Using the Younium API to Create or Update Accounts in Javascript
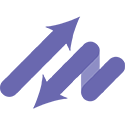
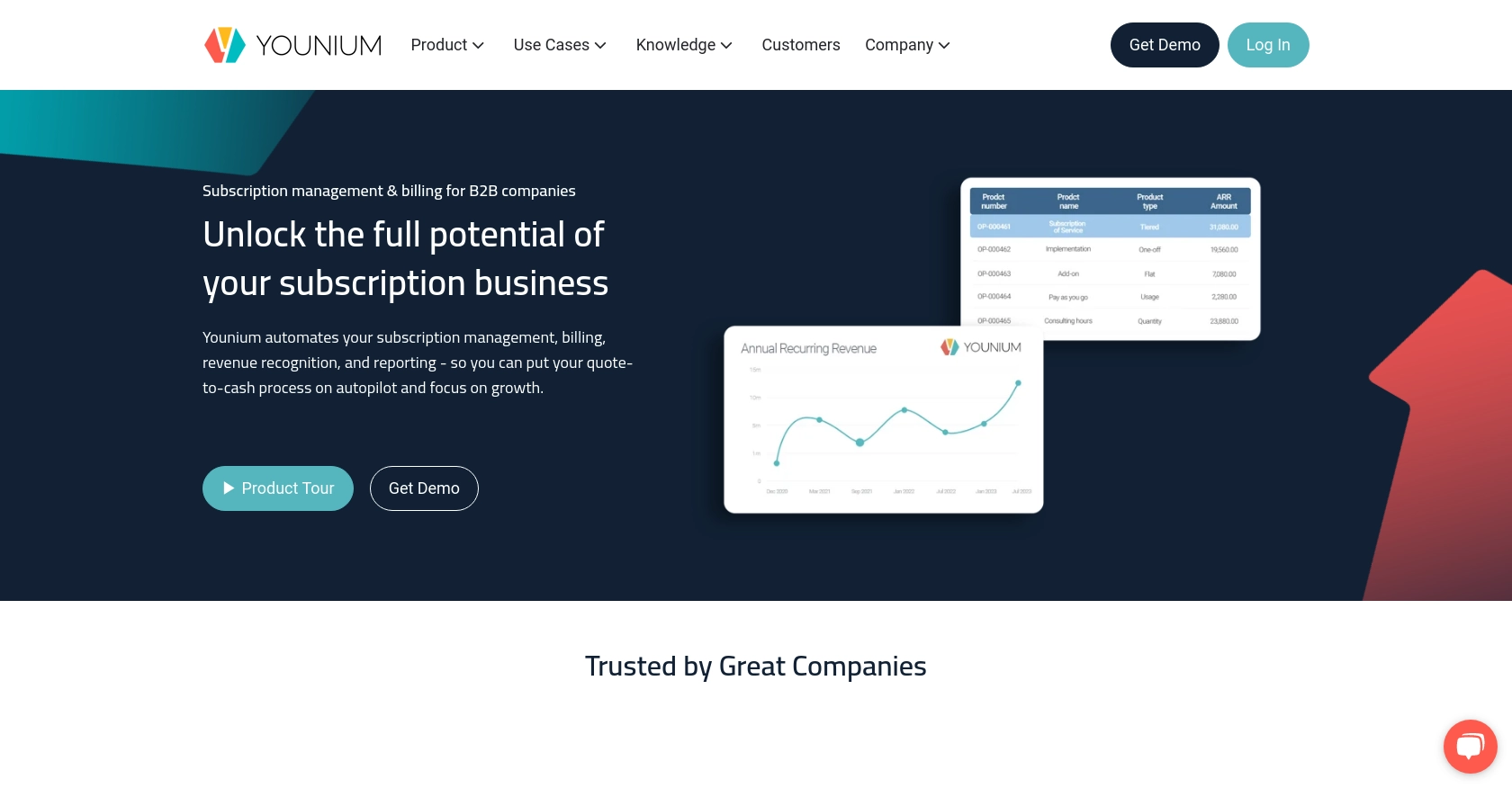
Introduction to Younium API Integration
Younium is a comprehensive subscription management platform designed to streamline billing, invoicing, and revenue recognition for B2B SaaS companies. It offers robust tools to manage complex subscription models, automate financial operations, and gain insights into revenue streams.
Integrating with Younium's API allows developers to efficiently manage account data, automate billing processes, and synchronize customer information across platforms. For example, a developer might use the Younium API to create or update customer accounts directly from a CRM system, ensuring that all customer data is consistent and up-to-date.
Setting Up Your Younium Sandbox Account for API Integration
Before you can start integrating with the Younium API, it's essential to set up a sandbox account. This allows you to test API interactions without affecting live data. Younium provides a sandbox environment where developers can experiment with API calls safely.
Creating a Younium Sandbox Account
To begin, you'll need to create a sandbox account on the Younium platform. Follow these steps:
- Visit the Younium Developer Portal and sign up for a developer account.
- Once registered, navigate to the sandbox environment section and create a new sandbox account.
- Fill in the necessary details and submit the form to generate your sandbox account.
Generating API Tokens for Younium Authentication
Younium uses JWT access tokens for API authentication. Follow these steps to generate the necessary tokens:
- Log in to your sandbox account and click on your name in the top right corner to open the user profile menu.
- Select “Privacy & Security” and then “Personal Tokens” from the left panel.
- Click “Generate Token” and provide a relevant description for your token.
- Click “Create” to generate your Client ID and Secret Key. Make sure to copy these credentials as they will not be visible again.
Acquiring a JWT Access Token
With your Client ID and Secret Key, you can now acquire a JWT access token:
// Example POST request to acquire JWT token
const fetch = require('node-fetch');
const getToken = async () => {
const response = await fetch('https://api.sandbox.younium.com/auth/token', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
clientId: 'Your_Client_ID',
secret: 'Your_Secret_Key'
})
});
const data = await response.json();
console.log('Access Token:', data.accessToken);
};
getToken();
Replace Your_Client_ID
and Your_Secret_Key
with the credentials you generated. This script will return an access token valid for 24 hours.
Handling Authentication Errors
While acquiring the JWT token, you may encounter errors:
- 400 or 401 Error: Indicates invalid credentials. Double-check your Client ID and Secret Key.
- 403 Forbidden: Ensure the legal entity specified is correct and that your user has the necessary permissions.
For more details, refer to the Younium Authentication Documentation.
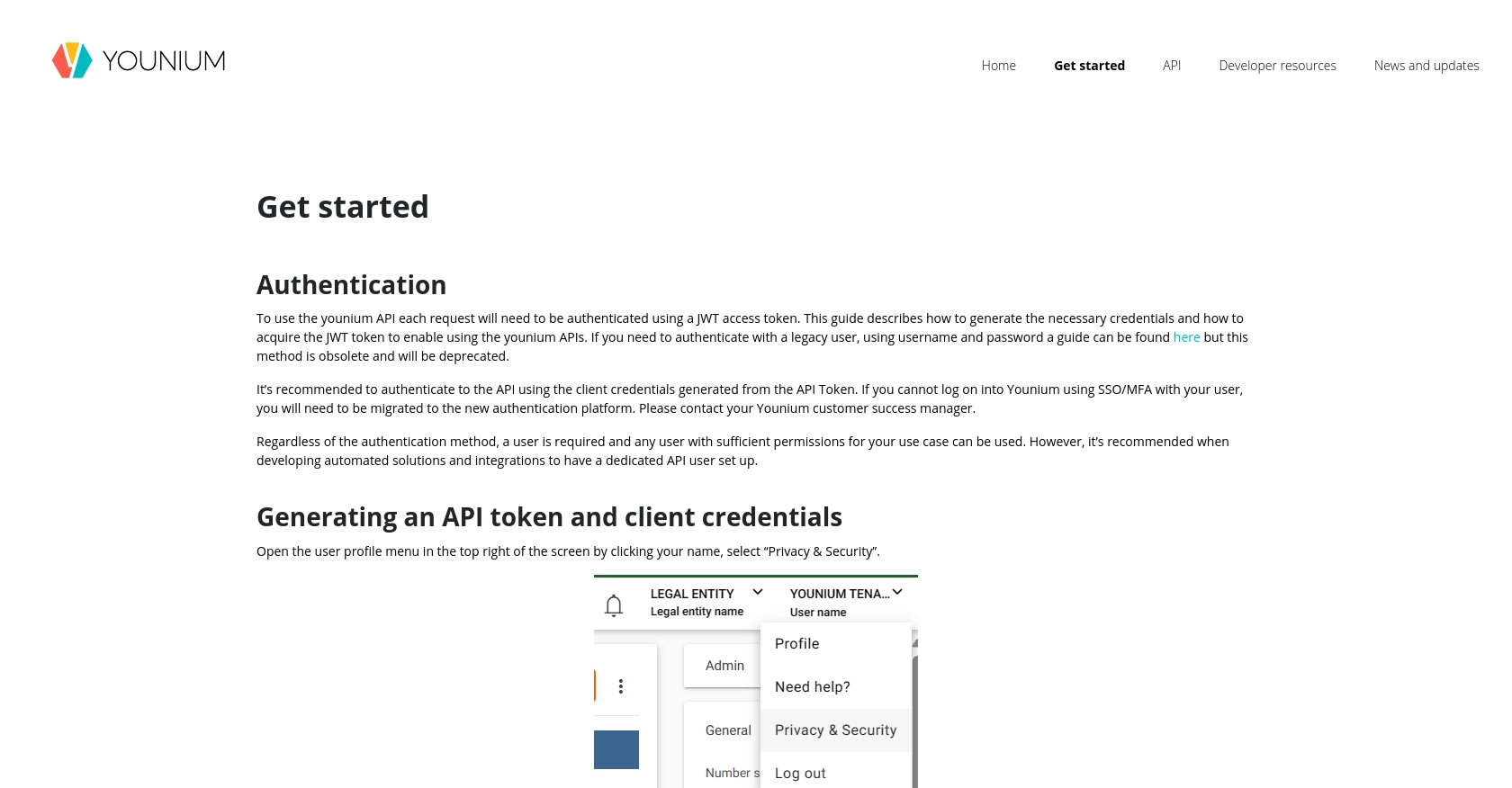
sbb-itb-96038d7
Making API Calls to Create or Update Accounts with Younium in JavaScript
Once you have your JWT access token, you can start making API calls to Younium to create or update accounts. This section will guide you through the process using JavaScript, ensuring you have the right setup and code to interact with Younium's API effectively.
Setting Up Your JavaScript Environment for Younium API Integration
Before making API calls, ensure your development environment is ready. You will need:
- Node.js installed on your machine.
- The
node-fetch
library to make HTTP requests. Install it using the command:
npm install node-fetch
Creating or Updating Accounts Using Younium API
To create or update accounts, you'll need to make a POST request to the Younium API endpoint. Here's a step-by-step guide:
// Import the fetch library
const fetch = require('node-fetch');
// Function to create or update an account
const createOrUpdateAccount = async (accessToken, accountData) => {
const response = await fetch('https://api.sandbox.younium.com/accounts', {
method: 'POST',
headers: {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json',
'api-version': '2.1', // Optional but recommended
'legal-entity': 'Your_Legal_Entity' // Replace with your legal entity
},
body: JSON.stringify(accountData)
});
if (response.ok) {
const data = await response.json();
console.log('Account Created/Updated:', data);
} else {
console.error('Error:', response.status, response.statusText);
}
};
// Example account data
const accountData = {
name: 'New Account',
email: 'newaccount@example.com',
// Add other necessary fields
};
// Replace 'Your_Access_Token' with the JWT token you acquired
createOrUpdateAccount('Your_Access_Token', accountData);
In this code, replace Your_Access_Token
and Your_Legal_Entity
with your actual JWT token and legal entity. The accountData
object should contain the necessary fields for the account you wish to create or update.
Verifying Successful API Requests in Younium Sandbox
After running the script, verify the account creation or update by checking your Younium sandbox account. The changes should be reflected in the account data.
Handling Errors and Troubleshooting Younium API Calls
When making API calls, you might encounter errors. Here are some common issues and how to resolve them:
- 401 Unauthorized: Check if your access token is expired or incorrect.
- 403 Forbidden: Ensure the legal entity is correct and your user has the necessary permissions.
- Other Errors: Review the response status and message for more details.
For further troubleshooting, refer to the Younium API Documentation.
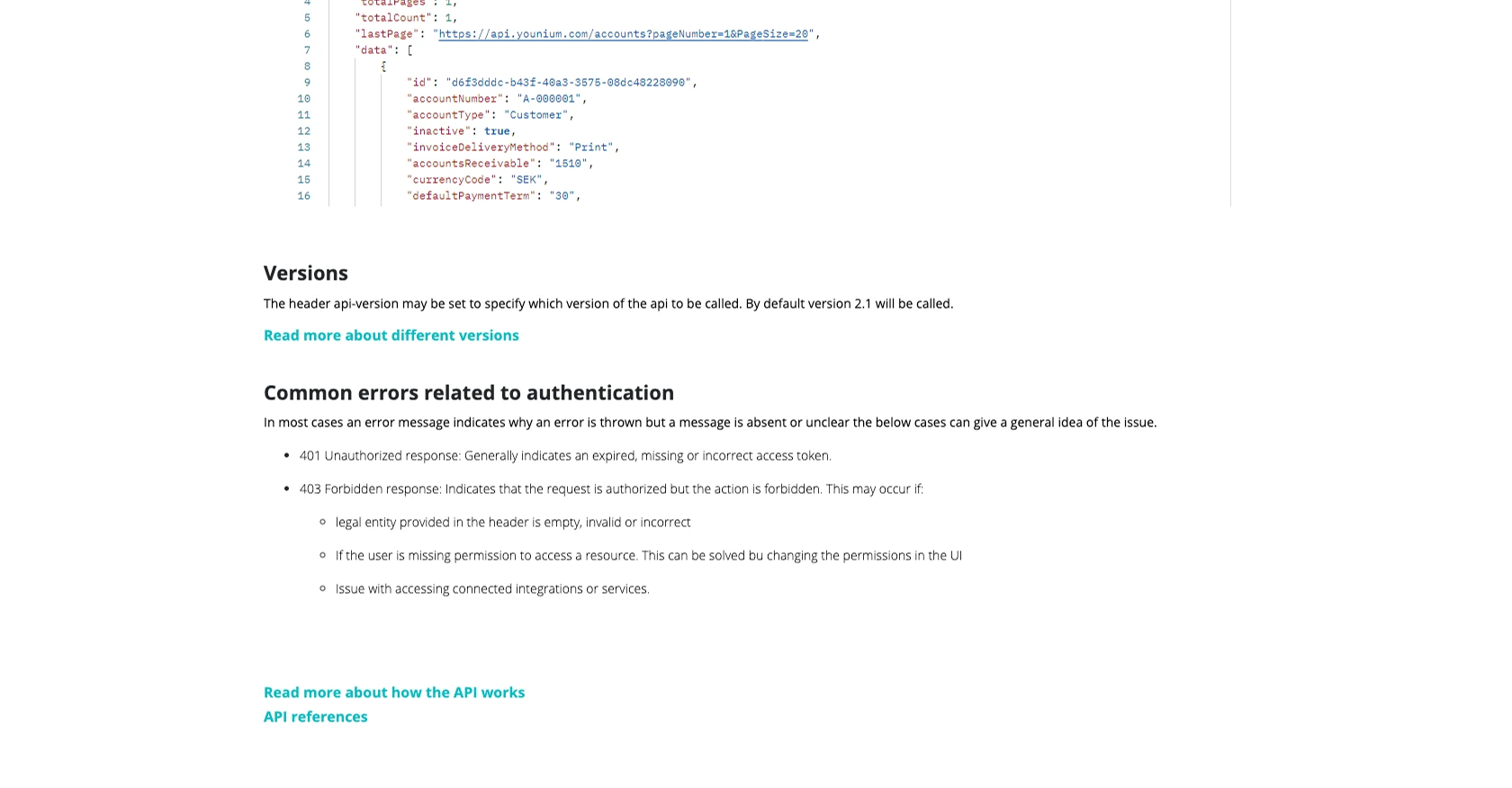
Conclusion and Best Practices for Younium API Integration
Integrating with the Younium API using JavaScript allows developers to efficiently manage account data, automate billing processes, and ensure data consistency across platforms. By following the steps outlined in this guide, you can create or update accounts seamlessly within the Younium ecosystem.
Best Practices for Secure and Efficient Younium API Usage
- Secure Storage of Credentials: Always store your Client ID, Secret Key, and JWT tokens securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handling Rate Limits: Be mindful of Younium's API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data being sent to Younium is standardized and validated to prevent errors during API calls.
- Regular Token Refresh: Since JWT tokens are valid for 24 hours, implement a mechanism to refresh tokens automatically to maintain uninterrupted API access.
Enhancing Integration Capabilities with Endgrate
While integrating with Younium's API can be straightforward, managing multiple integrations can become complex. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including Younium. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate and discover the benefits of a unified integration experience.
Read More
Ready to get started?