Using the Endear API to Get Notes (with Javascript examples)
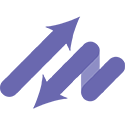
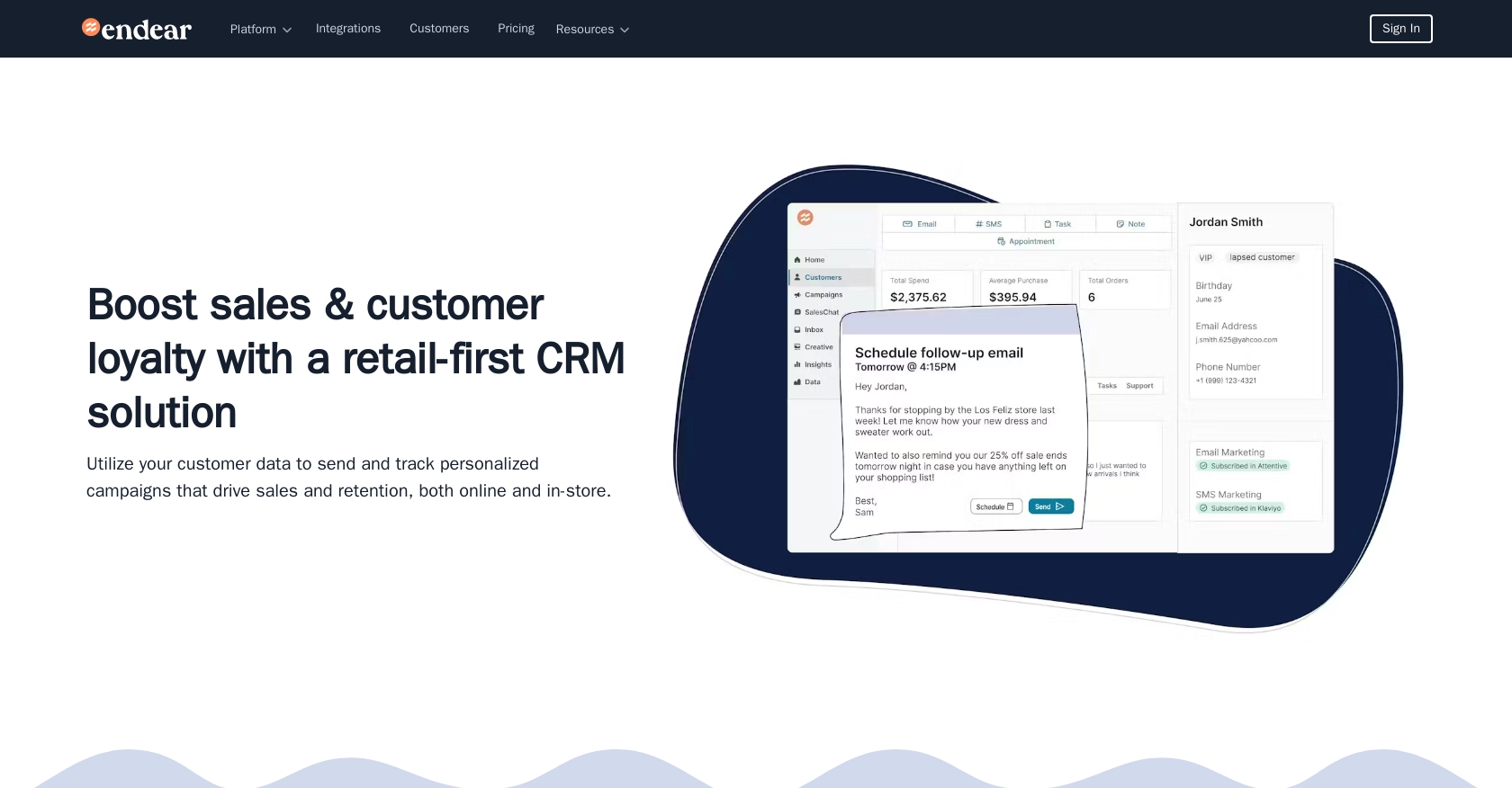
Introduction to Endear API Integration
Endear is a powerful CRM platform designed to enhance customer relationships by providing seamless integration capabilities for businesses. It offers a robust API that allows developers to access and manipulate customer data, making it an ideal choice for businesses looking to streamline their customer engagement processes.
Integrating with Endear's API can significantly benefit developers by enabling them to automate data retrieval and management tasks. For example, a developer might want to use the Endear API to fetch notes associated with customer interactions, allowing for better tracking and analysis of customer engagement. This can be particularly useful for businesses aiming to personalize their customer service and improve overall customer satisfaction.
Setting Up Your Endear Test Account for API Integration
Before diving into the Endear API integration, it's essential to set up a test account. This will allow you to safely experiment with API calls and understand how the integration works without affecting live data.
Creating an Endear Account
If you don't already have an Endear account, you can sign up for a free trial on the Endear website. Follow the instructions to create your account and log in.
Generating an API Key for Endear Integration
To interact with the Endear API, you'll need to generate an API key. Follow these steps to create one:
- Navigate to your Endear account settings.
- Click on Integrations in the menu.
- Select Add Integration and choose API.
- Fill in the required details and submit the form to generate your API key.
Once generated, your API key will be displayed. Make sure to copy and store it securely, as you'll need it to authenticate your API requests.
Authenticating API Requests with Your API Key
With your API key ready, you can now authenticate your requests to the Endear API. The API requires a POST request to the endpoint https://api.endearhq.com/graphql
. Include the following header in your request:
{
"X-Endear-Api-Key": "Your_API_Key"
}
Replace Your_API_Key
with the API key you generated earlier. This header is crucial for authenticating your requests and accessing the API.
For more detailed information on authentication, you can refer to the Endear authentication documentation.
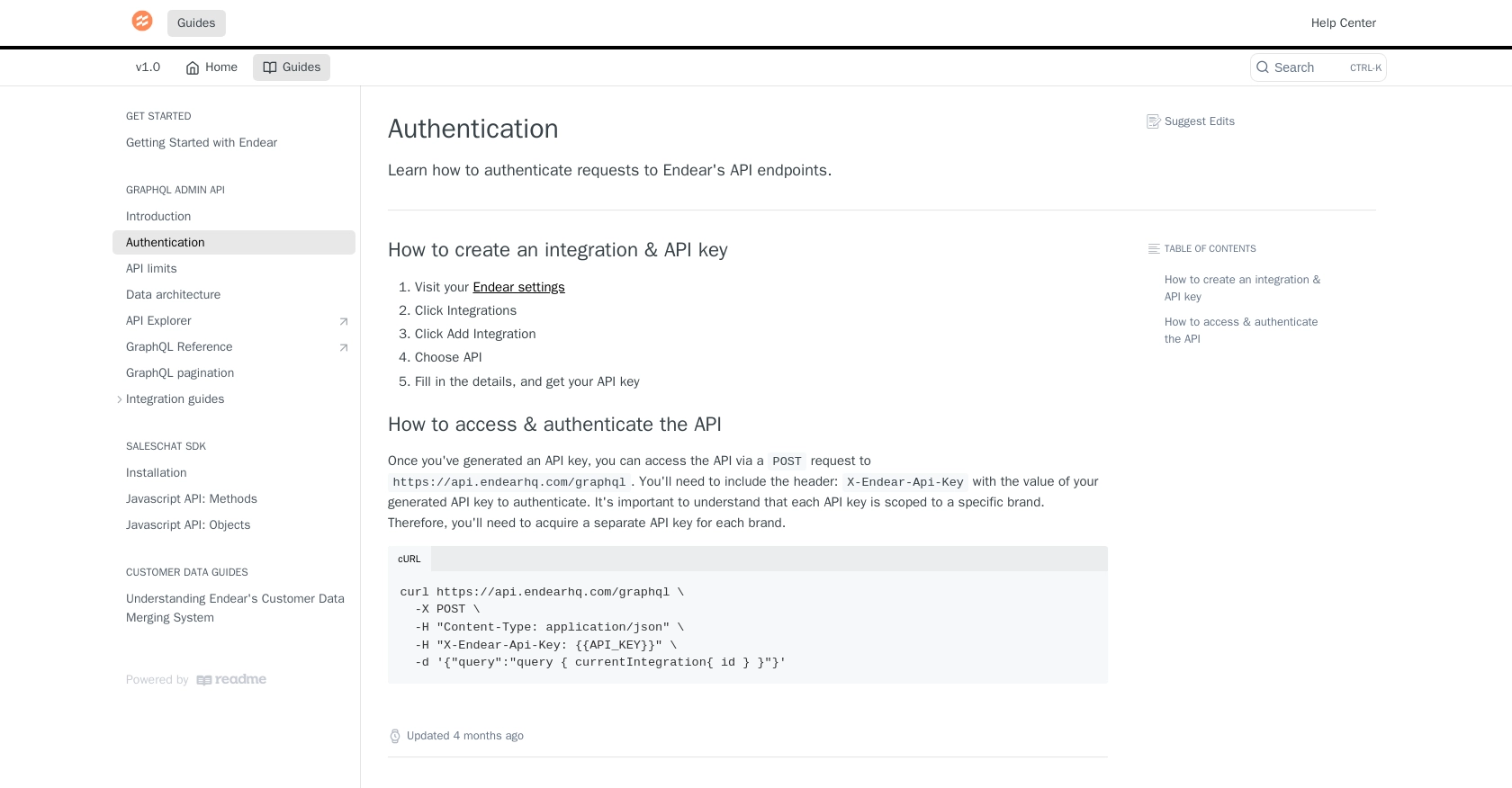
sbb-itb-96038d7
Making API Calls to Retrieve Notes from Endear Using JavaScript
To effectively interact with the Endear API and retrieve notes, you'll need to use JavaScript to send a POST request to the API endpoint. This section will guide you through the process, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for Endear API Integration
Before you begin coding, ensure you have the following prerequisites installed on your machine:
- Node.js (version 14 or higher)
- npm (Node Package Manager)
Once you have these installed, create a new project directory and initialize it with npm:
mkdir endear-api-integration
cd endear-api-integration
npm init -y
Installing Required Dependencies for Endear API Calls
You'll need the axios
library to make HTTP requests. Install it using the following command:
npm install axios
Writing JavaScript Code to Fetch Notes from Endear API
Create a new file named getNotes.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.endearhq.com/graphql';
const headers = {
'Content-Type': 'application/json',
'X-Endear-Api-Key': 'Your_API_Key'
};
// Define the GraphQL query
const query = `
query {
searchNotes {
edges {
node {
id
description
title
creator {
full_name
}
}
}
pageInfo {
endCursor
hasNextPage
}
}
}
`;
// Make a POST request to the API
axios.post(endpoint, { query }, { headers })
.then(response => {
const notes = response.data.data.searchNotes.edges;
notes.forEach(note => {
console.log(`ID: ${note.node.id}, Title: ${note.node.title}, Description: ${note.node.description}, Creator: ${note.node.creator.full_name}`);
});
})
.catch(error => {
console.error('Error fetching notes:', error.response ? error.response.data : error.message);
});
Replace Your_API_Key
with the API key you generated earlier. This script sends a POST request to the Endear API, retrieves notes, and logs them to the console.
Running Your JavaScript Code to Retrieve Endear Notes
Execute the script using Node.js:
node getNotes.js
You should see the notes printed in the console, including their IDs, titles, descriptions, and creators.
Handling Errors and Verifying API Call Success
To ensure your API call is successful, check the response status and data. If the request fails, the script logs an error message. Common errors include:
- HTTP 401: Unauthorized access. Verify your API key.
- HTTP 429: Rate limit exceeded. Endear's API allows 120 requests per minute. Consider implementing a retry mechanism.
For more details on error codes and rate limits, refer to the Endear API documentation.
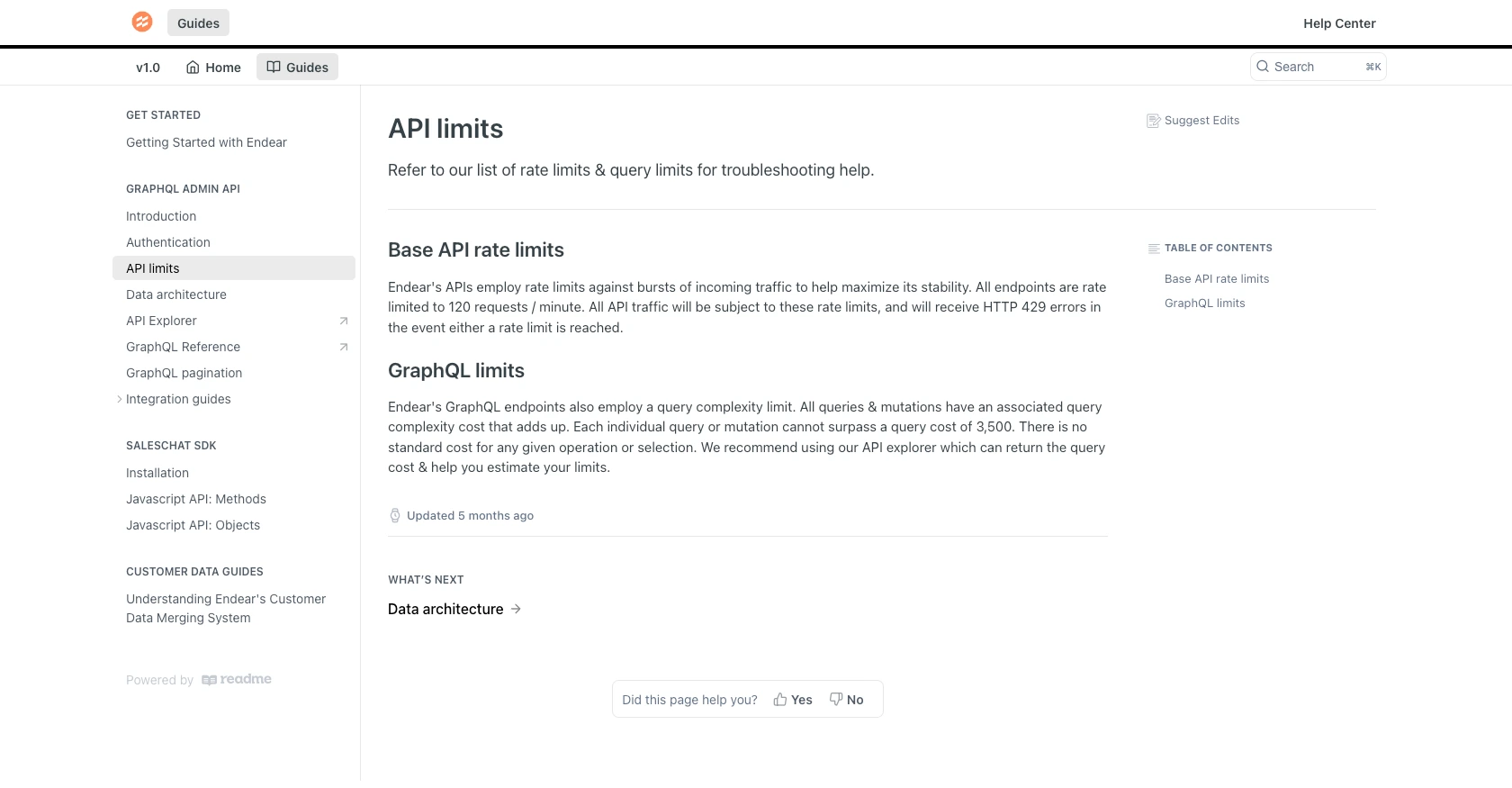
Conclusion and Best Practices for Using the Endear API with JavaScript
Integrating with the Endear API using JavaScript can greatly enhance your ability to manage and analyze customer interactions. By following the steps outlined in this guide, you can efficiently retrieve notes and leverage this data to improve customer engagement strategies.
Best Practices for Secure and Efficient Endear API Integration
- Secure API Key Storage: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Endear's rate limit of 120 requests per minute. Implement retry mechanisms or exponential backoff strategies to handle HTTP 429 errors gracefully.
- Data Standardization: Ensure that the data retrieved from Endear is standardized and transformed as needed to fit your application's requirements.
Enhance Your Integration Experience with Endgrate
While integrating with Endear's API directly is a powerful option, consider using Endgrate to streamline your integration process. Endgrate allows you to:
- Save time and resources by outsourcing complex integrations.
- Build once for each use case, reducing the need for multiple integrations.
- Provide an intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration needs by visiting Endgrate and discover the benefits of a unified API solution.
Read More
- https://endgrate.com/provider/endear
- https://docs.endearhq.com/docs/authentication
- https://docs.endearhq.com/docs/rate-limits-status-codes-and-errors
- https://docs.endearhq.com/docs/disclaimers
- https://developers.endearhq.com/docs/graphql/objects/Note
- https://docs.endearhq.com/docs/send-your-first-record
Ready to get started?