Using the Quickbooks API to Create Or Update Vendors in PHP
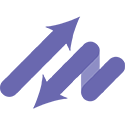
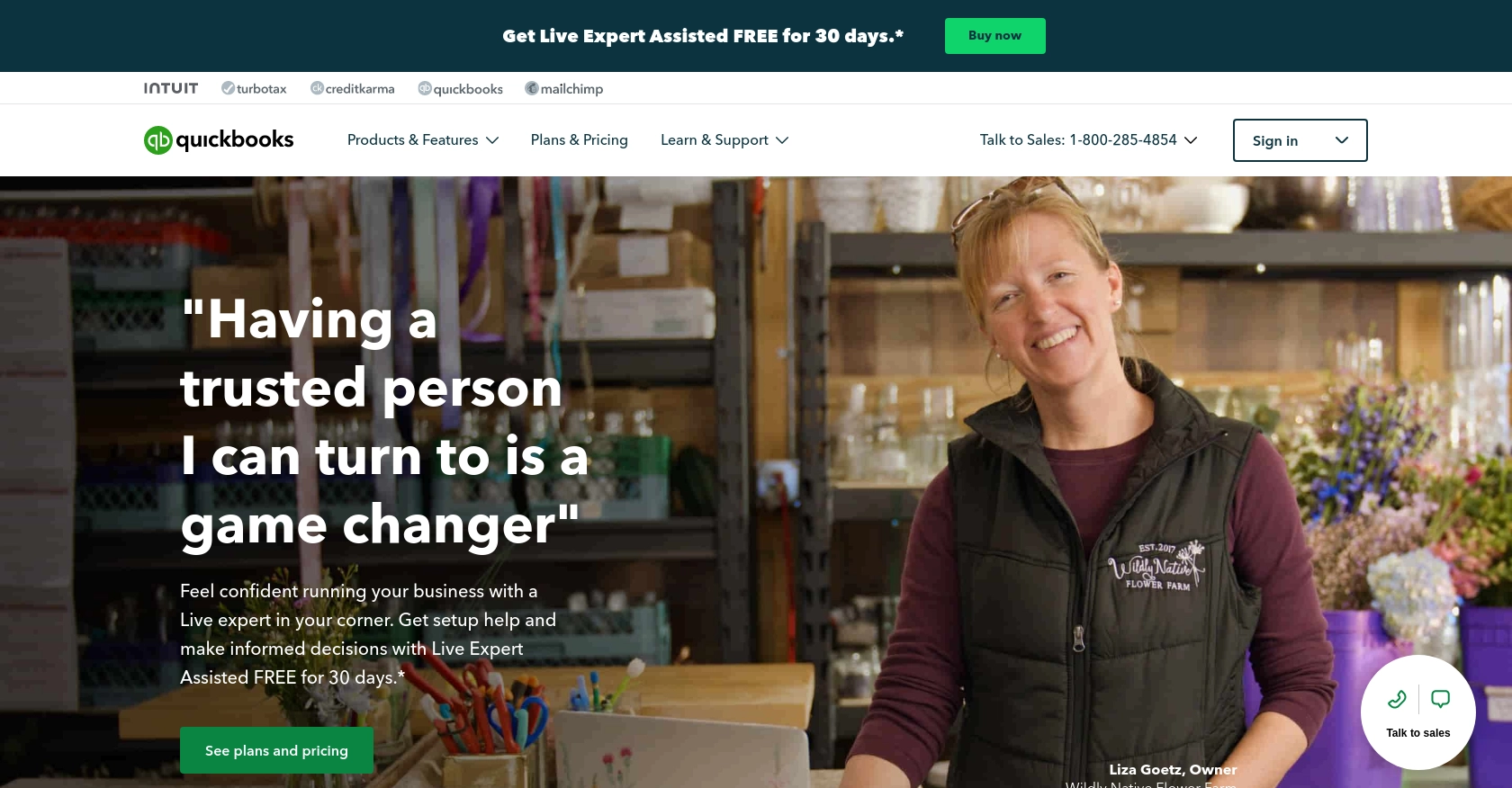
Introduction to QuickBooks API for Vendor Management
QuickBooks is a renowned accounting software that offers a comprehensive suite of tools for managing finances, invoicing, payroll, and more. It is widely used by businesses of all sizes to streamline their financial operations and maintain accurate records.
For developers, integrating with the QuickBooks API can significantly enhance the efficiency of financial management tasks. By connecting with QuickBooks, developers can automate processes such as creating or updating vendor information, which is crucial for maintaining up-to-date records and ensuring smooth business operations.
In this article, we will explore how to use the QuickBooks API to create or update vendors using PHP. This integration can be particularly useful for businesses that need to manage a large number of vendors and want to automate the process to save time and reduce errors.
Setting Up Your QuickBooks Sandbox Account for API Integration
Before you can start integrating with the QuickBooks API, you'll need to set up a sandbox account. This allows you to test your application in a safe environment without affecting real data. QuickBooks provides a sandbox environment that mimics the live environment, enabling developers to experiment and refine their integrations.
Creating a QuickBooks Developer Account
To begin, you'll need to create a QuickBooks Developer account. Follow these steps:
- Visit the QuickBooks Developer Portal.
- Click on "Sign Up" to create a new account or "Sign In" if you already have one.
- Fill in the required information and complete the registration process.
Setting Up a QuickBooks Sandbox Company
Once your developer account is ready, you can set up a sandbox company:
- Log in to your QuickBooks Developer account.
- Navigate to the "Sandbox" section in the dashboard.
- Click on "Create Sandbox" to generate a new sandbox company.
- Follow the prompts to configure your sandbox environment.
Creating a QuickBooks App for OAuth Authentication
QuickBooks uses OAuth 2.0 for authentication. You'll need to create an app to obtain the necessary credentials:
- Go to the App Management section in your developer account.
- Click on "Create an App" and select "QuickBooks Online and Payments" as the platform.
- Fill in the app details, including the name and description.
- Once the app is created, navigate to the "Keys & OAuth" section to find your Client ID and Client Secret.
- Save these credentials securely, as they will be used for authenticating API requests.
Configuring OAuth Redirect URIs
Ensure that your app's OAuth settings are correctly configured:
- In the app settings, locate the "Redirect URIs" section.
- Add the URI where users will be redirected after authentication. This is typically a URL on your server that handles OAuth responses.
- Save the changes to update your app's settings.
With your sandbox account and app set up, you're now ready to start making API calls to QuickBooks. In the next section, we'll explore how to create or update vendors using PHP.
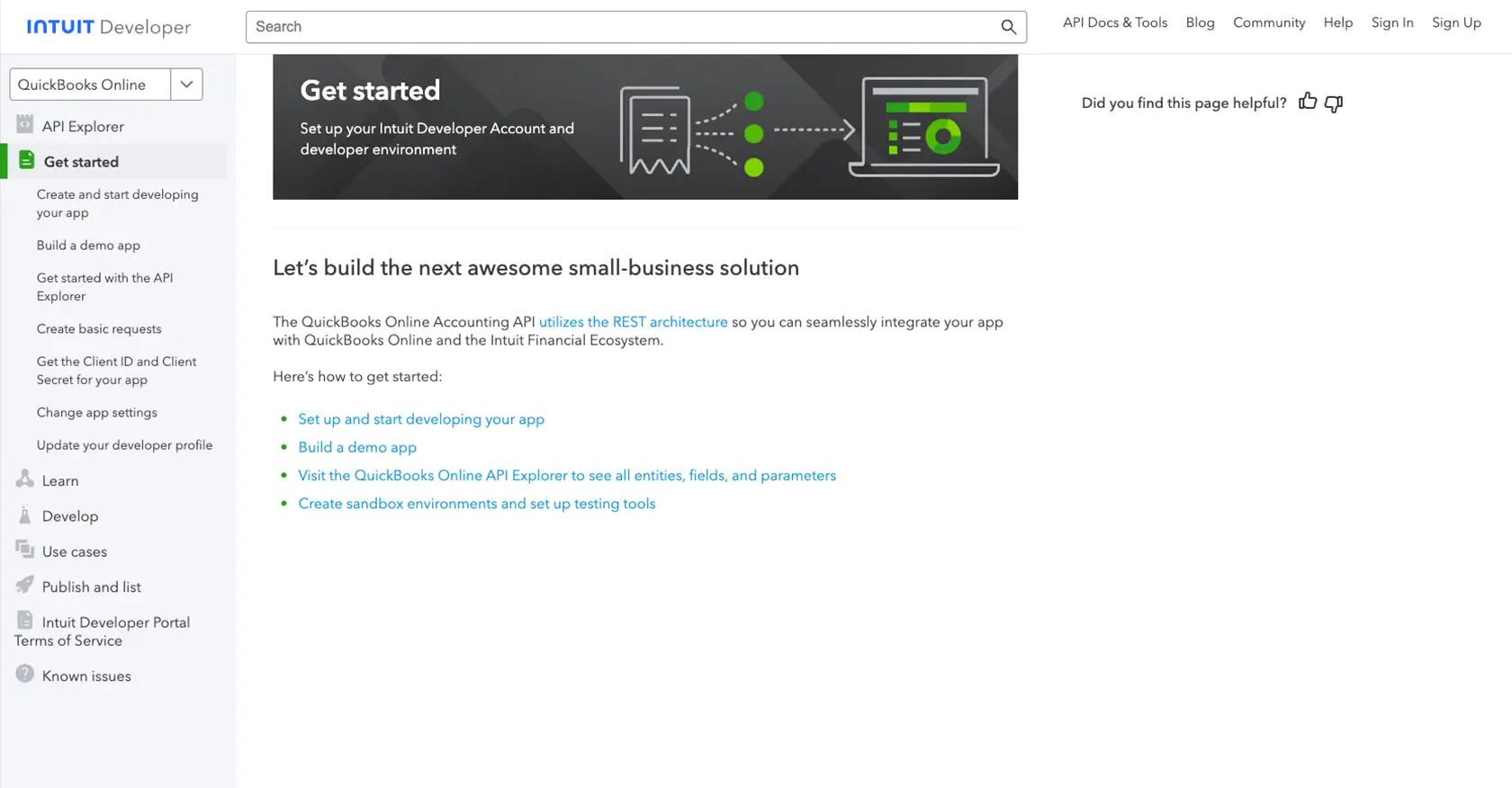
sbb-itb-96038d7
Making API Calls to QuickBooks for Vendor Management Using PHP
With your QuickBooks sandbox account and app configured, you can now proceed to make API calls to create or update vendors using PHP. This section will guide you through the process, including setting up your PHP environment, writing the code, and handling responses.
Setting Up Your PHP Environment for QuickBooks API Integration
Before making API calls, ensure your PHP environment is correctly set up. You'll need the following:
- PHP 7.4 or higher
- Composer for dependency management
- The
guzzlehttp/guzzle
library for making HTTP requests
Install the Guzzle library using Composer with the following command:
composer require guzzlehttp/guzzle
Creating or Updating Vendors with QuickBooks API Using PHP
Now, let's write the PHP code to interact with the QuickBooks API. This example demonstrates how to create or update a vendor.
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
// Set your QuickBooks API endpoint
$endpoint = 'https://sandbox-quickbooks.api.intuit.com/v3/company/YOUR_COMPANY_ID/vendor';
// Set your OAuth 2.0 access token
$accessToken = 'YOUR_ACCESS_TOKEN';
// Define the vendor data
$vendorData = [
'DisplayName' => 'New Vendor',
'PrimaryPhone' => [
'FreeFormNumber' => '(555) 555-5555'
],
'PrimaryEmailAddr' => [
'Address' => 'vendor@example.com'
]
];
try {
// Make the API request
$response = $client->request('POST', $endpoint, [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json',
'Accept' => 'application/json'
],
'json' => $vendorData
]);
// Parse the response
$responseData = json_decode($response->getBody(), true);
echo 'Vendor created/updated successfully: ' . $responseData['Vendor']['Id'];
} catch (\Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace YOUR_COMPANY_ID
and YOUR_ACCESS_TOKEN
with your actual QuickBooks company ID and OAuth 2.0 access token. The code above uses the Guzzle HTTP client to send a POST request to the QuickBooks API, creating or updating a vendor with the specified data.
Verifying API Call Success in QuickBooks Sandbox
After executing the code, verify the success of your API call by checking the QuickBooks sandbox environment. Navigate to the vendor section to confirm that the vendor has been created or updated as expected.
Handling Errors and QuickBooks API Error Codes
When making API calls, it's essential to handle potential errors gracefully. QuickBooks API may return various error codes, such as:
- 400 - Bad Request: The request was invalid or cannot be served.
- 401 - Unauthorized: Authentication failed or user does not have permissions.
- 404 - Not Found: The requested resource could not be found.
- 500 - Internal Server Error: An error occurred on the server.
Ensure your application checks for these error codes and implements appropriate error handling to provide informative feedback to users.
For more detailed information on error codes, refer to the QuickBooks API documentation.
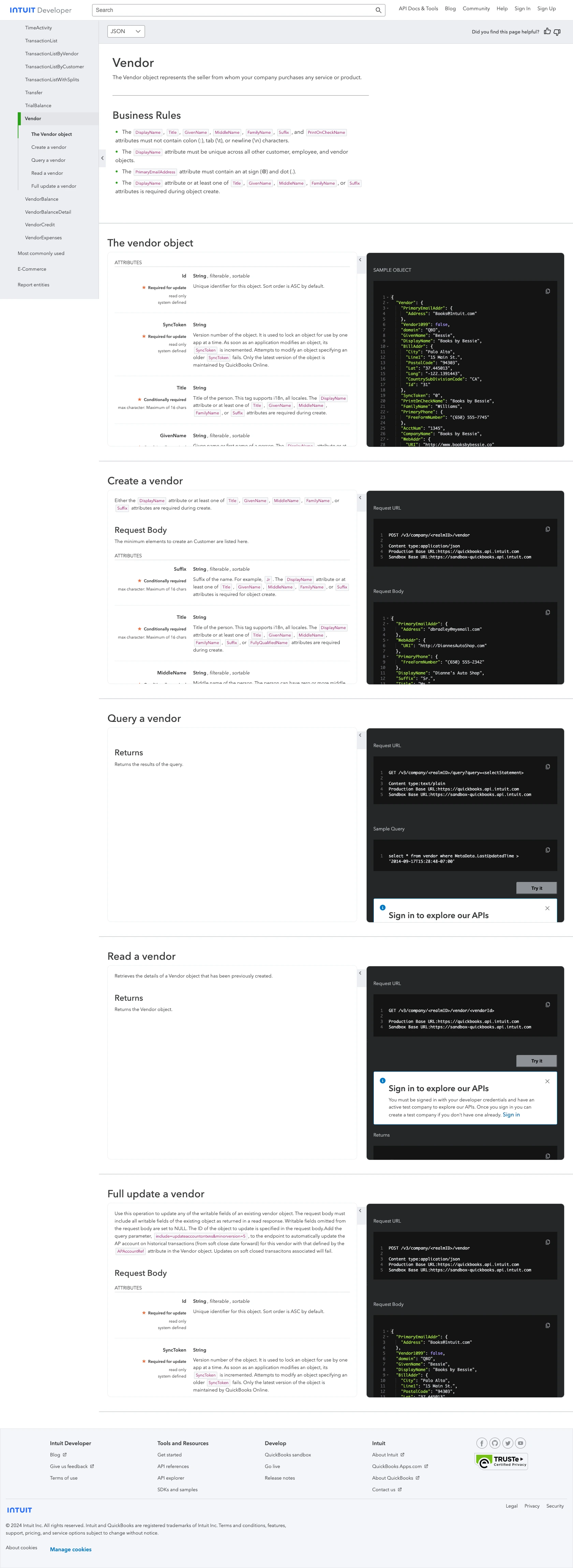
Conclusion and Best Practices for QuickBooks API Integration
Integrating with the QuickBooks API to manage vendor information using PHP can greatly enhance the efficiency of your financial operations. By automating the creation and updating of vendor records, businesses can save time, reduce errors, and ensure that their financial data is always current.
Best Practices for Secure and Efficient QuickBooks API Usage
- Secure Storage of Credentials: Always store your OAuth credentials, such as Client ID and Client Secret, securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: QuickBooks API imposes rate limits to ensure fair usage. Be sure to implement logic to handle rate limit responses and retry requests as needed. For specific rate limit details, refer to the QuickBooks API documentation.
- Data Validation and Standardization: Validate and standardize data before sending it to the API to prevent errors and ensure consistency across your systems.
- Error Handling: Implement comprehensive error handling to manage API responses effectively. Provide informative feedback to users when errors occur.
Streamlining Integration with Endgrate
If managing multiple integrations is becoming a challenge, consider using Endgrate to simplify the process. Endgrate allows you to build once for each use case and connect to multiple platforms through a single API endpoint. This can save you time and resources, allowing you to focus on your core product and deliver an intuitive integration experience for your customers.
Explore how Endgrate can help you streamline your integration efforts by visiting Endgrate.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/all-entities/vendor
Ready to get started?