How to Get Responses with the Google Forms API in Javascript
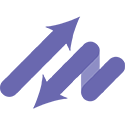
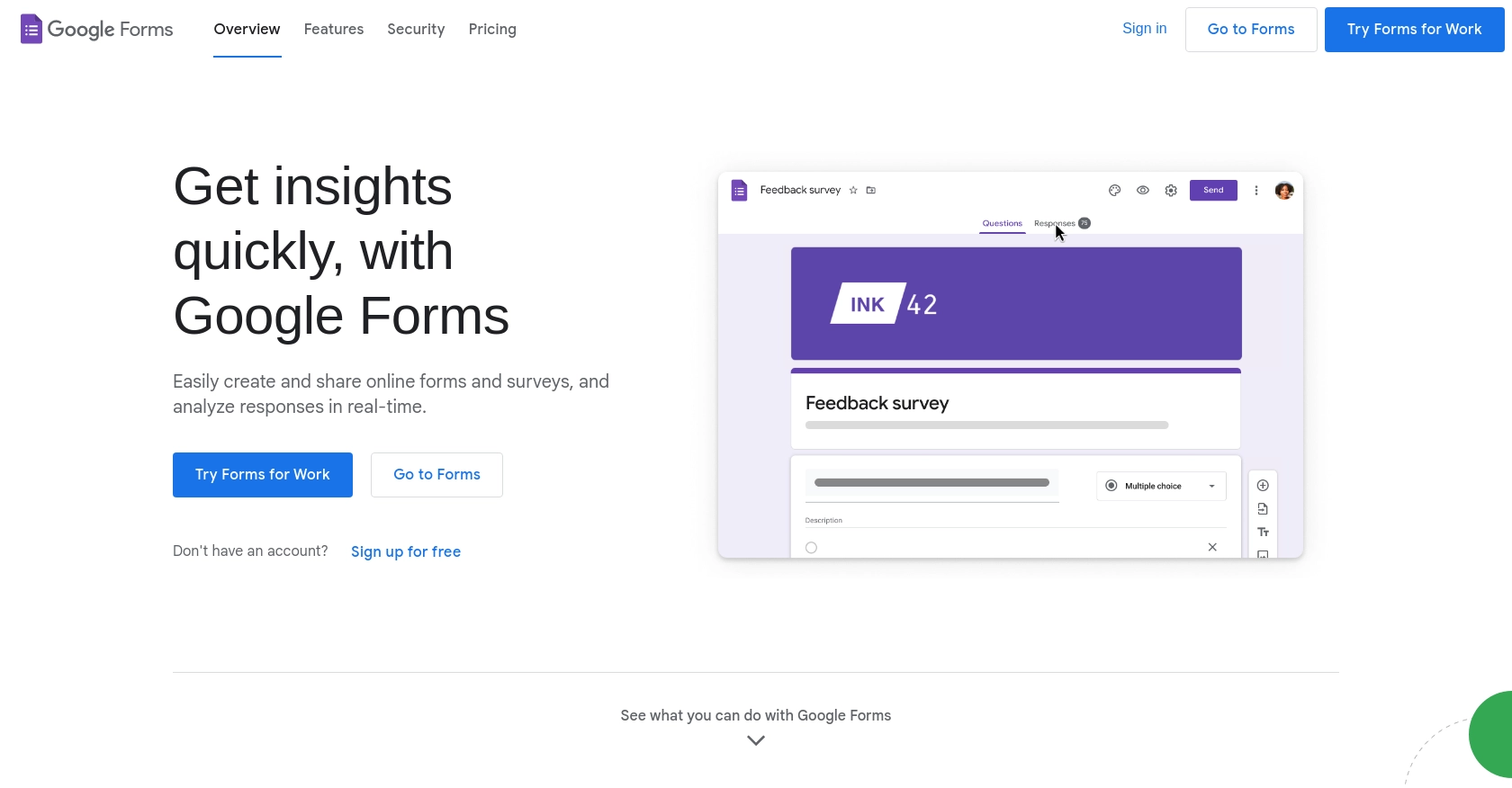
Introduction to Google Forms API
Google Forms is a versatile tool within the Google Workspace suite, allowing users to create surveys, quizzes, and forms with ease. Its integration capabilities make it an essential tool for businesses and developers looking to streamline data collection and analysis.
By integrating with the Google Forms API, developers can automate the retrieval and processing of form responses. This can be particularly useful for applications that require real-time data analysis or integration with other systems. For example, a developer might use the Google Forms API to automatically fetch survey responses and update a dashboard in real-time, enhancing decision-making processes.
Setting Up Your Google Forms API Test Account
Before you can start interacting with the Google Forms API, you need to set up a Google Cloud project and enable the necessary APIs. This process involves creating OAuth credentials to authenticate your requests. Follow these steps to get started:
Create a Google Cloud Project
- Go to the Google Cloud Console.
- Click on the project dropdown in the top navigation bar and select "New Project."
- Enter a name for your project and click "Create."
Enable Google Forms API
- In the Google Cloud Console, navigate to APIs & Services > Library.
- Search for "Google Forms API" and click on it.
- Click the "Enable" button to activate the API for your project.
Configure OAuth Consent Screen
- Go to APIs & Services > OAuth consent screen.
- Select the user type for your app and click "Create."
- Fill in the required fields and click "Save and Continue."
Create OAuth Credentials
- Navigate to APIs & Services > Credentials.
- Click "Create Credentials" and select "OAuth client ID."
- Choose "Web application" as the application type.
- Enter a name for the client ID and add authorized redirect URIs if needed.
- Click "Create" to generate your client ID and client secret.
Make sure to securely store your client ID and client secret, as you will need them to authenticate your API requests.
For more detailed instructions, refer to the official Google documentation on creating a Google Cloud project and configuring OAuth consent.
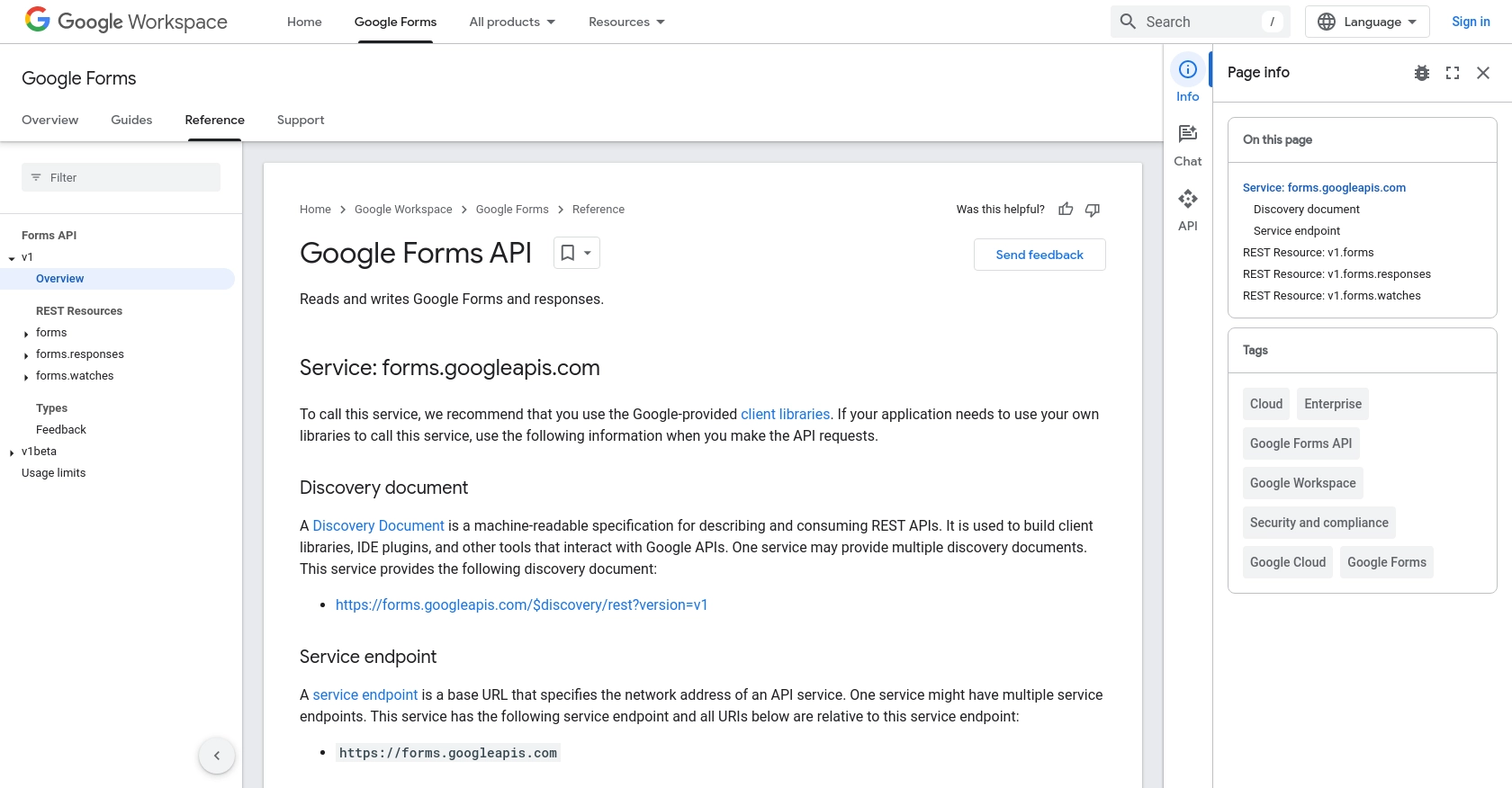
sbb-itb-96038d7
Making API Calls to Retrieve Google Forms Responses Using JavaScript
To interact with the Google Forms API and retrieve form responses, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling the responses effectively.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have a JavaScript environment set up. You can use Node.js or any browser-based environment. For this tutorial, we'll use Node.js. Ensure you have Node.js installed on your machine.
- Install Node.js from the official website if you haven't already.
- Create a new directory for your project and navigate into it using your terminal.
- Initialize a new Node.js project by running
npm init -y
. - Install the Axios library to handle HTTP requests by running
npm install axios
.
Writing JavaScript Code to Fetch Google Forms Responses
Now that your environment is set up, you can write the JavaScript code to interact with the Google Forms API. The following code demonstrates how to retrieve responses from a Google Form.
const axios = require('axios');
// Replace with your form ID and OAuth token
const formId = 'your_form_id';
const accessToken = 'your_access_token';
// Set the API endpoint
const endpoint = `https://forms.googleapis.com/v1/forms/${formId}/responses`;
// Make a GET request to the API
axios.get(endpoint, {
headers: {
'Authorization': `Bearer ${accessToken}`
}
})
.then(response => {
// Handle the successful response
console.log('Form Responses:', response.data);
})
.catch(error => {
// Handle errors
console.error('Error fetching responses:', error.response.data);
});
In this code, replace your_form_id
with the ID of your Google Form and your_access_token
with the OAuth token you obtained during the setup process. The code uses Axios to send a GET request to the Google Forms API endpoint, retrieving the form responses.
Verifying API Call Success and Handling Errors
After running the code, you should see the form responses printed in your console. If the request is successful, the data will include details such as response IDs, timestamps, and answers. You can verify the success by checking the output against the responses in your Google Forms dashboard.
To handle errors, the code includes a catch
block that logs any issues encountered during the API call. Common errors include invalid credentials or incorrect form IDs. Refer to the Google Forms API documentation for more information on error codes and troubleshooting.
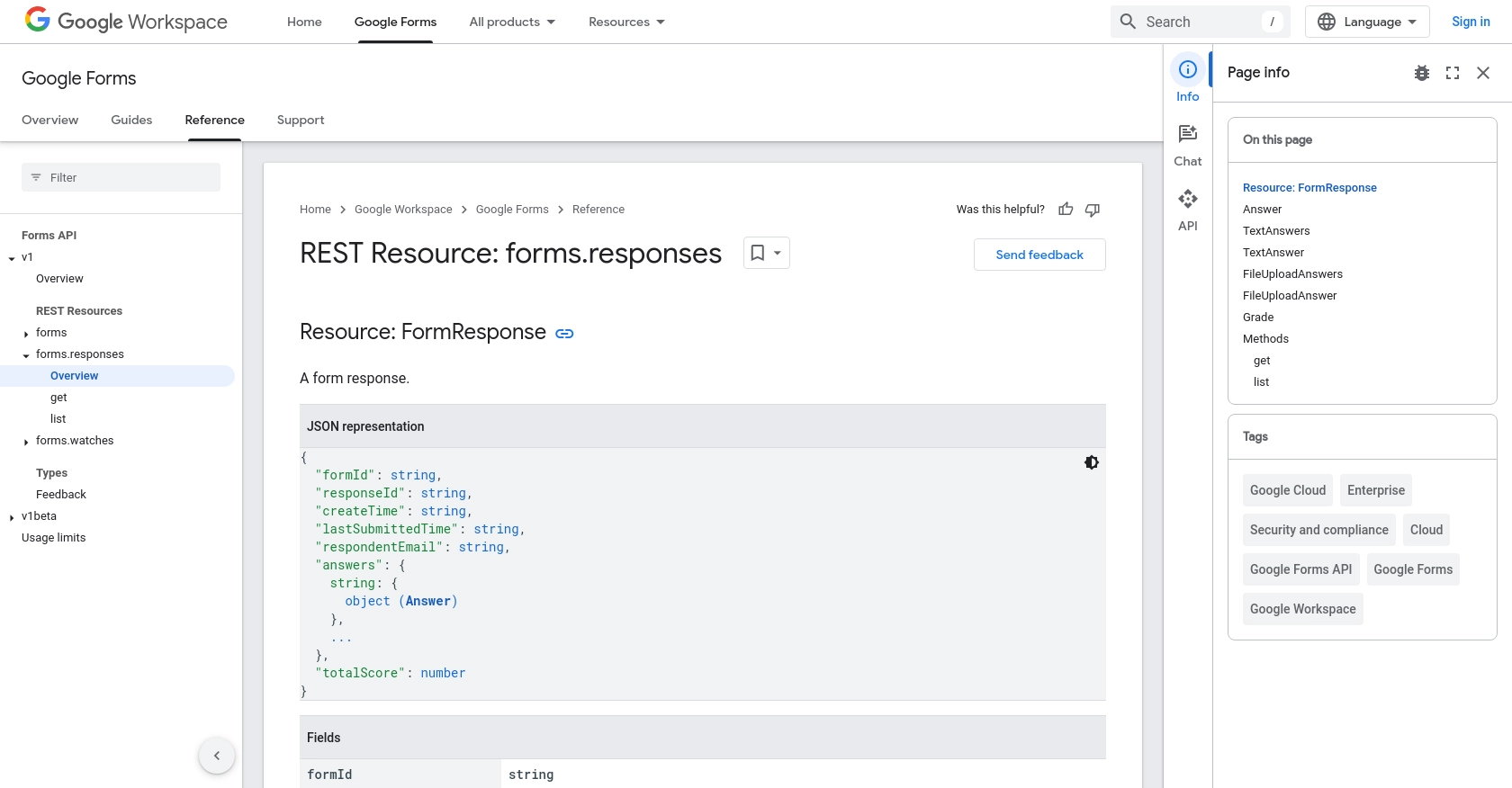
Conclusion and Best Practices for Using Google Forms API with JavaScript
Integrating with the Google Forms API using JavaScript provides developers with powerful capabilities to automate and streamline data collection processes. By following the steps outlined in this guide, you can efficiently retrieve form responses and integrate them into your applications, enhancing data-driven decision-making.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth client ID and client secret securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of Google's API rate limits to avoid exceeding the allowed number of requests. Implement exponential backoff strategies to handle retries gracefully.
- Data Transformation: Standardize and transform data fields as needed to ensure compatibility with your application’s data structure.
- Error Handling: Implement robust error handling to manage API errors effectively. Refer to the Google Forms API documentation for detailed error codes and resolutions.
Streamlining Integrations with Endgrate
While integrating with Google Forms API can be straightforward, managing multiple integrations can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Google Forms. By using Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can enhance your integration experience by visiting Endgrate's website. Leverage its intuitive platform to build once for each use case and enjoy a seamless integration experience for your customers.
Read More
- https://endgrate.com/provider/googleforms
- https://developers.google.com/forms/api/reference/rest
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/forms/api/reference/rest/v1/forms.responses
Ready to get started?