Using the Quickbooks API to Get Vendors in Javascript
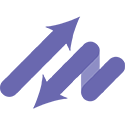
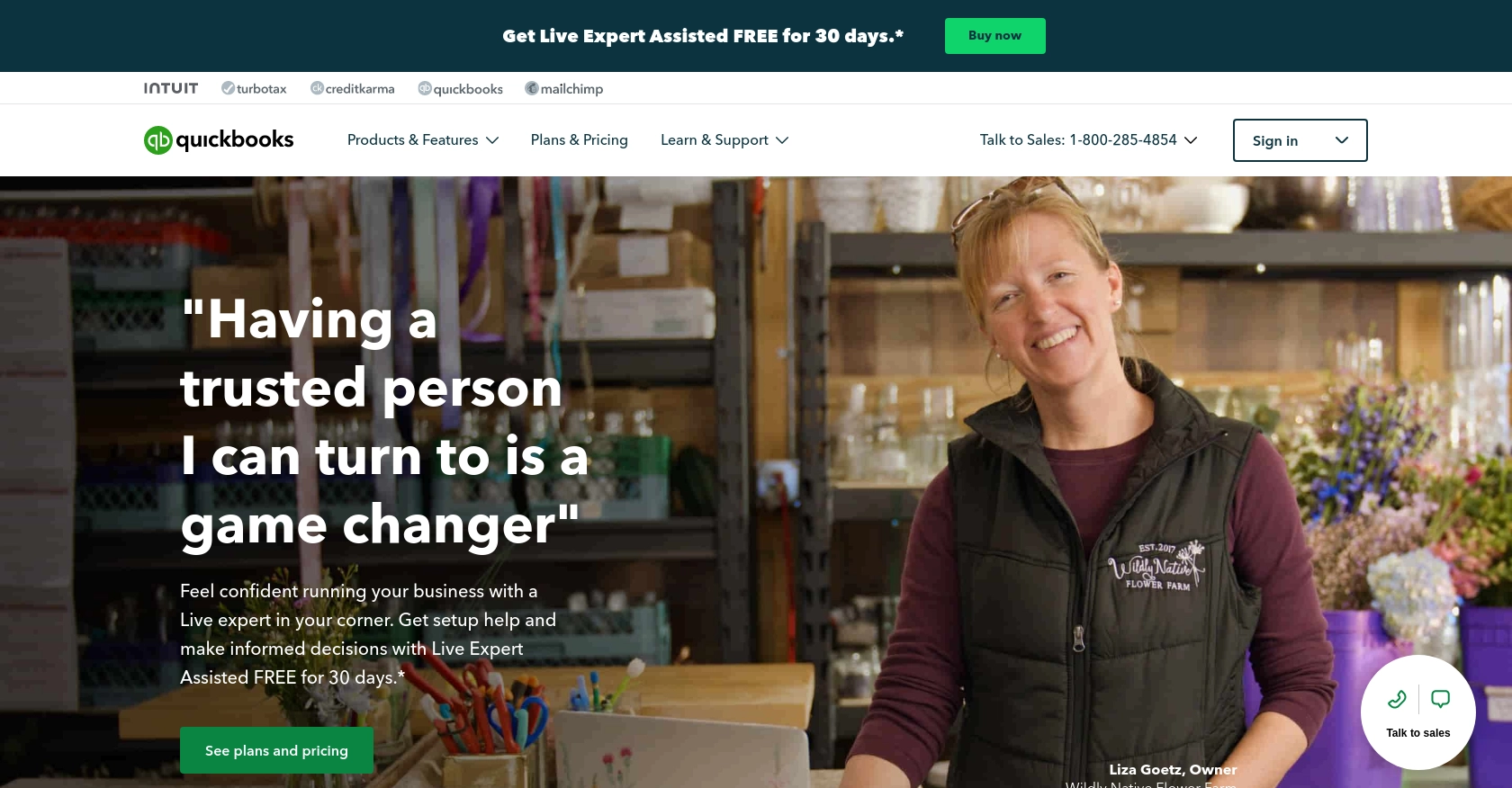
Introduction to Quickbooks API Integration
Quickbooks is a widely-used accounting software that helps businesses manage their finances with ease. It offers a robust set of tools for tracking expenses, invoicing, payroll, and more, making it an essential platform for small to medium-sized enterprises.
Developers may want to integrate with Quickbooks to streamline financial operations and automate data management. By accessing the Quickbooks API, you can retrieve vendor information, which is crucial for maintaining accurate records and ensuring smooth transactions. For example, a developer might use the Quickbooks API to automatically sync vendor details with an internal inventory system, reducing manual data entry and minimizing errors.
Setting Up Your Quickbooks Sandbox Account for API Integration
Before you can start interacting with the Quickbooks API, you'll need to set up a sandbox account. This allows you to test your integration without affecting live data. Quickbooks provides a sandbox environment that mimics the live environment, making it an ideal place for developers to experiment and build integrations.
Creating a Quickbooks Developer Account
To begin, you'll need to create a Quickbooks Developer account. Follow these steps:
- Visit the Quickbooks Developer Portal.
- Click on the "Sign Up" button and fill out the required information to create your account.
- Once your account is created, log in to the Quickbooks Developer Dashboard.
Setting Up a Quickbooks Sandbox Company
After creating your developer account, you need to set up a sandbox company:
- Navigate to the "Sandbox" section in the Quickbooks Developer Dashboard.
- Click on "Add Sandbox" to create a new sandbox company.
- Choose the type of company that best fits your testing needs and complete the setup process.
Creating a Quickbooks App for OAuth Authentication
Quickbooks uses OAuth 2.0 for authentication. You'll need to create an app to obtain the necessary credentials:
- In the Developer Dashboard, go to the "My Apps" section and click on "Create an App."
- Select "Quickbooks Online and Payments" as the platform.
- Fill in the required app details and click "Create App."
Obtaining Client ID and Client Secret
Once your app is created, you'll receive a Client ID and Client Secret. These are essential for authenticating API requests:
- Go to the "Keys & OAuth" section of your app settings.
- Copy the Client ID and Client Secret. Keep these credentials secure, as they are used to authorize API calls.
For more detailed instructions, refer to the Quickbooks OAuth Documentation.
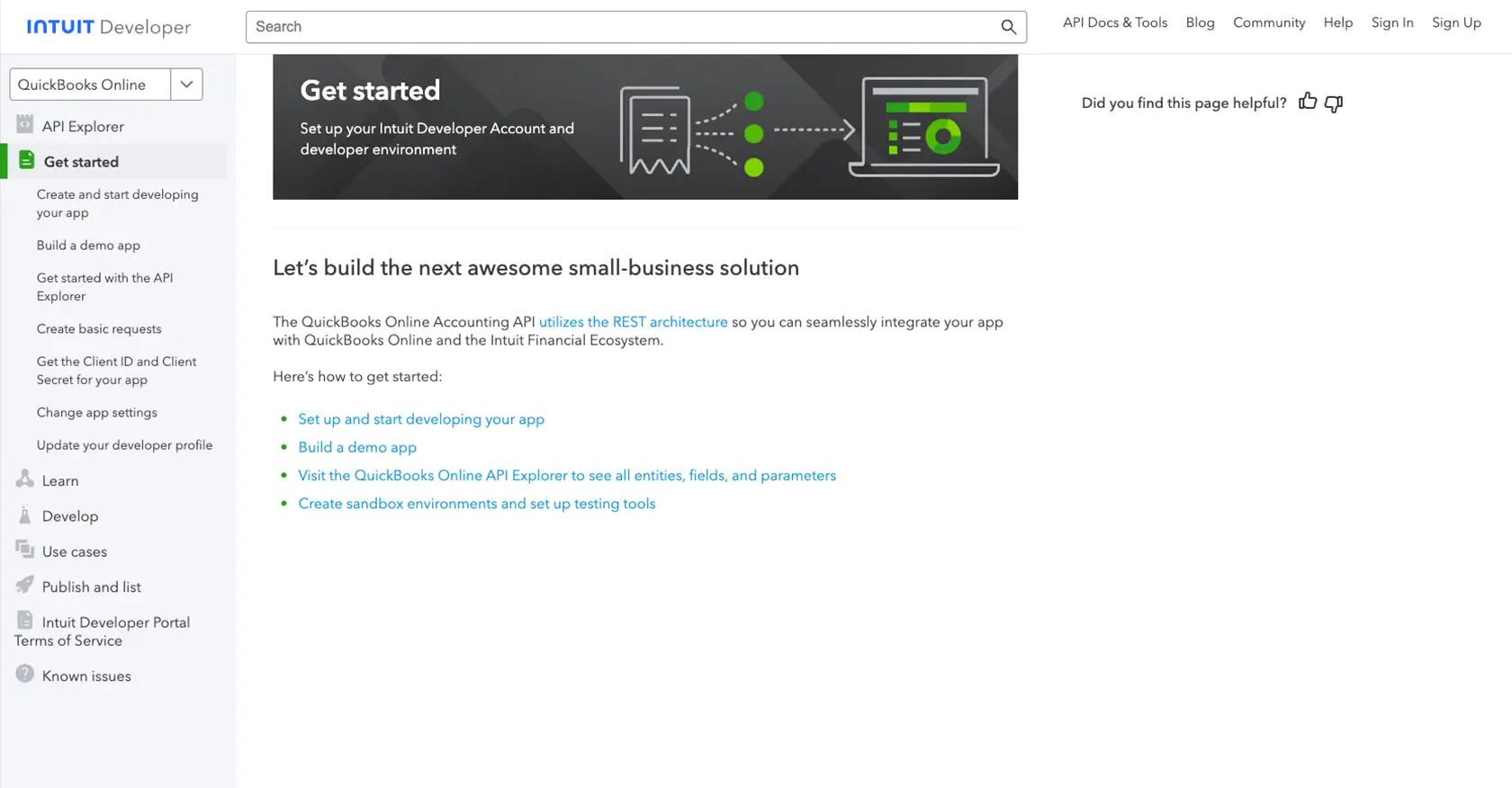
sbb-itb-96038d7
Making API Calls to Retrieve Vendors from Quickbooks Using JavaScript
To interact with the Quickbooks API and retrieve vendor information, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment and executing the necessary API calls.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A code editor such as Visual Studio Code.
- Install the Axios library for making HTTP requests by running the following command in your terminal:
npm install axios
Writing JavaScript Code to Fetch Vendors from Quickbooks
Now that your environment is set up, you can write the JavaScript code to interact with the Quickbooks API. Create a new file named getVendors.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://quickbooks.api.intuit.com/v3/company/YOUR_COMPANY_ID/vendor';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Accept': 'application/json'
};
// Function to get vendors
async function getVendors() {
try {
const response = await axios.get(endpoint, { headers });
const vendors = response.data.Vendor;
console.log('Vendors:', vendors);
} catch (error) {
console.error('Error fetching vendors:', error.response ? error.response.data : error.message);
}
}
// Execute the function
getVendors();
Replace YOUR_COMPANY_ID
and YOUR_ACCESS_TOKEN
with your actual Quickbooks company ID and access token obtained during the OAuth setup.
Running the JavaScript Code and Verifying Results
To execute the code, run the following command in your terminal:
node getVendors.js
If successful, you should see a list of vendors printed in the console. This confirms that the API call was made correctly and the data was retrieved from your Quickbooks sandbox account.
Handling Errors and Quickbooks API Response Codes
While making API calls, it's crucial to handle potential errors. The Quickbooks API may return various error codes, such as:
- 401 Unauthorized: Indicates an issue with authentication. Check your access token.
- 403 Forbidden: You might not have the necessary permissions to access the resource.
- 404 Not Found: The requested resource could not be found.
For more detailed error handling, refer to the Quickbooks API Documentation.
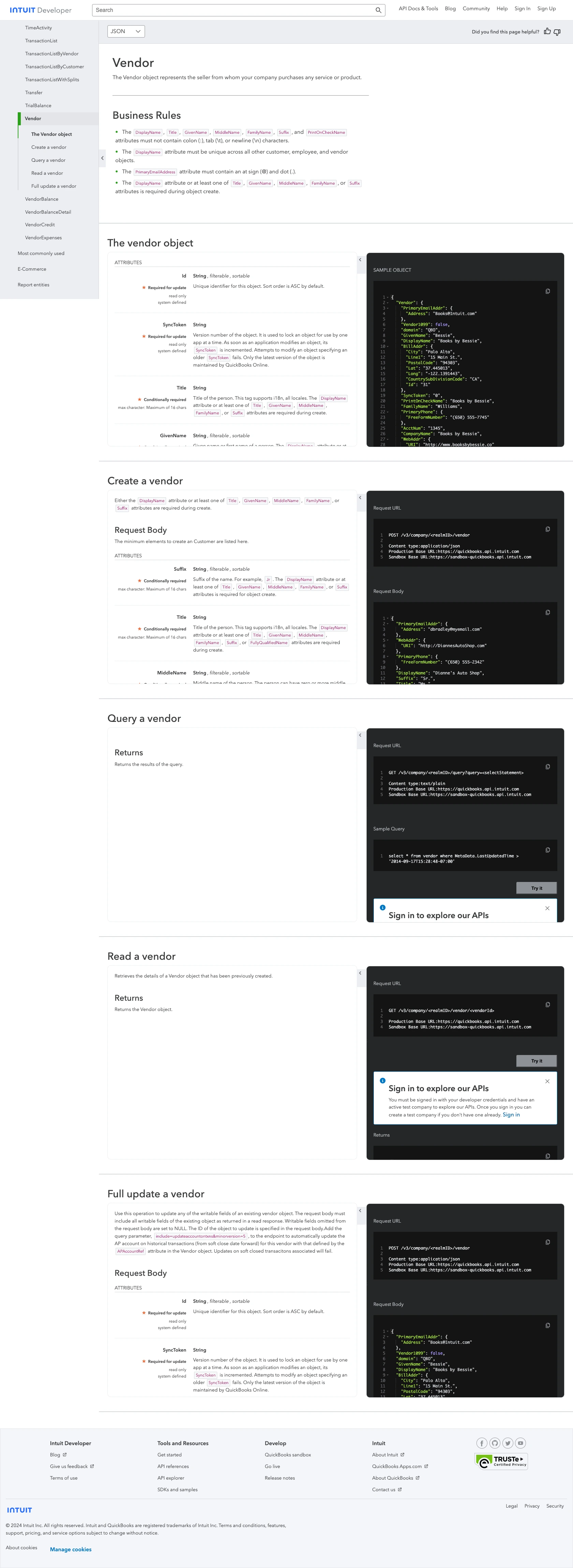
Conclusion and Best Practices for Quickbooks API Integration
Integrating with the Quickbooks API using JavaScript provides a powerful way to automate and streamline financial operations. By retrieving vendor information, developers can enhance data accuracy and reduce manual entry, leading to more efficient business processes.
Best Practices for Secure and Efficient Quickbooks API Usage
- Securely Store Credentials: Always keep your Client ID, Client Secret, and access tokens secure. Consider using environment variables or secure vaults to store these sensitive details.
- Handle Rate Limiting: Quickbooks API has rate limits that you must adhere to. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that data retrieved from Quickbooks is transformed and standardized to fit your internal systems, maintaining consistency across platforms.
Streamline Your Integration Process with Endgrate
While integrating with Quickbooks API is beneficial, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies this process, allowing you to focus on your core product development. By using Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers.
Explore how Endgrate can help you save time and resources by visiting Endgrate today.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/all-entities/vendor
Ready to get started?