Using the Sap Business One API to Get Customers in PHP
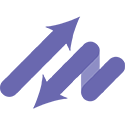
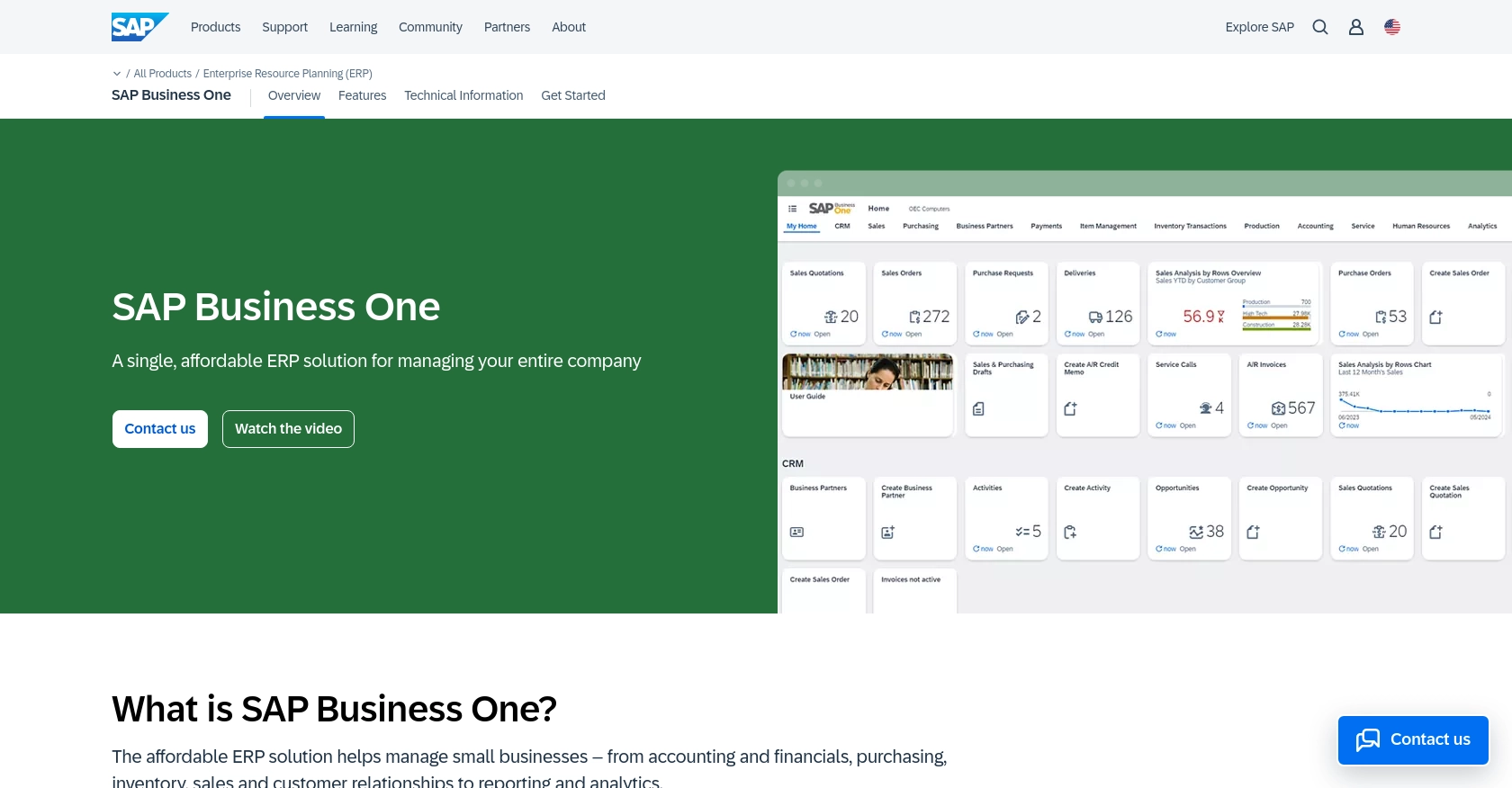
Introduction to SAP Business One
SAP Business One is a comprehensive enterprise resource planning (ERP) solution designed specifically for small and medium-sized businesses. It offers a wide range of functionalities, including financial management, sales, customer relationship management, and inventory control, all within a single, integrated platform.
Developers may want to integrate with the SAP Business One API to streamline business operations and enhance data accessibility. For example, using the API to retrieve customer data can help businesses maintain up-to-date records, improve customer service, and automate various sales processes.
This article will guide you through using PHP to interact with the SAP Business One API, specifically focusing on retrieving customer information. By following this tutorial, you'll be able to efficiently access and manage customer data within the SAP Business One environment.
Setting Up a SAP Business One Test/Sandbox Account
Before you can start interacting with the SAP Business One API, you need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data. Follow these steps to get started:
Creating a SAP Business One Sandbox Account
To create a sandbox account, you will need access to SAP Business One. If you don't already have an account, you can contact SAP or an authorized partner to inquire about trial or demo access. This will provide you with a controlled environment to test API interactions.
Generating API Credentials for SAP Business One
SAP Business One uses a custom authentication method. To interact with the API, you need to generate the necessary credentials. Follow these steps:
- Log in to your SAP Business One sandbox account.
- Navigate to the Service Layer Management section.
- Create a new application and note down the Client ID and Client Secret. These will be used to authenticate API requests.
Configuring OAuth for SAP Business One API Access
Although SAP Business One uses a custom authentication method, it may involve OAuth-like steps for token generation. Here's how you can configure it:
- Access the OAuth Configuration settings in your sandbox account.
- Enter the Redirect URI and other required details.
- Save the configuration and retrieve the Access Token for API calls.
For detailed guidance, refer to the official SAP Business One documentation: SAP Business One Service Layer Documentation.
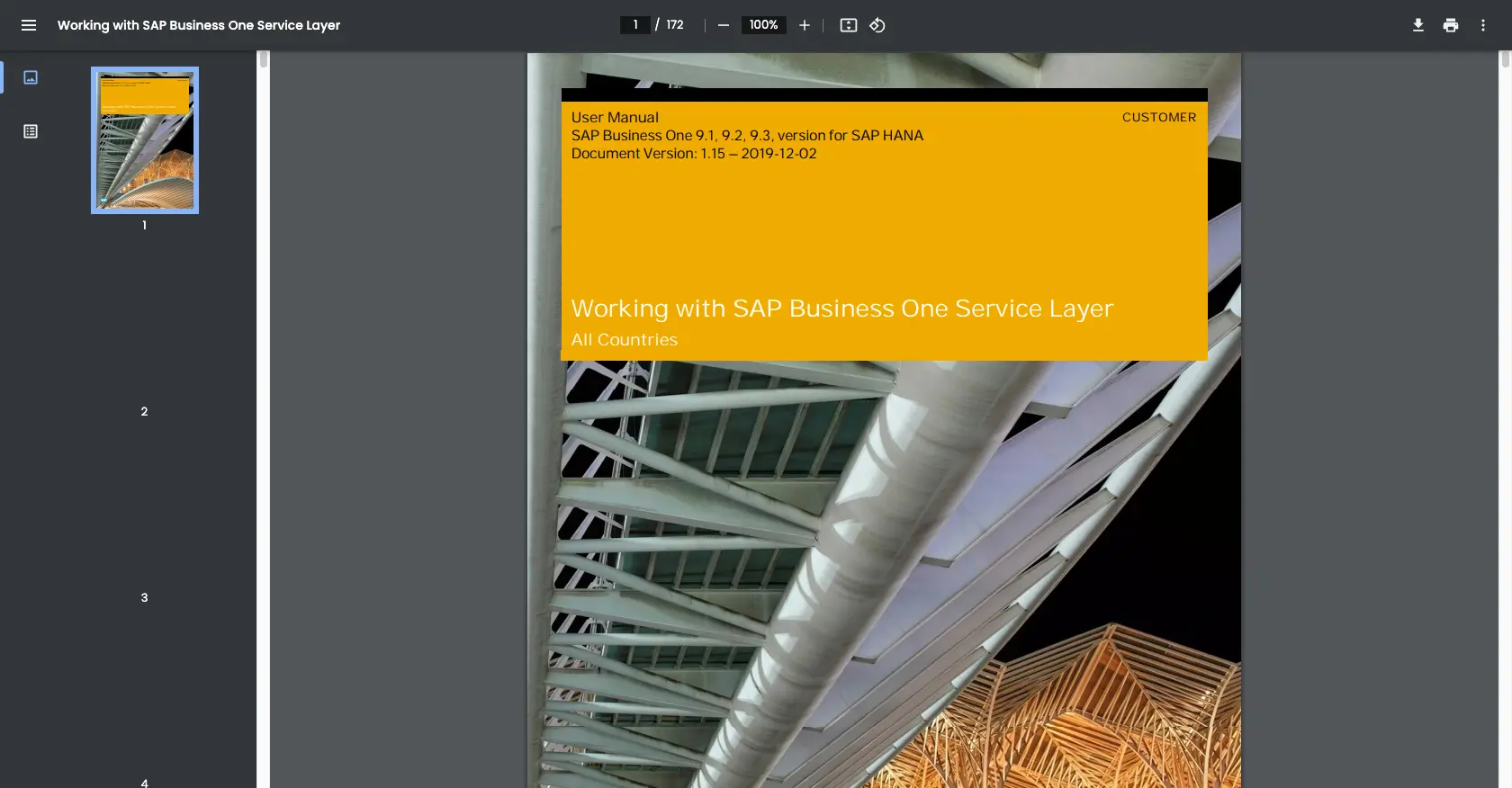
sbb-itb-96038d7
How to Make API Calls to Retrieve Customers Using SAP Business One in PHP
To interact with the SAP Business One API and retrieve customer data using PHP, you'll need to ensure that your development environment is properly set up. This section will guide you through the necessary steps, including setting up PHP, installing required dependencies, and executing the API call.
Setting Up Your PHP Environment for SAP Business One API
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer, the PHP package manager
Once you have these installed, open your terminal and navigate to your project directory. Run the following command to install the Guzzle HTTP client, which will be used to make HTTP requests:
composer require guzzlehttp/guzzle
Executing the API Call to Retrieve Customer Data
With your environment set up, you can now proceed to write the PHP code to interact with the SAP Business One API. Create a new PHP file named get_customers.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
// Initialize the Guzzle client
$client = new Client();
// Define the API endpoint and headers
$endpoint = 'https://your-sap-business-one-instance.com/b1s/v1/BusinessPartners';
$headers = [
'Content-Type' => 'application/json',
'Authorization' => 'Bearer Your_Access_Token'
];
try {
// Make a GET request to the API
$response = $client->request('GET', $endpoint, ['headers' => $headers]);
// Parse the JSON data from the response
$data = json_decode($response->getBody(), true);
// Loop through the customers and print their information
foreach ($data['value'] as $customer) {
echo 'Customer Code: ' . $customer['CardCode'] . '<br>';
echo 'Customer Name: ' . $customer['CardName'] . '<br><br>';
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace Your_Access_Token
with the access token obtained during the authentication setup.
Running the PHP Script and Verifying the Output
To execute the script, run the following command in your terminal:
php get_customers.php
If successful, you should see a list of customer codes and names printed in your terminal. This confirms that the API call was successful and that customer data was retrieved from the SAP Business One environment.
Handling Errors and Troubleshooting
While making API calls, you may encounter errors. Common error codes include:
- 401 Unauthorized: Check your access token and ensure it is valid.
- 404 Not Found: Verify the API endpoint URL.
- 500 Internal Server Error: This may indicate an issue with the SAP Business One server.
For more detailed error handling, refer to the official SAP Business One documentation: SAP Business One Service Layer Documentation.
Best Practices for Using the SAP Business One API in PHP
When working with the SAP Business One API, it's crucial to follow best practices to ensure efficient and secure integration. Here are some recommendations:
- Securely Store Credentials: Always store your API credentials, such as access tokens, securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Be aware of any rate limits imposed by the SAP Business One API. Implement logic to handle rate limit responses gracefully, such as retrying requests after a delay.
- Data Transformation and Standardization: Ensure that data retrieved from the API is transformed and standardized to fit your application's requirements. This may involve converting data formats or normalizing field names.
- Error Handling: Implement robust error handling to manage different types of API errors. Log errors for troubleshooting and provide meaningful feedback to users.
Streamlining Integrations with Endgrate
Integrating with multiple APIs can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including SAP Business One. By using Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product development.
- Build once for each use case instead of multiple times for different integrations, reducing redundancy.
- Offer an intuitive integration experience for your customers, enhancing user satisfaction.
Explore how Endgrate can transform your integration strategy by visiting Endgrate.
Read More
Ready to get started?