How to Create Or Update Contacts with the Front API in PHP
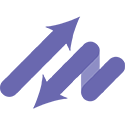
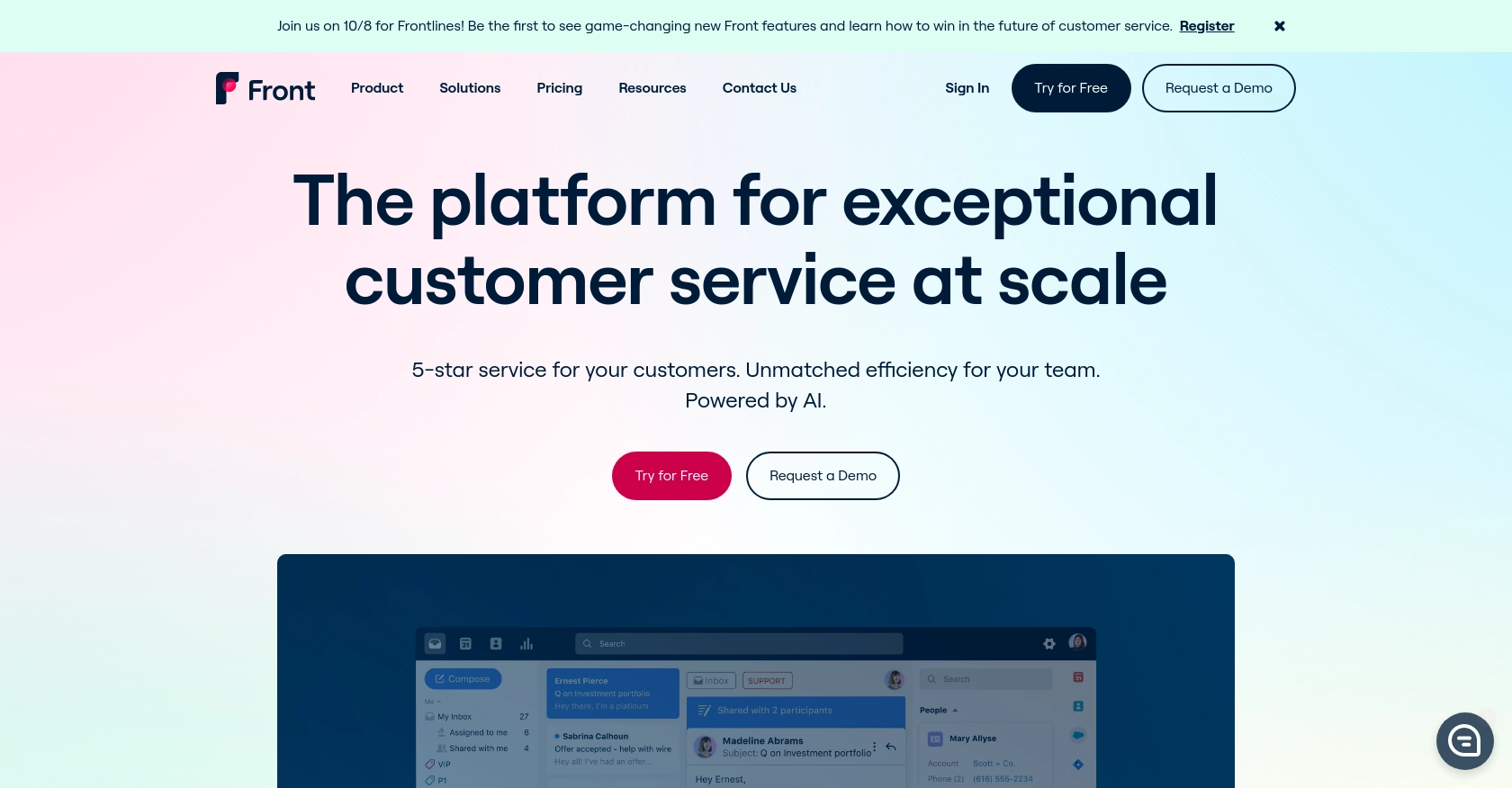
Introduction to Front API for Contact Management
Front is a powerful communication platform that centralizes emails, messages, and other communication channels into a single collaborative space. It is widely used by businesses to enhance team collaboration and streamline customer interactions.
Developers may want to integrate with Front's API to automate and manage contact information efficiently. For example, using the Front API, a developer can create or update contact details directly from a CRM system, ensuring that the contact information is always up-to-date and accessible to the team.
This article will guide you through the process of creating or updating contacts using the Front API with PHP, providing step-by-step instructions and code examples to help you seamlessly integrate Front's contact management capabilities into your application.
Setting Up Your Front Developer Account and API Access
Before you can start integrating with the Front API, you'll need to set up a developer account. This account will allow you to test your integration in a safe environment without affecting production data.
Creating a Front Developer Account
If you don't already have a Front account, you can create a developer account by signing up on the Front website. This account provides access to a free developer environment, which includes all the tools you need to begin building with Front's APIs.
- Visit the Front Developer Portal.
- Follow the instructions to sign up for a developer account.
- Once your account is created, you'll have access to the developer dashboard.
Generating an API Token for Front API Authentication
Front uses API tokens for authentication, allowing you to securely access the API. Follow these steps to generate your API token:
- Log in to your Front developer account.
- Navigate to the API tokens section in your account settings.
- Click on "Create API Token" and select the appropriate scopes for your integration.
- Copy the generated API token and store it securely, as you'll need it to authenticate your API requests.
For more detailed instructions, refer to the Front API documentation.
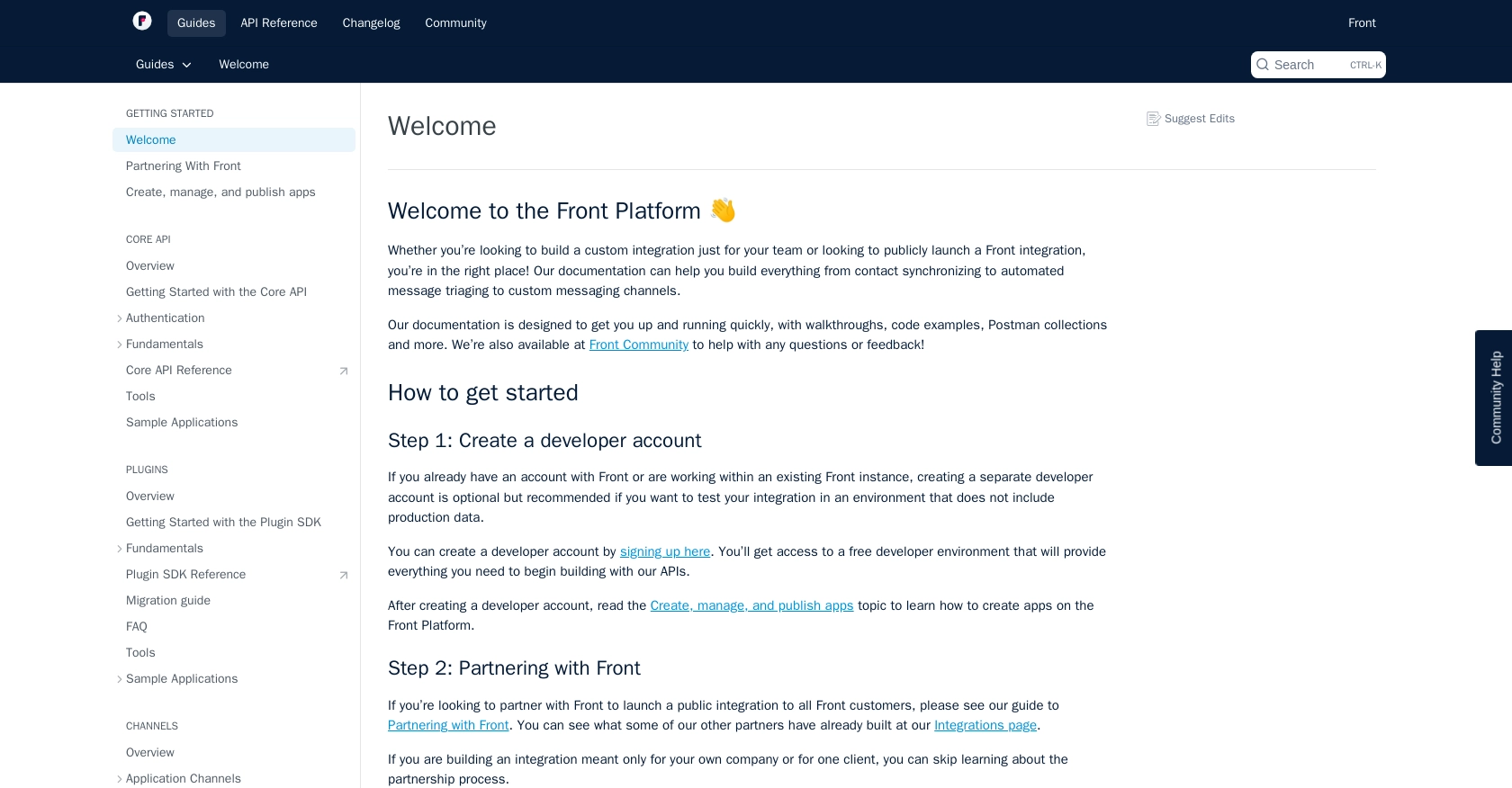
sbb-itb-96038d7
Making API Calls to Create or Update Contacts with Front API in PHP
To interact with the Front API for creating or updating contacts, you'll need to use PHP to make HTTP requests. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code to make API calls, and handling responses and errors effectively.
Setting Up Your PHP Environment for Front API Integration
Before making API calls, ensure you have the following prerequisites:
- PHP 7.4 or higher installed on your machine.
- Composer for managing dependencies.
- The
guzzlehttp/guzzle
library for making HTTP requests. Install it using Composer:
composer require guzzlehttp/guzzle
Creating a Contact with Front API Using PHP
To create a contact, you'll need to send a POST request to the Front API's contact endpoint. Here's a sample code snippet to guide you:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiToken = 'YOUR_API_TOKEN';
$response = $client->post('https://api2.frontapp.com/contacts', [
'headers' => [
'Authorization' => 'Bearer ' . $apiToken,
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'json' => [
'name' => 'John Doe',
'description' => 'Sample contact description',
'handles' => [
[
'source' => 'email',
'handle' => 'john.doe@example.com'
]
]
]
]);
if ($response->getStatusCode() === 201) {
echo "Contact created successfully!";
} else {
echo "Failed to create contact.";
}
Replace YOUR_API_TOKEN
with the API token you generated earlier. This code sends a request to create a contact named "John Doe" with an email handle.
Updating a Contact with Front API Using PHP
To update an existing contact, use the PATCH method. Here's how you can do it:
$contactId = 'CONTACT_ID'; // Replace with the actual contact ID
$response = $client->patch("https://api2.frontapp.com/contacts/{$contactId}", [
'headers' => [
'Authorization' => 'Bearer ' . $apiToken,
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'json' => [
'custom_fields' => [
'Job Title' => 'Software Engineer',
'Location' => 'New York'
]
]
]);
if ($response->getStatusCode() === 204) {
echo "Contact updated successfully!";
} else {
echo "Failed to update contact.";
}
Ensure you replace CONTACT_ID
with the actual ID of the contact you wish to update. This example updates the contact's job title and location.
Handling API Responses and Errors
It's crucial to handle API responses and errors gracefully. Check the status code of the response to determine if the request was successful. Common status codes include:
- 201 Created: The contact was successfully created.
- 204 No Content: The contact was successfully updated.
- 400 Bad Request: The request was invalid. Check your input data.
- 401 Unauthorized: Authentication failed. Verify your API token.
- 429 Too Many Requests: Rate limit exceeded. Refer to the rate limiting documentation for more details.
Implement error handling in your code to manage these scenarios effectively and ensure a robust integration.
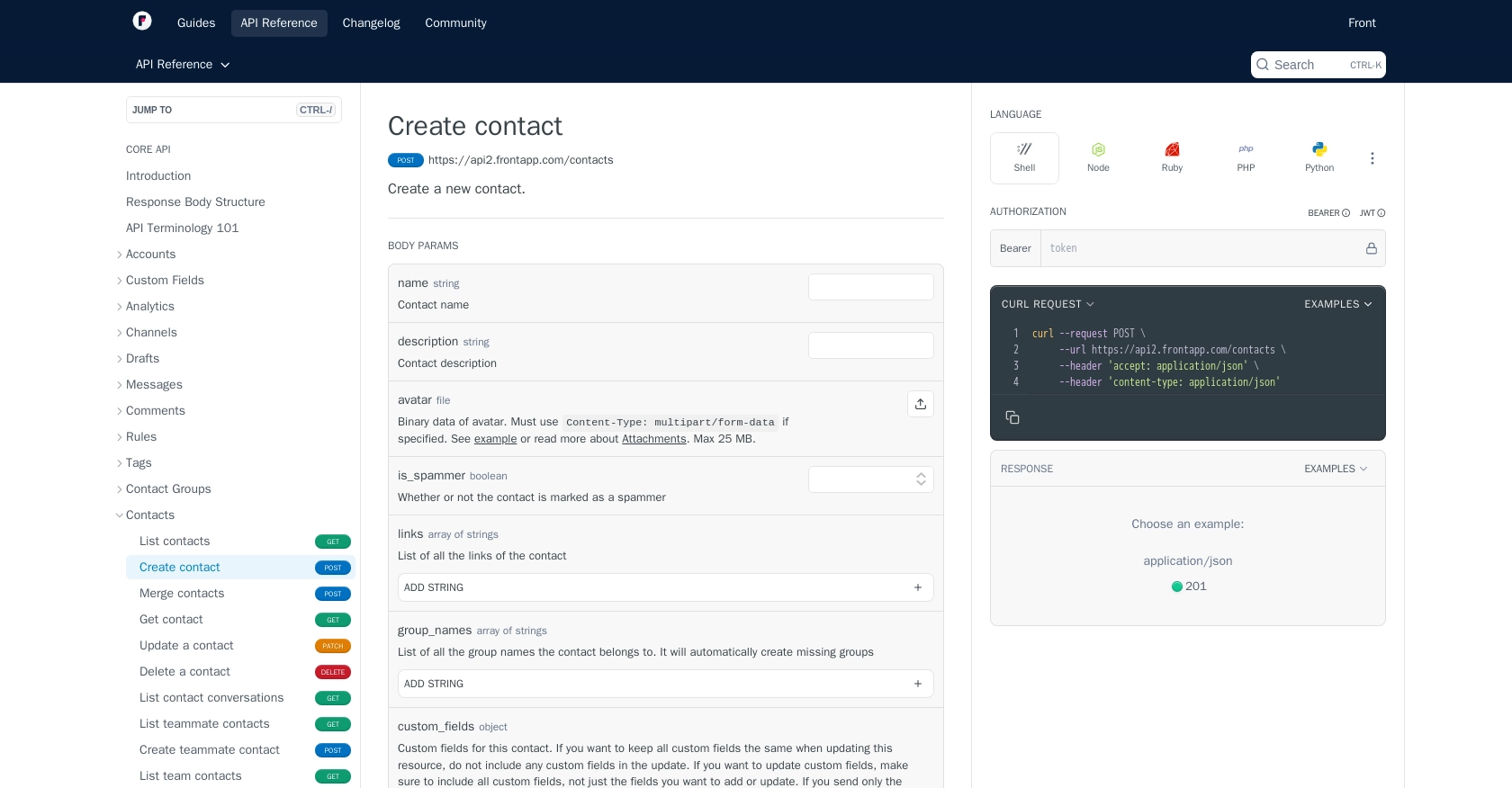
Best Practices for Using Front API in PHP
When integrating with the Front API, it's essential to follow best practices to ensure a secure and efficient implementation. Here are some recommendations:
- Securely Store API Tokens: Always store your API tokens securely and avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Implement Rate Limiting: Be mindful of Front's rate limits, which start at 50 requests per minute. Implement logic to handle
429 Too Many Requests
responses by waiting and retrying after the specified time. Refer to the rate limiting documentation for more information. - Data Standardization: Ensure that the data you send to Front is standardized and consistent. This includes formatting names, email addresses, and other contact details to maintain data integrity.
- Error Handling: Implement comprehensive error handling to manage different response codes and scenarios. This will help in maintaining a robust integration and provide better user experience.
Leveraging Endgrate for Seamless Integrations
Building and maintaining integrations can be time-consuming and complex. With Endgrate, you can simplify this process by leveraging a unified API endpoint that connects to multiple platforms, including Front. Here’s how Endgrate can benefit your integration efforts:
- Focus on Core Product: Save time and resources by outsourcing integrations, allowing your team to focus on enhancing your core product.
- Build Once, Use Multiple Times: Create integrations for specific use cases and reuse them across different platforms, reducing development overhead.
- Enhanced Customer Experience: Provide your customers with an easy and intuitive integration experience, improving satisfaction and engagement.
Explore how Endgrate can streamline your integration process by visiting Endgrate.
Read More
- https://endgrate.com/provider/front
- https://dev.frontapp.com/docs/welcome
- https://dev.frontapp.com/docs/core-api-getting-started
- https://dev.frontapp.com/docs/oauth
- https://dev.frontapp.com/docs/rate-limiting
- https://dev.frontapp.com/docs/pagination
- https://dev.frontapp.com/reference/create-contact
- https://dev.frontapp.com/reference/update-a-contact
Ready to get started?