How to Get Tax with the Avalara API in PHP
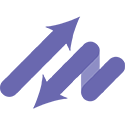
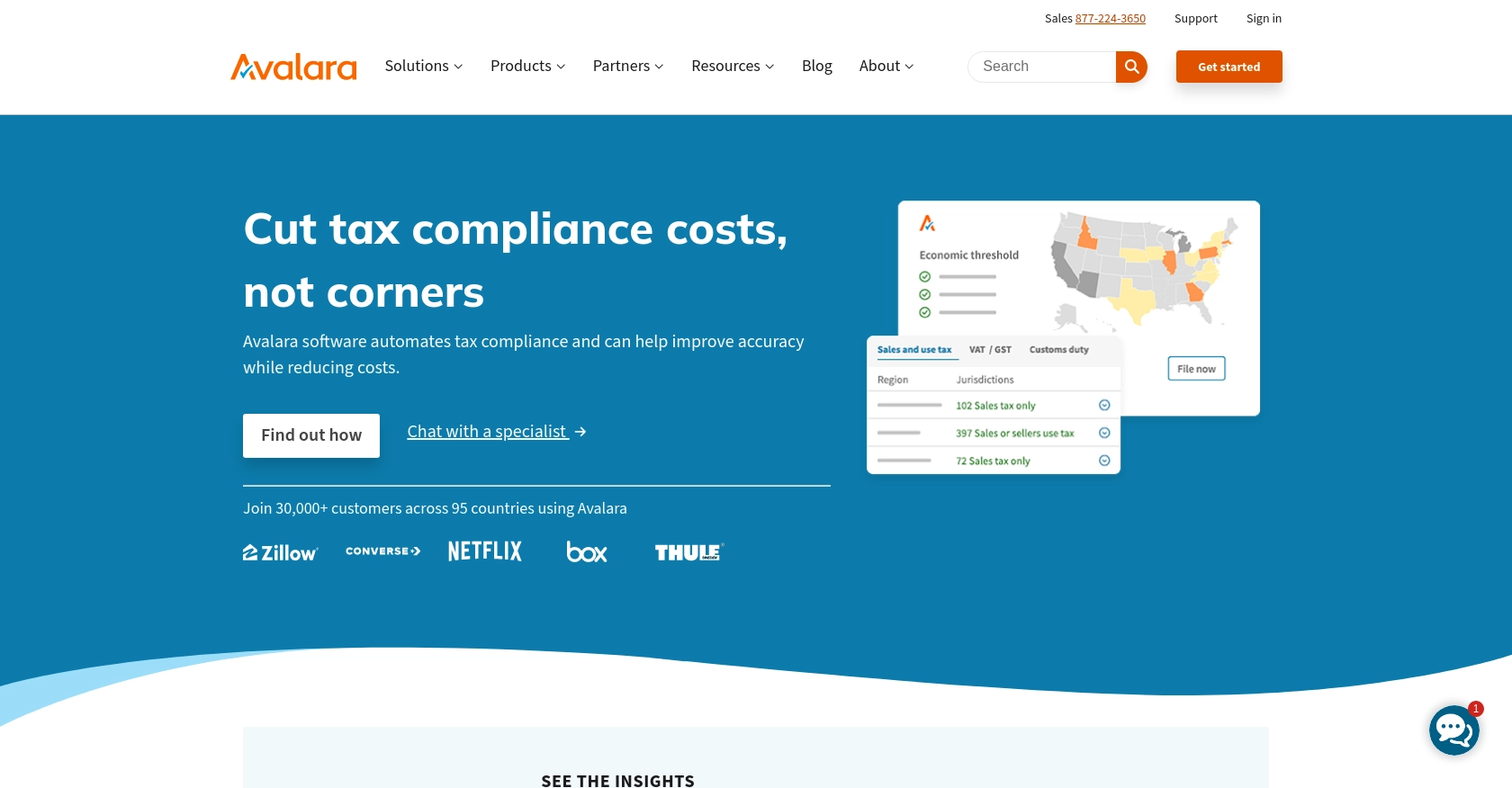
Introduction to Avalara API for Tax Calculation
Avalara is a leading provider of cloud-based tax compliance solutions, offering a comprehensive suite of tools to help businesses manage their tax obligations efficiently. With Avalara's AvaTax API, developers can automate the process of calculating taxes for various transactions, ensuring compliance with the latest tax regulations.
Integrating with Avalara's API can be particularly beneficial for businesses that need to handle complex tax calculations across multiple jurisdictions. For example, a developer might use the Avalara API to automatically calculate sales tax for an e-commerce platform, ensuring accurate tax rates are applied based on the customer's location.
This article will guide you through the process of using PHP to interact with the Avalara API, focusing on retrieving tax information for transactions. By following this tutorial, you'll learn how to set up authentication, make API calls, and handle responses effectively.
Setting Up Your Avalara Sandbox Account for API Integration
Before you can start using the Avalara API to calculate taxes, you'll need to set up a sandbox account. This allows you to test your integration without affecting live data. Avalara provides a robust sandbox environment that mimics the production environment, enabling developers to experiment and refine their API interactions.
Creating an Avalara Sandbox Account
To begin, you'll need to create a sandbox account on the Avalara developer portal. Follow these steps to get started:
- Visit the Avalara Developer Portal.
- Sign up for a new account or log in if you already have one.
- Navigate to the sandbox environment section and create a new sandbox account.
- Once your account is set up, you'll receive credentials including an account ID and a license key.
Configuring Authentication for Avalara API
The Avalara API uses Basic HTTP Authentication, which requires a combination of your account ID and license key. Here's how to configure it:
- Combine your account ID and license key in the format
accountid:licensekey
. - Encode this string using Base64 encoding.
- Use the encoded string as the authorization header in your API requests.
For example, if your account ID is 123456789
and your license key is ABCDEF123456789
, your authorization header will look like this:
Authorization: Basic MTIzNDU2Nzg5OkFCQ0RFRjEyMzQ1Njc4OQ==
Testing Your Avalara API Setup
With your sandbox account and authentication configured, you can now test your setup by making a simple API call. This will ensure that your credentials are working correctly and that you can interact with the Avalara API.
Refer to the Avalara API documentation for specific endpoints and parameters to use in your test calls. Make sure to verify the response to confirm successful authentication and data retrieval.
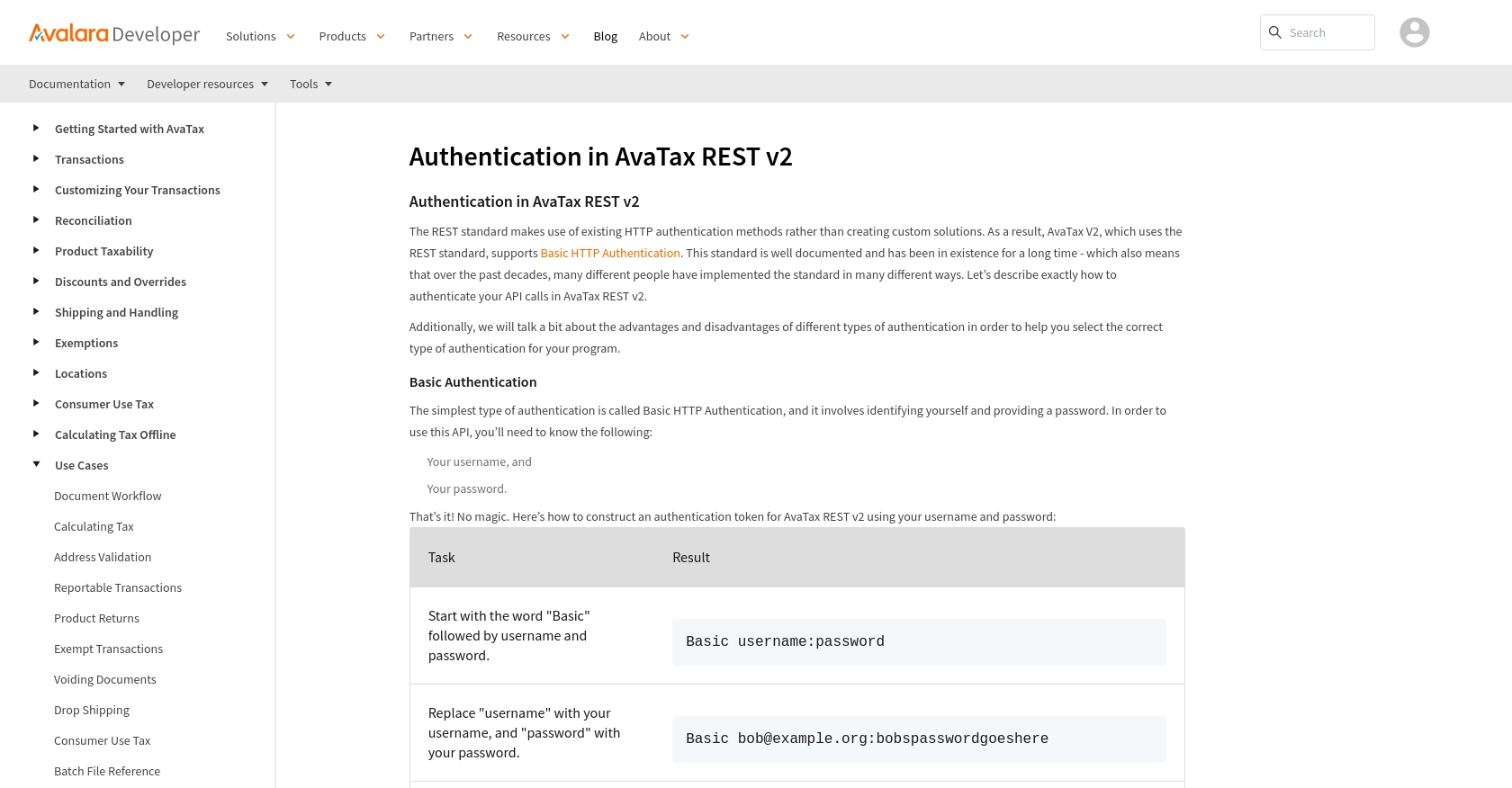
sbb-itb-96038d7
Making API Calls to Retrieve Tax Information with Avalara API in PHP
To interact with the Avalara API and retrieve tax information using PHP, you'll need to ensure you have the correct setup and dependencies. This section will guide you through the process of making API calls, handling responses, and verifying the results.
Setting Up Your PHP Environment for Avalara API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need PHP 7.4 or later and the cURL extension enabled, as it is essential for making HTTP requests.
- Verify your PHP version by running
php -v
in your terminal. - Ensure the cURL extension is enabled by checking your
php.ini
file or runningphp -m | grep curl
.
Installing Required PHP Libraries for Avalara API
To simplify the process of making HTTP requests, you can use the Guzzle HTTP client. Install it using Composer:
composer require guzzlehttp/guzzle
Example Code to Retrieve Tax Information Using Avalara API
Below is a sample PHP script to retrieve tax information using the Avalara API. Replace Your_Account_ID
and Your_License_Key
with your actual credentials.
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accountId = 'Your_Account_ID';
$licenseKey = 'Your_License_Key';
$auth = base64_encode("$accountId:$licenseKey");
$response = $client->request('GET', 'https://sandbox-rest.avatax.com/api/v2/taxrates/byaddress', [
'headers' => [
'Authorization' => "Basic $auth",
'Accept' => 'application/json',
],
'query' => [
'line1' => '2000 Main Street',
'city' => 'Irvine',
'region' => 'CA',
'postalCode' => '92614',
'country' => 'US'
]
]);
$data = json_decode($response->getBody(), true);
echo "Total Tax: " . $data['totalRate'];
This script sends a GET request to the Avalara API to retrieve tax rates based on an address. The response is parsed to display the total tax rate.
Verifying API Call Success and Handling Errors
After executing the script, verify the response to ensure the API call was successful. Check the HTTP status code and the response body:
- If the status code is 200, the request was successful.
- Inspect the response body to confirm the data is as expected.
Handle potential errors by checking for non-200 status codes and implementing error handling logic:
if ($response->getStatusCode() !== 200) {
echo "Error: " . $response->getReasonPhrase();
}
Refer to the Avalara API documentation for a comprehensive list of error codes and their meanings.
Conclusion and Best Practices for Using Avalara API in PHP
Integrating Avalara's API into your PHP application can significantly streamline tax calculations, ensuring compliance with various tax regulations. By following the steps outlined in this guide, you can effectively authenticate, make API calls, and handle responses to retrieve accurate tax information.
Best Practices for Storing Avalara API Credentials
- Store your account ID and license key securely, using environment variables or a secure vault, to prevent unauthorized access.
- Avoid hardcoding credentials directly in your codebase to enhance security.
Handling Avalara API Rate Limiting
While interacting with the Avalara API, be mindful of rate limits to avoid disruptions. Implement logic to handle rate limit responses and consider using exponential backoff strategies for retrying requests.
Transforming and Standardizing Tax Data
Ensure that the tax data retrieved from Avalara is transformed and standardized to fit your application's data model. This will facilitate seamless integration and data consistency across your systems.
Call to Action: Simplify Integrations with Endgrate
For developers looking to streamline multiple integrations, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate provides a unified API endpoint that simplifies the integration experience across various platforms, including Avalara.
Explore how Endgrate can enhance your integration capabilities by visiting Endgrate today.
Read More
Ready to get started?