How to Get Items with the Xero API in Python
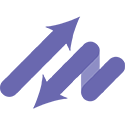
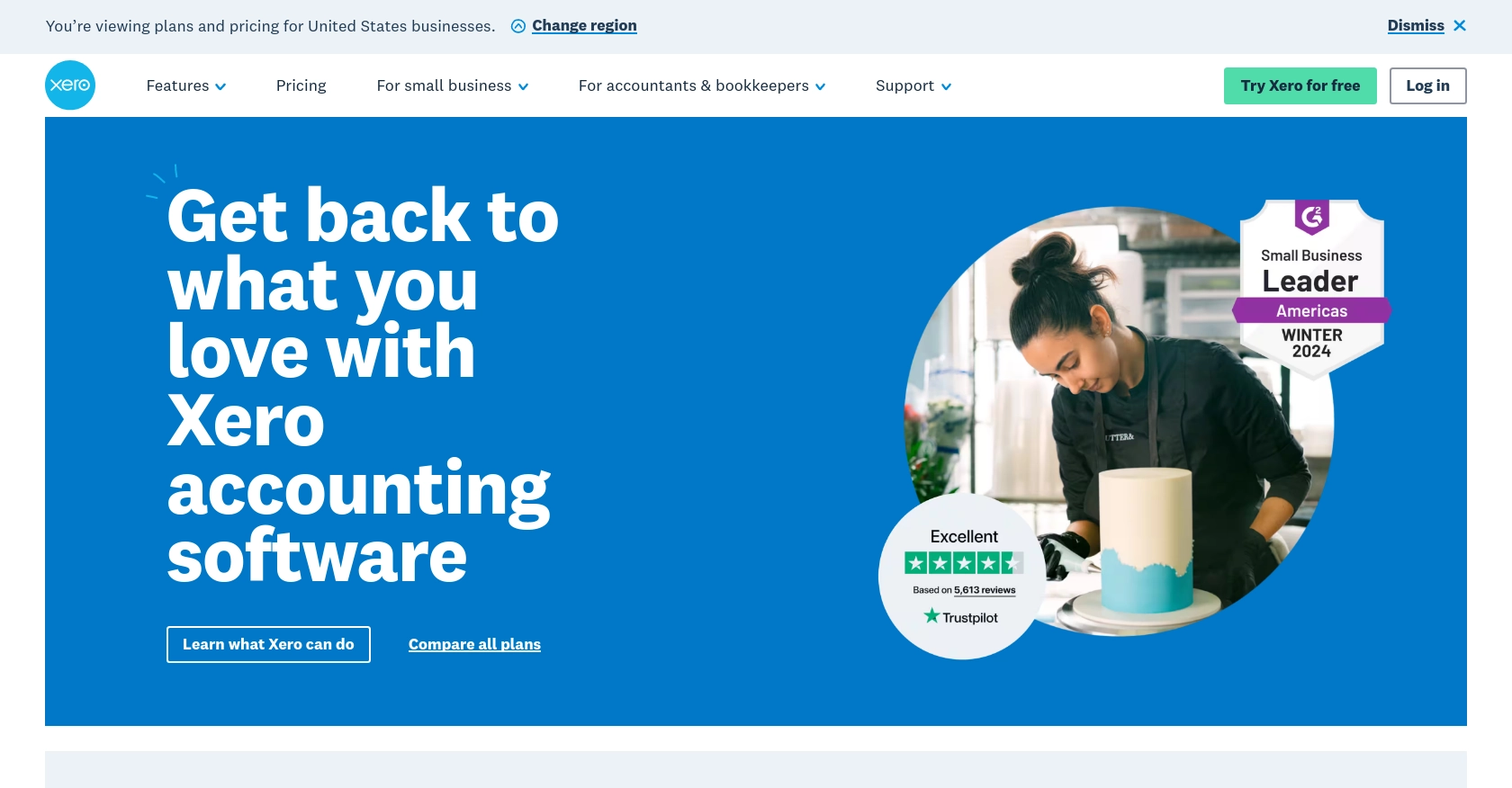
Introduction to Xero API Integration
Xero is a powerful cloud-based accounting software platform designed to help small and medium-sized businesses manage their finances efficiently. With features like invoicing, bank reconciliation, and financial reporting, Xero provides a comprehensive solution for businesses looking to streamline their accounting processes.
Integrating with Xero's API allows developers to access and manipulate financial data programmatically, enabling automation and customization of accounting workflows. For example, a developer might use the Xero API to retrieve item data, such as inventory details, to keep an external inventory management system in sync with Xero's records.
Setting Up Your Xero Test/Sandbox Account for API Integration
Before diving into the Xero API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data. Xero provides a demo company that you can use for testing purposes.
Creating a Xero Account and Accessing the Demo Company
If you don't already have a Xero account, start by signing up for a free trial on the Xero website. Once your account is created, you can access the demo company by following these steps:
- Log in to your Xero account.
- Click on your organization name in the top left corner.
- Select "My Xero" and then "Try the Demo Company" from the dropdown menu.
The demo company provides a pre-populated set of data that you can use to test API interactions.
Setting Up OAuth 2.0 Authentication for Xero API
Xero uses OAuth 2.0 for authentication, which requires you to create an app to obtain the necessary credentials. Follow these steps to set up OAuth 2.0:
- Navigate to the Xero Developer Portal and log in with your Xero account.
- Click on "New App" and fill in the required information, such as the app name and company URL.
- Set the redirect URI to a valid URL where you can receive authorization codes.
- Once your app is created, note down the client ID and client secret. These will be used to authenticate API requests.
For more details on OAuth 2.0 authentication, refer to the Xero OAuth 2.0 documentation.
Configuring Scopes and Permissions
When creating your app, you'll need to configure the necessary scopes to access specific API endpoints. For retrieving items, ensure you have the appropriate scopes set:
- Open your app settings in the Xero Developer Portal.
- Navigate to the "Scopes" section and select the scopes related to items and inventory.
These scopes grant your app the permissions needed to interact with the Xero API.
With your sandbox account and OAuth 2.0 authentication set up, you're ready to start making API calls to Xero. In the next section, we'll explore how to retrieve item data using Python.
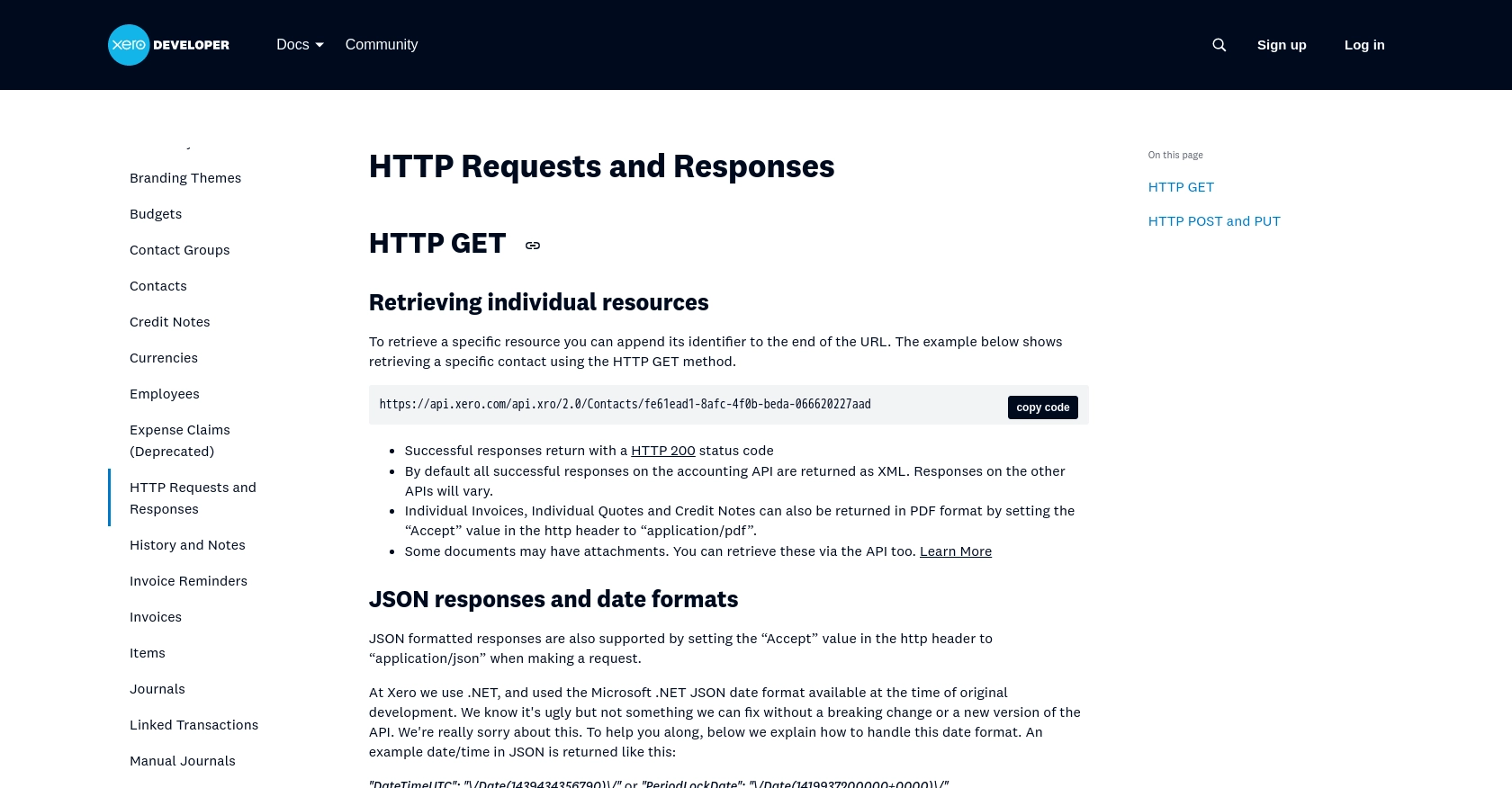
sbb-itb-96038d7
Making API Calls to Retrieve Items from Xero Using Python
To interact with the Xero API and retrieve item data, you'll need to use Python, a versatile programming language known for its simplicity and readability. This section will guide you through setting up your Python environment, making the API call, and handling the response.
Setting Up Your Python Environment for Xero API Integration
Before you begin, ensure you have Python 3.11.1 installed on your machine. You'll also need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
Writing Python Code to Fetch Items from Xero API
Create a new Python file named get_xero_items.py
and add the following code:
import requests
# Define the API endpoint and headers
endpoint = "https://api.xero.com/api.xro/2.0/Items"
headers = {
"Authorization": "Bearer Your_Token",
"Accept": "application/json"
}
# Make a GET request to the Xero API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
items = response.json()
for item in items['Items']:
print(f"Item Name: {item['Name']}, Item Code: {item['Code']}")
else:
print(f"Failed to retrieve items: {response.status_code} - {response.text}")
Replace Your_Token
with the access token obtained during the OAuth 2.0 authentication process.
Running the Python Script and Verifying the Output
Execute the script using the following command:
python get_xero_items.py
If successful, the script will print the names and codes of items retrieved from your Xero demo company. If there's an error, the script will output the status code and error message.
Handling Errors and Understanding Xero API Response Codes
When making API calls, it's crucial to handle potential errors gracefully. The Xero API may return various HTTP status codes indicating the result of your request:
- 200 OK: The request was successful, and the items were retrieved.
- 401 Unauthorized: Authentication failed. Check your access token.
- 403 Forbidden: The request is not allowed. Verify your app's scopes and permissions.
- 429 Too Many Requests: You've hit the rate limit. Wait before retrying.
For more information on error codes, refer to the Xero API documentation.
By following these steps, you can efficiently retrieve item data from Xero using Python, enabling seamless integration with your applications.
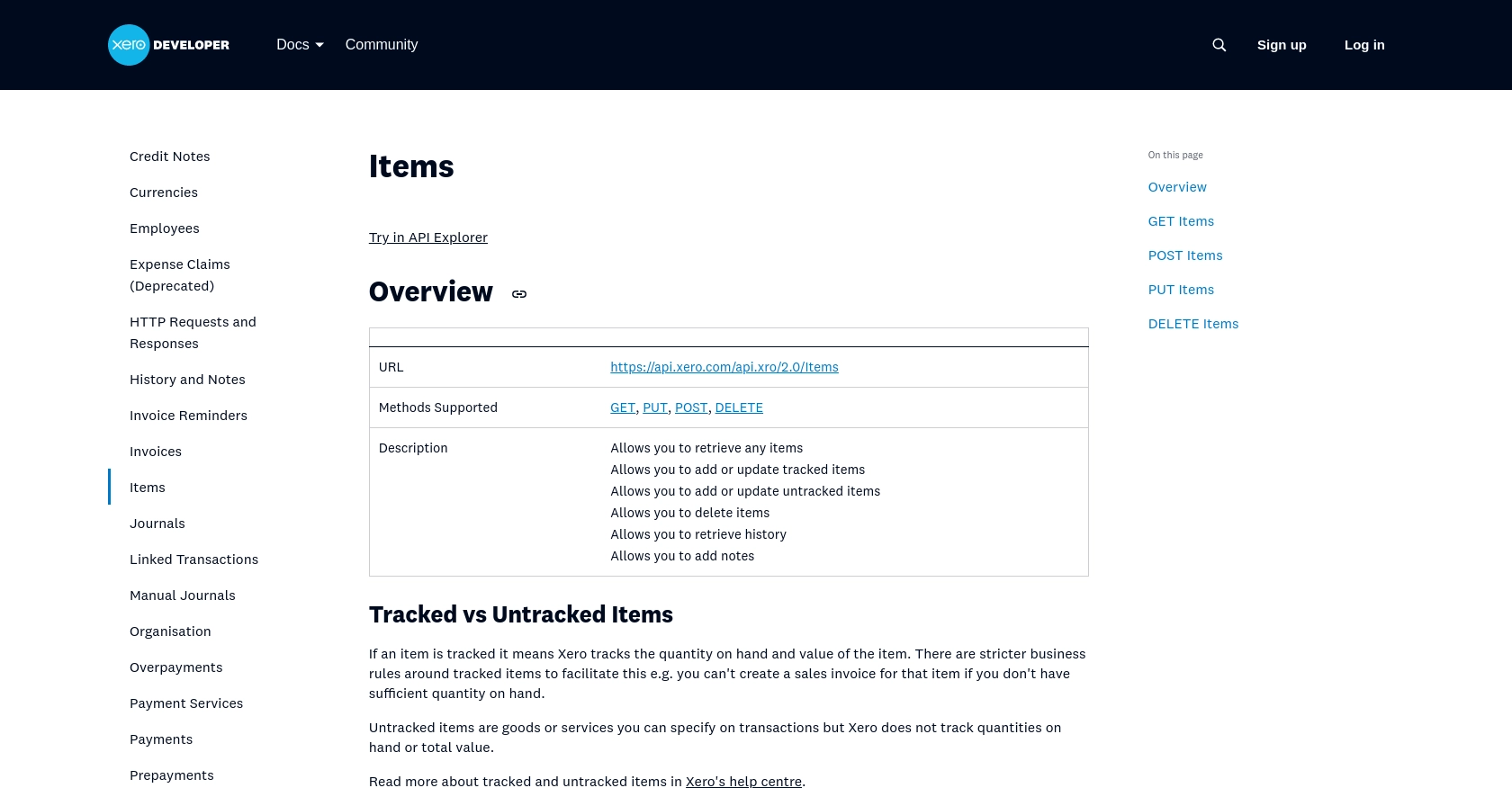
Conclusion and Best Practices for Xero API Integration
Integrating with the Xero API using Python provides a powerful way to automate and customize your accounting workflows. By retrieving item data programmatically, you can ensure that your inventory management systems remain synchronized with Xero, enhancing efficiency and accuracy.
Best Practices for Secure and Efficient Xero API Usage
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limiting: Xero imposes rate limits on API requests. If you encounter a
429 Too Many Requests
error, implement exponential backoff or retry logic to manage your request rate. For more details, refer to the Xero API rate limits documentation. - Transform and Standardize Data: When integrating data from Xero into other systems, ensure that data fields are transformed and standardized to match the target system's requirements.
Leverage Endgrate for Seamless Integration
While building integrations with the Xero API can be rewarding, it can also be time-consuming and complex. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to multiple platforms, including Xero. This allows you to focus on your core product while outsourcing integration complexities.
With Endgrate, you can build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can save you time and resources by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/items
Ready to get started?