Using the Copper API to Get Leads in Javascript
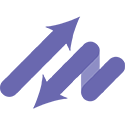
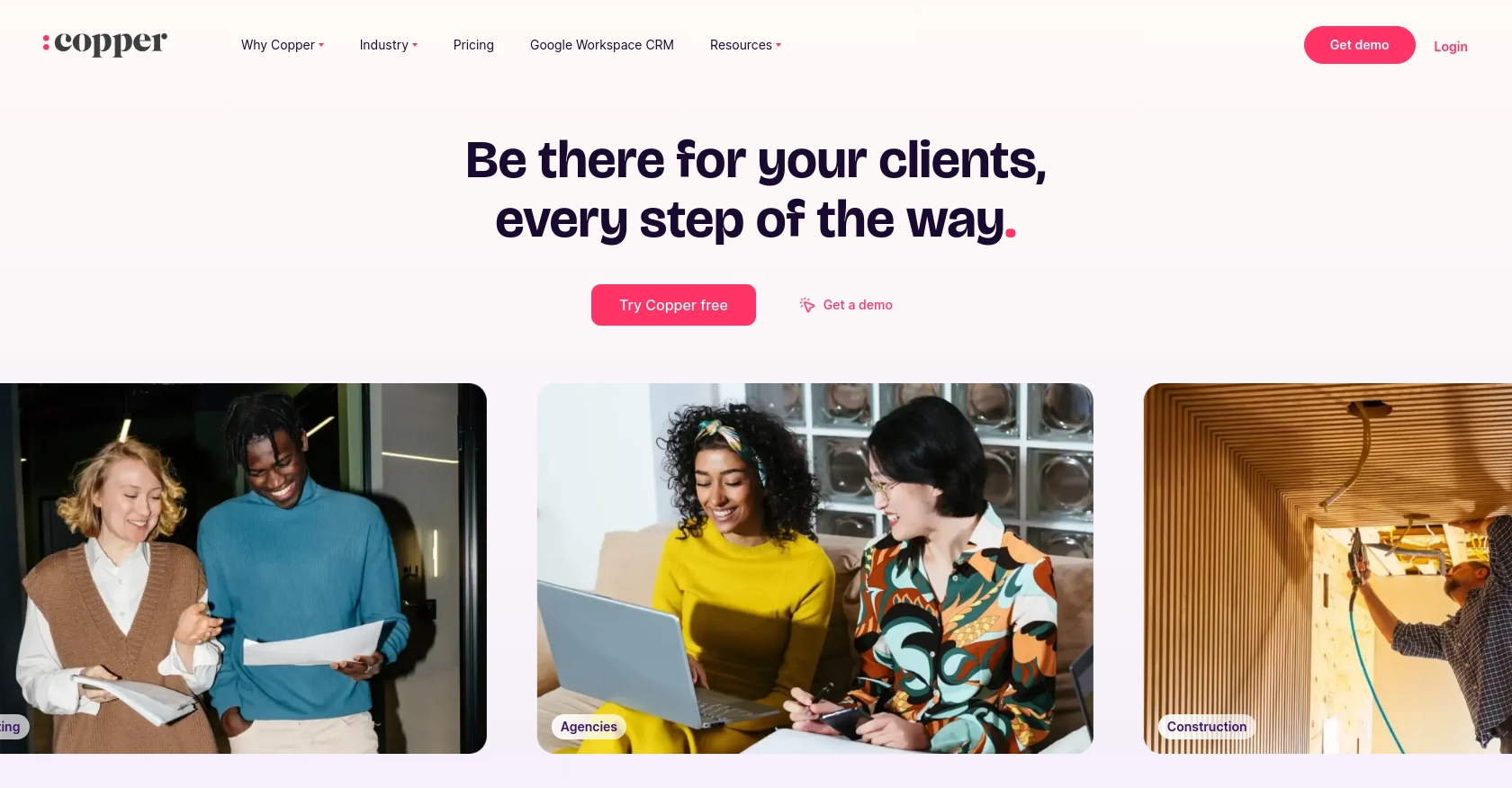
Introduction to Copper API for Lead Management
Copper is a powerful CRM platform designed to seamlessly integrate with Google Workspace, providing businesses with an efficient way to manage customer relationships and streamline sales processes. With its intuitive interface and robust features, Copper is a popular choice for companies looking to enhance their CRM capabilities.
Developers may want to integrate with Copper's API to automate and optimize lead management tasks. For example, using the Copper API, you can retrieve and manage leads directly from your application, enabling you to create a more personalized and efficient sales process.
This article will guide you through using JavaScript to interact with the Copper API, focusing on retrieving leads to enhance your sales workflow.
Setting Up Your Copper API Test Account
Before you can start interacting with the Copper API using JavaScript, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data.
Creating a Copper Account
If you don't already have a Copper account, you can sign up for a free trial on the Copper website. This will give you access to the platform's features and allow you to explore its capabilities.
Generating Copper API Key and Secret
To authenticate your API requests, you'll need an API key and secret. Follow these steps to generate them:
- Log in to your Copper account.
- Navigate to the Settings section.
- Under Integrations, find the API Keys option.
- Click on Create API Key and fill in the required details.
- Once created, you'll receive an API key and secret. Make sure to store these securely, as they will be used to authenticate your API requests.
Configuring OAuth for Copper API
Since Copper uses a custom authentication method, ensure you configure OAuth for secure access:
- In the API Keys section, set up OAuth by specifying the redirect URI for your application.
- Ensure that your application can handle the OAuth callback to receive the authorization code.
- Exchange the authorization code for an access token using Copper's token endpoint.
Testing Your Copper API Setup
With your API key, secret, and OAuth configured, you can now test your setup:
// Example JavaScript code to test Copper API authentication
const axios = require('axios');
const apiKey = 'YOUR_API_KEY';
const apiSecret = 'YOUR_API_SECRET';
const accessToken = 'YOUR_ACCESS_TOKEN';
axios.get('https://api.copper.com/v1/leads', {
headers: {
'Authorization': `Bearer ${accessToken}`,
'X-Api-Key': apiKey
}
})
.then(response => {
console.log('Leads:', response.data);
})
.catch(error => {
console.error('Error fetching leads:', error);
});
Replace YOUR_API_KEY
, YOUR_API_SECRET
, and YOUR_ACCESS_TOKEN
with your actual credentials. This code snippet demonstrates how to authenticate and make a request to retrieve leads from Copper.
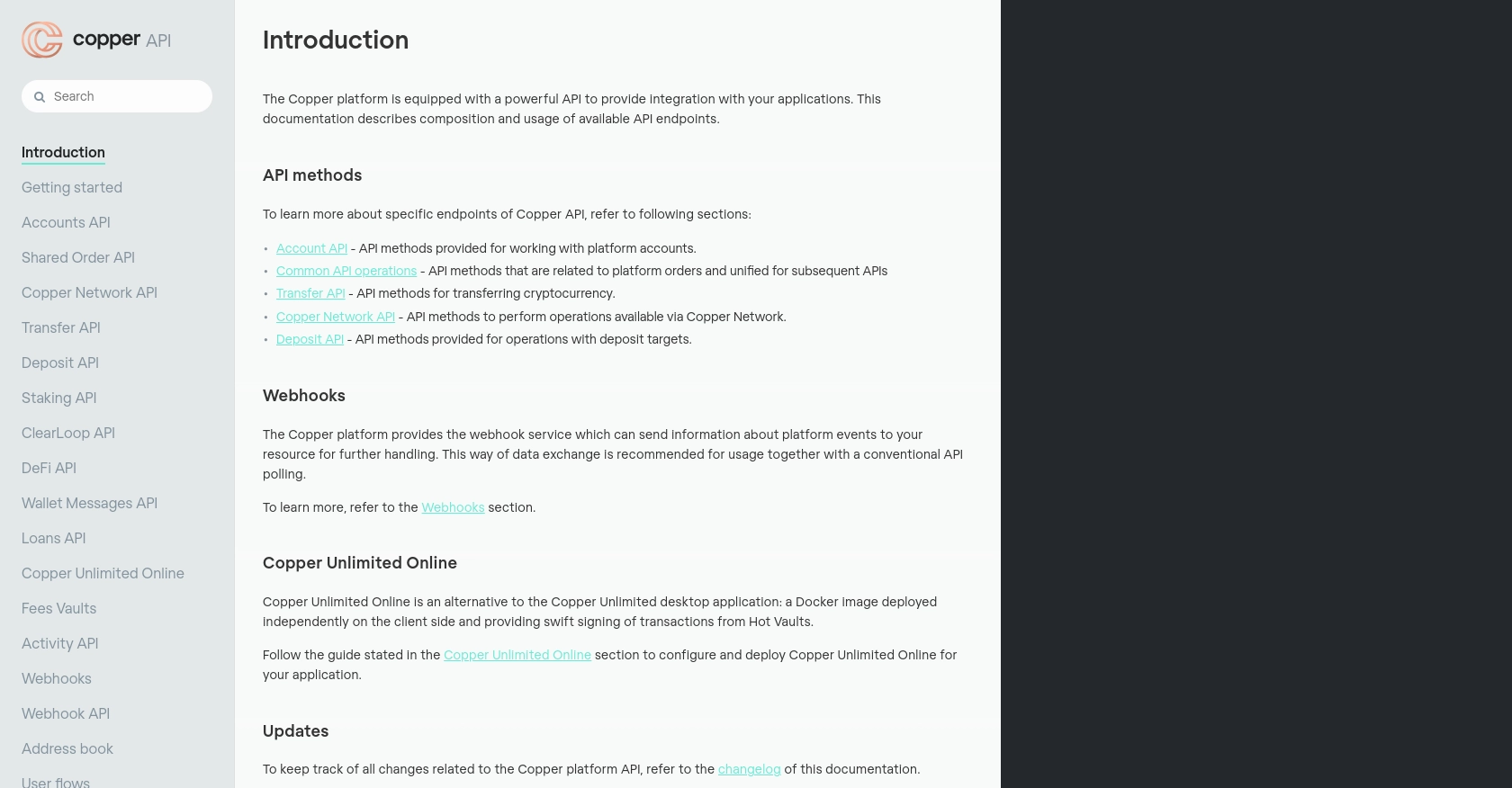
sbb-itb-96038d7
Making API Calls to Retrieve Leads from Copper Using JavaScript
To effectively interact with the Copper API and retrieve leads using JavaScript, you'll need to ensure your environment is properly set up. This section will guide you through the necessary steps, including setting up your JavaScript environment, installing dependencies, and executing API calls.
Setting Up Your JavaScript Environment for Copper API Integration
Before making API calls, ensure you have Node.js installed on your machine. You can download it from the official Node.js website. Once installed, you can use npm (Node Package Manager) to manage your project dependencies.
Installing Required Dependencies for Copper API
For this tutorial, we'll use the axios
library to handle HTTP requests. Install it by running the following command in your terminal:
npm install axios
Writing JavaScript Code to Fetch Leads from Copper API
With your environment set up, you can now write the JavaScript code to make API calls to Copper. Below is an example of how to retrieve leads:
const axios = require('axios');
// Replace with your actual credentials
const apiKey = 'YOUR_API_KEY';
const apiSecret = 'YOUR_API_SECRET';
const accessToken = 'YOUR_ACCESS_TOKEN';
// Define the Copper API endpoint for leads
const copperApiUrl = 'https://api.copper.com/v1/leads';
// Function to fetch leads
async function fetchLeads() {
try {
const response = await axios.get(copperApiUrl, {
headers: {
'Authorization': `Bearer ${accessToken}`,
'X-Api-Key': apiKey
}
});
console.log('Leads:', response.data);
} catch (error) {
console.error('Error fetching leads:', error);
}
}
// Execute the function
fetchLeads();
Ensure you replace YOUR_API_KEY
, YOUR_API_SECRET
, and YOUR_ACCESS_TOKEN
with your actual Copper API credentials. This script will authenticate and retrieve leads from your Copper account.
Verifying Successful API Requests and Handling Errors
After executing the script, you should see a list of leads printed in your console. If the request fails, the error message will be logged, helping you identify and resolve issues. Common errors include incorrect credentials or network issues.
Checking Lead Data in Copper Sandbox Account
To verify the leads retrieved, log in to your Copper sandbox account and navigate to the leads section. Ensure the data matches the output from your API call.
Handling Copper API Error Codes
When interacting with the Copper API, it's crucial to handle potential errors gracefully. Copper API may return various HTTP status codes, such as:
- 401 Unauthorized: Invalid API key or access token.
- 404 Not Found: Incorrect endpoint URL.
- 429 Too Many Requests: Rate limit exceeded.
Implement error handling in your code to manage these scenarios effectively.
Conclusion and Best Practices for Using Copper API with JavaScript
Integrating with the Copper API using JavaScript can significantly enhance your lead management processes by automating tasks and providing seamless access to customer data. By following the steps outlined in this guide, you can efficiently retrieve and manage leads, improving your sales workflow and overall CRM strategy.
Best Practices for Secure and Efficient Copper API Integration
- Secure Storage of Credentials: Always store your API key, secret, and access tokens securely. Consider using environment variables or secure vaults to protect sensitive information.
- Implement Rate Limiting: Be mindful of Copper's API rate limits to avoid exceeding them and receiving a 429 error. Implement retry logic with exponential backoff to handle rate limiting gracefully.
- Data Standardization: Ensure that the data retrieved from Copper is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage various HTTP status codes and provide meaningful feedback to users or logs for debugging.
Enhance Your Integration Strategy with Endgrate
For developers looking to streamline multiple integrations, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate provides a unified API endpoint that connects to various platforms, including Copper, offering an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can optimize your integration strategy and deliver seamless experiences to your users.
Read More
Ready to get started?