How to Get Product Variants with the Shopify API in Python
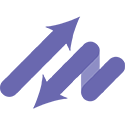
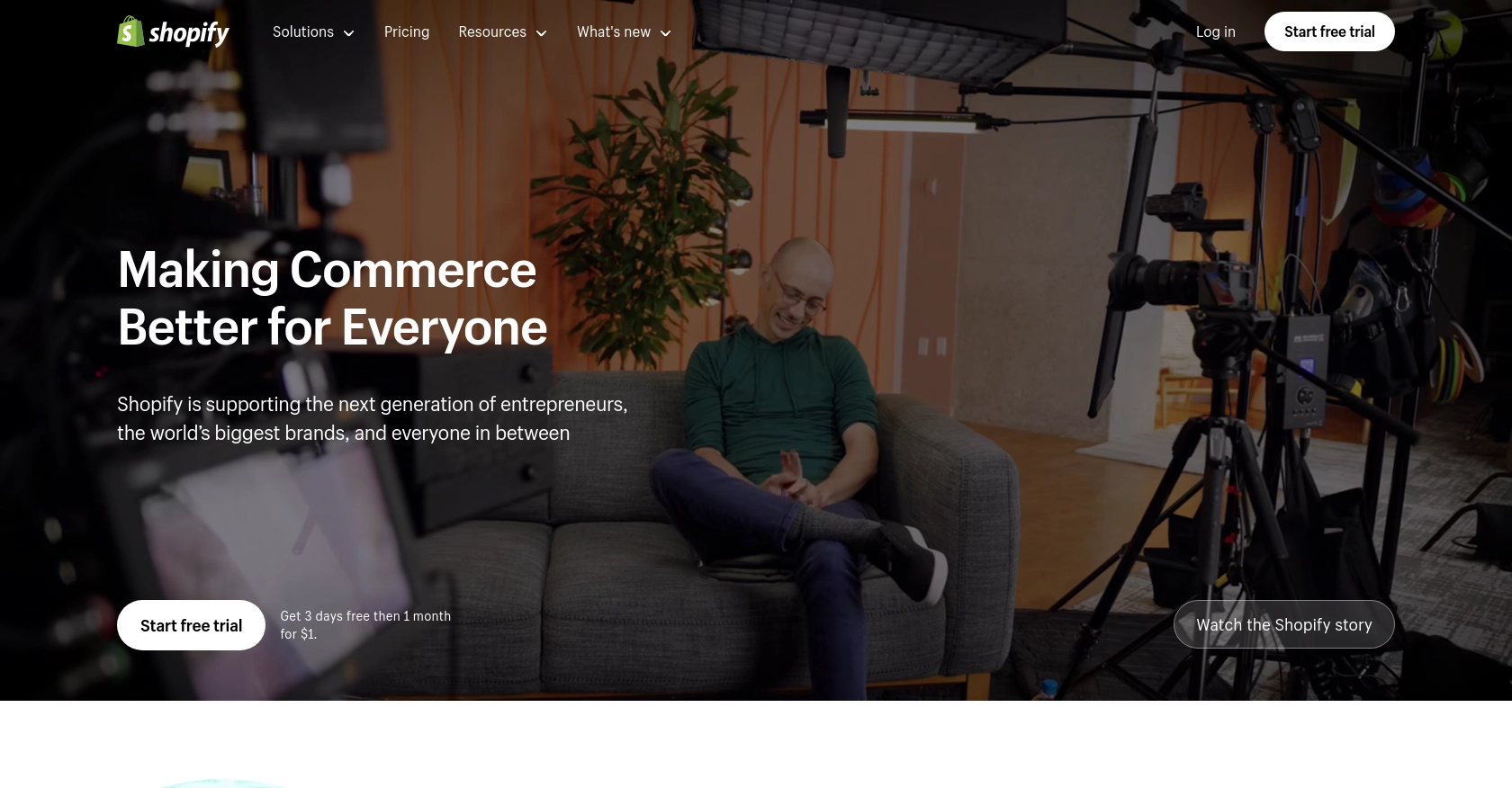
Introduction to Shopify API for Product Variants
Shopify is a leading e-commerce platform that empowers businesses to create and manage online stores with ease. It offers a robust set of tools and features, including inventory management, payment processing, and customer engagement, making it a preferred choice for entrepreneurs and enterprises alike.
For developers, integrating with Shopify's API can unlock powerful capabilities to enhance store functionality. One such integration involves accessing product variants, which are different versions of a product, such as size or color options. By leveraging the Shopify API, developers can efficiently manage these variants, ensuring accurate inventory tracking and seamless customer experiences.
For example, a developer might use the Shopify API to retrieve product variants in order to display real-time inventory levels on a storefront, helping customers make informed purchasing decisions. This article will guide you through the process of using Python to interact with the Shopify API and retrieve product variants, providing a practical solution for enhancing your e-commerce applications.
Setting Up Your Shopify Test Account for API Access
Before you can start interacting with the Shopify API to retrieve product variants, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting a live store.
Create a Shopify Partner Account
To begin, you need to create a Shopify Partner account. This account will enable you to create development stores, which are ideal for testing and development purposes.
- Visit the Shopify Partners page and sign up for a free account.
- Once registered, log in to your Shopify Partner dashboard.
Create a Development Store
With your Shopify Partner account set up, you can now create a development store:
- In the Shopify Partner dashboard, navigate to the "Stores" section.
- Click on "Add store" and select "Development store."
- Fill in the necessary details, such as store name and password, and click "Save."
Your development store is now ready for testing API integrations.
Set Up Shopify App for OAuth Authentication
Shopify uses OAuth for authentication, so you'll need to create an app to obtain the necessary credentials:
- In your Shopify Partner dashboard, go to "Apps" and click on "Create app."
- Enter the app name and select the development store you created earlier.
- Under "App setup," note down the API key and API secret key, as you'll need these for authentication.
Configure OAuth Scopes
To access product variants, you need to configure the appropriate OAuth scopes:
- In the app settings, navigate to "API scopes."
- Select the necessary scopes, such as "read_products" and "write_products," to access product data.
- Save your changes.
Generate Access Token
With your app configured, you can now generate an access token to authenticate API requests:
- Use the API key and secret key to initiate the OAuth flow.
- Follow the OAuth process to obtain an access token for your development store.
For detailed steps on OAuth authentication, refer to the Shopify authentication documentation.
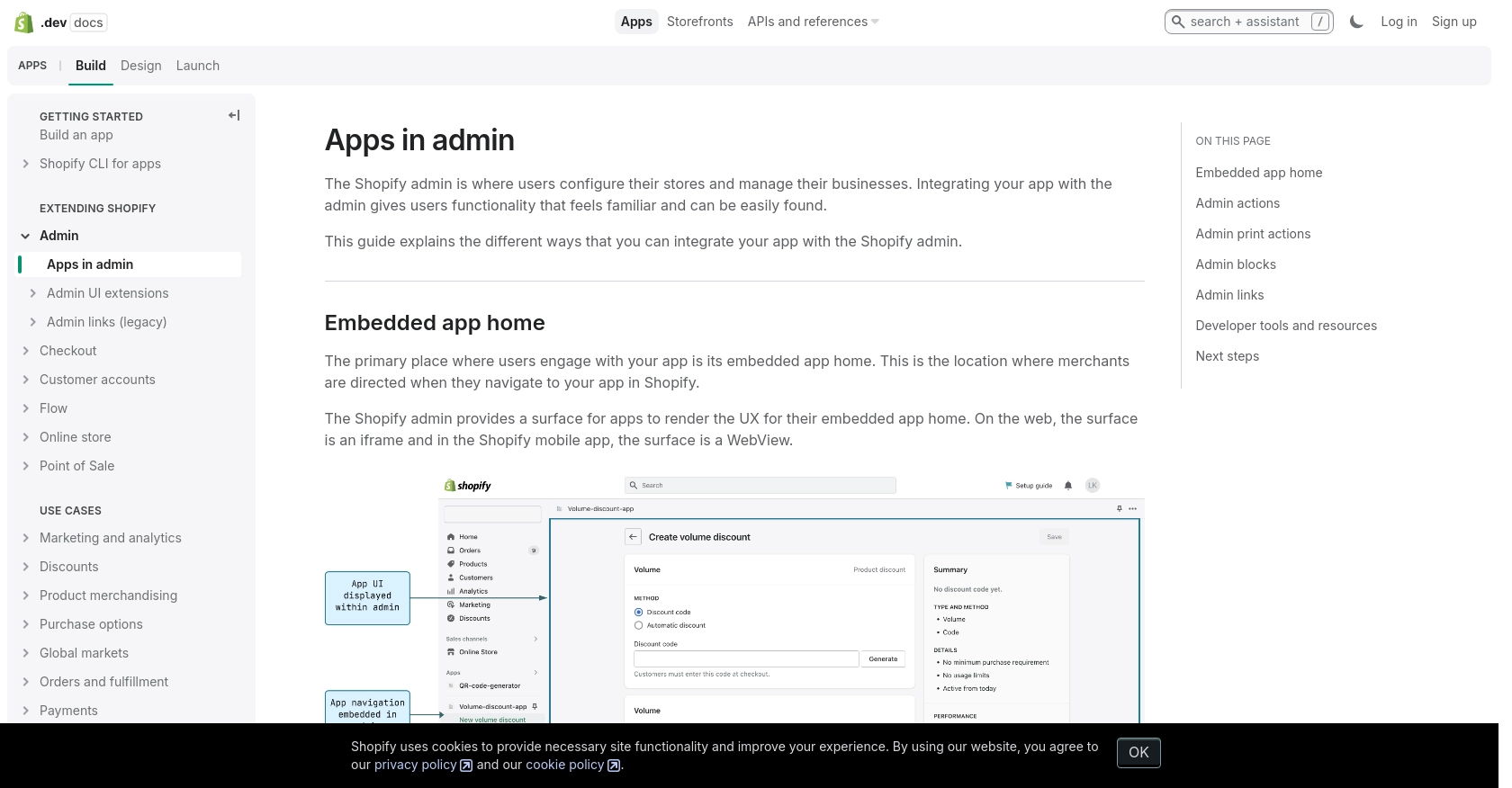
sbb-itb-96038d7
How to Make API Calls to Retrieve Product Variants with Shopify API in Python
To interact with the Shopify API and retrieve product variants, you'll need to use Python, a versatile programming language known for its simplicity and readability. This section will guide you through the process of setting up your Python environment, making API calls, and handling responses effectively.
Setting Up Your Python Environment for Shopify API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python from the official Python website if you haven't already.
- Use pip to install the
requests
library by running the following command in your terminal:
pip install requests
Making a GET Request to Shopify API to Retrieve Product Variants
With your environment set up, you can now write a Python script to retrieve product variants from Shopify. Create a file named get_product_variants.py
and add the following code:
import requests
# Define the API endpoint and headers
store_name = "your-development-store"
product_id = "632910392"
endpoint = f"https://{store_name}.myshopify.com/admin/api/2024-07/products/{product_id}/variants.json"
headers = {
"Content-Type": "application/json",
"X-Shopify-Access-Token": "Your_Access_Token"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
variants = response.json().get("variants", [])
for variant in variants:
print(f"Variant ID: {variant['id']}, Title: {variant['title']}, Price: {variant['price']}")
else:
print(f"Failed to retrieve variants. Status code: {response.status_code}")
Replace Your_Access_Token
with the access token obtained from the OAuth process. This script sends a GET request to the Shopify API to retrieve product variants for a specific product ID. If successful, it prints the variant details.
Verifying API Call Success and Handling Errors
After running your script, you should see the product variants printed in the console. If the request fails, the script will output the status code, helping you diagnose the issue.
Shopify API uses standard HTTP status codes to indicate success or failure. Here are some common error codes you might encounter:
- 401 Unauthorized: Incorrect authentication credentials.
- 403 Forbidden: Incorrect access scopes.
- 404 Not Found: The requested resource was not found.
- 429 Too Many Requests: Exceeded the rate limit. Refer to the Shopify rate limits documentation for more information.
For a complete list of status codes, refer to the Shopify status and error codes documentation.
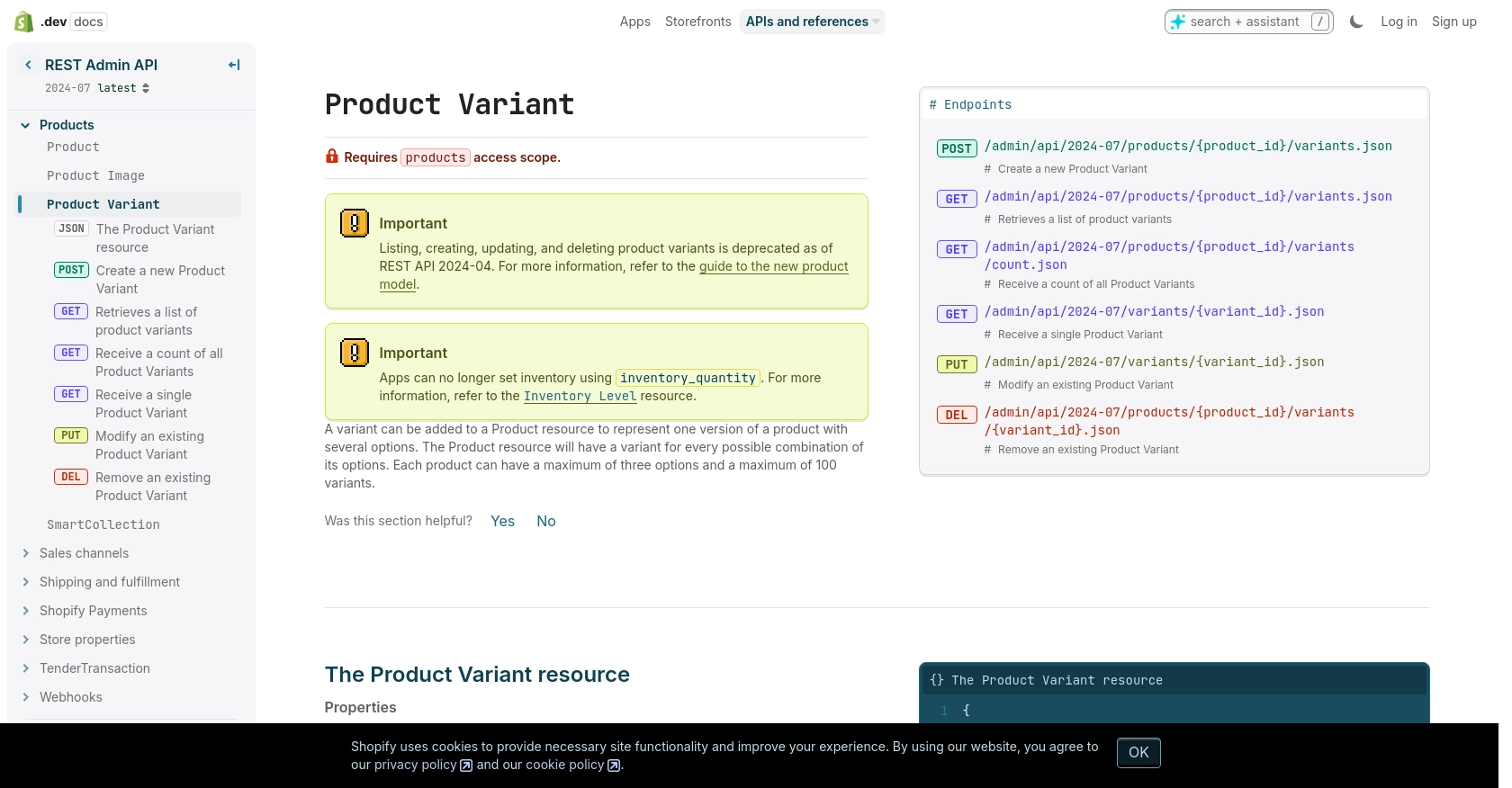
Conclusion and Best Practices for Integrating Shopify API with Python
Integrating with the Shopify API using Python provides developers with powerful tools to manage and enhance e-commerce functionalities. By retrieving product variants, developers can offer real-time inventory insights, improve customer experience, and streamline store operations.
Best Practices for Secure and Efficient Shopify API Integration
- Securely Store Credentials: Always store your API keys and access tokens securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limits: Shopify's API has a rate limit of 40 requests per app per store per minute. Implement logic to handle 429 errors by checking the
Retry-After
header and pausing requests accordingly. For more details, refer to the Shopify rate limits documentation. - Standardize Data Fields: Ensure that data retrieved from the API is standardized and consistent with your application's requirements. This helps maintain data integrity and improves user experience.
- Error Handling: Implement robust error handling to manage different HTTP status codes. This ensures that your application can gracefully handle issues like authentication errors or resource not found.
Streamlining Shopify Integrations with Endgrate
While building custom integrations with Shopify can be rewarding, it can also be time-consuming and complex. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including Shopify. This allows developers to focus on core product development while outsourcing integration complexities.
With Endgrate, you can build once for each use case instead of multiple times for different integrations, saving time and resources. Explore Endgrate to enhance your integration experience and provide seamless functionality for your customers.
Read More
Ready to get started?