the changes made by your script.
Handling Errors and Common Error Codes
When making API calls, it's crucial to handle potential errors. The Teamleader API may return various HTTP status codes to indicate success or failure. Here are some common codes:
- 200 OK: The request was successful.
- 400 Bad Request: The request was malformed or invalid.
- 401 Unauthorized: Authentication failed. Check your access token.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server.
For more detailed error handling, refer to the Teamleader API Documentation.
Conclusion and Best Practices for Using Teamleader API with PHP
Integrating with the Teamleader API using PHP allows developers to efficiently manage contact information, ensuring data consistency and accessibility across platforms. By following the steps outlined in this guide, you can create or update contacts seamlessly within your Teamleader account.
Best Practices for Storing User Credentials Securely
- Always store your client ID, client secret, and access tokens securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Regularly rotate your access tokens to minimize the risk of token compromise.
Handling Teamleader API Rate Limits
Be mindful of the Teamleader API's rate limits to avoid service disruptions. Implement logic to handle rate limit responses and retry requests after the specified wait time. For more details, refer to the Teamleader API Documentation.
Data Transformation and Standardization
Ensure that the data you send to the Teamleader API is properly formatted and standardized. This includes validating email formats, ensuring required fields are populated, and adhering to any specific data structure requirements outlined in the API documentation.
Streamlining Integrations with Endgrate
For developers looking to simplify their integration processes, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate provides a unified API endpoint that connects to multiple platforms, including Teamleader, offering an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website.
Read More
Ready to get started?