How to Import Data from CSV with the CSV API in Python
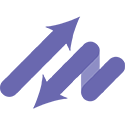
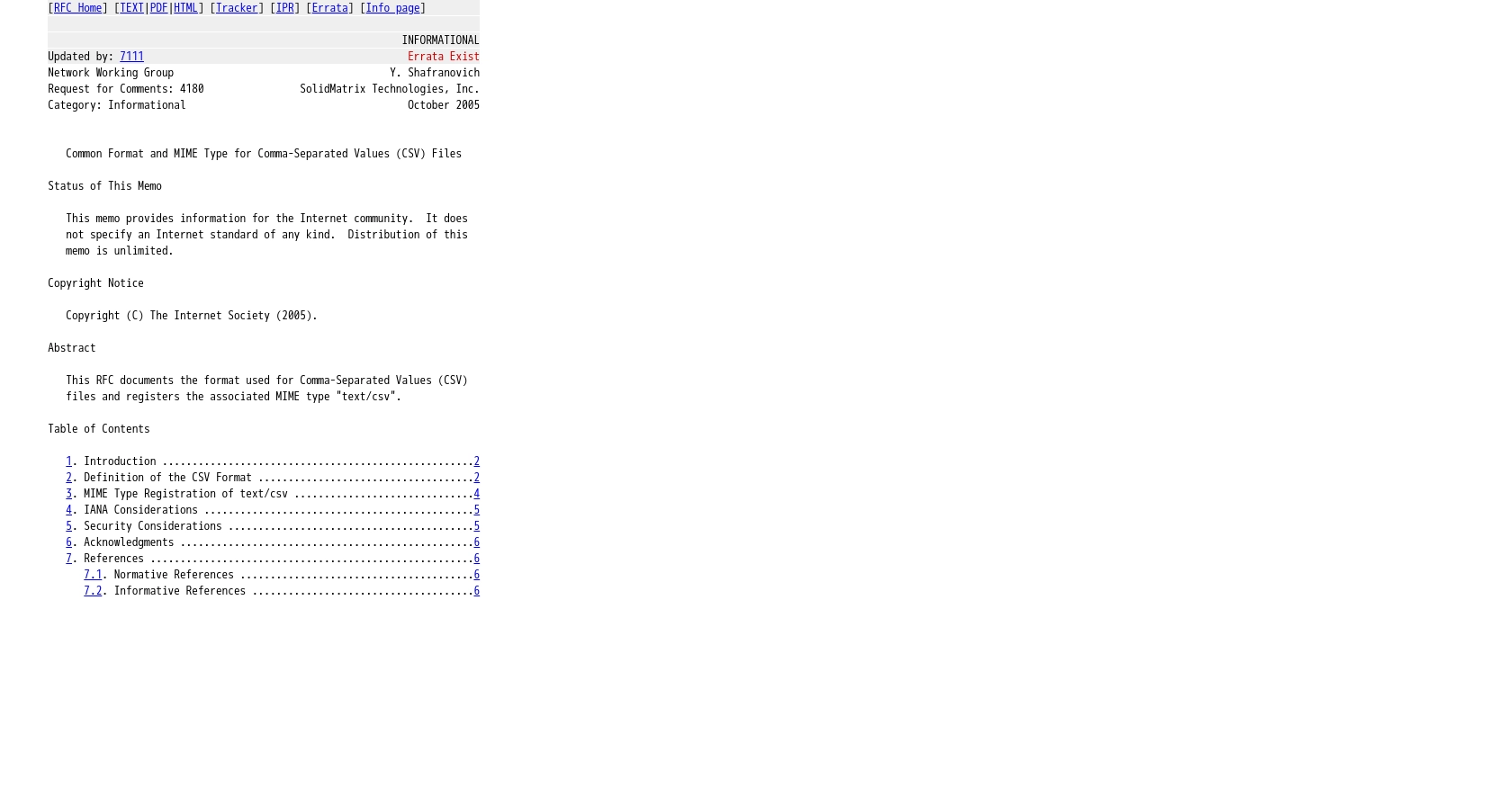
Introduction to CSV Integration
CSV (Comma-Separated Values) files are a widely used format for storing and exchanging data. They are simple, human-readable, and compatible with numerous applications, making them an ideal choice for data import and export tasks.
Integrating with a CSV API allows developers to automate the process of importing data from CSV files into their applications. This can be particularly useful for B2B SaaS products that need to handle large volumes of data efficiently. For example, a developer might use a CSV API to import customer data from a CSV file into a CRM system, streamlining data management and reducing manual entry errors.
Setting Up Your CSV API Test Environment
Before you begin importing data from CSV files using the CSV API, it's essential to set up a test environment. This will allow you to experiment with the API without affecting your production data. Follow these steps to get started:
Create a CSV API Test Account
To interact with the CSV API, you need to create a test account. This account will provide you with access to the necessary tools and resources for testing CSV data imports.
- Visit the CSV API website and sign up for a free trial or demo account.
- Follow the instructions to complete the registration process.
- Once your account is created, log in to access the CSV API dashboard.
Generate API Credentials for CSV Integration
With your test account set up, the next step is to generate the API credentials required for authentication. The CSV API uses a custom integration method, so follow these steps to obtain your credentials:
- Navigate to the API settings section in your CSV API dashboard.
- Locate the option to create new API credentials.
- Generate your API key and secret, and store them securely for use in your application.
Upload Sample CSV Files for Testing
To effectively test the CSV API, you'll need sample CSV files. These files will help you understand how the API processes data and ensure your integration works as expected.
- Create or download sample CSV files that match the data structure you plan to use.
- Upload these files to your CSV API test account using the provided interface.
- Verify that the files are correctly uploaded and accessible in your test environment.
With your test environment set up, you're ready to start importing data from CSV files using the CSV API in Python. In the next section, we'll explore how to make the actual API calls and handle the data efficiently.
Making API Calls to Import CSV Data Using Python
To import data from CSV files using the CSV API in Python, you'll need to set up your environment and write code that interacts with the API. This section will guide you through the necessary steps, including setting up Python, installing dependencies, and writing the code to make API calls.
Setting Up Your Python Environment for CSV API Integration
Before you begin, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. You'll also need the requests
library to handle HTTP requests.
- Install Python 3.11.1 from the official Python website if you haven't already.
- Use the following command to install the
requests
library:
pip install requests
Writing Python Code to Import CSV Data
With your environment set up, you can now write the Python code to import CSV data using the CSV API. Follow these steps to create a script that uploads CSV data to your test account.
import requests
# Define the API endpoint and headers
url = "https://api.csvintegration.com/upload"
headers = {
"Authorization": "Bearer Your_API_Key",
"Content-Type": "multipart/form-data"
}
# Open the CSV file you want to upload
with open("sample.csv", "rb") as file:
# Define the files parameter for the request
files = {"file": file}
# Make a POST request to upload the CSV file
response = requests.post(url, headers=headers, files=files)
# Check if the request was successful
if response.status_code == 200:
print("CSV file uploaded successfully.")
else:
print(f"Failed to upload CSV file. Status code: {response.status_code}")
Replace Your_API_Key
with the API key you generated earlier. This script uploads a CSV file named sample.csv
to the CSV API. Ensure the file path is correct and accessible from your script's location.
Verifying Successful CSV Data Import
After running the script, you should verify that the CSV data was imported successfully into your test environment. Check the CSV API dashboard to confirm that the data appears as expected. If the data is not visible, review the error messages and adjust your code accordingly.
Handling Errors and Troubleshooting CSV API Calls
When working with API calls, it's crucial to handle potential errors gracefully. The CSV API may return various status codes indicating success or failure. Here are some common error codes and their meanings:
- 400 Bad Request: The request was malformed. Check your request parameters and data format.
- 401 Unauthorized: Authentication failed. Verify your API key and credentials.
- 500 Internal Server Error: The server encountered an error. Try again later or contact support.
Implement error handling in your code to manage these scenarios and ensure a robust integration.
Conclusion and Best Practices for CSV API Integration in Python
Integrating with the CSV API in Python offers a streamlined approach to managing data imports, enhancing efficiency and reducing errors in data handling. By following the steps outlined in this guide, developers can effectively automate the import process, ensuring data integrity and consistency.
Best Practices for Secure and Efficient CSV API Integration
- Secure Storage of API Credentials: Always store your API keys and credentials securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the CSV API. Implement retry logic and exponential backoff strategies to handle rate limit errors gracefully.
- Data Validation and Transformation: Validate and transform your CSV data before importing it to ensure it meets the required format and standards. This can prevent errors and improve data quality.
- Error Handling: Implement robust error handling to manage API call failures. Log errors for troubleshooting and provide meaningful feedback to users.
Enhancing Your Integration Strategy with Endgrate
While integrating with the CSV API directly can be effective, leveraging a tool like Endgrate can further simplify the process. Endgrate allows you to manage multiple integrations through a single API endpoint, saving time and resources. By outsourcing integrations to Endgrate, you can focus on your core product development and deliver a seamless integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of a unified integration platform.
Read More
Ready to get started?