Using the Microsoft Dynamics 365 Business Central API to Create or Update Customers in Python
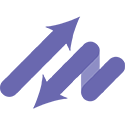
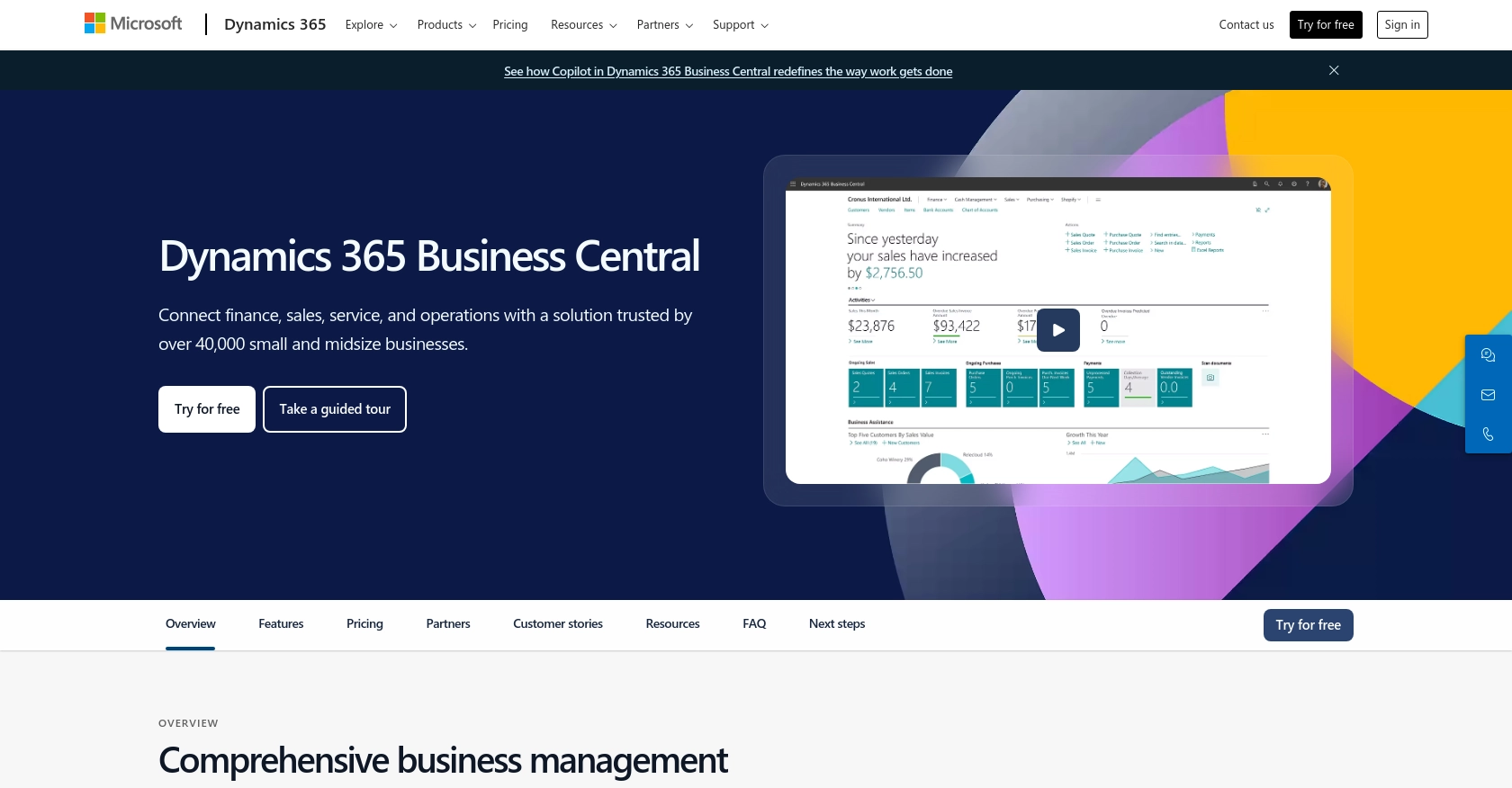
Introduction to Microsoft Dynamics 365 Business Central
Microsoft Dynamics 365 Business Central is a comprehensive business management solution designed for small to medium-sized businesses. It offers a wide range of functionalities, including finance, sales, service, and operations, all integrated into a single platform. This allows businesses to streamline their processes, improve customer interactions, and make informed decisions based on real-time data.
Integrating with the Microsoft Dynamics 365 Business Central API enables developers to automate and enhance business processes. For example, you can create or update customer records programmatically, ensuring that your customer data is always up-to-date and consistent across your systems. This can be particularly useful for businesses that need to synchronize customer information between different platforms or applications.
Setting Up Your Microsoft Dynamics 365 Business Central Sandbox Account
Before you can start integrating with the Microsoft Dynamics 365 Business Central API, you need to set up a sandbox account. This environment allows you to test and develop without affecting your live data, ensuring a safe space for experimentation and learning.
Creating a Microsoft Dynamics 365 Business Central Sandbox Account
To begin, you'll need to create a sandbox account. Follow these steps:
- Visit the Microsoft Dynamics 365 Business Central website and sign up for a free trial if you don't already have an account.
- Once logged in, navigate to the Admin Center.
- In the Admin Center, select Environments from the menu.
- Click on New to create a new environment.
- Select Sandbox as the type of environment and fill in the required details.
- Click Create to set up your sandbox environment.
Registering an Application for OAuth Authentication
Microsoft Dynamics 365 Business Central uses OAuth for authentication. You'll need to register an application in Microsoft Entra ID to obtain the necessary credentials:
- Go to the Azure Portal and sign in with your Microsoft account.
- Navigate to Azure Active Directory and select App registrations.
- Click on New registration and provide a name for your application.
- Set the Redirect URI to
https://localhost
for local development. - Click Register to create the application.
- Once registered, note down the Application (client) ID and Directory (tenant) ID.
- Navigate to Certificates & secrets and create a new client secret. Save this secret securely as it will not be shown again.
For more detailed instructions, refer to the Microsoft OAuth documentation.
Configuring API Permissions
To interact with the Microsoft Dynamics 365 Business Central API, you need to configure the appropriate permissions:
- In the Azure Portal, go to your registered app and select API permissions.
- Click on Add a permission and choose Dynamics 365 Business Central.
- Select the necessary permissions, such as user_impersonation, to allow your app to access the API on behalf of a user.
- Click Add permissions to apply the changes.
With your sandbox account and application registration complete, you're now ready to start integrating with the Microsoft Dynamics 365 Business Central API using Python.
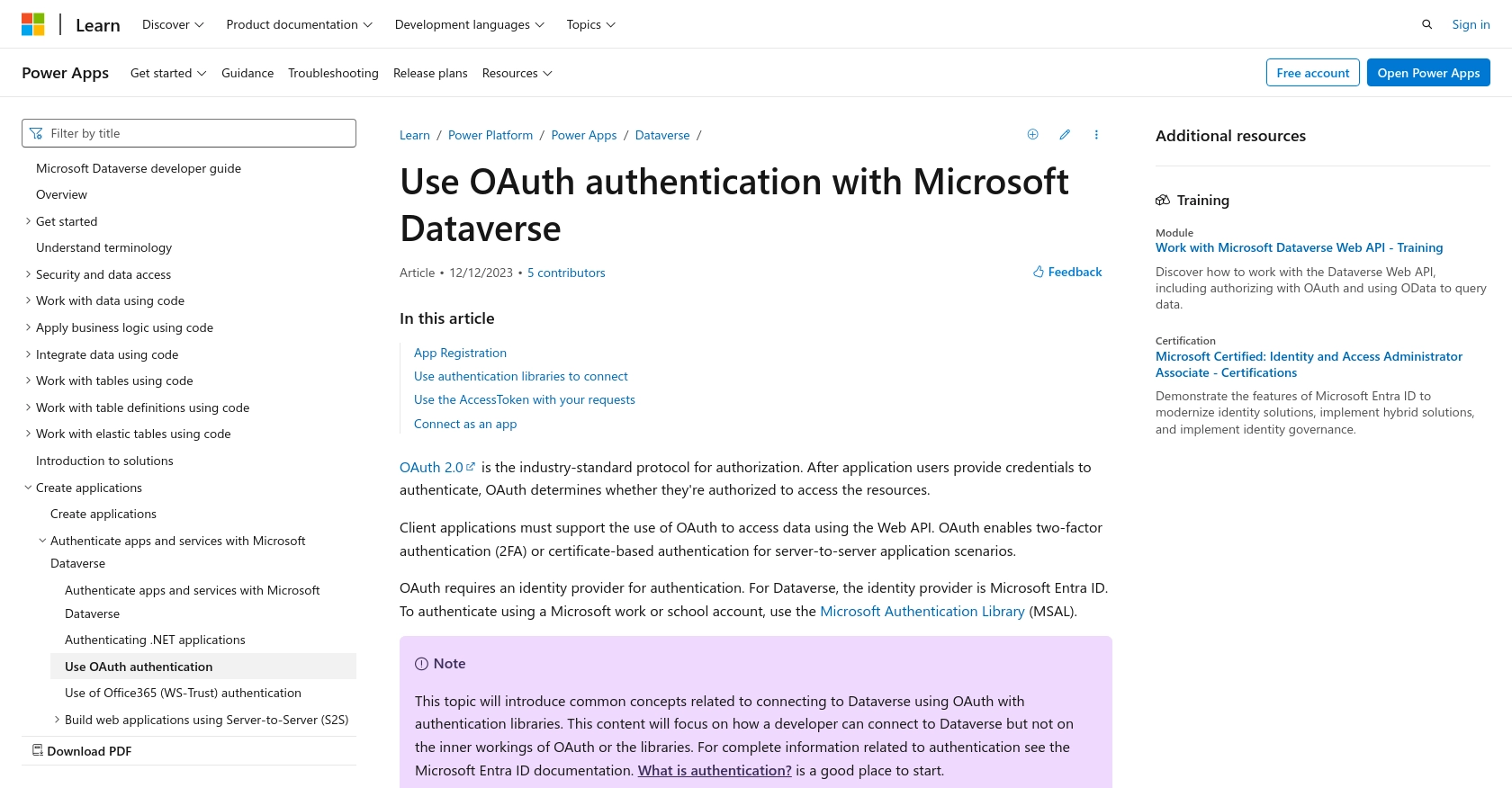
sbb-itb-96038d7
Making API Calls to Microsoft Dynamics 365 Business Central Using Python
To interact with the Microsoft Dynamics 365 Business Central API, you'll need to use Python to create or update customer records. This section will guide you through the process of setting up your Python environment, making API calls, and handling responses.
Setting Up Your Python Environment for Microsoft Dynamics 365 Business Central API
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer
pip
Once you have these installed, open your terminal or command prompt and install the requests
library using the following command:
pip install requests
Creating or Updating Customers with Microsoft Dynamics 365 Business Central API
To create or update customer records, you'll use the requests
library to send HTTP requests to the API. Follow these steps to make an API call:
Example Code to Create a Customer
Create a file named create_customer.py
and add the following code:
import requests
# Set the API endpoint and headers
url = "https://{businesscentralPrefix}/api/v2.0/companies({id})/customers"
headers = {
"Authorization": "Bearer Your_Token",
"Content-Type": "application/json"
}
# Define the customer data
customer_data = {
"displayName": "Adatum Corporation",
"type": "Company",
"addressLine1": "192 Market Square",
"city": "Atlanta",
"state": "GA",
"country": "US",
"postalCode": "31772",
"email": "robert.townes@contoso.com",
"currencyCode": "USD"
}
# Send the POST request
response = requests.post(url, json=customer_data, headers=headers)
# Check the response status
if response.status_code == 201:
print("Customer created successfully.")
else:
print(f"Failed to create customer: {response.status_code} - {response.text}")
Replace Your_Token
with the access token obtained during the OAuth authentication process. For more details, refer to the Microsoft Dynamics 365 Business Central API documentation.
Verifying the API Call Success
After running the script, verify the success of your API call by checking the response status code. A status code of 201
indicates that the customer was created successfully. You can also log into your Microsoft Dynamics 365 Business Central sandbox environment to confirm the new customer record.
Handling Errors and Common Error Codes
When making API calls, it's essential to handle potential errors. Common error codes include:
400
- Bad Request: The request was invalid or cannot be served.401
- Unauthorized: Authentication failed or user does not have permissions.404
- Not Found: The requested resource could not be found.500
- Internal Server Error: An error occurred on the server.
Implement error handling in your code to manage these scenarios effectively.
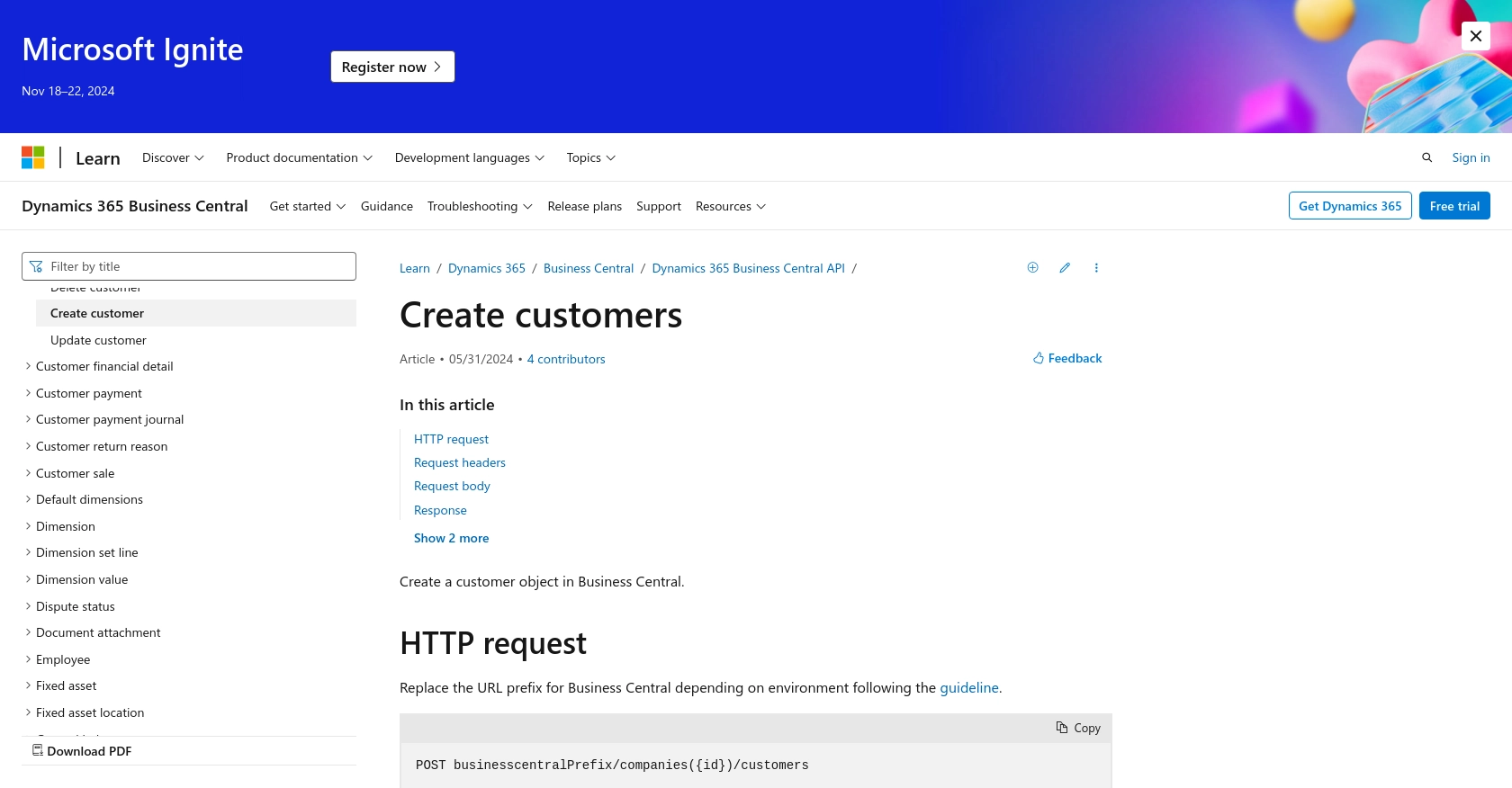
Conclusion and Best Practices for Using Microsoft Dynamics 365 Business Central API
Integrating with the Microsoft Dynamics 365 Business Central API allows developers to automate and streamline business processes, ensuring that customer data remains consistent and up-to-date across various platforms. By following the steps outlined in this guide, you can efficiently create or update customer records using Python.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth tokens and client secrets securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of API rate limits to avoid service disruptions. Implement retry logic with exponential backoff to handle rate limit errors gracefully. For more information on rate limits, refer to the official documentation.
- Implement Error Handling: Use comprehensive error handling to manage different HTTP status codes and ensure your application can recover from failures.
- Data Transformation and Standardization: Ensure that data being sent to and received from the API is properly transformed and standardized to match your application's requirements.
Enhance Your Integration Experience with Endgrate
For developers looking to streamline their integration processes further, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate provides a unified API endpoint that connects to multiple platforms, including Microsoft Dynamics 365 Business Central, offering an intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration needs by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/microsoftdynamics365businesscentral
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/authenticate-oauth
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/dynamics365/business-central/dev-itpro/api-reference/v2.0/api/dynamics_customer_create
Ready to get started?