Using the Google Sheets API to Create or Update Records in Javascript
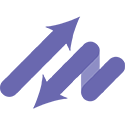
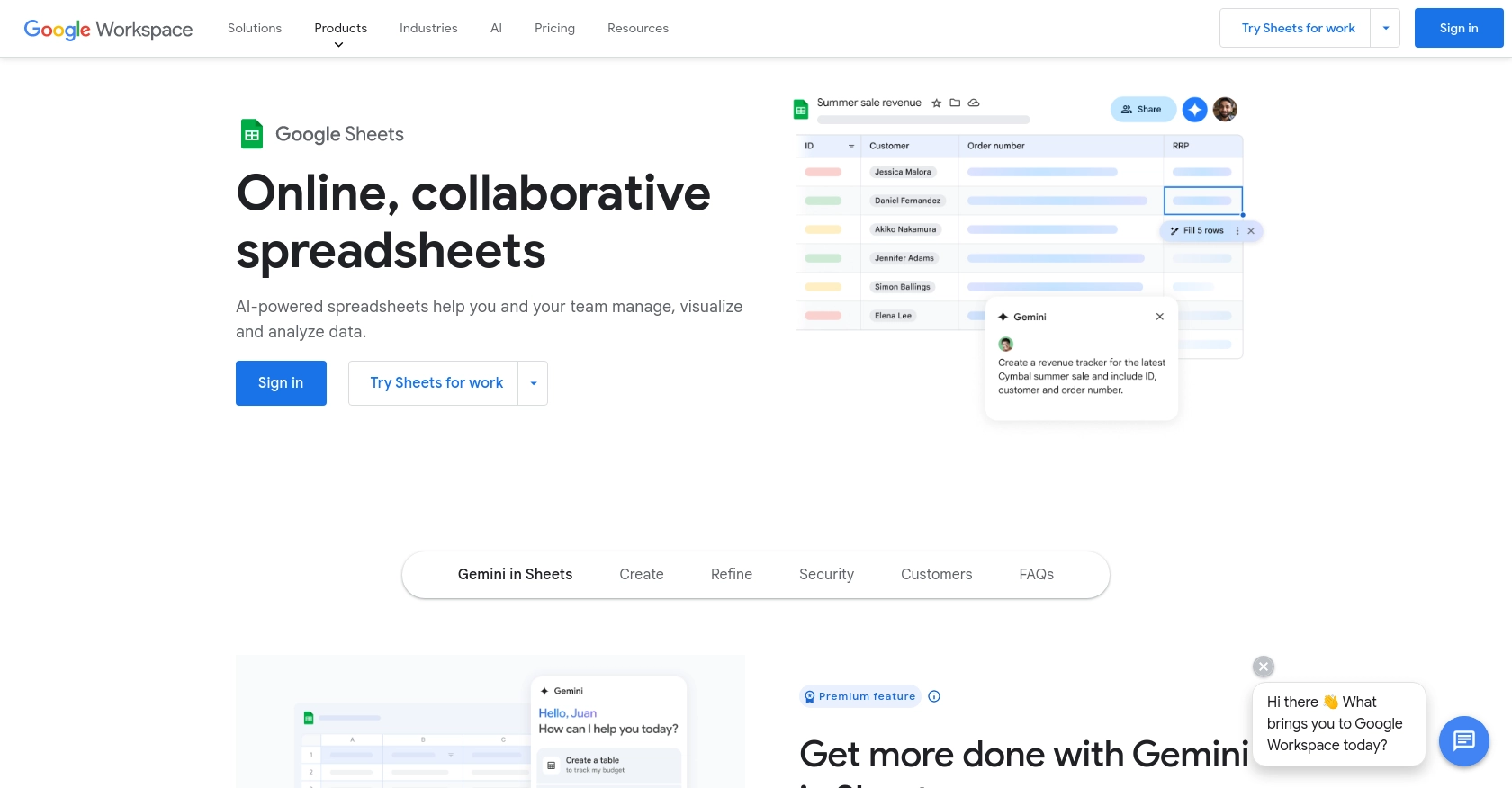
Introduction to Google Sheets API
Google Sheets is a powerful, cloud-based spreadsheet application that allows users to create, edit, and collaborate on spreadsheets in real-time. It's part of the Google Workspace suite and is widely used for data management, analysis, and reporting.
Integrating with the Google Sheets API enables developers to automate and streamline data operations, such as creating or updating records programmatically. For example, a developer might use the Google Sheets API to automatically update sales data from an external CRM system into a Google Sheet, ensuring that stakeholders always have access to the latest information.
Setting Up Your Google Sheets API Test Environment
Before you can start interacting with the Google Sheets API using JavaScript, you'll need to set up a Google Cloud project and configure the necessary credentials. This setup allows you to authenticate and authorize your requests securely.
Create a Google Cloud Project for Google Sheets API
- Go to the Google Cloud Console and sign in with your Google account.
- Navigate to Menu > IAM & Admin > Create a Project.
- Enter a descriptive name for your project and click Create.
Enable Google Sheets API in Your Project
- In the Google Cloud Console, go to Menu > APIs & Services > Library.
- Search for "Google Sheets API" and click on it.
- Click Enable to activate the API for your project.
Configure OAuth Consent Screen for Google Sheets API
- Go to APIs & Services > OAuth consent screen.
- Select your user type and click Create.
- Fill out the required fields such as App name and User support email, then click Save and Continue.
Create OAuth Credentials for Google Sheets API Access
- Navigate to APIs & Services > Credentials.
- Click Create Credentials and select OAuth client ID.
- Choose Web application as the application type.
- Enter a name for your OAuth client and add your authorized redirect URIs.
- Click Create to generate your Client ID and Client Secret. Save these credentials securely.
With your Google Cloud project and OAuth credentials set up, you're ready to start making requests to the Google Sheets API using JavaScript. For more detailed guidance, refer to the official documentation: Create a Google Cloud project, Enable Google Workspace APIs, Configure OAuth consent, and Create access credentials.
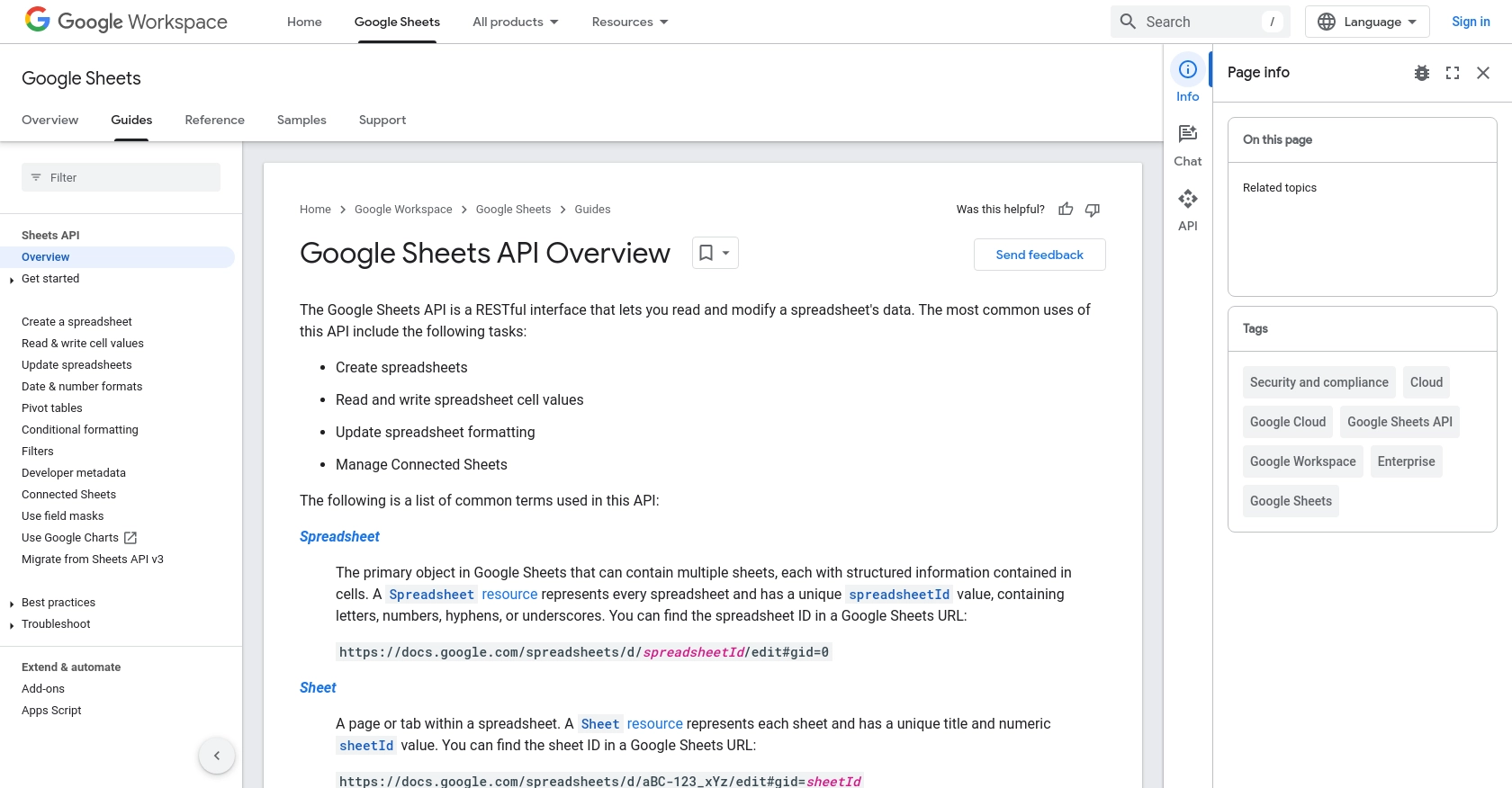
sbb-itb-96038d7
Making API Calls to Google Sheets Using JavaScript
To interact with the Google Sheets API using JavaScript, you need to set up your development environment and write code that can send requests to the API endpoints. This section will guide you through the process of creating or updating records in Google Sheets using JavaScript.
Setting Up Your JavaScript Environment for Google Sheets API
Before making API calls, ensure you have Node.js installed on your machine. You will also need the googleapis
package, which provides a client library for interacting with Google APIs.
- Install Node.js from the official website if you haven't already.
- Open your terminal and run the following command to install the
googleapis
package:
npm install googleapis
Authenticating with Google Sheets API Using OAuth 2.0
To authenticate your requests, use the OAuth 2.0 credentials you created earlier. This involves setting up the OAuth2 client and obtaining an access token.
const { google } = require('googleapis');
const sheets = google.sheets('v4');
const { OAuth2 } = google.auth;
const oauth2Client = new OAuth2(
'YOUR_CLIENT_ID',
'YOUR_CLIENT_SECRET',
'YOUR_REDIRECT_URI'
);
// Set credentials
oauth2Client.setCredentials({
refresh_token: 'YOUR_REFRESH_TOKEN'
});
// Obtain an access token
async function getAccessToken() {
const { token } = await oauth2Client.getAccessToken();
return token;
}
Creating or Updating Records in Google Sheets
Once authenticated, you can make API calls to create or update records in a Google Sheet. Here's an example of how to append data to a sheet:
async function appendDataToSheet() {
const auth = await getAccessToken();
const request = {
spreadsheetId: 'YOUR_SPREADSHEET_ID',
range: 'Sheet1!A1:D1',
valueInputOption: 'RAW',
insertDataOption: 'INSERT_ROWS',
resource: {
values: [
['Name', 'Email', 'Phone', 'Address']
],
},
auth: oauth2Client,
};
try {
const response = await sheets.spreadsheets.values.append(request);
console.log('Data appended successfully:', response.data);
} catch (error) {
console.error('Error appending data:', error);
}
}
appendDataToSheet();
Replace YOUR_SPREADSHEET_ID
, YOUR_CLIENT_ID
, YOUR_CLIENT_SECRET
, YOUR_REDIRECT_URI
, and YOUR_REFRESH_TOKEN
with your actual credentials and spreadsheet details.
Handling Errors and Verifying API Requests
It's crucial to handle errors gracefully and verify that your API requests succeed. Check the response status and data to ensure the operation was successful. If an error occurs, log the error message for debugging purposes.
For more detailed information on error codes and handling, refer to the Google Sheets API documentation.
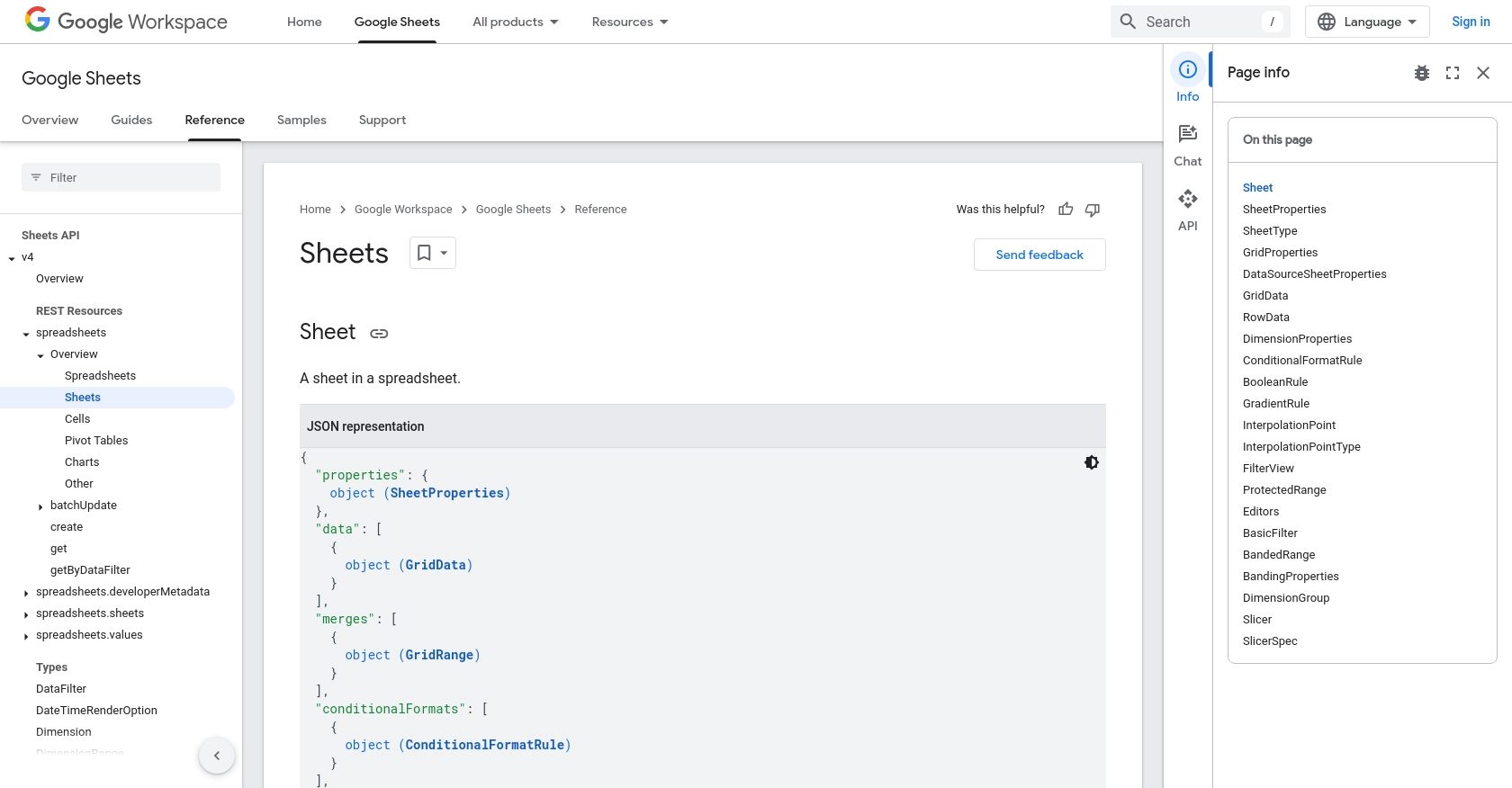
Conclusion and Best Practices for Using Google Sheets API in JavaScript
Integrating with the Google Sheets API using JavaScript offers a powerful way to automate data management tasks, ensuring that your spreadsheets are always up-to-date with the latest information. By following the steps outlined in this guide, you can efficiently create or update records in Google Sheets, enhancing productivity and collaboration within your team.
Best Practices for Secure and Efficient Google Sheets API Integration
- Securely Store Credentials: Always store your OAuth credentials securely. Avoid hardcoding sensitive information like client secrets and refresh tokens directly in your code.
- Handle Rate Limiting: Be mindful of the Google Sheets API rate limits. Implement exponential backoff strategies to handle rate limit errors gracefully. For more details, refer to the Google Sheets API documentation.
- Optimize Data Handling: When working with large datasets, consider using batch requests to minimize the number of API calls and improve performance.
- Data Standardization: Ensure that data formats are consistent across your applications and Google Sheets to avoid discrepancies and errors.
Enhance Your Integration Experience with Endgrate
While building integrations with the Google Sheets API can be rewarding, it can also be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Google Sheets. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can streamline your integration efforts and provide an intuitive experience for your customers. Visit Endgrate to learn more and start optimizing your integration workflows today.
Read More
- https://endgrate.com/provider/googlesheets
- https://developers.google.com/sheets/api/guides/concepts
- https://developers.google.com/sheets/api/reference/rest
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/sheets/api/reference/rest/v4/spreadsheets/sheets
- https://developers.google.com/sheets/api/reference/rest/v4/spreadsheets/cells
Ready to get started?