Using the Mailshake API to Create Leads in Javascript
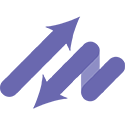
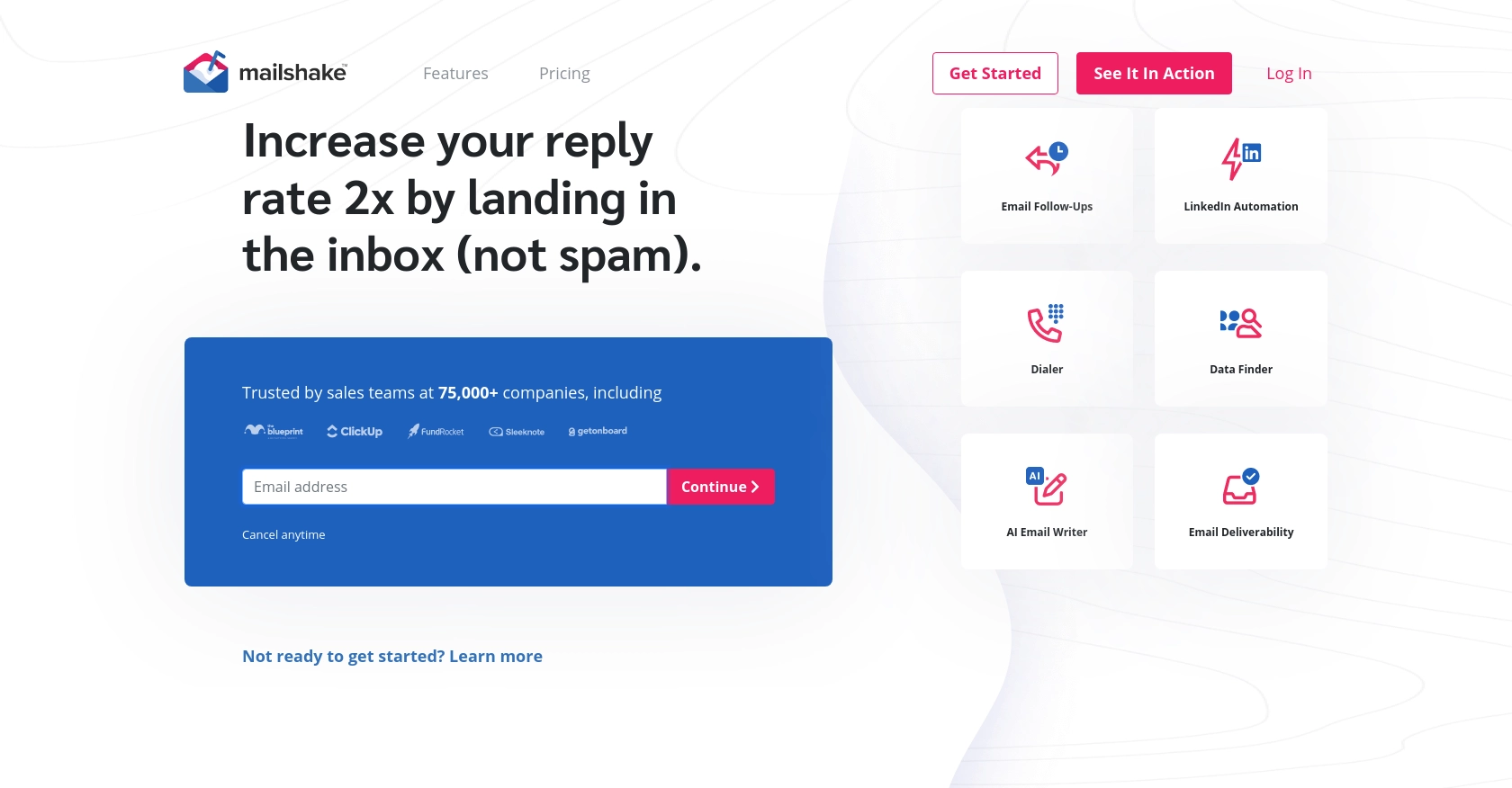
Introduction to Mailshake API for Lead Creation
Mailshake is a powerful sales engagement platform designed to help businesses streamline their outreach efforts through email campaigns and automated follow-ups. It offers a suite of tools that enable sales teams to connect with prospects, manage leads, and drive conversions efficiently.
Integrating with the Mailshake API allows developers to automate lead management processes, such as creating leads directly from email campaigns. For example, you can use the Mailshake API to automatically generate leads from recipients who engage with your email content, ensuring that your sales team can focus on high-potential prospects.
Setting Up Your Mailshake API Test Account
Before you can start creating leads using the Mailshake API, you need to set up your Mailshake account and obtain an API key. This key will allow you to authenticate your requests and interact with the Mailshake platform programmatically.
Creating a Mailshake Account
If you don't already have a Mailshake account, follow these steps to create one:
- Visit the Mailshake website and sign up for a free trial or choose a plan that suits your needs.
- Complete the registration process by providing the necessary information, such as your email address and password.
- Once your account is set up, log in to access the Mailshake dashboard.
Generating Your Mailshake API Key
To interact with the Mailshake API, you need to generate an API key. Follow these steps to obtain your key:
- Navigate to the Extensions section in the Mailshake dashboard.
- Select the API option from the menu.
- Click on Create API Key to generate a new key.
- Copy the generated API key and store it securely, as you will need it to authenticate your API requests.
Authenticating with the Mailshake API Using API Key
Mailshake uses simple API key-based authentication. You can include your API key in your requests in one of the following ways:
- As a query string parameter:
?apiKey=your-api-key
- In the request body as JSON:
{"apiKey": "your-api-key"}
- Via an HTTP Authorization header:
Authorization: Basic [base-64 encoded version of your api key]
Ensure you replace your-api-key
with the actual API key you generated.
With your Mailshake account set up and API key in hand, you're ready to start integrating and creating leads using the Mailshake API in JavaScript.
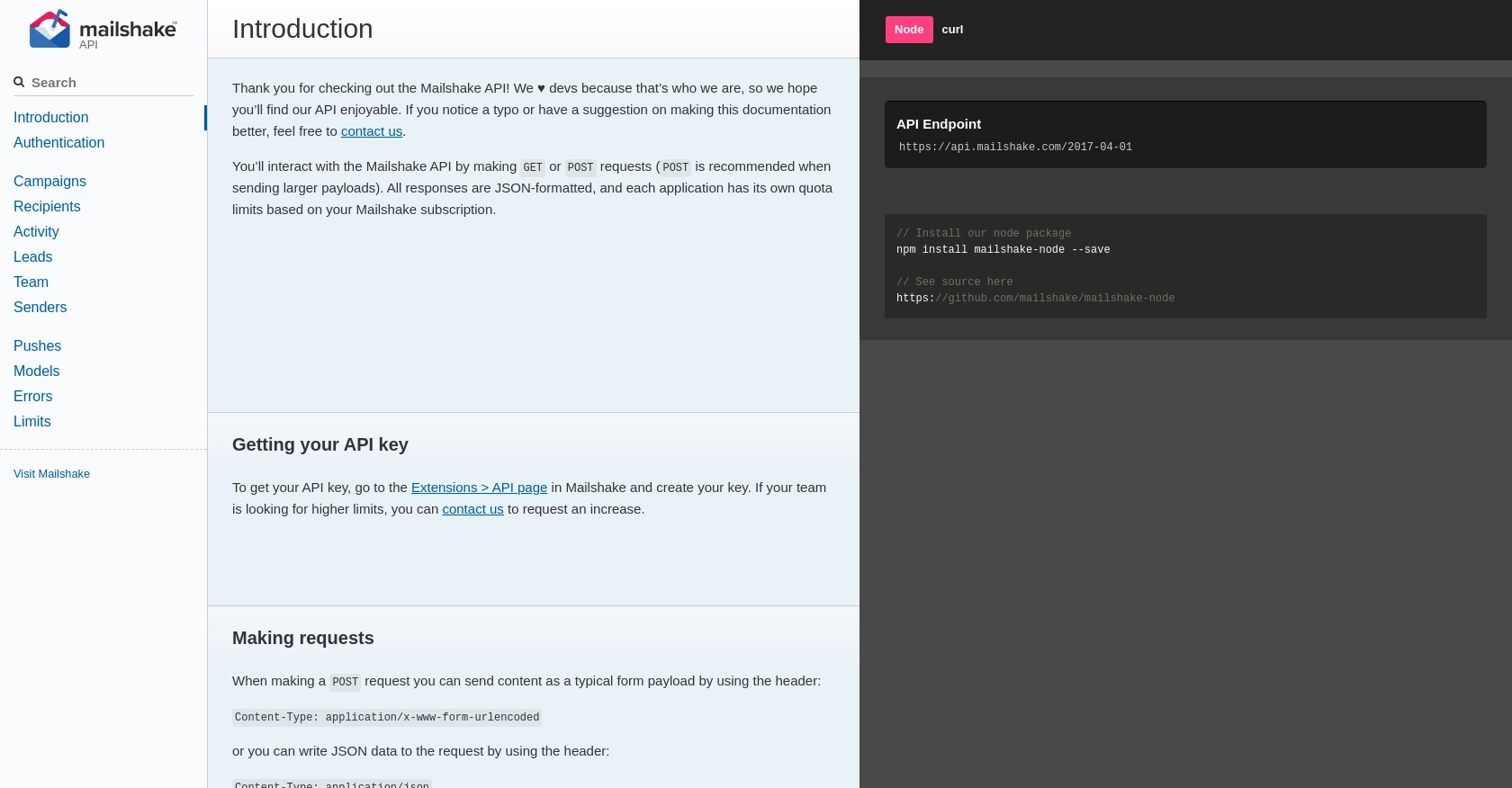
sbb-itb-96038d7
Making API Calls to Create Leads with Mailshake in JavaScript
To create leads using the Mailshake API in JavaScript, you'll need to set up your environment and write the necessary code to interact with the API. This section will guide you through the process, including setting up your JavaScript environment, writing the API call, and handling responses and errors.
Setting Up Your JavaScript Environment for Mailshake API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Node.js (version 14.x or later)
- NPM (Node Package Manager)
Once you have Node.js and NPM installed, you can create a new project directory and initialize it:
mkdir mailshake-integration
cd mailshake-integration
npm init -y
Next, install the axios
library, which will be used to make HTTP requests:
npm install axios
Writing the JavaScript Code to Create Leads Using Mailshake API
Now, create a new JavaScript file named createLeads.js
and add the following code:
const axios = require('axios');
// Set your Mailshake API key
const apiKey = 'your-api-key';
// Define the API endpoint for creating leads
const endpoint = 'https://api.mailshake.com/2017-04-01/leads/create';
// Set the request headers
const headers = {
'Content-Type': 'application/json',
'Authorization': `Basic ${Buffer.from(apiKey).toString('base64')}`
};
// Define the data for the leads you want to create
const data = {
campaignID: 1, // Replace with your campaign ID
emailAddresses: [
'lead1@example.com',
'lead2@example.com'
]
};
// Make the POST request to create leads
axios.post(endpoint, data, { headers })
.then(response => {
console.log('Leads created successfully:', response.data);
})
.catch(error => {
console.error('Error creating leads:', error.response ? error.response.data : error.message);
});
Replace your-api-key
with your actual Mailshake API key and campaignID
with the ID of the campaign you want to associate the leads with.
Handling Responses and Errors from Mailshake API
After making the API call, you should verify the response to ensure the leads were created successfully. The response will contain details about the created leads or any errors encountered.
If the request is successful, you'll see a message indicating the leads were created. If there's an error, the error message will help you diagnose the issue. Common errors include invalid API keys or missing parameters. Refer to the Mailshake API documentation for more details on error codes and handling.
Verifying Lead Creation in Mailshake Dashboard
To confirm that the leads were created, log in to your Mailshake dashboard and navigate to the Leads section. You should see the newly created leads listed there.
By following these steps, you can efficiently create leads using the Mailshake API in JavaScript, streamlining your sales engagement processes.
Conclusion and Best Practices for Using Mailshake API in JavaScript
Integrating the Mailshake API into your JavaScript applications can significantly enhance your sales engagement processes by automating lead creation and management. By following the steps outlined in this guide, you can efficiently create leads from your email campaigns, allowing your sales team to focus on high-potential prospects.
Best Practices for Secure and Efficient Mailshake API Integration
- Secure API Key Storage: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Mailshake's rate limits and plan your API calls accordingly. If you encounter a
limit_reached
error, refer to the error message for when you can retry. For more details, visit the Mailshake API documentation. - Data Standardization: Ensure that the data you send to Mailshake is standardized and validated to prevent errors such as
invalid_parameter
orinvalidEmails
.
Enhancing Your Integration Strategy with Endgrate
While integrating with Mailshake API can streamline your processes, managing multiple integrations can be complex. Endgrate offers a unified API solution that simplifies integration management across various platforms, including Mailshake. By using Endgrate, you can save time and resources, allowing your team to focus on core product development.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover a more intuitive integration experience for your customers.
Read More
Ready to get started?