Using the Sage Accounting API to Create or Update Customers in Python
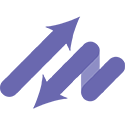
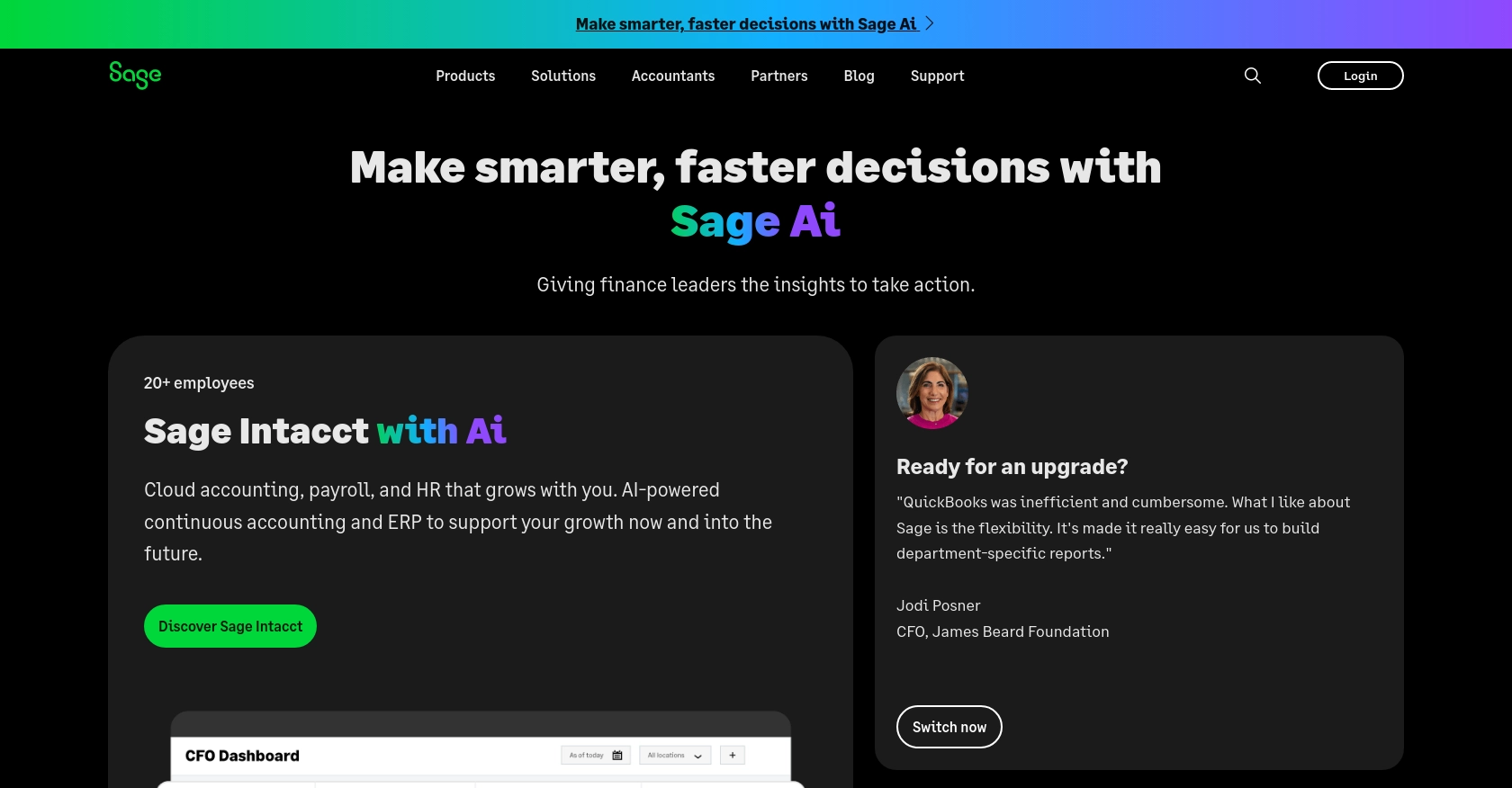
Introduction to Sage Accounting API
Sage Accounting is a robust cloud-based accounting solution tailored for small and medium-sized businesses. It offers a comprehensive suite of tools to manage financial transactions, invoicing, and reporting, making it an essential platform for businesses aiming to streamline their accounting processes.
Integrating with the Sage Accounting API allows developers to automate and enhance business operations by interacting directly with financial data. For example, developers can use the API to create or update customer records, ensuring that client information is always up-to-date and accurate. This capability is particularly useful for businesses that need to synchronize customer data across multiple systems, reducing manual entry and minimizing errors.
Setting Up a Sage Accounting Test or Sandbox Account
Before you can start integrating with the Sage Accounting API, you'll need to set up a test or sandbox account. This environment allows you to safely develop and test your integration without affecting live data.
Create a Sage Developer Account
To begin, you'll need a Sage Developer account. This account will enable you to register and manage your applications, obtain client credentials, and specify essential details such as callback URLs.
- Visit the Sage Developer Portal and sign up using your GitHub account or an email address.
- Follow the guide on signing up to ensure you have all necessary information ready.
Register a Trial Business Account
Next, set up a trial business account for development purposes. Sage offers trial accounts for various regions and subscription tiers, allowing you to test different functionalities.
- Choose the appropriate region and subscription tier from the Sage Developer Portal.
- Sign up for the trial account using your preferred email service. Consider using email aliasing to manage multiple environments efficiently.
Create a Sage Accounting App for OAuth Authentication
Since the Sage Accounting API uses OAuth for authentication, you'll need to create an app to obtain the necessary credentials.
- Log in to your Sage Developer account and navigate to the Create an App section.
- Click on "Create App" and enter a name and callback URL for your app. Optionally, provide an alternative email address and homepage URL.
- Save the app to generate your Client ID and Client Secret, which you'll use for authentication.
Upgrade to a Developer Account
To fully utilize the Sage Accounting API, upgrade your trial account to a developer account. This upgrade provides 12 months of free access for testing your integration.
- Submit a request through the Upgrade Your Account page, providing details such as your name, email, app name, and Client ID.
- Wait for confirmation from Sage, which typically takes 3-5 working days.
Once you've completed these steps, you'll be ready to start developing and testing your integration with the Sage Accounting API.
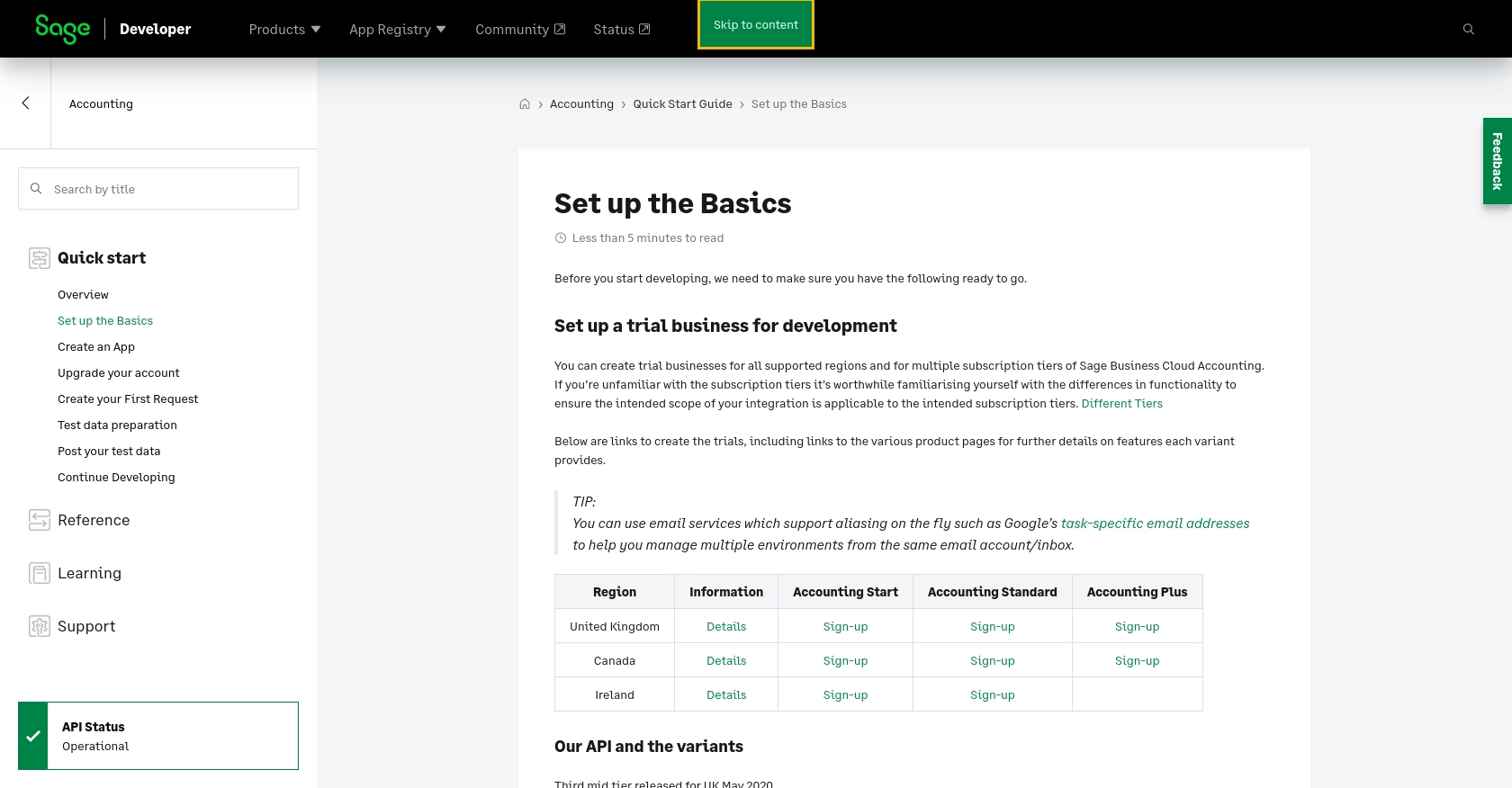
sbb-itb-96038d7
Making API Calls to Sage Accounting for Customer Management in Python
To interact with the Sage Accounting API for creating or updating customer records, you'll need to set up your Python environment and write the necessary code to make API calls. This section will guide you through the process, ensuring you can efficiently manage customer data using Python.
Setting Up Your Python Environment for Sage Accounting API
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Once these are installed, use the following command to install the requests
library, which is essential for making HTTP requests:
pip install requests
Creating or Updating Customers with the Sage Accounting API
Now that your environment is set up, you can proceed to create or update customer records using the Sage Accounting API. Below is a step-by-step guide with example code.
Example Code for Creating a Customer
Create a file named create_customer.py
and add the following code:
import requests
# Define the API endpoint and headers
url = "https://api.sage.com/accounting/contacts"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json"
}
# Define the customer data
customer_data = {
"contact": {
"name": "John Doe",
"email": "johndoe@example.com",
"telephone": "123-456-7890"
}
}
# Make a POST request to create a customer
response = requests.post(url, json=customer_data, headers=headers)
# Check the response status
if response.status_code == 201:
print("Customer created successfully.")
else:
print(f"Failed to create customer: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the token obtained during the OAuth authentication setup. This code sends a POST request to the Sage Accounting API to create a new customer. If successful, it prints a confirmation message.
Example Code for Updating a Customer
To update an existing customer, modify the code as follows:
import requests
# Define the API endpoint and headers
customer_id = "existing_customer_id"
url = f"https://api.sage.com/accounting/contacts/{customer_id}"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json"
}
# Define the updated customer data
updated_data = {
"contact": {
"name": "John Doe",
"email": "john.doe@newexample.com",
"telephone": "987-654-3210"
}
}
# Make a PUT request to update the customer
response = requests.put(url, json=updated_data, headers=headers)
# Check the response status
if response.status_code == 200:
print("Customer updated successfully.")
else:
print(f"Failed to update customer: {response.status_code} - {response.text}")
Replace existing_customer_id
with the ID of the customer you wish to update. This code sends a PUT request to update the customer's details.
Verifying API Call Success and Handling Errors
After making an API call, it's crucial to verify its success by checking the response status code. A status code of 201 indicates successful creation, while 200 indicates a successful update. If the request fails, the response will contain an error code and message, which you should log for troubleshooting.
For more detailed error handling, refer to the Sage Accounting API documentation for a list of possible error codes and their meanings.
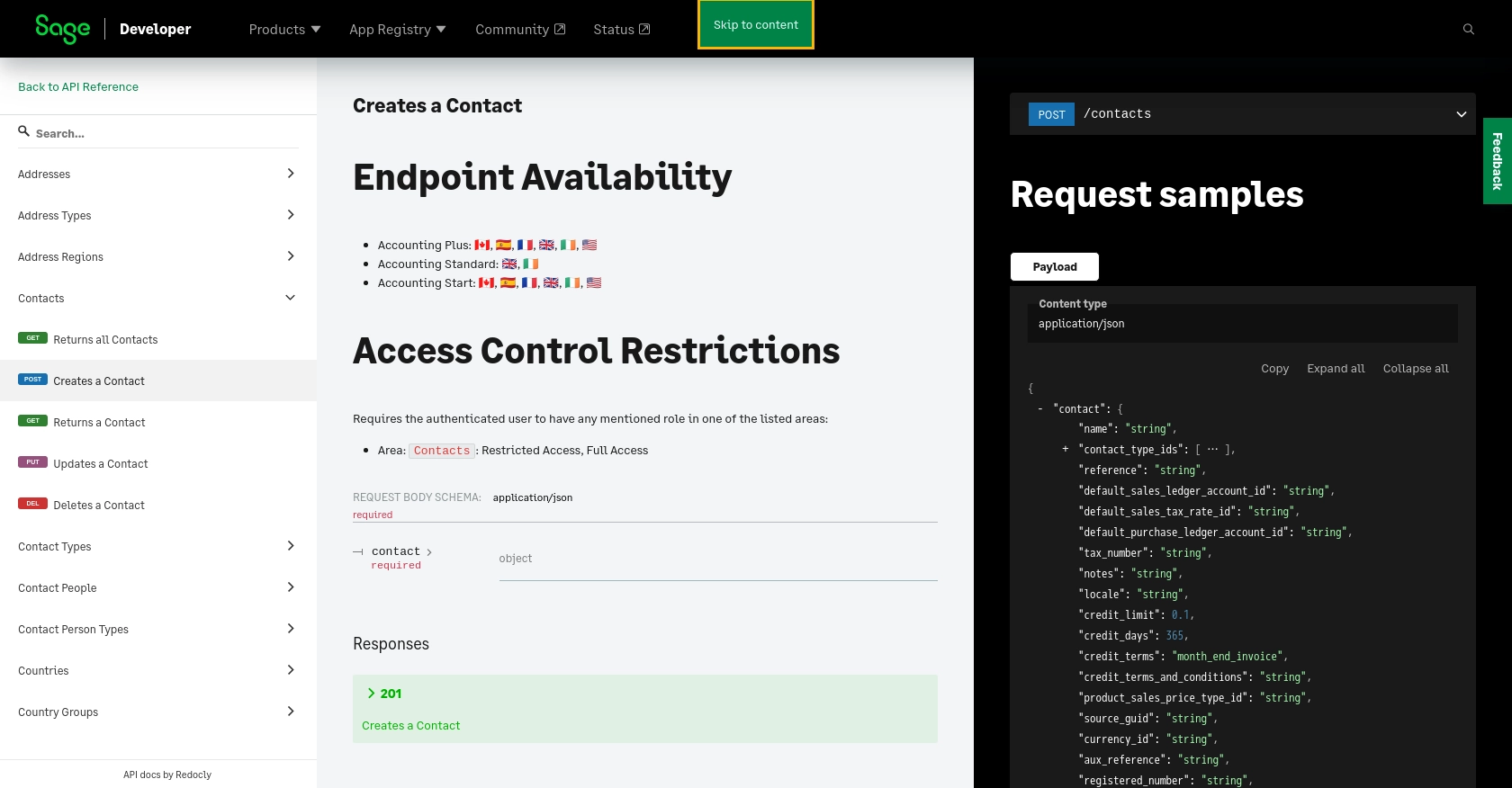
Conclusion and Best Practices for Using Sage Accounting API in Python
Integrating with the Sage Accounting API provides developers with powerful tools to automate and streamline financial operations. By creating or updating customer records programmatically, businesses can ensure data accuracy and reduce manual entry errors, enhancing overall efficiency.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth credentials, such as the Client ID and Client Secret, securely. Consider using environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to manage requests efficiently.
- Standardize Data Fields: Ensure that data fields are consistent across different systems to facilitate seamless integration and data synchronization.
- Log and Monitor API Calls: Implement logging to track API requests and responses. This will help in troubleshooting and improving the integration over time.
Enhance Your Integration Strategy with Endgrate
While integrating with Sage Accounting API can significantly improve your business processes, managing multiple integrations can be challenging. Endgrate offers a unified API solution that simplifies integration management across various platforms, including Sage Accounting.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. Build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate today.
Read More
- https://endgrate.com/provider/sage
- https://developer.sage.com/accounting/quick-start/set-up-the-basics/
- https://developer.sage.com/accounting/quick-start/create-an-app/
- https://developer.sage.com/accounting/quick-start/upgrade-your-account/
- https://developer.sage.com/accounting/reference/contacts/#tag/Contacts/operation/postContacts
Ready to get started?