How to Get Tax Rates with the Quickbooks API in PHP
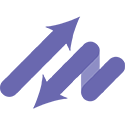
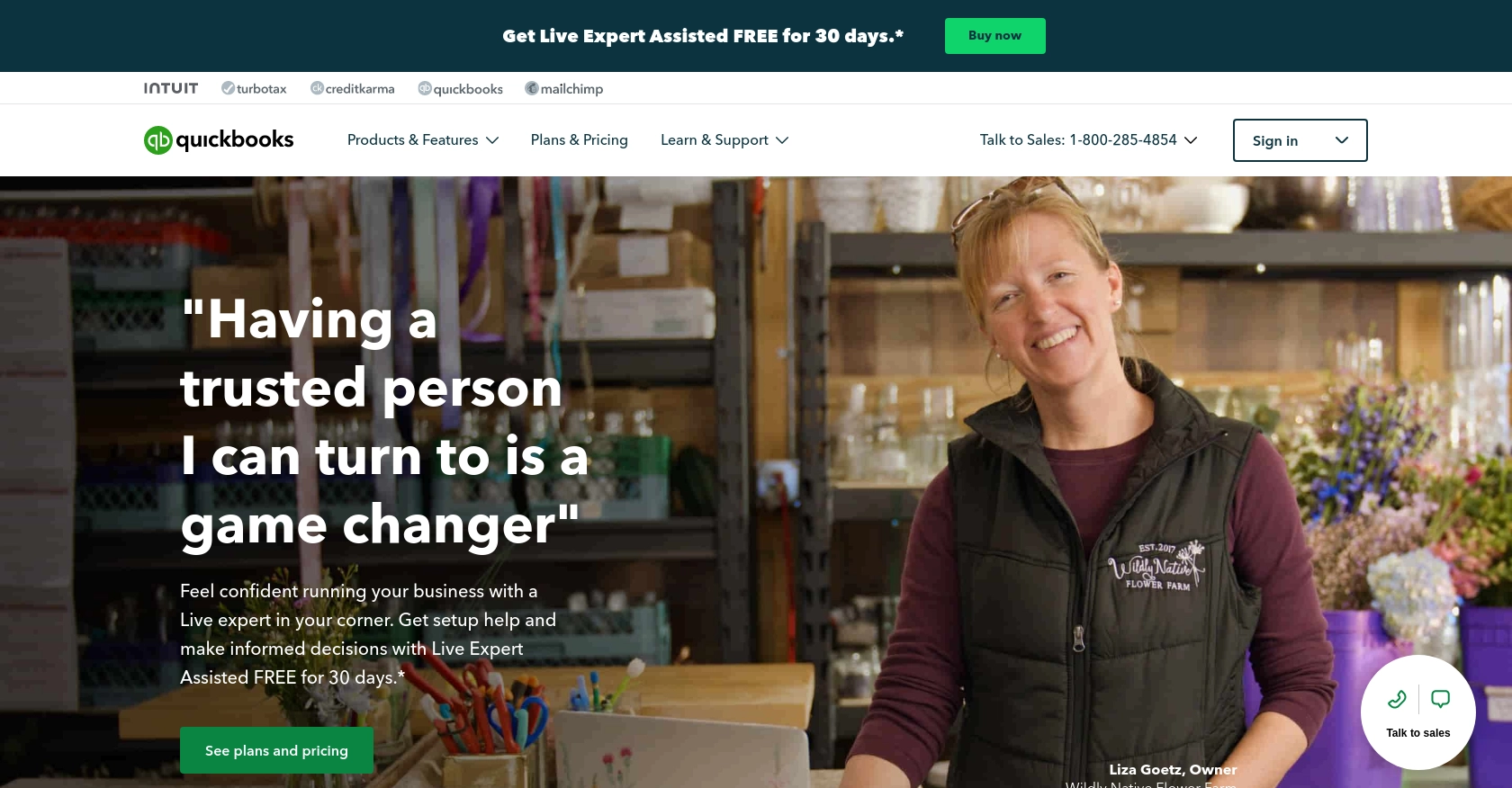
Introduction to QuickBooks API
QuickBooks is a widely-used accounting software that offers a comprehensive suite of tools for managing financial tasks, including invoicing, payroll, and tax calculations. Its robust features and user-friendly interface make it a popular choice for businesses of all sizes looking to streamline their financial operations.
For developers, integrating with the QuickBooks API can provide powerful capabilities to automate and enhance financial workflows. One common use case is retrieving tax rates, which can be essential for applications that need to calculate taxes accurately for transactions. By accessing tax rate data through the QuickBooks API, developers can ensure their applications remain compliant with the latest tax regulations and provide accurate financial reporting.
Setting Up Your QuickBooks Sandbox Account for API Integration
Before you can start retrieving tax rates using the QuickBooks API, you'll need to set up a QuickBooks sandbox account. This sandbox environment allows developers to test their applications without affecting live data, ensuring a safe and effective development process.
Creating a QuickBooks Developer Account
To begin, you'll need to create a QuickBooks Developer account. Follow these steps:
- Visit the QuickBooks Developer Portal.
- Sign up for a new account or log in if you already have one.
- Once logged in, navigate to the dashboard to start creating your app.
Generating OAuth Credentials for QuickBooks API
QuickBooks uses OAuth 2.0 for authentication. You'll need to generate a client ID and client secret to authenticate your API requests:
- Go to the App Management section.
- Create a new app by providing the necessary details.
- Once the app is created, navigate to the "Keys & OAuth" section to find your client ID and client secret.
- Make sure to save these credentials securely, as you'll need them to authenticate API requests.
Configuring App Settings for QuickBooks API Access
After obtaining your OAuth credentials, configure your app settings to ensure proper access:
- In the app settings, specify the scopes required for accessing tax rates. This typically includes read permissions for tax data.
- Ensure that your redirect URI is set correctly to handle OAuth callbacks.
- Review and save your app settings.
With your QuickBooks sandbox account and OAuth credentials set up, you're ready to start making API calls to retrieve tax rates. For more detailed information, refer to the official QuickBooks API documentation.
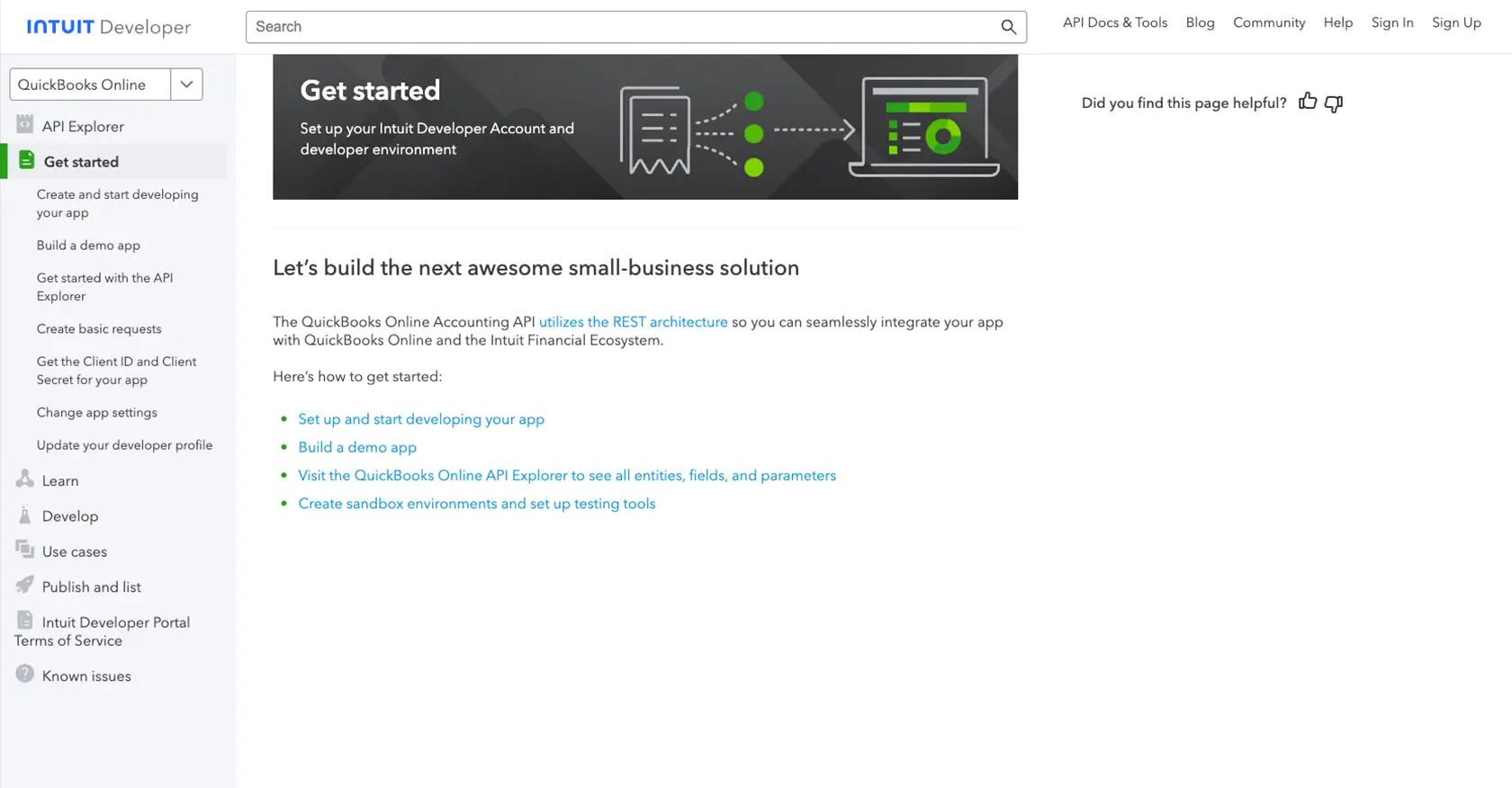
sbb-itb-96038d7
Making API Calls to Retrieve Tax Rates with QuickBooks API in PHP
To retrieve tax rates using the QuickBooks API in PHP, you'll need to set up your development environment and write the necessary code to interact with the API. This section will guide you through the process, including setting up PHP, installing dependencies, and writing the code to make the API call.
Setting Up Your PHP Environment for QuickBooks API Integration
Before you begin, ensure that you have the following installed on your machine:
- PHP 7.4 or later
- Composer, the PHP package manager
Once you have these prerequisites, you can proceed to install the necessary dependencies for making HTTP requests.
Installing Required PHP Libraries for QuickBooks API
To interact with the QuickBooks API, you'll need the Guzzle HTTP client, which simplifies making HTTP requests in PHP. Install it using Composer with the following command:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Tax Rates from QuickBooks API
With your environment set up, you can now write the PHP code to make the API call. Create a file named get_tax_rates.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token'; // Replace with your OAuth access token
$realmId = 'Your_Realm_ID'; // Replace with your QuickBooks company ID
$url = "https://quickbooks.api.intuit.com/v3/company/$realmId/query?query=SELECT * FROM TaxRate";
$response = $client->request('GET', $url, [
'headers' => [
'Authorization' => "Bearer $accessToken",
'Accept' => 'application/json',
],
]);
if ($response->getStatusCode() == 200) {
$data = json_decode($response->getBody(), true);
foreach ($data['QueryResponse']['TaxRate'] as $taxRate) {
echo "Tax Rate Name: " . $taxRate['Name'] . "\n";
echo "Rate: " . $taxRate['RateValue'] . "%\n\n";
}
} else {
echo "Failed to retrieve tax rates. Status code: " . $response->getStatusCode();
}
?>
Replace Your_Access_Token
and Your_Realm_ID
with your actual OAuth access token and QuickBooks company ID, respectively.
Running the PHP Script and Verifying API Response
Run the script from the command line using the following command:
php get_tax_rates.php
If successful, the script will output the tax rates retrieved from QuickBooks. You can verify the results by checking the tax rates in your QuickBooks sandbox account.
Handling Errors and QuickBooks API Response Codes
It's important to handle potential errors when making API calls. The QuickBooks API may return various HTTP status codes to indicate success or failure:
- 200 OK: The request was successful, and the tax rates are returned.
- 401 Unauthorized: Authentication failed. Check your access token.
- 403 Forbidden: You do not have permission to access the resource.
- 500 Internal Server Error: An error occurred on the server. Try again later.
Ensure that you handle these errors appropriately in your application to provide a smooth user experience.
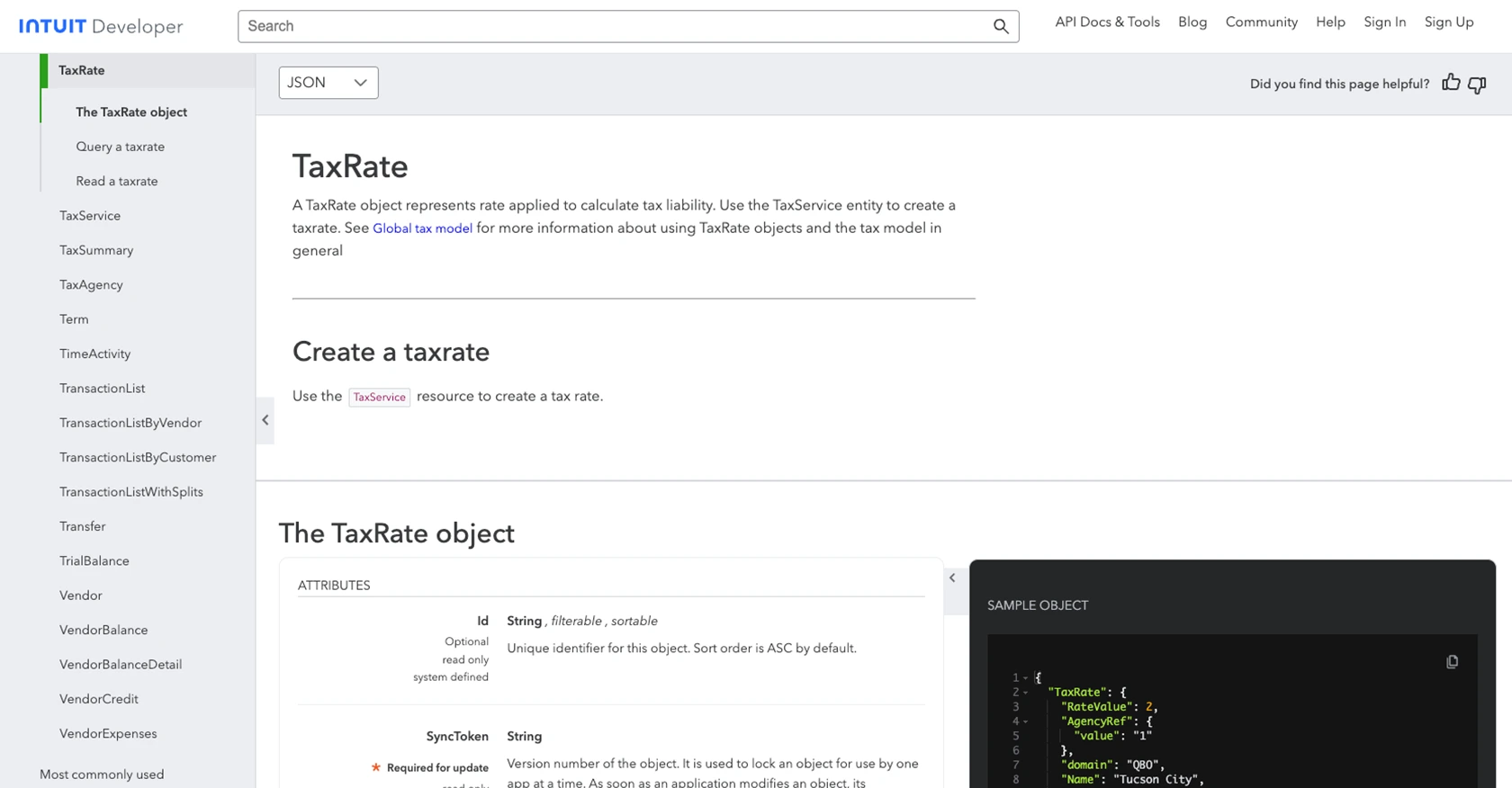
Best Practices for QuickBooks API Integration and Error Handling
When working with the QuickBooks API, it's crucial to follow best practices to ensure a secure and efficient integration. Here are some key recommendations:
- Securely Store Credentials: Always store your OAuth credentials, such as client ID and client secret, securely. Use environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: QuickBooks API has rate limits to prevent abuse. Monitor the response headers for rate limit information and implement retry logic with exponential backoff to handle rate limits gracefully.
- Standardize Data Fields: Ensure that the data retrieved from QuickBooks is standardized and transformed as needed to fit your application's requirements.
- Implement Robust Error Handling: Handle various HTTP status codes returned by the API to provide meaningful feedback to users and ensure a smooth experience.
Enhance Your Integration Experience with Endgrate
Integrating with multiple platforms can be complex and time-consuming. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including QuickBooks. With Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations.
- Provide an easy, intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts and enhance your application's capabilities by visiting Endgrate.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/all-entities/taxrate
Ready to get started?