How to Get Invoices with the Chargebee API in PHP
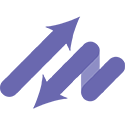
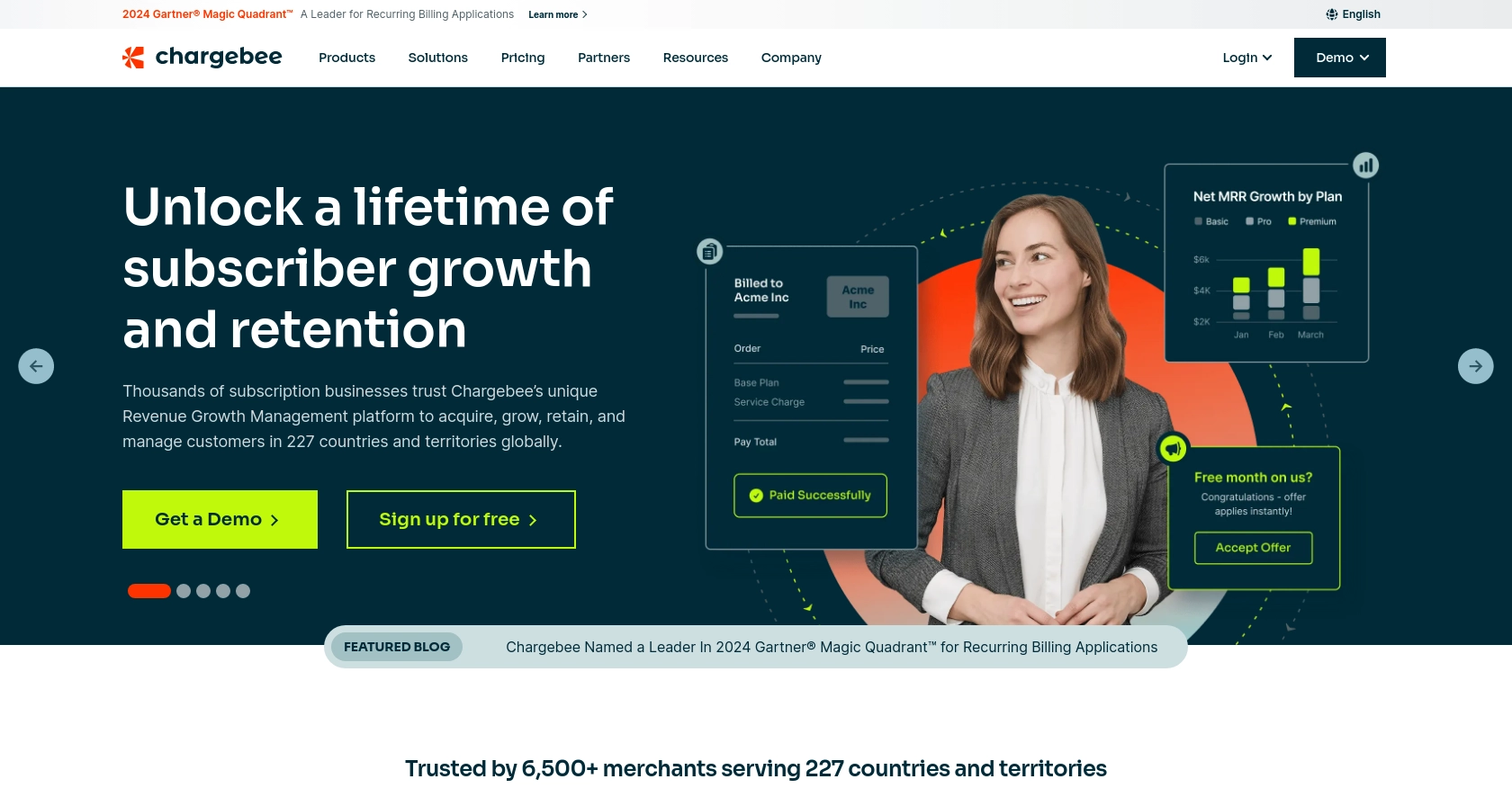
Introduction to Chargebee API for Invoice Management
Chargebee is a robust subscription management platform that simplifies billing, invoicing, and revenue operations for businesses. It offers a comprehensive API that allows developers to seamlessly integrate Chargebee's functionalities into their applications, enabling efficient management of customer subscriptions and financial transactions.
Integrating with Chargebee's API can significantly enhance a developer's ability to automate billing processes, such as retrieving invoices. For example, a developer might want to fetch a list of invoices to generate financial reports or to synchronize billing data with an internal accounting system. This integration can streamline operations and improve financial accuracy.
Setting Up Your Chargebee Test/Sandbox Account for API Integration
Before you can begin interacting with the Chargebee API to retrieve invoices, you need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data.
Creating a Chargebee Test Account
To get started, sign up for a Chargebee test account. This account will provide you with a sandbox environment where you can test API interactions:
- Visit the Chargebee Signup Page.
- Fill in the required details to create your account.
- Once registered, log in to access your Chargebee dashboard.
Generating API Keys for Chargebee
Chargebee uses HTTP Basic authentication for API calls, where the username is your API key and the password is left empty. Follow these steps to generate your API keys:
- Navigate to the Settings section in your Chargebee dashboard.
- Under API & Webhooks, select API Keys.
- Click on Create a Key and choose the appropriate permissions for your use case.
- Copy the generated API key and store it securely, as it will be used for authenticating your API requests.
Configuring Your Chargebee Sandbox Environment
Ensure your sandbox environment is configured to simulate real-world scenarios:
- Set up sample customers and subscriptions within the Chargebee dashboard.
- Create test invoices to verify API interactions.
- Utilize Chargebee's API Documentation to explore available endpoints and test various API calls.
With your Chargebee test account and API keys ready, you can now proceed to make API calls to retrieve invoices using PHP.
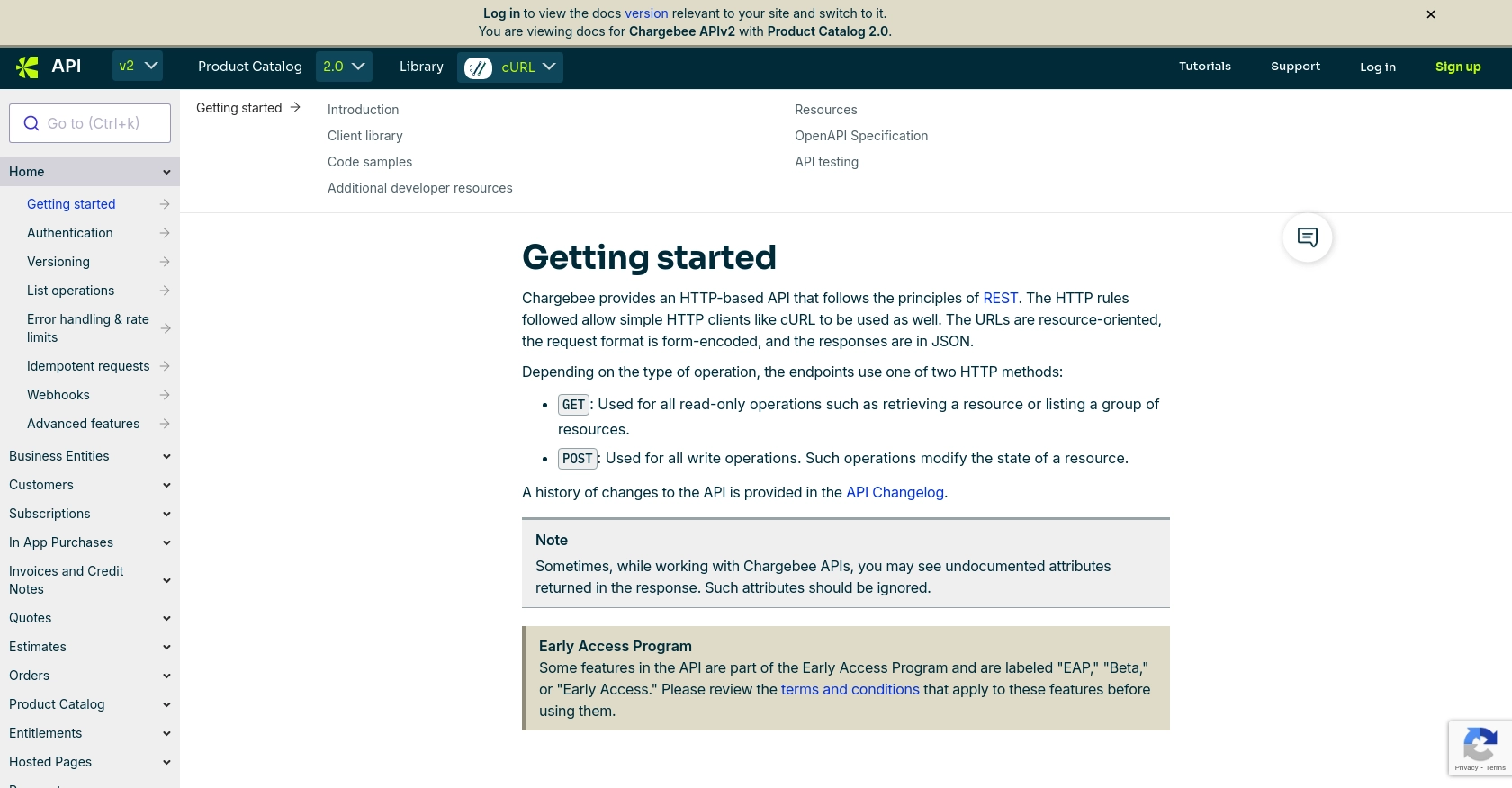
sbb-itb-96038d7
Making API Calls to Retrieve Invoices Using Chargebee API in PHP
To interact with the Chargebee API and retrieve invoices using PHP, you need to ensure your development environment is properly set up. This includes having the correct PHP version and necessary libraries installed.
Setting Up Your PHP Environment for Chargebee API Integration
Before making API calls, ensure your PHP environment is configured correctly:
- Ensure you have PHP 7.4 or later installed on your system.
- Install the
curl
extension for PHP, which is required for making HTTP requests. - Use Composer to manage dependencies and install the Chargebee PHP library:
composer require chargebee/chargebee-php
Writing PHP Code to Retrieve Invoices from Chargebee
With your environment set up, you can now write PHP code to interact with the Chargebee API. Below is a sample script to fetch a list of invoices:
require 'vendor/autoload.php';
use Chargebee\Chargebee\Environment;
use Chargebee\Chargebee\Invoice;
// Set your Chargebee site and API key
Environment::configure('your-site', 'your-api-key');
// Fetch invoices
try {
$invoices = Invoice::all([
'limit' => 5,
'status[in]' => ['paid', 'payment_due']
]);
foreach ($invoices->list as $invoice) {
echo "Invoice ID: " . $invoice->invoice()->id . "\n";
echo "Amount Due: " . $invoice->invoice()->amountDue . "\n";
}
} catch (Exception $e) {
echo "Error: " . $e->getMessage();
}
Replace your-site
and your-api-key
with your Chargebee site name and API key. This script retrieves a list of invoices with a status of 'paid' or 'payment_due' and displays their IDs and amounts due.
Verifying API Call Success and Handling Errors
After running the script, verify the success of your API call by checking the output. The retrieved invoices should match those in your Chargebee sandbox account. If there are any errors, they will be caught and displayed in the console.
Common error codes include:
- 401 Unauthorized: Check your API key and site name.
- 404 Not Found: Ensure the endpoint and parameters are correct.
- 429 Too Many Requests: You have exceeded the API rate limit. Refer to the Chargebee API documentation for more details.
For more information on error handling, visit the Chargebee API error handling documentation.
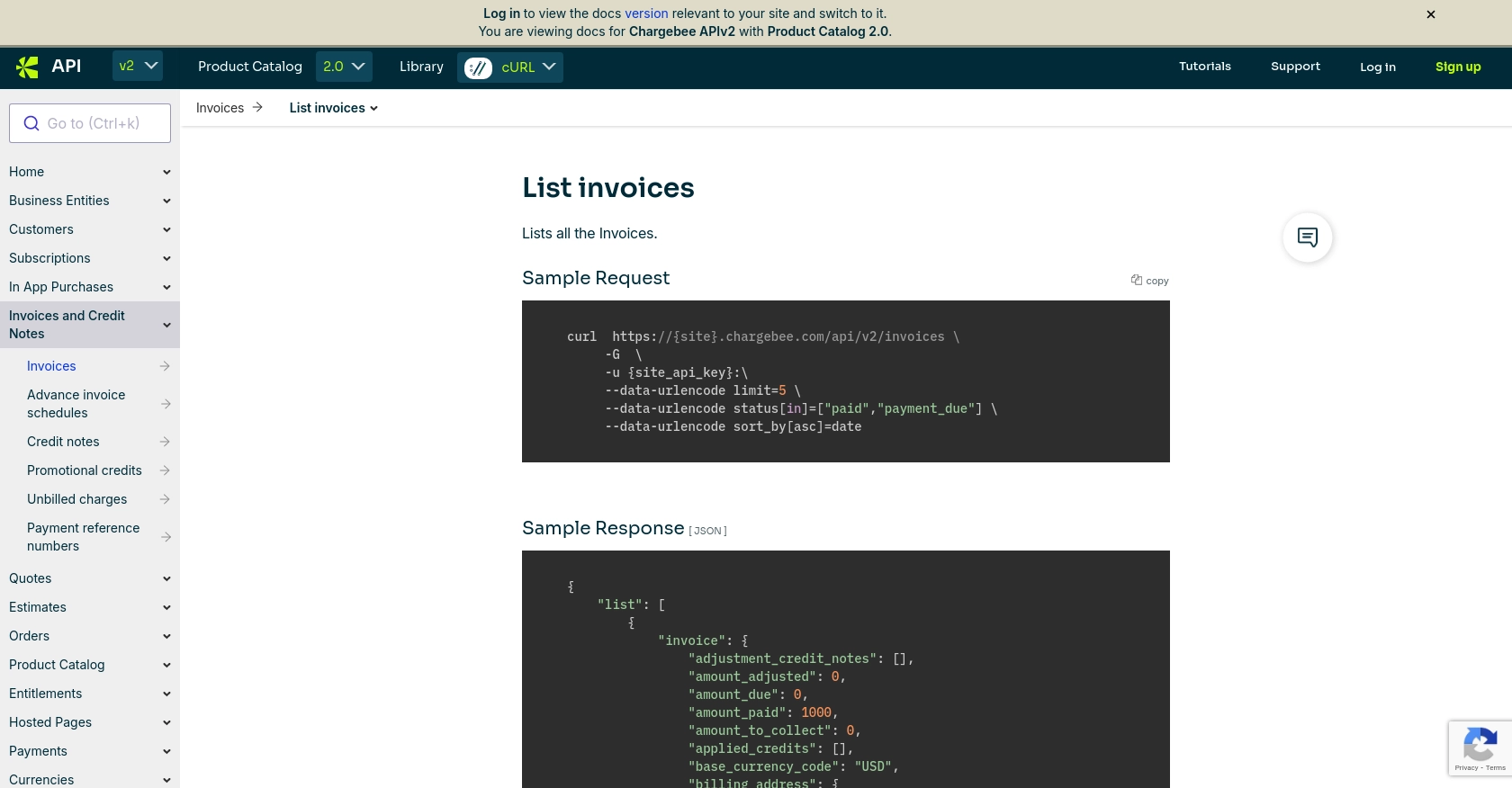
Conclusion and Best Practices for Using Chargebee API in PHP
Integrating Chargebee's API into your PHP applications can greatly enhance your ability to manage invoices and streamline billing processes. By following the steps outlined in this guide, you can efficiently retrieve invoices and handle billing data with ease.
Best Practices for Secure and Efficient Chargebee API Integration
- Secure API Keys: Store your API keys securely and avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limits: Be mindful of Chargebee's API rate limits. Implement retry logic with exponential backoff to handle HTTP 429 errors gracefully. For more details, refer to the Chargebee API documentation.
- Data Standardization: Ensure consistent data formats when integrating Chargebee with other systems. This helps maintain data integrity and simplifies data processing.
Enhance Your Integration Experience with Endgrate
For developers looking to streamline their integration processes further, consider using Endgrate. Endgrate allows you to build once for each use case and connect to multiple platforms through a single API endpoint. This can save time and resources, allowing you to focus on your core product.
Visit Endgrate to learn more about how you can simplify your integration efforts and provide an intuitive experience for your customers.
Read More
Ready to get started?