Using the Microsoft Dynamics 365 API to Create or Update Contact (with PHP examples)
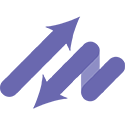
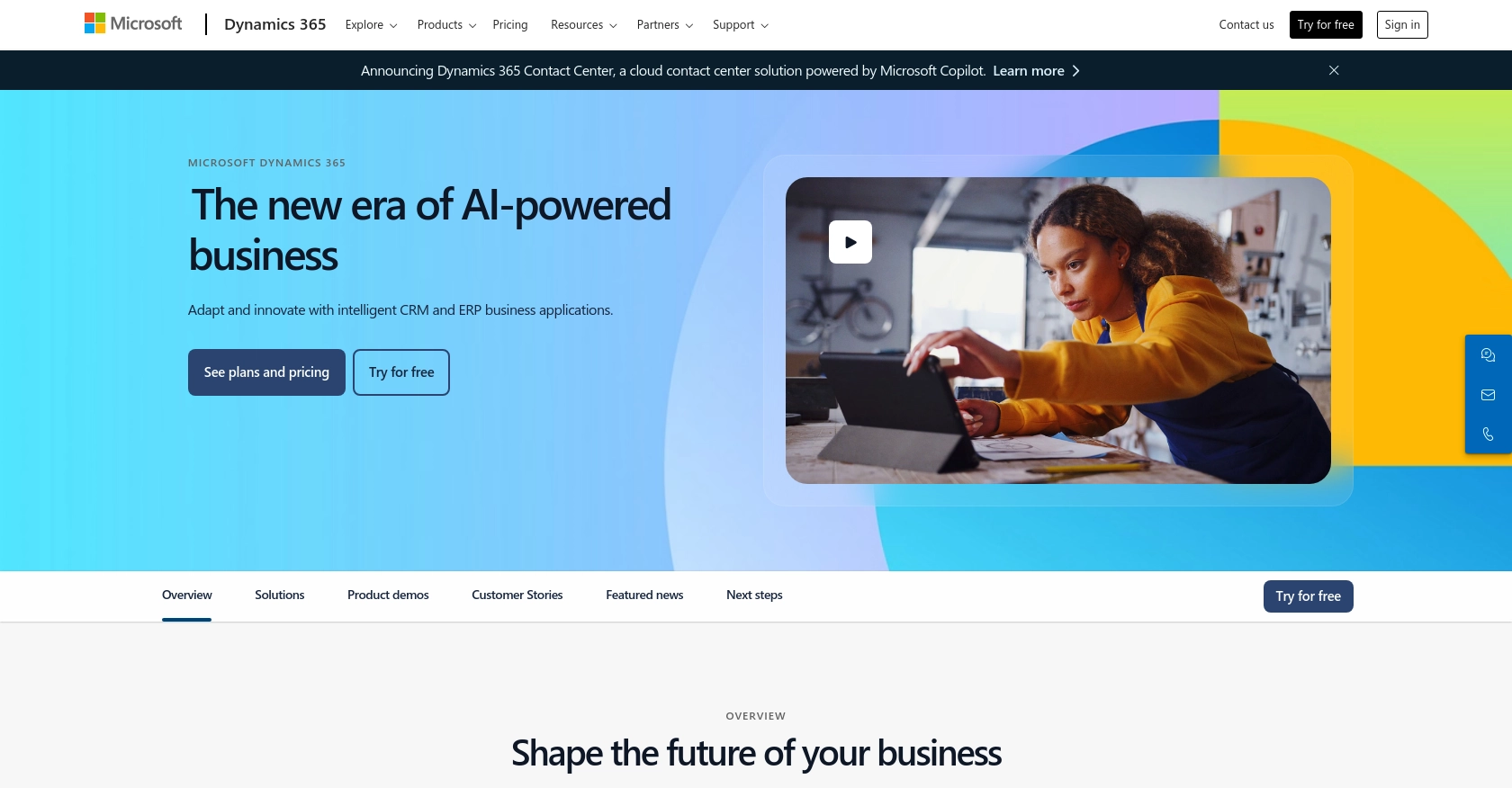
Introduction to Microsoft Dynamics 365 API
Microsoft Dynamics 365 is a comprehensive suite of business applications that seamlessly integrate CRM and ERP capabilities. It empowers organizations to drive digital transformation by connecting data, processes, and teams. With its robust API, developers can extend and customize Dynamics 365 to meet specific business needs.
Integrating with the Microsoft Dynamics 365 API allows developers to automate and streamline business processes, such as managing customer relationships and operational workflows. For example, a developer might use the API to create or update contact information, ensuring that customer data is always up-to-date and accessible across the organization.
In this article, we will explore how to use PHP to interact with the Microsoft Dynamics 365 API for creating or updating contact records. This guide will provide step-by-step instructions to help developers efficiently manage contact data within Dynamics 365.
Setting Up Your Microsoft Dynamics 365 Test/Sandbox Account
Before you can start integrating with the Microsoft Dynamics 365 API, you need to set up a test or sandbox account. This environment allows you to safely test your API interactions without affecting live data.
Creating a Microsoft Dynamics 365 Sandbox Account
If you don't already have a Microsoft Dynamics 365 account, you can sign up for a free trial or a sandbox account. Follow these steps to get started:
- Visit the Microsoft Dynamics 365 website and navigate to the trial or sandbox account section.
- Sign up by providing the necessary information, such as your name, email address, and company details.
- Once registered, you will receive an email with instructions to access your sandbox environment.
Registering an Application for OAuth Authentication
To interact with the Microsoft Dynamics 365 API, you need to register an application in your Microsoft Entra ID tenant. This registration provides you with the client ID and client secret required for OAuth authentication.
- Go to the Azure Portal and sign in with your Microsoft account.
- Navigate to "Azure Active Directory" and select "App registrations."
- Click on "New registration" and fill in the application details, such as the name and redirect URI.
- After registration, note down the Application (client) ID and Directory (tenant) ID.
- Under "Certificates & secrets," create a new client secret and save it securely.
Configuring API Permissions
To allow your application to access Microsoft Dynamics 365 data, you need to configure the necessary API permissions:
- In the Azure Portal, navigate to your registered application.
- Select "API permissions" and click on "Add a permission."
- Choose "Dynamics CRM" and select the permissions required for your application, such as "user_impersonation."
- Grant admin consent for the permissions to ensure they are active.
With your sandbox account set up and your application registered, you're ready to start making API calls to Microsoft Dynamics 365 using PHP.
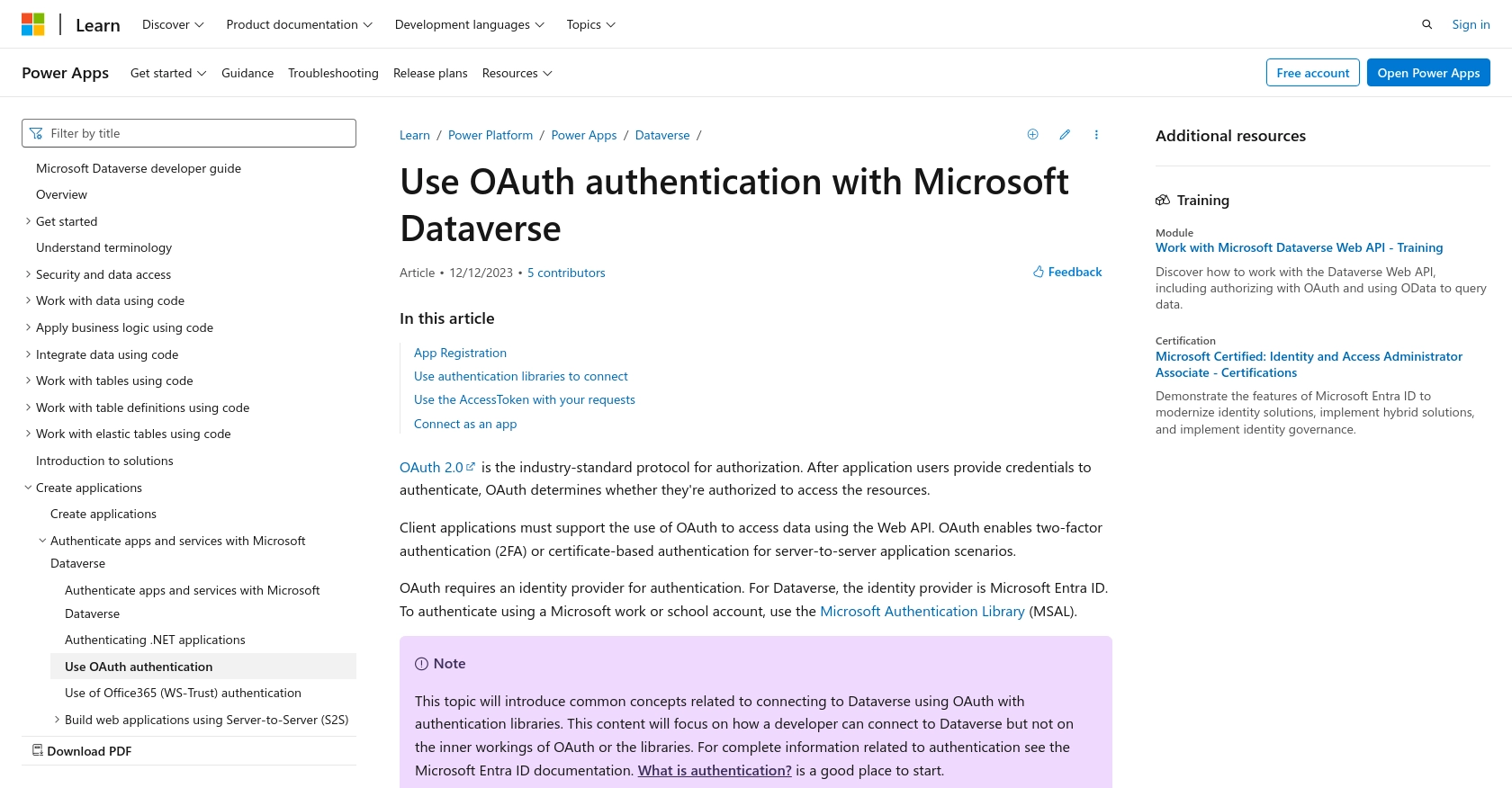
sbb-itb-96038d7
Making API Calls to Microsoft Dynamics 365 Using PHP
To interact with the Microsoft Dynamics 365 API using PHP, you'll need to set up your environment and write the necessary code to create or update contact records. This section will guide you through the process, including setting up PHP, installing dependencies, and executing API calls.
Setting Up Your PHP Environment for Microsoft Dynamics 365 API
Before making API calls, ensure that your PHP environment is properly configured. You'll need PHP version 7.4 or higher and the Composer dependency manager.
- Install PHP from the official PHP website.
- Download and install Composer from Composer's official site.
Installing Required PHP Libraries for Microsoft Dynamics 365 API Integration
To handle HTTP requests and OAuth authentication, you'll need the following PHP libraries:
composer require guzzlehttp/guzzle
composer require league/oauth2-client
Creating or Updating Contacts in Microsoft Dynamics 365 Using PHP
With your environment set up, you can now write PHP code to create or update contacts in Microsoft Dynamics 365. Below is an example of how to achieve this using the Guzzle HTTP client and OAuth2 client libraries.
require 'vendor/autoload.php';
use GuzzleHttp\Client;
use League\OAuth2\Client\Provider\GenericProvider;
// Set up the OAuth2 provider
$provider = new GenericProvider([
'clientId' => 'Your_Client_ID',
'clientSecret' => 'Your_Client_Secret',
'redirectUri' => 'Your_Redirect_URI',
'urlAuthorize' => 'https://login.microsoftonline.com/common/oauth2/v2.0/authorize',
'urlAccessToken' => 'https://login.microsoftonline.com/common/oauth2/v2.0/token',
'urlResourceOwnerDetails' => 'https://graph.microsoft.com/v1.0/me'
]);
// Obtain an access token
$accessToken = $provider->getAccessToken('client_credentials');
// Set up the HTTP client
$client = new Client([
'base_uri' => 'https://yourorg.crm.dynamics.com/api/data/v9.2/',
'headers' => [
'Authorization' => 'Bearer ' . $accessToken->getToken(),
'Content-Type' => 'application/json',
'OData-MaxVersion' => '4.0',
'OData-Version' => '4.0',
'Accept' => 'application/json'
]
]);
// Define the contact data
$contactData = [
'firstname' => 'John',
'lastname' => 'Doe',
'emailaddress1' => 'john.doe@example.com'
];
// Create or update the contact
$response = $client->post('contacts', [
'json' => $contactData
]);
// Check the response
if ($response->getStatusCode() == 204) {
echo "Contact created or updated successfully.";
} else {
echo "Failed to create or update contact.";
}
Verifying API Call Success in Microsoft Dynamics 365
After executing the API call, verify the success by checking the response status code. A status code of 204 indicates that the contact was successfully created or updated. You can also log in to your Microsoft Dynamics 365 sandbox account to confirm the changes.
Handling Errors and Error Codes in Microsoft Dynamics 365 API
When making API calls, it's crucial to handle potential errors. Common error codes include:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions.
- 403 Forbidden: The request is understood, but it has been refused.
- 404 Not Found: The requested resource could not be found.
For more detailed error handling, refer to the Microsoft Dynamics 365 API documentation.
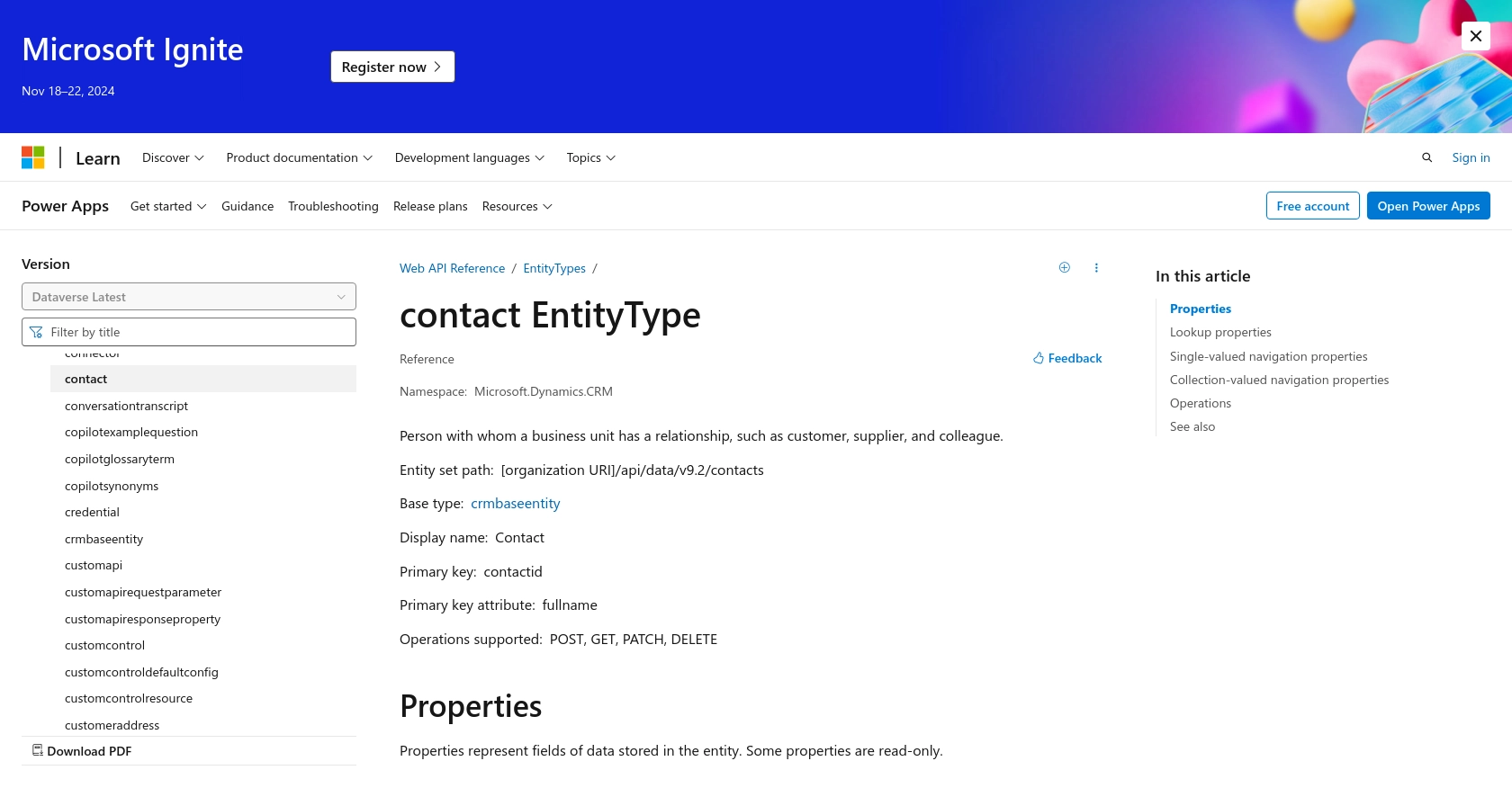
Conclusion and Best Practices for Microsoft Dynamics 365 API Integration
Integrating with the Microsoft Dynamics 365 API using PHP provides a powerful way to automate and manage customer data efficiently. By following the steps outlined in this guide, developers can create or update contact records seamlessly, ensuring data consistency across the organization.
Best Practices for Managing Microsoft Dynamics 365 API Integrations
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: Be aware of API rate limits to avoid throttling. Implement retry logic with exponential backoff to manage requests efficiently. For more details, refer to the Microsoft Graph API documentation.
- Standardize Data Fields: Ensure that data fields are standardized across your application to maintain consistency and avoid errors during API interactions.
- Implement Error Handling: Use robust error handling to manage API response codes effectively. Log errors for troubleshooting and consider implementing alerts for critical failures.
Streamlining Integrations with Endgrate
While integrating with Microsoft Dynamics 365 can be highly beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes, allowing developers to focus on core product development.
By leveraging Endgrate, you can build once for each use case and connect to various platforms effortlessly. This approach not only saves time and resources but also enhances the integration experience for your customers. Explore how Endgrate can transform your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/microsoftdynamics365
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/authenticate-oauth
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/webapi/reference/contact?view=dataverse-latest
Ready to get started?