Using the Younium API to Create or Update Invoices (with Python examples)
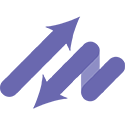
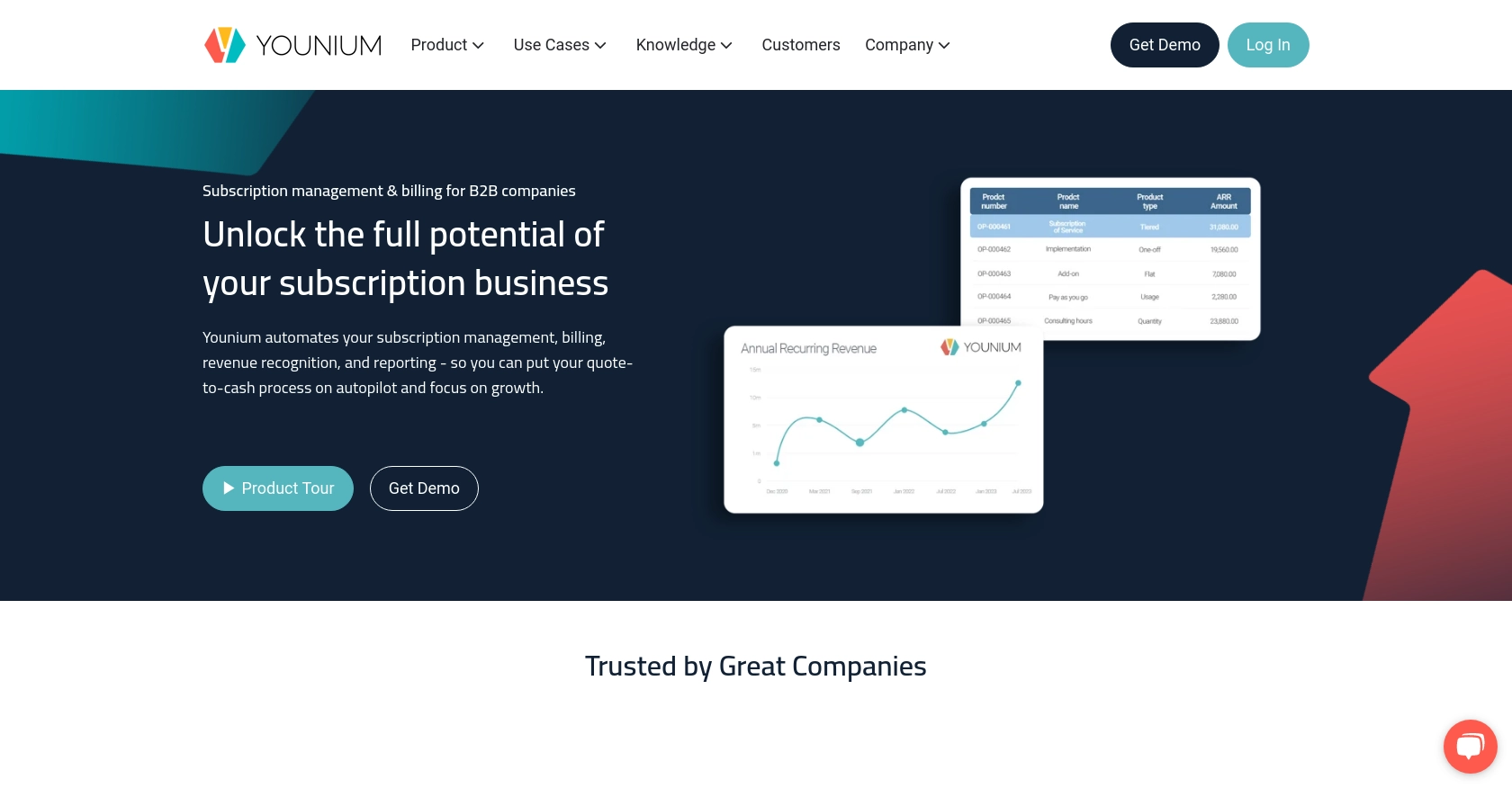
Introduction to Younium API for Invoice Management
Younium is a robust subscription management platform designed to streamline billing and invoicing processes for B2B SaaS companies. It offers a comprehensive suite of tools to manage subscriptions, automate billing, and generate detailed financial reports, making it an essential tool for businesses looking to optimize their financial operations.
Integrating with the Younium API allows developers to automate invoice creation and updates, enhancing efficiency and accuracy in financial management. For example, a developer might use the Younium API to automatically generate invoices based on subscription data, ensuring timely billing and reducing manual errors.
Setting Up Your Younium Sandbox Account for API Integration
Before you can start integrating with the Younium API, you'll need to set up a sandbox account. This environment allows you to test API interactions without affecting your production data, ensuring a safe and controlled development process.
Creating a Younium Sandbox Account
If you don't already have a Younium account, you can sign up for a sandbox account on the Younium website. This account will provide you with the necessary environment to test API calls and develop your integration.
- Visit the Younium Developer Portal.
- Follow the instructions to create a sandbox account.
- Once your account is set up, log in to access the sandbox environment.
Generating API Tokens and Client Credentials
To authenticate your API requests, you'll need to generate API tokens and client credentials. This process involves creating a dedicated API user with the necessary permissions.
- Log in to your Younium account and open the user profile menu by clicking your name in the top right corner.
- Select "Privacy & Security" from the dropdown menu.
- Navigate to "Personal Tokens" and click "Generate Token".
- Provide a relevant description and click "Create".
- Copy the generated Client ID and Secret Key. These credentials are crucial for generating your JWT access token and will not be visible again.
Acquiring a JWT Access Token
With your client credentials ready, you can now generate a JWT access token. This token is required for authenticating API requests to Younium.
import requests
# Define the endpoint and headers
url = "https://api.sandbox.younium.com/auth/token"
headers = {"Content-Type": "application/json"}
# Set the request body with your client credentials
body = {
"clientId": "Your_Client_ID",
"secret": "Your_Secret_Key"
}
# Make the POST request to acquire the JWT token
response = requests.post(url, json=body, headers=headers)
# Extract the access token from the response
access_token = response.json().get("accessToken")
print("Access Token:", access_token)
Replace Your_Client_ID
and Your_Secret_Key
with the credentials you generated earlier. The access token is valid for 24 hours, after which you'll need to request a new one.
Handling Authentication Errors
If you encounter any errors during authentication, refer to the following common issues:
- 401 Unauthorized: Indicates an expired, missing, or incorrect access token.
- 403 Forbidden: Occurs if the legal entity provided is invalid or if the user lacks necessary permissions.
For more details, visit the Younium Authentication Documentation.
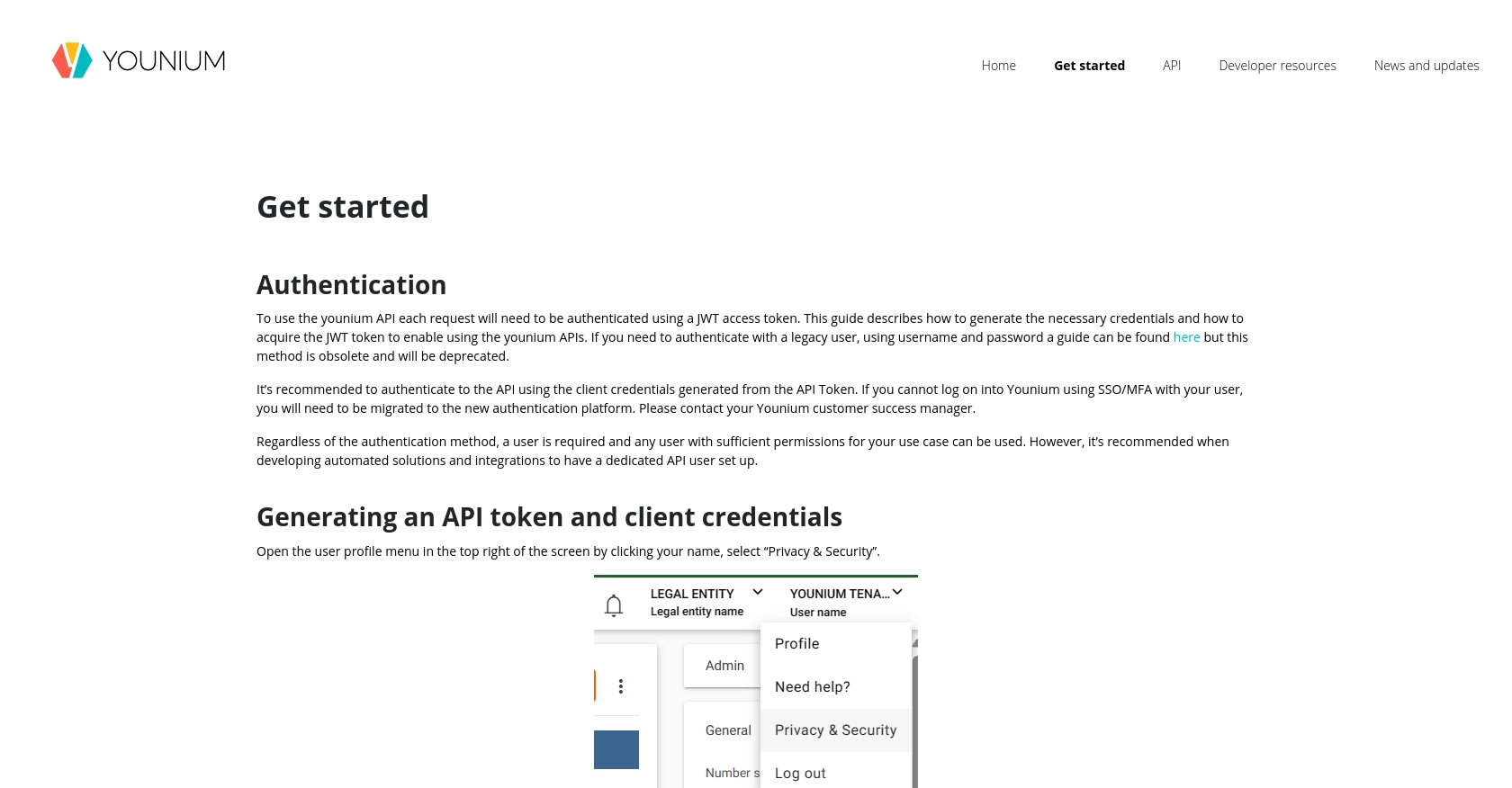
sbb-itb-96038d7
Making API Calls to Younium for Invoice Management Using Python
With your authentication set up, you can now proceed to make API calls to Younium for creating or updating invoices. This section will guide you through the process using Python, ensuring you have the necessary tools and knowledge to interact with the Younium API effectively.
Prerequisites for Younium API Integration with Python
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer
pip
Additionally, you need to install the requests
library to handle HTTP requests. You can do this by running the following command in your terminal:
pip install requests
Creating an Invoice with Younium API
To create an invoice using the Younium API, you'll need to make a POST request to the appropriate endpoint. Below is a Python example demonstrating how to achieve this:
import requests
# Define the endpoint and headers
url = "https://api.sandbox.younium.com/invoices"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json",
"api-version": "2.1"
}
# Set the request body with invoice details
invoice_data = {
"accountId": "your_account_id",
"currency": "USD",
"invoiceDate": "2023-10-01",
"dueDate": "2023-10-15",
"items": [
{
"description": "Subscription Service",
"quantity": 1,
"unitPrice": 100.00
}
]
}
# Make the POST request to create the invoice
response = requests.post(url, json=invoice_data, headers=headers)
# Check if the request was successful
if response.status_code == 201:
print("Invoice Created Successfully")
else:
print("Failed to Create Invoice:", response.json())
Replace Your_Access_Token
and your_account_id
with the appropriate values. If the request is successful, you should see "Invoice Created Successfully" in the output.
Updating an Invoice with Younium API
To update an existing invoice, you will need to make a PUT request to the Younium API. Here is an example of how to update an invoice using Python:
import requests
# Define the endpoint and headers
invoice_id = "your_invoice_id"
url = f"https://api.sandbox.younium.com/invoices/{invoice_id}"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json",
"api-version": "2.1"
}
# Set the request body with updated invoice details
updated_invoice_data = {
"dueDate": "2023-10-20",
"items": [
{
"description": "Updated Subscription Service",
"quantity": 2,
"unitPrice": 90.00
}
]
}
# Make the PUT request to update the invoice
response = requests.put(url, json=updated_invoice_data, headers=headers)
# Check if the request was successful
if response.status_code == 200:
print("Invoice Updated Successfully")
else:
print("Failed to Update Invoice:", response.json())
Ensure you replace Your_Access_Token
and your_invoice_id
with the correct values. A successful update will result in "Invoice Updated Successfully" being printed.
Handling Errors and Verifying API Requests
When making API calls, it's crucial to handle potential errors effectively. Common error codes include:
- 400 Bad Request: Indicates a malformed request. Check the request body and headers.
- 401 Unauthorized: Indicates an issue with the access token. Ensure it is valid and correctly included.
- 403 Forbidden: Occurs if the user lacks the necessary permissions or if the legal entity is incorrect.
After making API calls, verify the changes in your Younium sandbox account to ensure the invoices are created or updated as expected. For more information, refer to the Younium API Documentation.
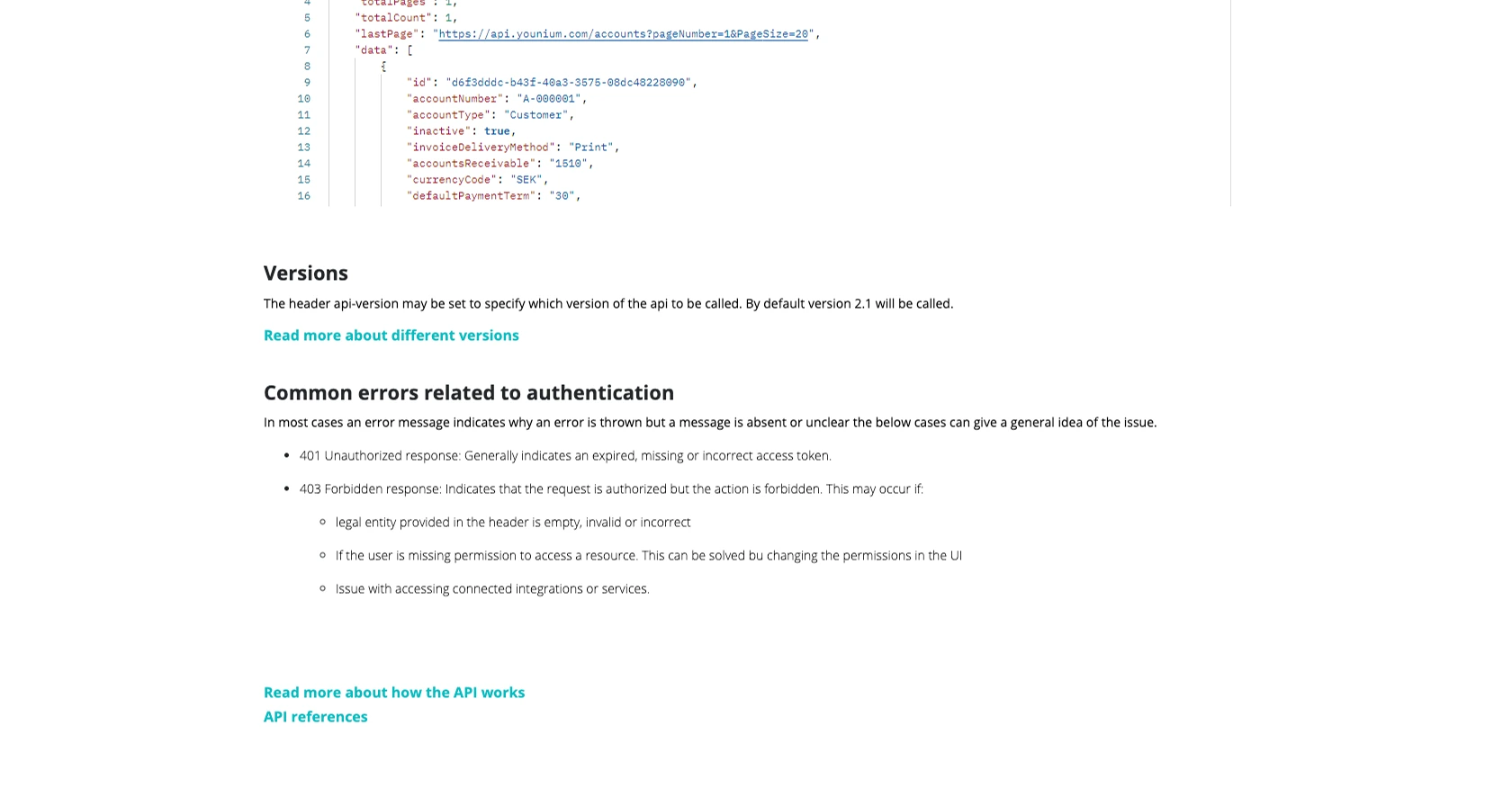
Conclusion and Best Practices for Younium API Integration
Integrating with the Younium API for invoice management can significantly enhance the efficiency and accuracy of your financial operations. By automating invoice creation and updates, you can ensure timely billing and reduce manual errors, ultimately optimizing your business processes.
Best Practices for Secure and Efficient Younium API Usage
- Secure Storage of Credentials: Always store your API credentials securely. Use environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of Younium's rate limits to avoid service disruptions. Implement retry logic with exponential backoff to handle rate-limited responses gracefully.
- Data Standardization: Ensure that your data fields are standardized and consistent with Younium's requirements to prevent errors during API interactions.
- Regular Token Renewal: Remember that JWT access tokens are valid for 24 hours. Implement a mechanism to refresh tokens automatically to maintain uninterrupted access.
Enhance Your Integration Strategy with Endgrate
While integrating with Younium's API can streamline your invoicing processes, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies integration management across various platforms, including Younium.
By leveraging Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product development.
- Build once for each use case instead of multiple times for different integrations, enhancing efficiency.
- Provide an intuitive integration experience for your customers, improving user satisfaction.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?