Using the Google Docs API to Get Document Texts in PHP
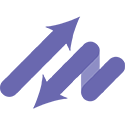
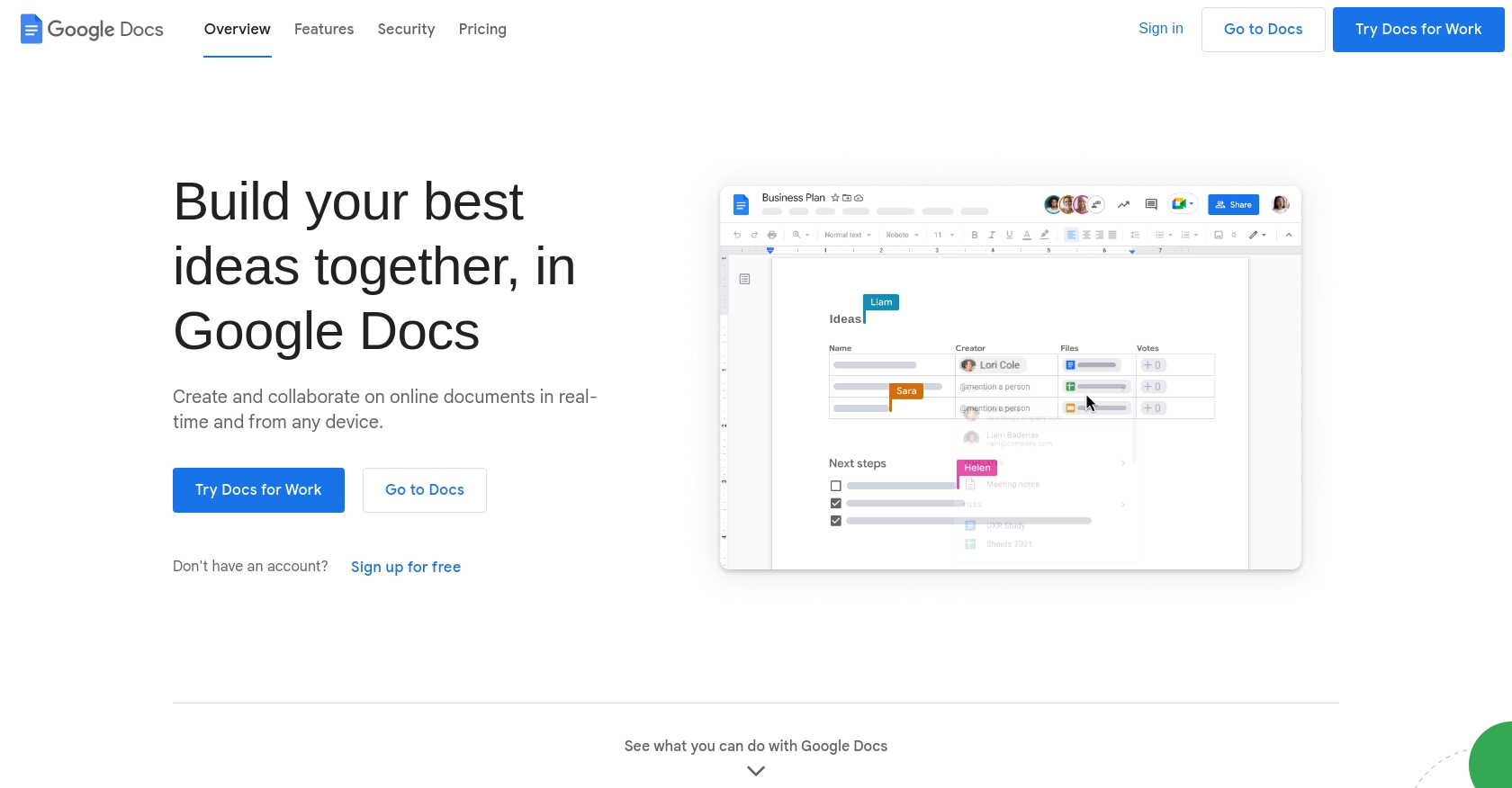
Introduction to Google Docs API
Google Docs is a widely used cloud-based word processing application that allows users to create, edit, and collaborate on documents in real-time. Its seamless integration with other Google Workspace tools makes it an essential platform for businesses and individuals alike.
For developers, integrating with the Google Docs API opens up a world of possibilities for automating document management tasks. By accessing and manipulating document content programmatically, developers can create applications that enhance productivity and streamline workflows.
One practical use case for the Google Docs API is retrieving text from documents to perform operations such as data analysis, content generation, or integration with other systems. This article will guide you through using PHP to interact with the Google Docs API and extract document texts efficiently.
Setting Up Your Google Docs API Test Account
Before you can start interacting with the Google Docs API using PHP, you need to set up a Google Cloud project and configure OAuth 2.0 authentication. This setup will allow you to securely access the API and retrieve document texts.
Create a Google Cloud Project for Google Docs API
- Go to the Google Cloud Console.
- Click on the menu icon and navigate to IAM & Admin > Create a Project.
- Enter a project name and select your organization and location if applicable.
- Click Create to set up your new project.
Enable the Google Docs API
- In the Google Cloud Console, navigate to APIs & Services > Library.
- Search for "Google Docs API" and click on it.
- Click Enable to activate the API for your project.
Configure OAuth Consent Screen
- Go to APIs & Services > OAuth consent screen.
- Select the user type for your app and click Create.
- Fill out the necessary fields such as app name, support email, and developer contact information.
- Click Save and Continue.
Create OAuth 2.0 Credentials for Google Docs API
- Navigate to APIs & Services > Credentials.
- Click Create Credentials and select OAuth client ID.
- Choose the application type that suits your needs, such as Web application.
- Fill in the required fields, including authorized redirect URIs.
- Click Create to generate your client ID and client secret.
Make sure to securely store your client ID and client secret, as you will need them to authenticate API requests. For more detailed instructions, refer to the official Google documentation: Create a Google Cloud Project, Enable Google Workspace APIs, Configure OAuth Consent, and Create Credentials.
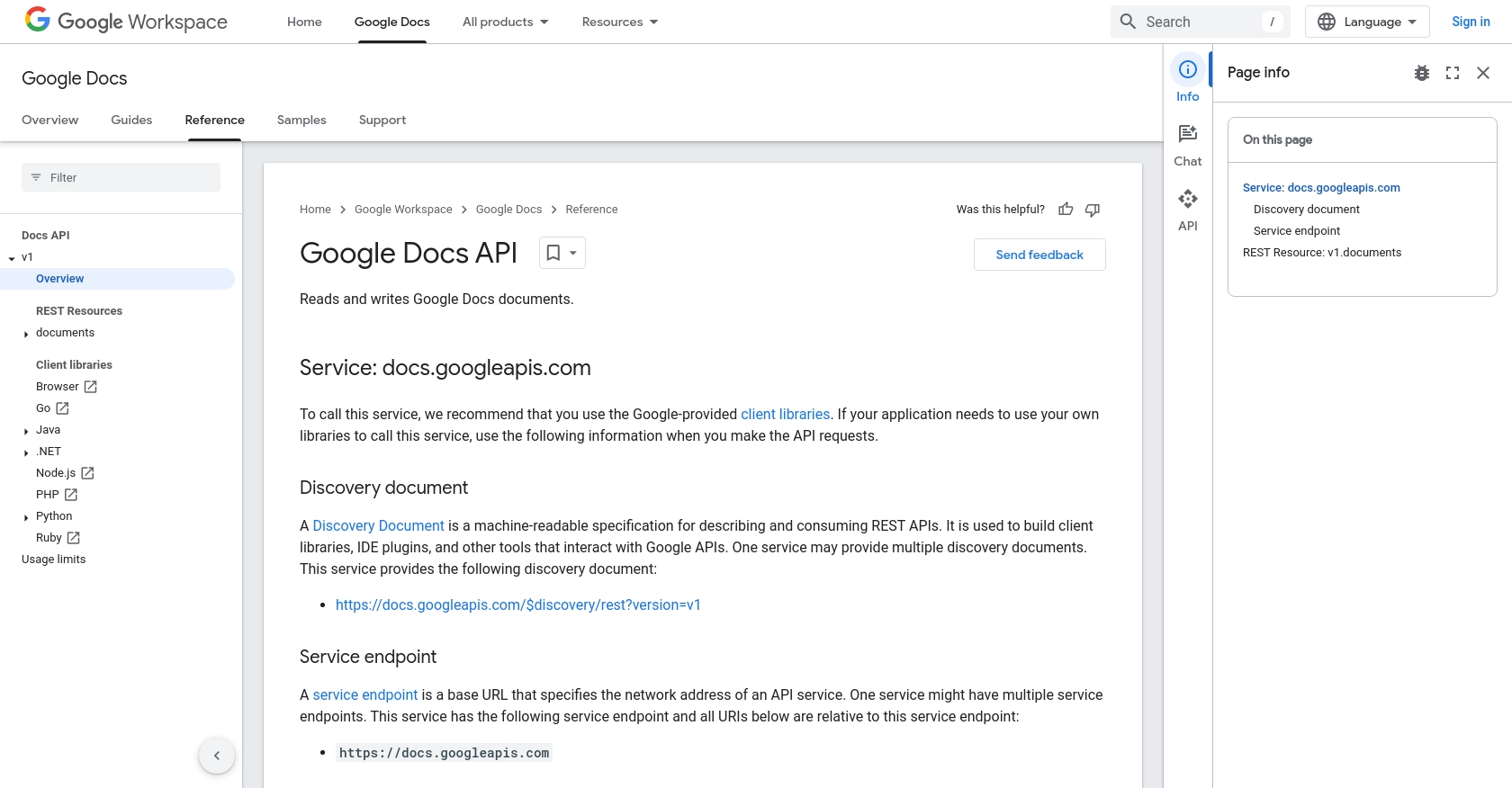
sbb-itb-96038d7
Making API Calls to Google Docs Using PHP
To interact with the Google Docs API using PHP, you'll need to set up your environment and write code to authenticate and make API requests. This section will guide you through the necessary steps to retrieve document texts using PHP.
Setting Up Your PHP Environment
Before making API calls, ensure you have the following prerequisites:
- PHP 7.4 or higher installed on your machine.
- Composer, the PHP package manager, for managing dependencies.
Install the Google Client Library for PHP using Composer:
composer require google/apiclient:^2.0
Authenticating with Google Docs API Using OAuth 2.0
To authenticate your requests, you'll need to use OAuth 2.0 credentials. Follow these steps to set up authentication:
- Ensure your OAuth 2.0 client ID and client secret are securely stored.
- Create a file named
oauth-credentials.json
and place your credentials in it.
Writing PHP Code to Retrieve Google Docs Text
Create a PHP script to authenticate and fetch document content:
require 'vendor/autoload.php';
use Google\Client;
use Google\Service\Docs;
// Initialize the Google Client
$client = new Client();
$client->setAuthConfig('oauth-credentials.json');
$client->addScope(Google\Service\Docs::DOCUMENTS_READONLY);
// Initialize the Google Docs Service
$service = new Docs($client);
// Specify the document ID
$documentId = 'your-document-id-here';
try {
// Retrieve the document
$document = $service->documents->get($documentId);
$content = $document->getBody()->getContent();
// Extract and display text content
foreach ($content as $element) {
if (isset($element->getParagraph()->getElements())) {
foreach ($element->getParagraph()->getElements() as $textElement) {
if ($textElement->getTextRun()) {
echo $textElement->getTextRun()->getContent();
}
}
}
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace your-document-id-here
with the actual ID of the Google Doc you wish to access. This script initializes the Google Client, authenticates using OAuth 2.0, and retrieves the document content.
Verifying API Call Success and Handling Errors
After running the script, verify the output by checking the retrieved text against the document in your Google Docs account. If the request is successful, the text content will be displayed in your terminal.
Handle potential errors by catching exceptions and displaying error messages. This will help you diagnose issues such as invalid credentials or incorrect document IDs.
For more information on the API call, refer to the official documentation: Google Docs API Reference.
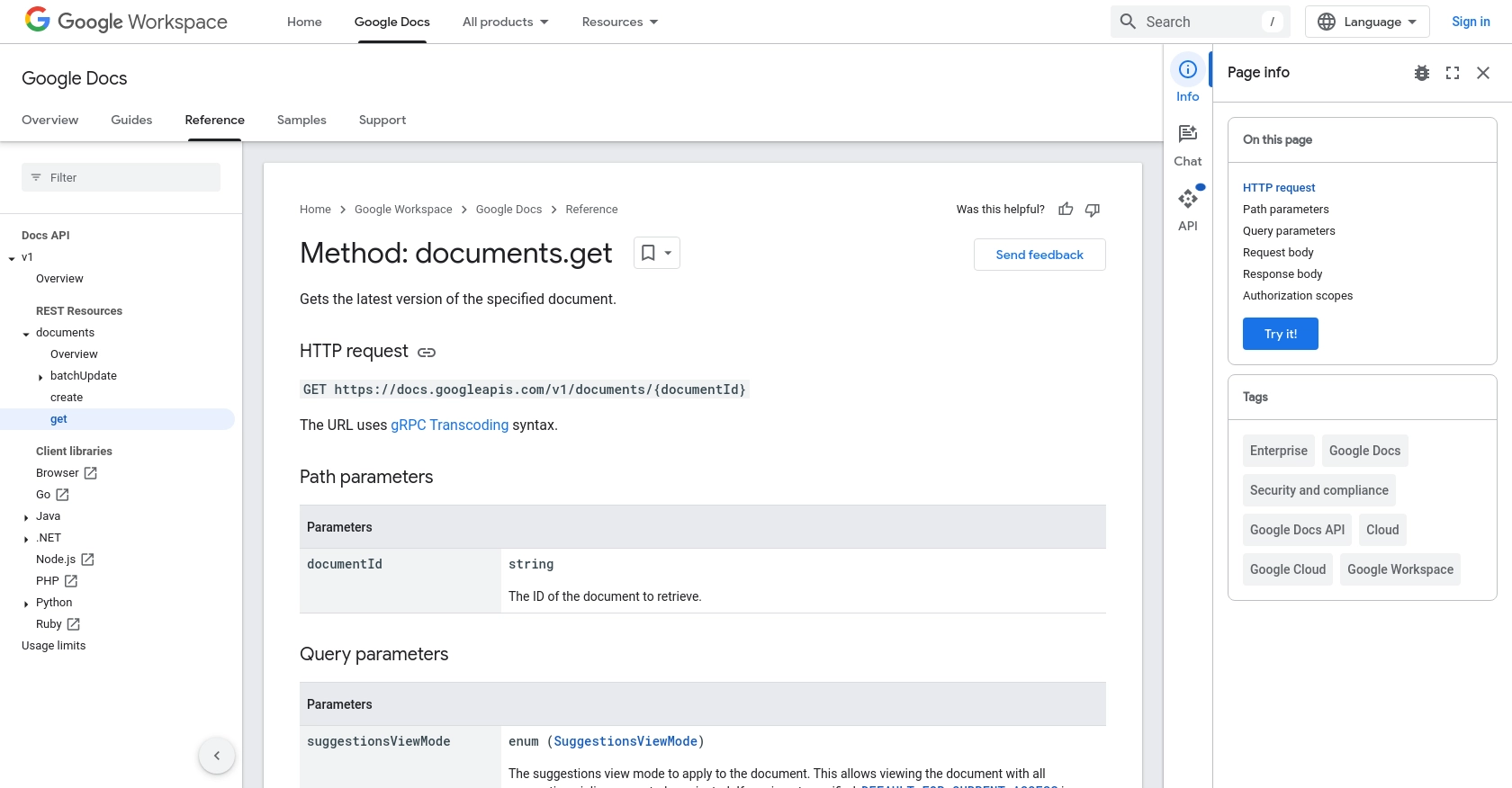
Conclusion: Best Practices for Using Google Docs API with PHP
Integrating the Google Docs API with PHP offers a powerful way to automate document management and enhance productivity. By following the steps outlined in this guide, you can efficiently retrieve document texts and incorporate them into your applications.
Best Practices for Securely Storing OAuth Credentials
- Always store your OAuth client ID and client secret securely, avoiding hardcoding them in your scripts.
- Use environment variables or secure storage solutions to manage sensitive information.
Handling Google Docs API Rate Limits and Errors
- Be mindful of the API's rate limits to avoid exceeding the allowed number of requests. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Consult the Google Docs API documentation for detailed information on error codes and handling strategies.
Transforming and Standardizing Google Docs Data
- Consider transforming and standardizing the retrieved text data to fit your application's requirements.
- Utilize PHP's string manipulation functions to clean and format the document content as needed.
By leveraging the Google Docs API, you can streamline workflows and create seamless integrations with other systems. If you're looking to simplify and scale your integration efforts, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Explore the benefits of a unified API experience and enhance your application's capabilities today.
Read More
- https://endgrate.com/provider/googledocs
- https://developers.google.com/docs/api/reference/rest
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/docs/api/reference/rest/v1/documents/get
Ready to get started?