Using the NetHunt API to Get Records (with Python examples)
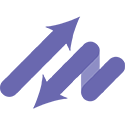
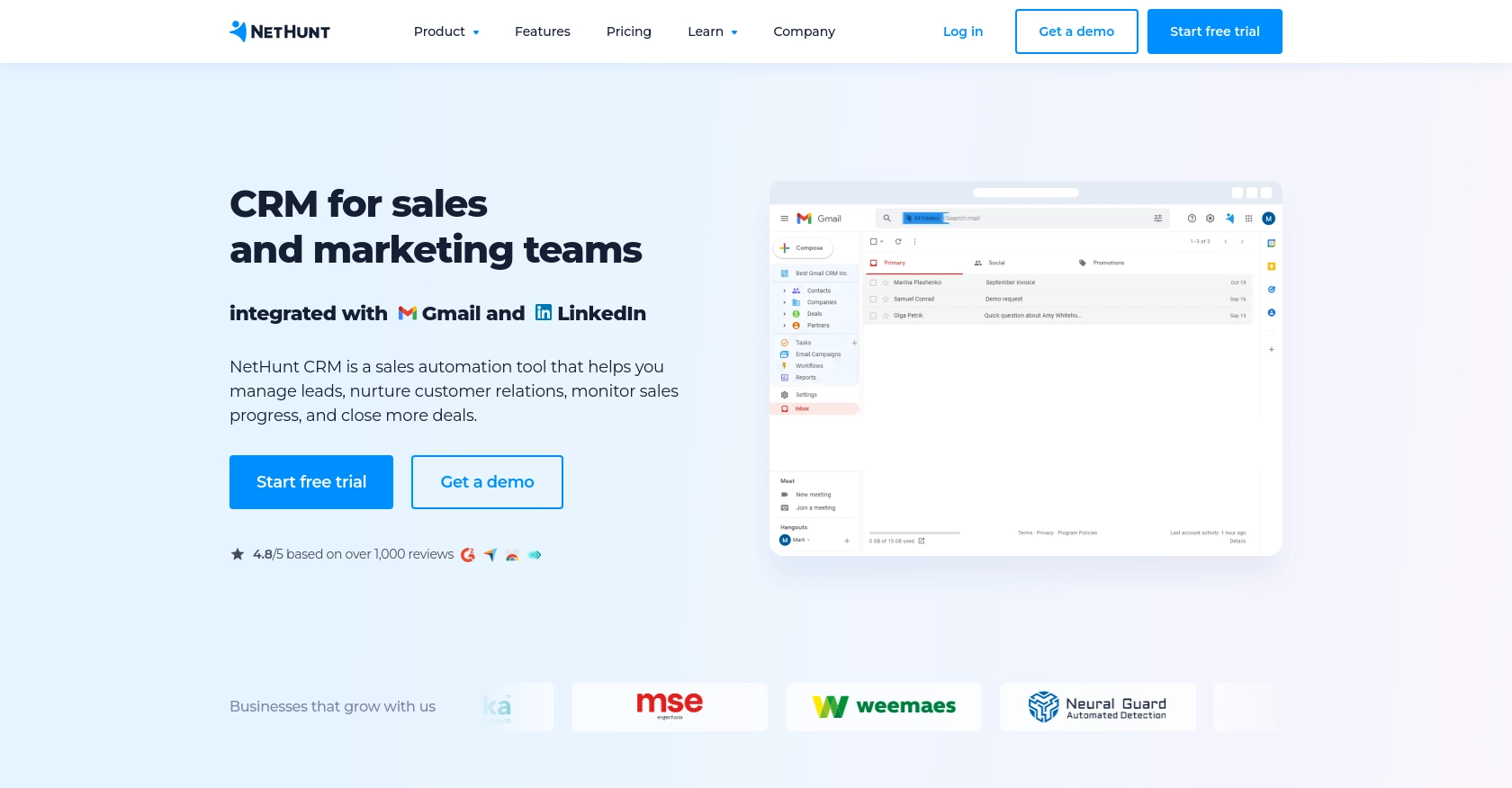
Introduction to NetHunt CRM
NetHunt CRM is a powerful customer relationship management tool that seamlessly integrates with Gmail, providing businesses with an efficient way to manage customer interactions and data. It offers a range of features including lead management, sales automation, and email campaigns, all within the familiar Gmail interface.
Developers might want to integrate with the NetHunt API to automate and enhance CRM processes. For example, using the API, a developer can retrieve records from NetHunt to analyze customer data or synchronize it with other business applications, streamlining workflows and improving data accuracy.
Setting Up Your NetHunt CRM Test Account
Before you can start integrating with the NetHunt API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting your live data.
Creating a NetHunt CRM Account
If you don't already have a NetHunt CRM account, you can sign up for a free trial on the NetHunt website. This trial will give you access to all the features you need to test the API integration.
- Visit the NetHunt website and click on "Start free trial."
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the NetHunt dashboard.
Generating Your NetHunt API Key
To interact with the NetHunt API, you'll need to generate an API key. This key will authenticate your requests and ensure secure communication with the NetHunt servers.
- Log in to your NetHunt account and navigate to the "Settings" section.
- Select "Integrations" from the menu.
- Find the option to generate an API key and click on it.
- Copy the generated API key and store it securely. You will need this key to authenticate your API requests.
For more details, refer to the NetHunt API key documentation.
Understanding NetHunt API Authentication
NetHunt uses Basic Authentication for its API. This involves combining your email and API key, encoding them in base64, and including them in the request headers.
import base64
# Replace with your email and API key
email = "your_email@example.com"
api_key = "your_api_key"
# Combine and encode credentials
credentials = f"{email}:{api_key}"
encoded_credentials = base64.b64encode(credentials.encode()).decode()
# Use the encoded credentials in the headers
headers = {
"Authorization": f"Basic {encoded_credentials}",
"Content-Type": "application/json"
}
Ensure that your API key is kept confidential and not exposed in your code repositories.
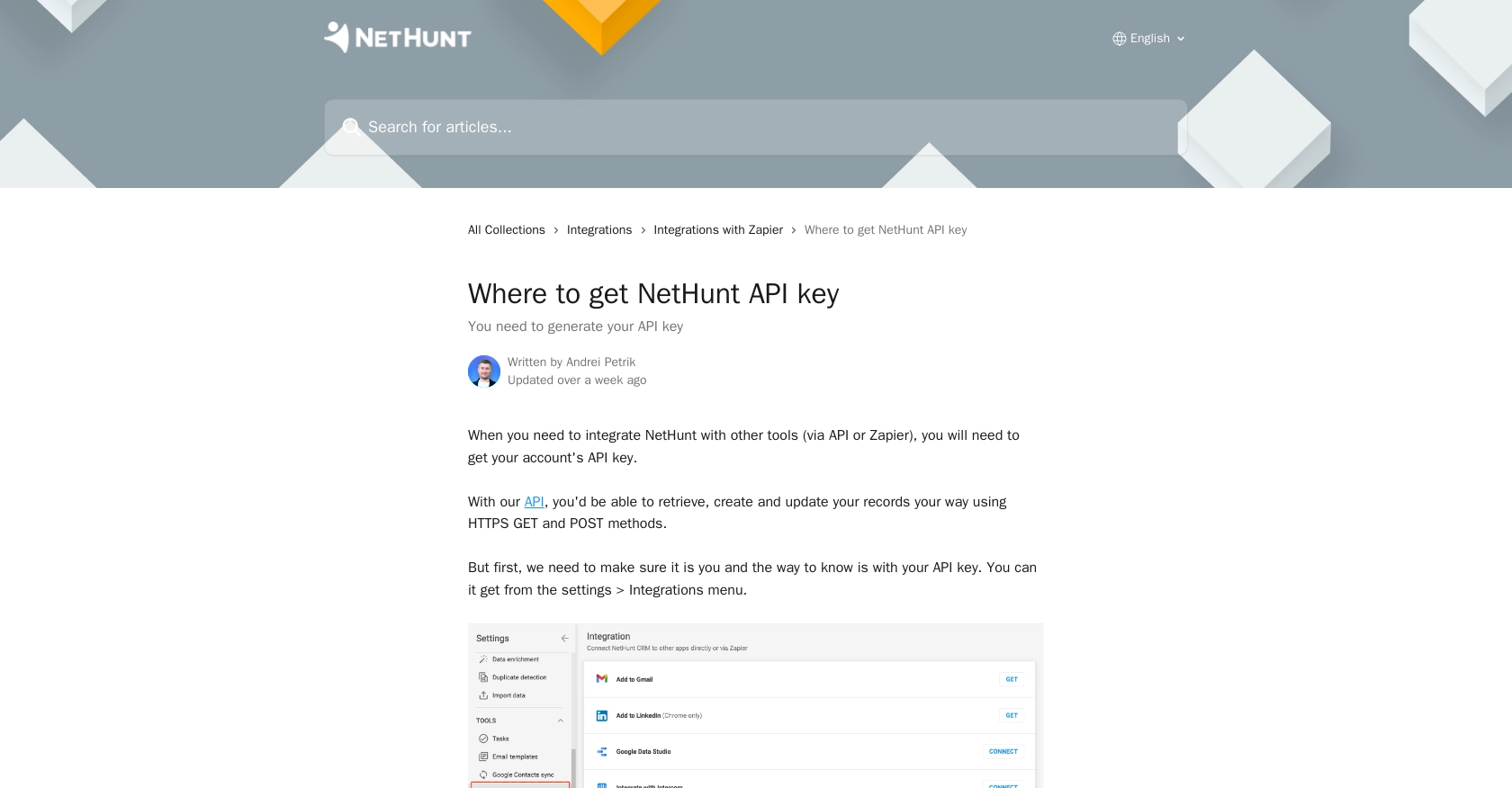
sbb-itb-96038d7
Making API Calls to Retrieve Records from NetHunt CRM Using Python
To interact with the NetHunt API and retrieve records, you'll need to use Python. This section will guide you through the process of making API calls to NetHunt CRM, ensuring you have the necessary setup and code to get started.
Setting Up Your Python Environment for NetHunt API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
# Install the requests library
pip install requests
Writing Python Code to Retrieve Records from NetHunt CRM
Once your environment is set up, you can write a Python script to retrieve records from NetHunt CRM. The following example demonstrates how to make a GET request to the NetHunt API to fetch records.
import requests
import base64
# Replace with your email and API key
email = "your_email@example.com"
api_key = "your_api_key"
# Combine and encode credentials
credentials = f"{email}:{api_key}"
encoded_credentials = base64.b64encode(credentials.encode()).decode()
# Set the API endpoint and headers
endpoint = "https://nethunt.com/api/v1/zapier/searches/find-record/your_folder_id"
headers = {
"Authorization": f"Basic {encoded_credentials}",
"Content-Type": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
for record in data:
print(record)
else:
print(f"Failed to retrieve records: {response.status_code}")
Replace your_folder_id
with the actual folder ID from which you want to retrieve records. This script will print the records if the request is successful.
Verifying API Call Success and Handling Errors
After running the script, you should verify the success of your API call by checking the returned data. If the request fails, the script will print an error message with the status code. Common error codes include:
- 401 Unauthorized: Check your API key and credentials.
- 404 Not Found: Verify the endpoint URL and folder ID.
- 500 Internal Server Error: Try the request again later.
For more information on error codes, refer to the NetHunt API documentation.
Conclusion and Best Practices for Using NetHunt API with Python
Integrating with the NetHunt API using Python can significantly enhance your CRM capabilities by automating data retrieval and synchronization processes. By following the steps outlined in this guide, you can efficiently access and manage your NetHunt records, ensuring seamless integration with other business applications.
Best Practices for Secure and Efficient NetHunt API Integration
- Secure Storage of API Credentials: Always store your API key securely, avoiding exposure in code repositories. Consider using environment variables or secure vaults for sensitive information.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the NetHunt API to avoid service disruptions. Implement retry logic with exponential backoff to manage rate limits effectively.
- Data Standardization: Ensure that data retrieved from NetHunt is standardized and transformed as needed to maintain consistency across integrated systems.
- Error Handling: Implement robust error handling to manage API call failures gracefully, providing informative feedback and retry mechanisms where appropriate.
Streamlining Integrations with Endgrate
While integrating with the NetHunt API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate offers a unified API solution that simplifies this process by providing a single endpoint to connect with various platforms, including NetHunt.
By leveraging Endgrate, you can save time and resources, allowing your development team to focus on core product features while ensuring a seamless integration experience for your customers. Explore how Endgrate can transform your integration strategy by visiting Endgrate.
Read More
Ready to get started?