Using the Sage 100 API to Create or Update Sales Orders (with PHP examples)
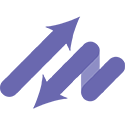
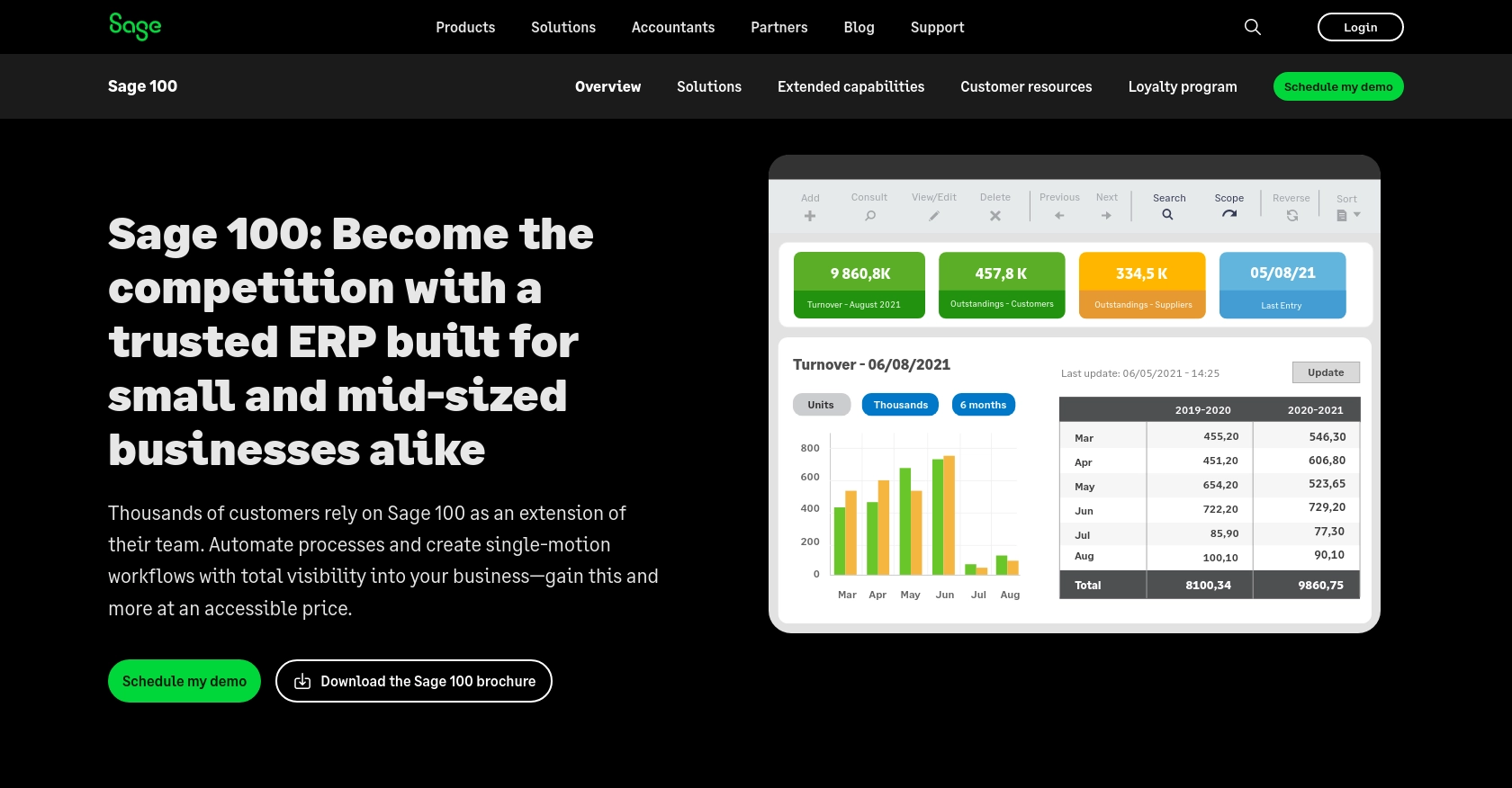
Introduction to Sage 100 API for Sales Order Management
Sage 100 is a comprehensive ERP solution designed to streamline business operations, including accounting, inventory management, and sales order processing. It is widely used by small to medium-sized businesses to enhance productivity and efficiency.
Integrating with the Sage 100 API allows developers to automate and manage sales orders effectively. By using the API, you can create or update sales orders directly from your application, ensuring seamless data flow and reducing manual entry errors.
For example, a developer might want to automate the process of updating sales order statuses based on inventory levels or customer interactions, thereby improving order fulfillment speed and accuracy.
Setting Up a Test/Sandbox Account for Sage 100 API Integration
Before you can start creating or updating sales orders using the Sage 100 API, you need to set up a test or sandbox environment. This allows you to safely experiment with API calls without affecting your live data.
Installing and Configuring the Sage 100 ODBC Driver
To interact with the Sage 100 API, ensure that the Sage 100 ODBC driver is installed and properly configured. Follow these steps to set up the ODBC driver:
- Access the ODBC Data Source Administrator on your system.
- Create a Data Source Name (DSN) that connects to the Sage 100 ERP system using the correct server, database, and authentication settings.
- Refer to the official Sage 100 documentation for detailed instructions on configuring the ODBC driver.
Creating a DSN for Sage 100
Once the ODBC driver is installed, create a DSN to establish a connection to the Sage 100 database:
- Open the ODBC Data Source Administrator.
- Navigate to the User DSN or System DSN tab and click on "Add".
- Select the Sage 100 ODBC driver from the list and click "Finish".
- Enter the necessary connection details, including server name, database, and authentication credentials.
- Test the connection to ensure it is successful.
Configuring Sage 100 for API Access
After setting up the DSN, configure Sage 100 to allow API access:
- In Sage 100, go to Library Master > Setup > System Configuration.
- On the ODBC Driver tab, select the "Enable C/S ODBC Driver" checkbox.
- Enter the server name or IP address where the ODBC application or service is running.
- Specify the server port or leave it blank to use the default port, 20222.
- Enable the ODBC driver for all users or individual users as needed.
Testing the ODBC Connection
To ensure everything is set up correctly, test the ODBC connection:
- For 32-bit systems, open the Windows Control Panel and double-click Administrative Tools, then Data Sources (ODBC).
- For 64-bit systems, navigate to
c:\windows\SysWOW64
and double-clickOdbcad32.exe
. - Double-click the SOTAMAS90 item and click the Debug tab, then click "Test Connection".
- If the connection is successful, you are ready to proceed with API calls.
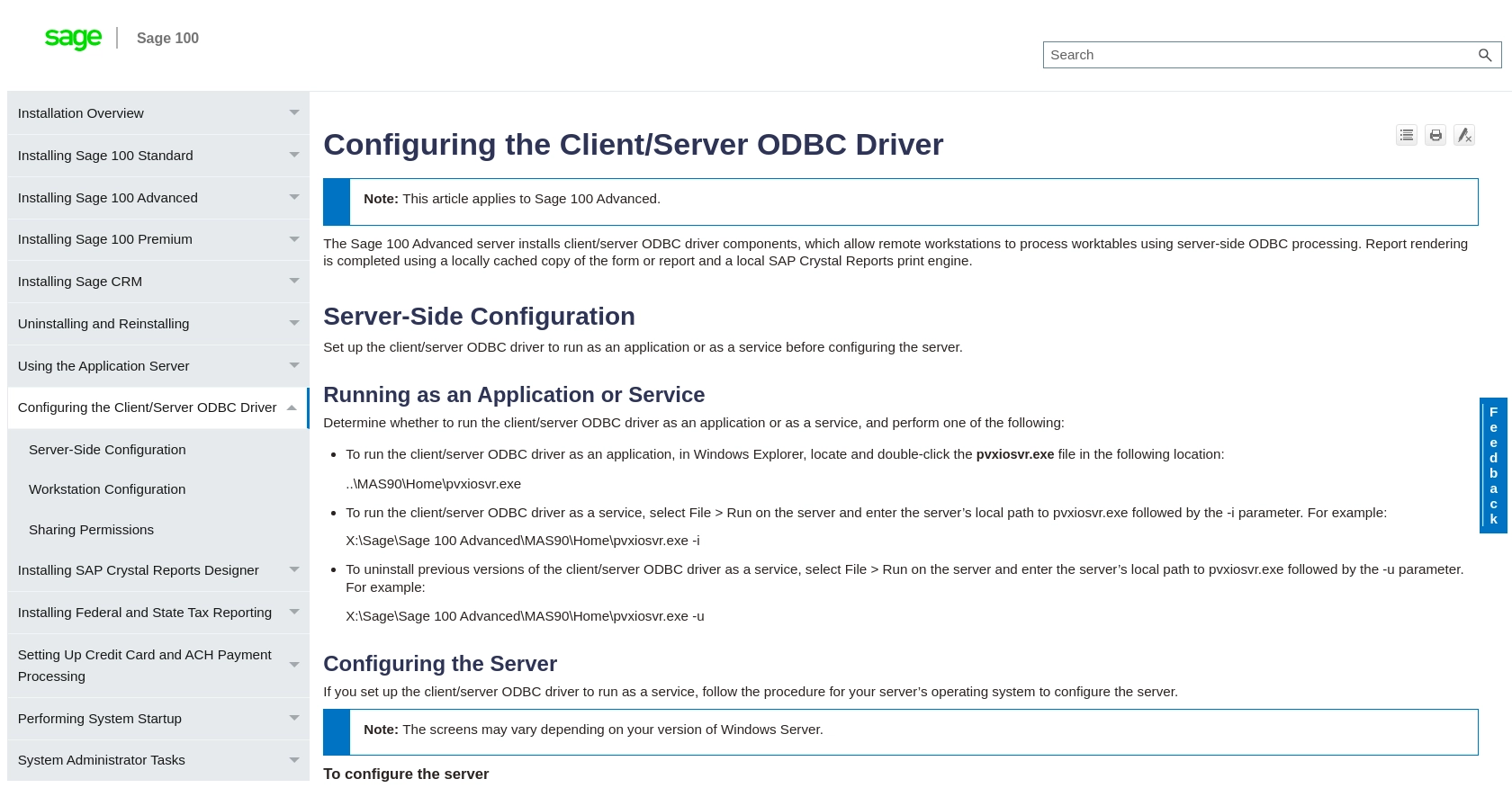
sbb-itb-96038d7
Making API Calls to Sage 100 for Sales Order Management Using PHP
To interact with the Sage 100 API for creating or updating sales orders, you'll need to use PHP to establish a connection and execute the necessary API calls. This section will guide you through setting up your PHP environment, writing the code to interact with the API, and handling responses effectively.
Setting Up Your PHP Environment for Sage 100 API Integration
Before making API calls, ensure your PHP environment is properly configured:
- Install PHP 7.4 or later on your system.
- Ensure the ODBC extension is enabled in your
php.ini
file. - Install necessary dependencies using Composer, if applicable.
Creating a PHP Script to Connect to Sage 100
Begin by creating a PHP script to establish a connection to the Sage 100 database using the ODBC driver:
<?php
// Define DSN and credentials
$dsn = 'DSN=SOTAMAS90';
$user = 'your_username';
$password = 'your_password';
// Establish ODBC connection
$conn = odbc_connect($dsn, $user, $password);
if (!$conn) {
die("Connection failed: " . odbc_errormsg());
}
echo "Connected successfully to Sage 100.";
?>
Replace your_username
and your_password
with your actual Sage 100 credentials. This script establishes a connection to the Sage 100 database using the DSN configured earlier.
Executing API Calls to Create or Update Sales Orders in Sage 100
Once connected, you can execute SQL queries to create or update sales orders. Here's an example of how to create a new sales order:
<?php
// SQL query to insert a new sales order
$sql = "INSERT INTO SO_SalesOrderHeader (SalesOrderNo, CustomerNo, OrderDate) VALUES ('SO12345', 'CUST001', '2023-10-01')";
// Execute the query
$result = odbc_exec($conn, $sql);
if ($result) {
echo "Sales order created successfully.";
} else {
echo "Error creating sales order: " . odbc_errormsg($conn);
}
// Close the connection
odbc_close($conn);
?>
This script inserts a new sales order into the SO_SalesOrderHeader
table. Modify the SQL query to update existing sales orders as needed.
Handling Responses and Errors from Sage 100 API Calls
Proper error handling is crucial when interacting with the Sage 100 API. Use try-catch blocks to manage exceptions and log errors for troubleshooting:
<?php
try {
// Attempt to execute the query
$result = odbc_exec($conn, $sql);
if (!$result) {
throw new Exception("Query failed: " . odbc_errormsg($conn));
}
echo "Operation successful.";
} catch (Exception $e) {
// Log error message
error_log($e->getMessage());
echo "An error occurred. Please check the logs.";
} finally {
// Ensure the connection is closed
odbc_close($conn);
}
?>
This approach ensures that your application can gracefully handle errors and maintain a stable connection to the Sage 100 database.
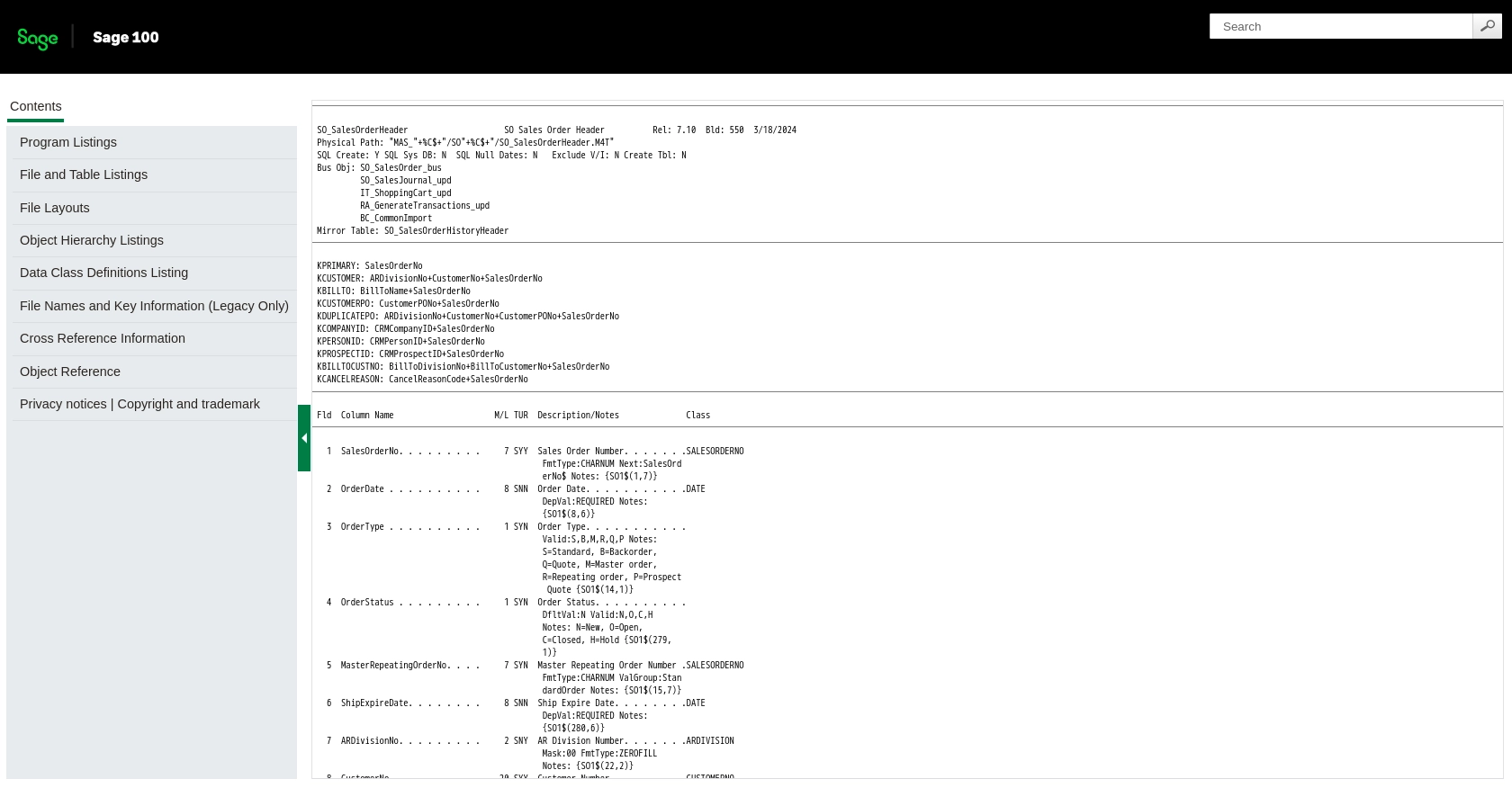
Conclusion and Best Practices for Sage 100 API Integration
Integrating with the Sage 100 API for sales order management can significantly enhance your business operations by automating processes and reducing manual errors. By following the steps outlined in this article, you can efficiently create or update sales orders using PHP, ensuring a seamless flow of data between your application and the Sage 100 ERP system.
Best Practices for Secure and Efficient Sage 100 API Usage
- Secure Credentials: Always store your Sage 100 credentials securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the Sage 100 API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data fields are standardized and validated before sending them to the API to maintain data integrity.
- Error Logging: Implement robust error logging to capture and analyze any issues that arise during API interactions. This will aid in troubleshooting and improving your integration.
Streamlining Integrations with Endgrate
While integrating with Sage 100 can be a powerful way to enhance your application, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Sage 100.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. With Endgrate's intuitive integration experience, you can build once for each use case and easily extend support to other platforms, providing a seamless experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/sage100
- https://help-sage100.na.sage.com/InstallGuide/2022/Content/InstallGuide/Config_ODBC_Driver.htm
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Sales_Order/SO_SalesOrderHeader.htm?Highlight=SO_SalesOrderHeader
- https://help-sage100.na.sage.com/2022/FLOR/index.htm#File_Layouts/Sales_Order/SO_SalesOrderDetail.htm?Highlight=SO_SalesOrderDetail
Ready to get started?