Using the Hubspot API to Create or Update Companies (with Python examples)
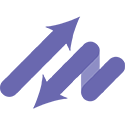
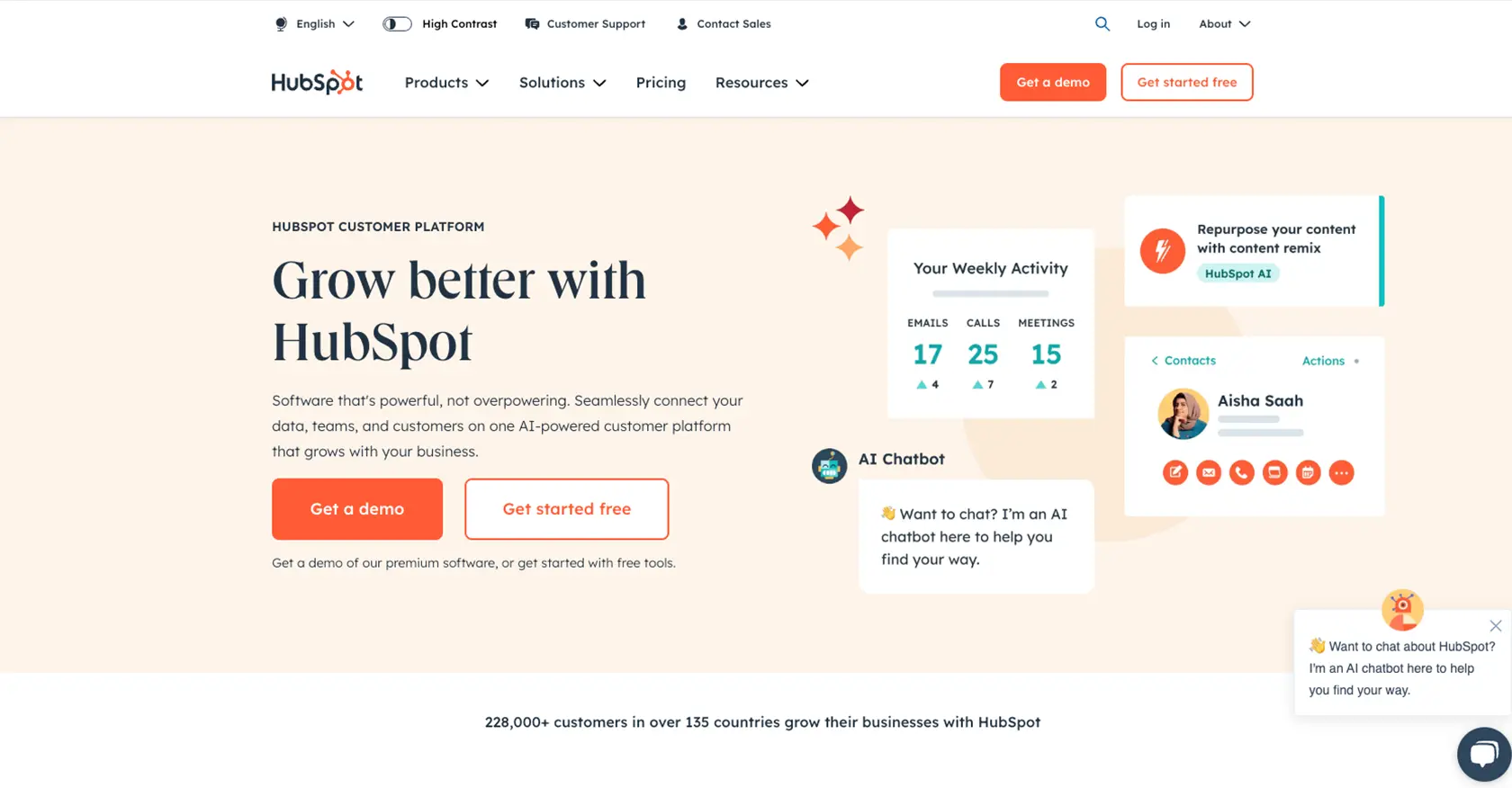
Introduction to HubSpot API for Company Management
HubSpot is a comprehensive CRM platform that empowers businesses to manage their marketing, sales, and customer service operations seamlessly. With its robust suite of tools, HubSpot enables organizations to streamline their workflows and enhance customer engagement.
For developers, integrating with HubSpot's API offers the opportunity to automate and optimize various CRM tasks. One such task is managing company records, which can be crucial for maintaining up-to-date business information and fostering better client relationships. By using the HubSpot API, developers can create or update company records programmatically, ensuring data consistency and reducing manual effort.
Consider a scenario where a developer needs to update company details in HubSpot based on changes in an external database. By leveraging the HubSpot API, this process can be automated, allowing for real-time synchronization of company data across platforms.
Setting Up Your HubSpot Developer Account for API Integration
Before you can start creating or updating companies using the HubSpot API, you need to set up a HubSpot developer account. This account will allow you to create a private app, which is essential for generating the necessary OAuth credentials to authenticate your API requests.
Step-by-Step Guide to Creating a HubSpot Developer Account
-
Sign Up for a Developer Account:
Visit the HubSpot Developer Portal and sign up for a free developer account. This account will give you access to HubSpot's API documentation and tools.
-
Create a Test Account:
Once your developer account is set up, create a test account. This sandbox environment allows you to safely test your API integrations without affecting live data.
Creating a HubSpot Private App for OAuth Authentication
HubSpot uses OAuth for authentication, which requires you to create a private app to obtain the client ID and client secret. Follow these steps to set up your private app:
-
Access the Private Apps Section:
In your HubSpot account, navigate to Settings and then to Integrations > Private Apps.
-
Create a New Private App:
Click on Create a private app and fill in the required details, such as the app name and description.
-
Set Scopes for Company Management:
Under the Scopes section, ensure you select the necessary scopes for managing companies, such as
crm.objects.companies.read
andcrm.objects.companies.write
. This will grant your app the permissions needed to interact with company records. -
Generate OAuth Credentials:
After setting up your app, HubSpot will provide you with a client ID and client secret. Keep these credentials secure, as they are essential for authenticating your API requests.
With your HubSpot developer account and private app set up, you are now ready to start integrating with the HubSpot API to create or update company records programmatically.
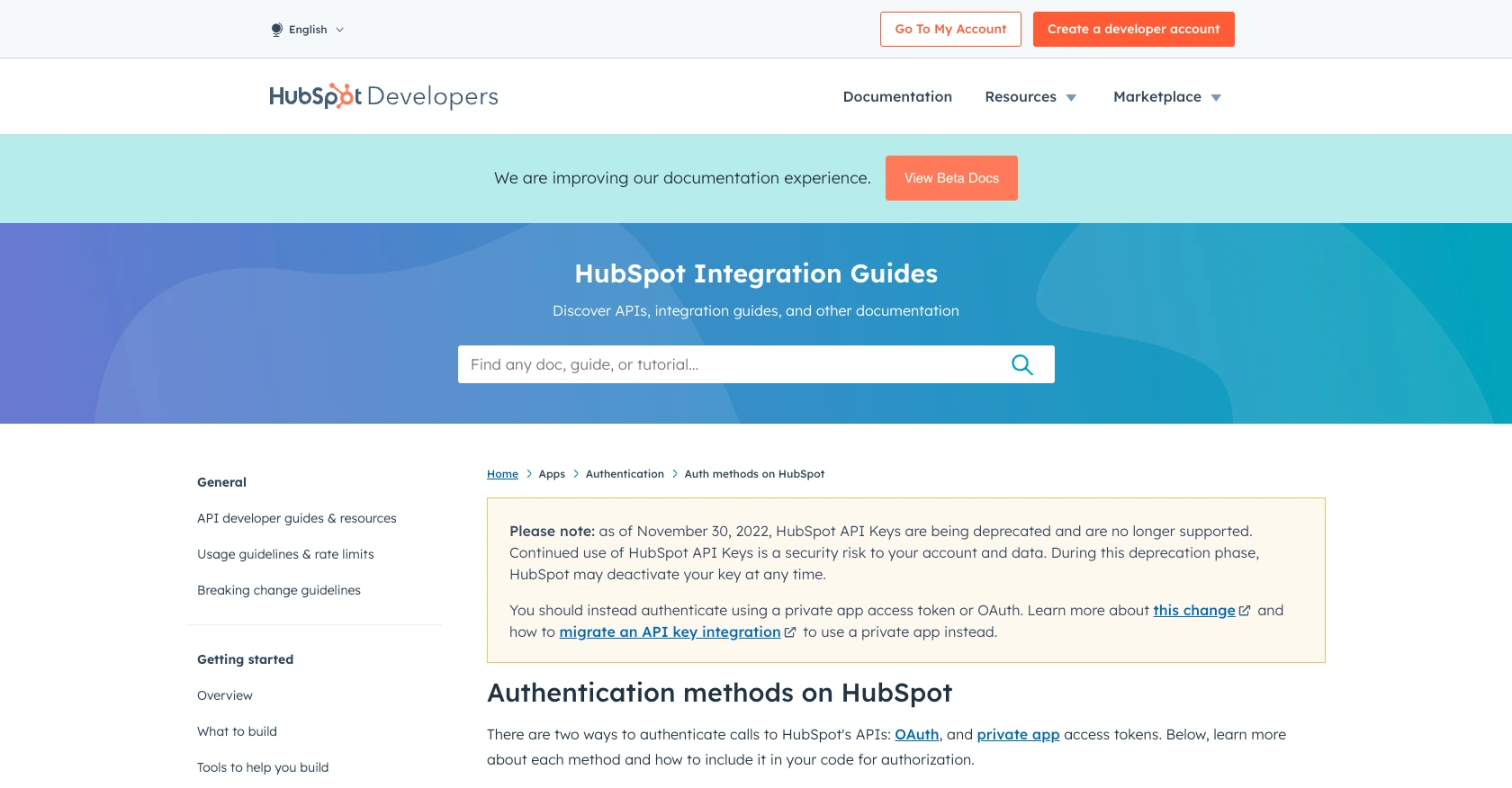
sbb-itb-96038d7
Making API Calls to Create or Update Companies in HubSpot Using Python
To interact with the HubSpot API for creating or updating company records, you'll need to use Python. This section will guide you through the process, including setting up your environment, writing the code, and handling responses.
Setting Up Your Python Environment for HubSpot API Integration
Before making API calls, ensure you have the necessary tools and libraries installed:
- Python 3.11.1: Ensure you have Python installed on your machine. You can download it from the official Python website.
- Requests Library: This library is essential for making HTTP requests in Python. Install it using pip:
pip install requests
Creating a Company in HubSpot Using Python
To create a new company in HubSpot, you'll need to make a POST request to the HubSpot API. Here's a step-by-step guide:
import requests
# Set the API endpoint
url = "https://api.hubapi.com/crm/v3/objects/companies"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Token"
}
# Define the company data
company_data = {
"properties": {
"name": "Example Company",
"domain": "example.com",
"city": "New York",
"industry": "Technology"
}
}
# Make the POST request
response = requests.post(url, json=company_data, headers=headers)
# Check the response status
if response.status_code == 201:
print("Company created successfully.")
else:
print("Failed to create company:", response.json())
Replace Your_Token
with your OAuth token. This code sends a POST request to create a new company with specified properties. If successful, you'll receive a confirmation message.
Updating an Existing Company in HubSpot Using Python
To update an existing company, use a PATCH request. Here's how you can do it:
import requests
# Set the API endpoint with the company ID
company_id = "123456"
url = f"https://api.hubapi.com/crm/v3/objects/companies/{company_id}"
# Set the request headers
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Token"
}
# Define the updated company data
updated_data = {
"properties": {
"city": "Boston",
"industry": "Software"
}
}
# Make the PATCH request
response = requests.patch(url, json=updated_data, headers=headers)
# Check the response status
if response.status_code == 200:
print("Company updated successfully.")
else:
print("Failed to update company:", response.json())
Replace Your_Token
with your OAuth token and company_id
with the ID of the company you wish to update. This code updates the specified properties of an existing company.
Handling API Responses and Errors
When making API calls, it's crucial to handle responses and potential errors effectively:
- Success: A status code of 201 indicates successful creation, while 200 indicates a successful update.
- Errors: If you encounter errors, such as a 400 or 401 status code, check the response message for details. Common issues include invalid tokens or missing required fields.
For more information on error codes, refer to the HubSpot API documentation.
Verifying Your API Requests in HubSpot
After making API calls, verify the changes in your HubSpot test account. Navigate to the Companies section to confirm that the new or updated company records appear as expected.
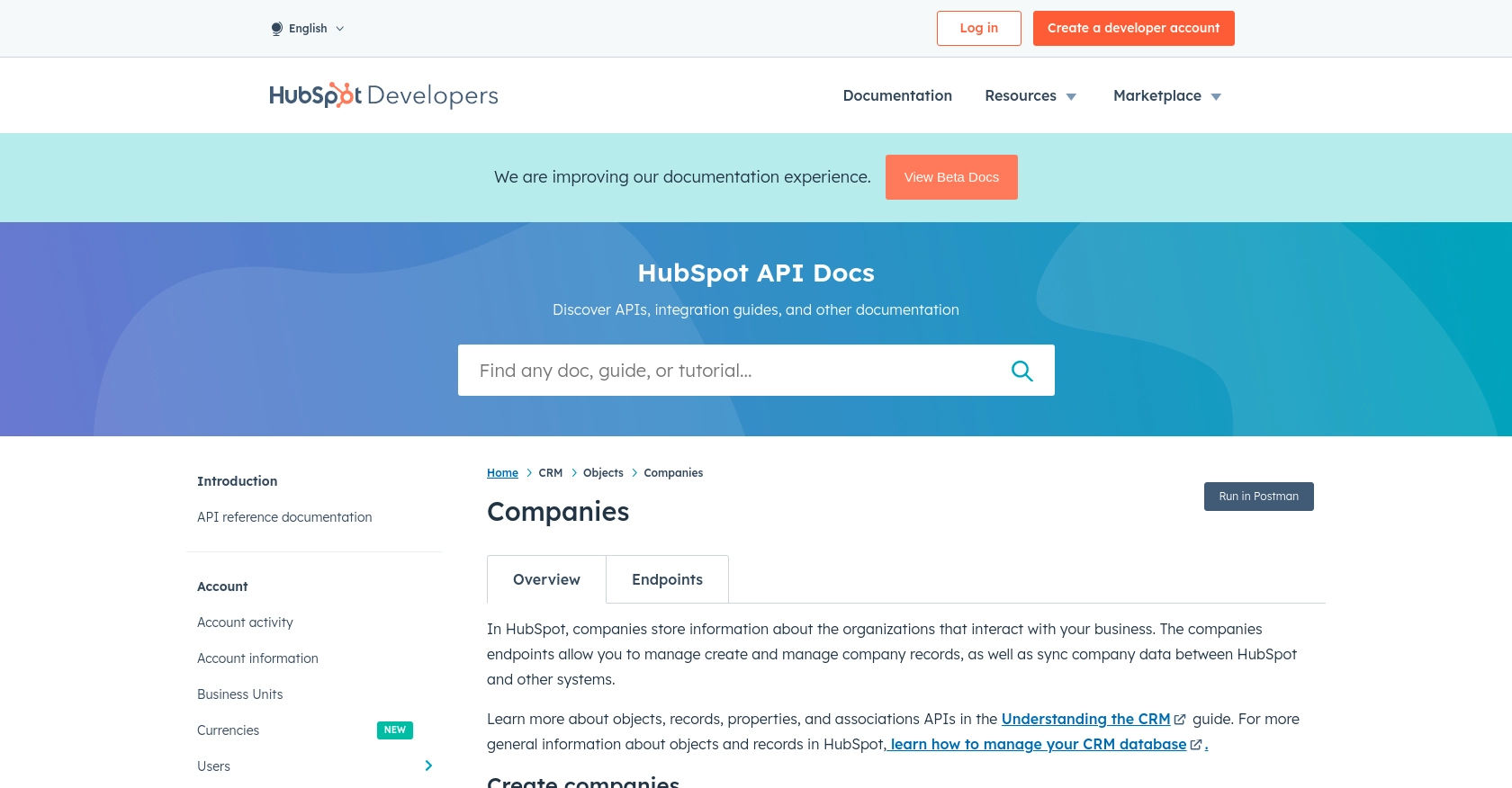
Conclusion and Best Practices for Using HubSpot API for Company Management
Integrating with the HubSpot API to manage company records offers developers a powerful way to automate CRM tasks and ensure data consistency. By following the steps outlined in this guide, you can efficiently create and update company information using Python, streamlining your business processes.
Best Practices for Secure and Efficient HubSpot API Integration
- Secure Storage of Credentials: Always store your OAuth credentials securely. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of HubSpot's rate limits. For public apps using OAuth, the limit is 100 requests every 10 seconds per account. Implement throttling to avoid hitting these limits. For more details, refer to the HubSpot API usage guidelines.
- Error Handling: Implement robust error handling to manage API response errors gracefully. Check for common error codes like 429 for rate limits and 401 for unauthorized access.
- Data Standardization: Ensure that the data you send to HubSpot is standardized and consistent. This helps in maintaining data integrity across your CRM and other integrated systems.
Enhance Your Integration Strategy with Endgrate
While integrating with HubSpot's API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate simplifies this process by providing a unified API endpoint for various integrations, including HubSpot. By using Endgrate, you can:
- Save time and resources by outsourcing integration management.
- Build once for each use case instead of multiple times for different integrations.
- Offer an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration processes and allow you to focus on your core product. Visit Endgrate to learn more.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/companies
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?