Using the Quickbooks API to Get Products (with PHP examples)
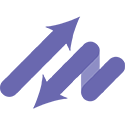
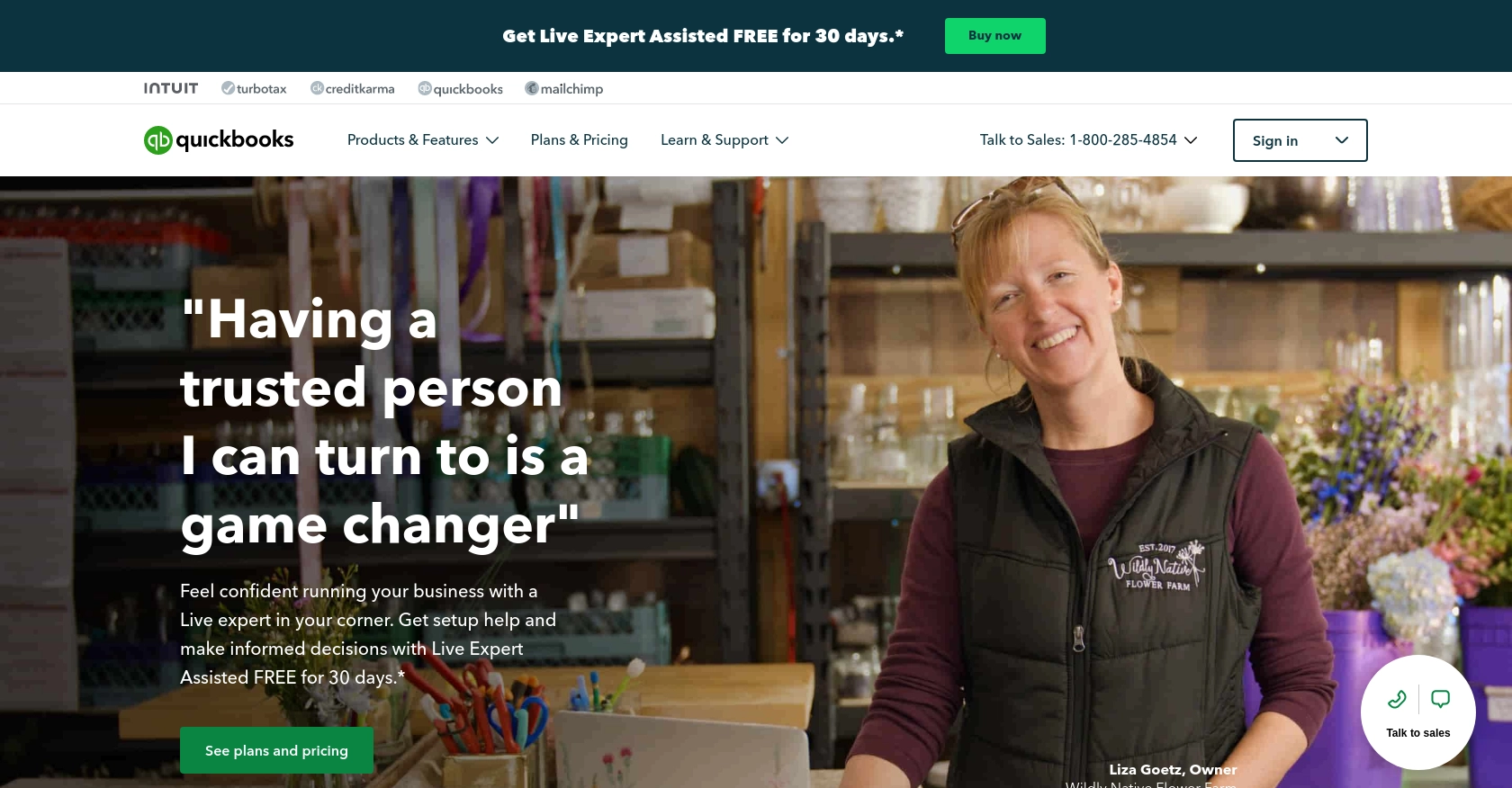
Introduction to QuickBooks API Integration
QuickBooks is a widely-used accounting software that offers a comprehensive suite of tools for managing financial operations. It is particularly popular among small to medium-sized businesses due to its user-friendly interface and robust feature set, which includes invoicing, expense tracking, payroll, and financial reporting.
Integrating with QuickBooks' API allows developers to automate and streamline financial processes, such as retrieving product information. For example, a developer might use the QuickBooks API to fetch product details and synchronize them with an e-commerce platform, ensuring that inventory levels and pricing are always up-to-date.
Setting Up Your QuickBooks Sandbox Account for API Integration
Before you can start interacting with the QuickBooks API, you'll need to set up a sandbox account. This allows you to test API calls without affecting live data, providing a safe environment to develop and debug your integration.
Creating a QuickBooks Developer Account
To begin, you'll need a QuickBooks Developer account. If you don't have one, follow these steps:
- Visit the Intuit Developer Portal.
- Click on "Sign Up" and fill in the required details to create your account.
- Once registered, log in to your developer account.
Creating a QuickBooks Sandbox Company
After setting up your developer account, you can create a sandbox company:
- Navigate to the "Dashboard" on the Intuit Developer Portal.
- Select "Sandbox" from the menu and click on "Add Sandbox Company."
- Follow the prompts to create a new sandbox company, which will be used for testing your API calls.
Generating OAuth Credentials for QuickBooks API
QuickBooks API uses OAuth 2.0 for authentication. Here's how to generate the necessary credentials:
- In your developer account, go to the "My Apps" section and click on "Create an App."
- Select "QuickBooks Online and Payments" as the platform.
- Fill in the required app details and click "Create App."
- Once the app is created, navigate to the "Keys & OAuth" tab.
- Here, you'll find your Client ID and Client Secret. These are essential for authenticating your API requests.
For more detailed instructions, refer to the Intuit Developer Documentation.
Configuring App Settings for API Access
To ensure your app has the correct permissions, configure the app settings:
- In the "My Apps" section, select your app and go to the "App Settings" tab.
- Ensure that the necessary scopes are selected for accessing product data.
- Save your settings to apply the changes.
For further guidance, consult the Intuit Developer Documentation.
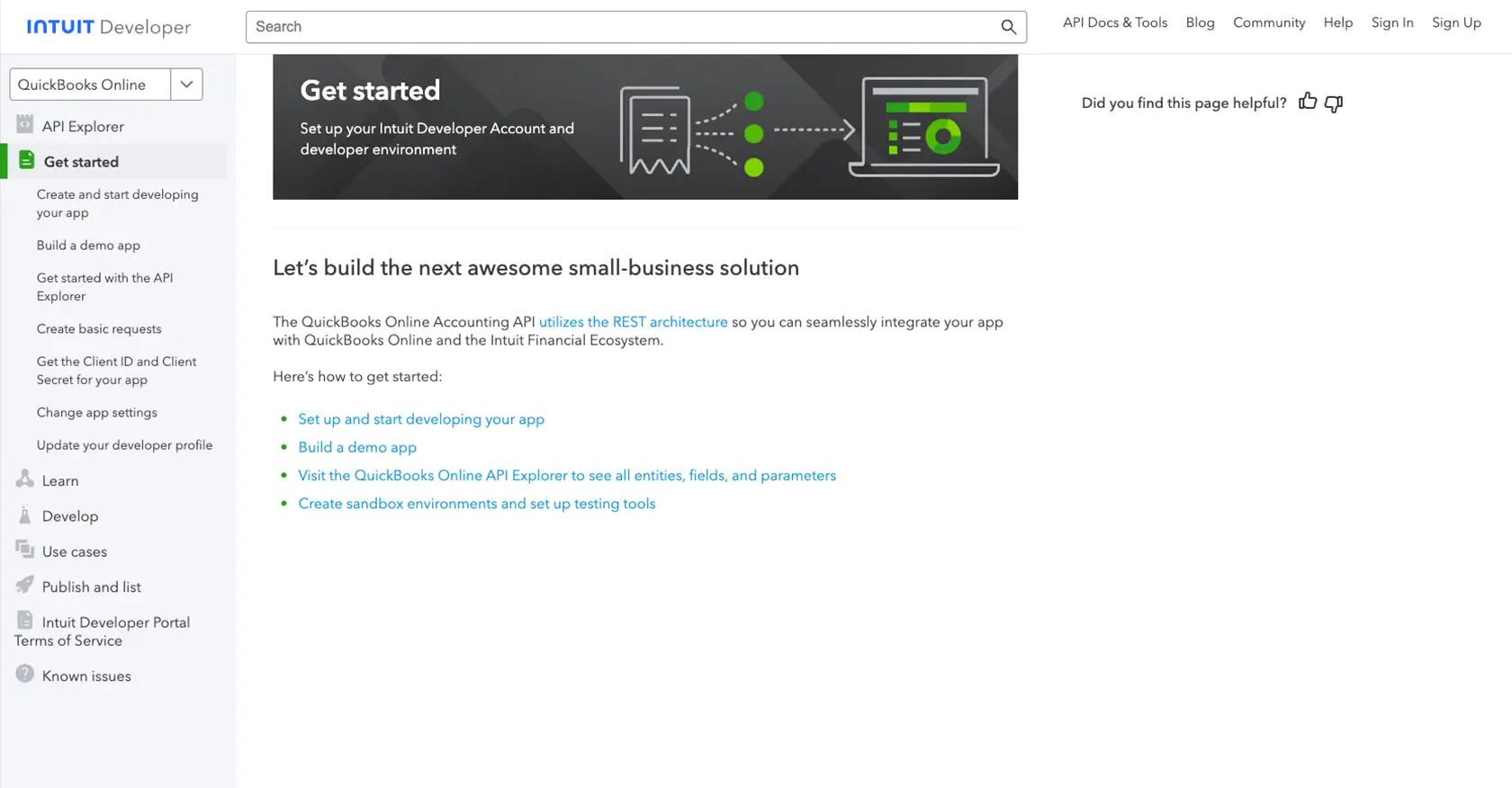
sbb-itb-96038d7
Making API Calls to Retrieve Products from QuickBooks Using PHP
To interact with the QuickBooks API and retrieve product information, you'll need to make HTTP requests using PHP. This section will guide you through the process, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your PHP Environment for QuickBooks API Integration
Before you begin coding, ensure that your development environment is ready:
- Install PHP 7.4 or later on your machine.
- Ensure you have Composer installed for managing dependencies.
- Install the
guzzlehttp/guzzle
library, which simplifies making HTTP requests. Run the following command in your terminal:
composer require guzzlehttp/guzzle
Writing PHP Code to Fetch Products from QuickBooks API
Now, let's write the PHP code to make an API call to QuickBooks and retrieve product data:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token';
$realmId = 'Your_Realm_ID';
$response = $client->request('GET', "https://quickbooks.api.intuit.com/v3/company/$realmId/item", [
'headers' => [
'Authorization' => "Bearer $accessToken",
'Accept' => 'application/json',
]
]);
if ($response->getStatusCode() === 200) {
$products = json_decode($response->getBody(), true);
foreach ($products['QueryResponse']['Item'] as $product) {
echo 'Product Name: ' . $product['Name'] . '<br>';
}
} else {
echo 'Failed to retrieve products. Status code: ' . $response->getStatusCode();
}
Replace Your_Access_Token
and Your_Realm_ID
with your actual OAuth access token and QuickBooks company ID.
Verifying API Call Success and Handling Errors
After running the code, verify that the request succeeded by checking the output. You should see a list of product names retrieved from your QuickBooks sandbox account.
If the request fails, the code will display an error message with the HTTP status code. Common error codes include:
- 401 Unauthorized: Check your access token and ensure it hasn't expired.
- 403 Forbidden: Verify that your app has the necessary permissions.
- 404 Not Found: Ensure the endpoint URL and realm ID are correct.
For more detailed error handling, refer to the Intuit Developer Documentation.
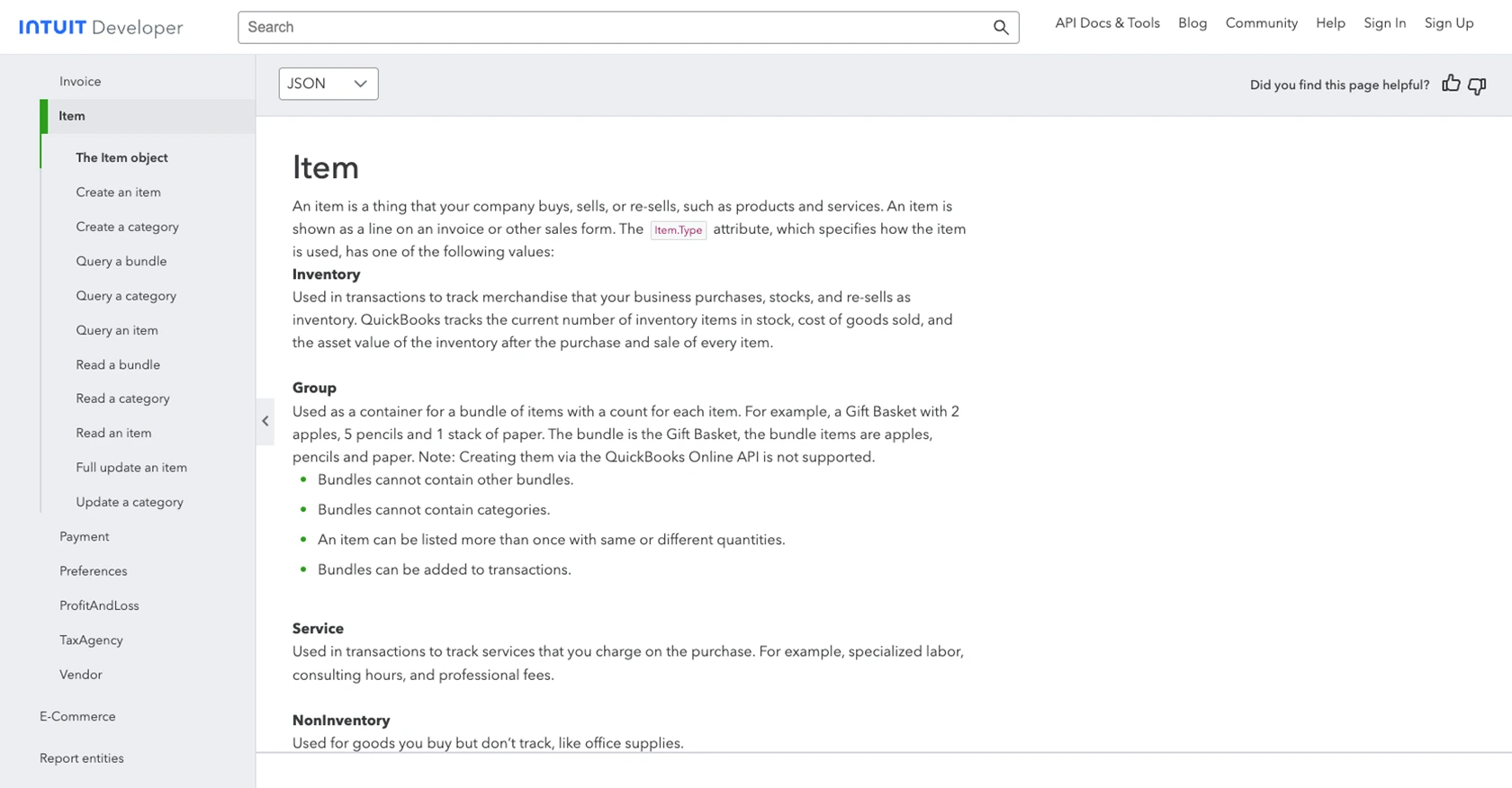
Best Practices for QuickBooks API Integration
When integrating with the QuickBooks API, it's essential to follow best practices to ensure a secure and efficient implementation. Here are some recommendations:
- Securely Store OAuth Credentials: Always store your Client ID, Client Secret, and access tokens securely. Use environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: QuickBooks API has rate limits to prevent abuse. Monitor the response headers for rate limit information and implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from QuickBooks is transformed and standardized to match your application's data model. This will help maintain consistency across your systems.
Streamlining Integrations with Endgrate
Building and maintaining integrations with multiple platforms like QuickBooks can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including QuickBooks.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the integration complexities.
- Build Once, Use Everywhere: Develop a single integration for each use case and apply it across multiple platforms.
- Enhance User Experience: Offer your customers a seamless and intuitive integration experience.
Explore how Endgrate can help you streamline your integration efforts by visiting Endgrate.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/most-commonly-used/item
Ready to get started?