Using the Zoho CRM API to Create or Update Contacts (with PHP examples)
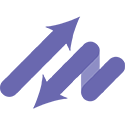
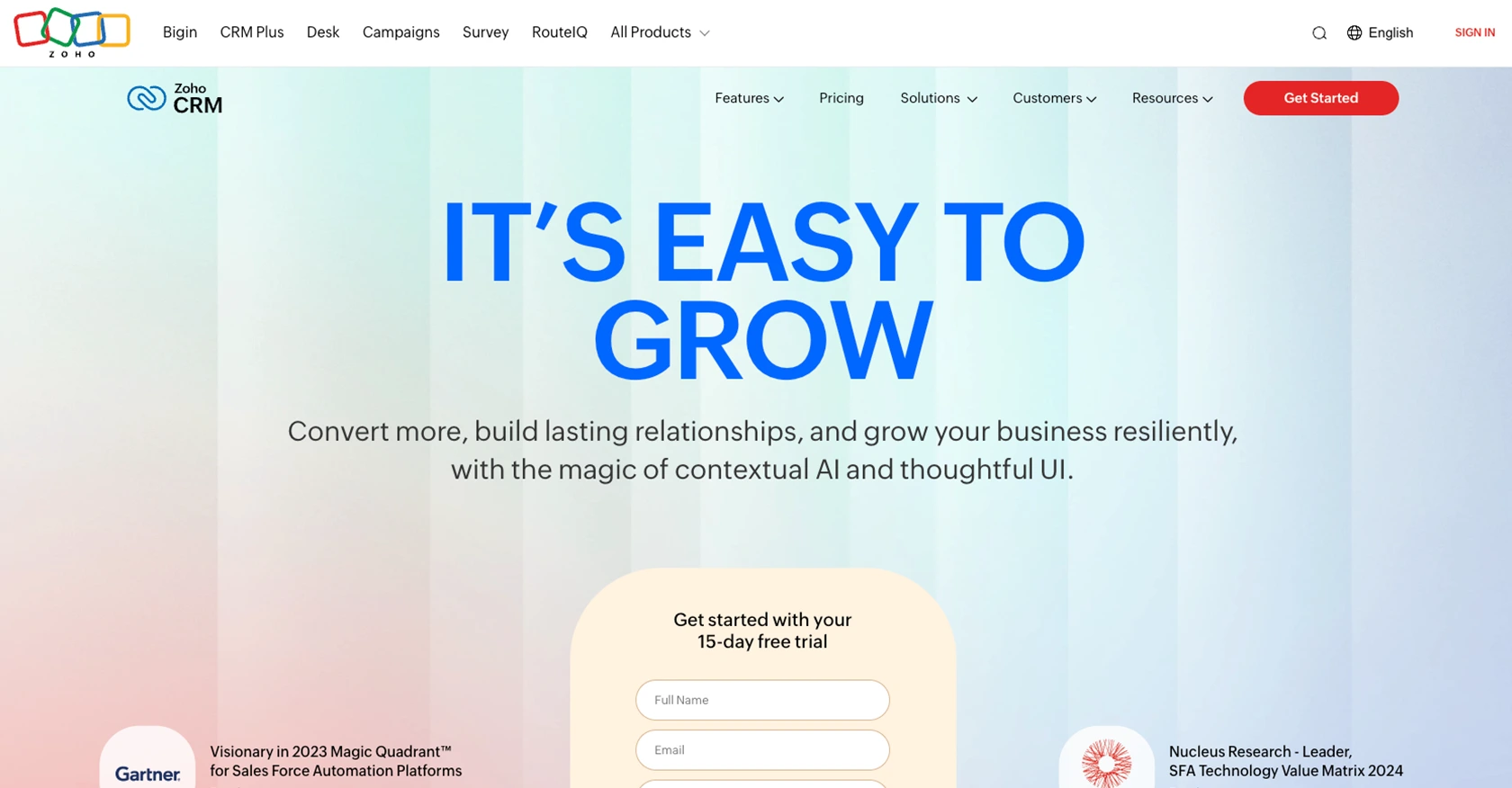
Introduction to Zoho CRM API
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and support in a unified system. Known for its flexibility and robust features, Zoho CRM is a popular choice for businesses looking to enhance their customer interactions and streamline operations.
Integrating with the Zoho CRM API allows developers to automate and enhance CRM functionalities, such as managing contacts. For example, developers can use the API to create or update contact information directly from their applications, ensuring that customer data is always up-to-date and accessible.
This article will guide you through using PHP to interact with the Zoho CRM API for creating or updating contacts, providing a step-by-step approach to streamline your CRM processes.
Setting Up Your Zoho CRM Sandbox Account
Before you can start interacting with the Zoho CRM API, you need to set up a sandbox account. This allows you to test API calls without affecting your live data. Zoho CRM provides a developer-friendly environment to experiment with API integrations.
Creating a Zoho CRM Sandbox Account
- Visit the Zoho Developer Console.
- Sign in with your Zoho account credentials. If you don't have an account, you can create one for free.
- Navigate to the "Sandbox" section and follow the instructions to set up your sandbox environment.
Registering Your Application for OAuth Authentication
Zoho CRM uses OAuth 2.0 for authentication. Follow these steps to register your application:
- In the Zoho Developer Console, select "Register Client."
- Choose the client type that suits your application (e.g., Web Based, Mobile).
- Fill in the required details:
- Client Name: The name of your application.
- Homepage URL: The URL of your web page.
- Authorized Redirect URIs: A valid URL where Zoho will redirect after authentication.
- Click "CREATE" to generate your Client ID and Client Secret.
Generating Access and Refresh Tokens
Once your application is registered, you need to generate access and refresh tokens to authenticate API requests:
- Make an authorization request to Zoho's OAuth server using your Client ID and Redirect URI.
- Receive an authorization code and exchange it for access and refresh tokens.
- Store these tokens securely, as they will be used to authenticate your API calls.
For detailed steps on generating tokens, refer to the Zoho CRM OAuth Documentation.
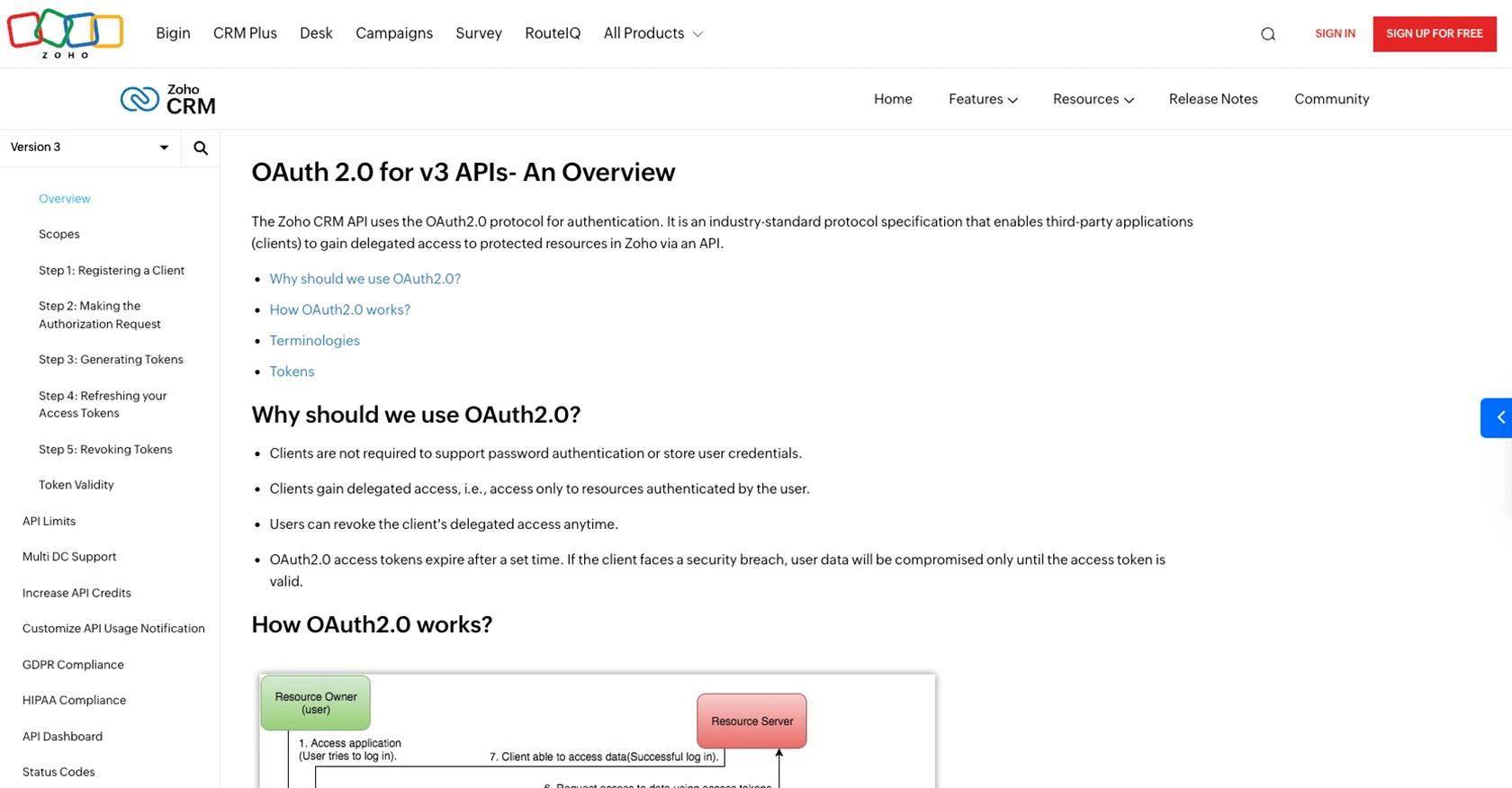
sbb-itb-96038d7
How to Make API Calls to Zoho CRM for Creating or Updating Contacts Using PHP
To interact with the Zoho CRM API for creating or updating contacts, you'll need to use PHP. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses.
Setting Up Your PHP Environment for Zoho CRM API Integration
Before making API calls, ensure your PHP environment is correctly configured:
- Install PHP 7.4 or later.
- Ensure the
cURL
extension is enabled in yourphp.ini
file. - Install Composer, a dependency manager for PHP, if you haven't already.
Installing Required PHP Libraries for Zoho CRM API
Use Composer to install the necessary libraries for making HTTP requests:
composer require guzzlehttp/guzzle
Writing PHP Code to Create or Update Contacts in Zoho CRM
Below is a sample PHP script to create or update contacts in Zoho CRM:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'your_access_token_here';
$apiUrl = 'https://www.zohoapis.com/crm/v3/Contacts';
$data = [
'data' => [
[
'First_Name' => 'John',
'Last_Name' => 'Doe',
'Email' => 'john.doe@example.com',
'Phone' => '1234567890'
]
]
];
$response = $client->request('POST', $apiUrl, [
'headers' => [
'Authorization' => 'Zoho-oauthtoken ' . $accessToken,
'Content-Type' => 'application/json'
],
'json' => $data
]);
echo $response->getBody();
Understanding the API Response and Handling Errors
After executing the API call, you should receive a response from Zoho CRM. Here's how to handle it:
- Check the HTTP status code to determine if the request was successful (e.g., 200 for success).
- Parse the JSON response to access contact details or error messages.
- Handle common error codes such as 400 (Bad Request) or 401 (Unauthorized) by checking the response body for details.
Verifying API Call Success in Zoho CRM Sandbox
To ensure your API call was successful, log in to your Zoho CRM sandbox account and verify the contact details:
- If creating a contact, check the Contacts module for the new entry.
- If updating, ensure the contact's information reflects the changes.
For more information on handling errors and API limits, refer to the Zoho CRM API Status Codes Documentation.
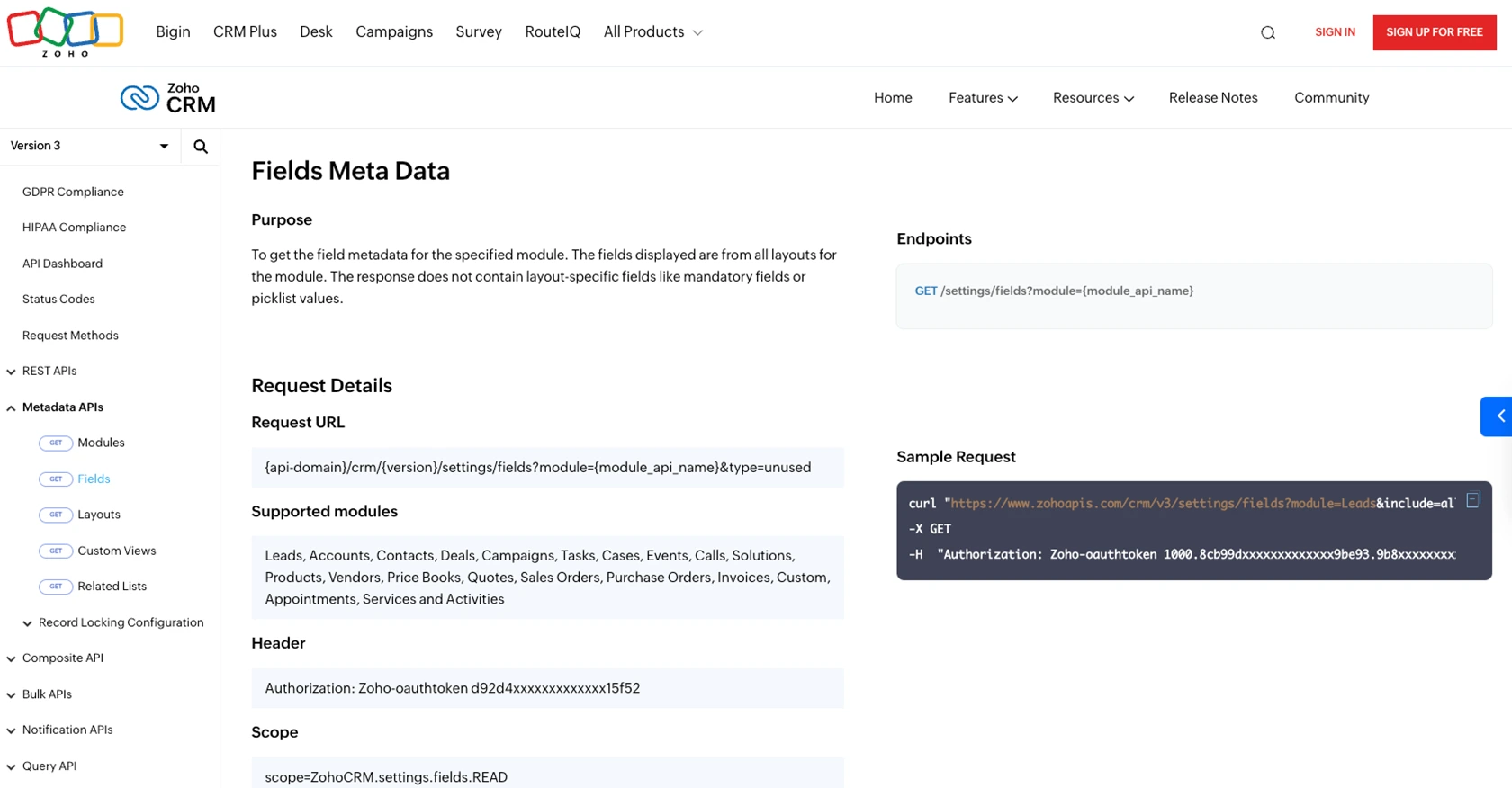
Best Practices for Zoho CRM API Integration
When integrating with the Zoho CRM API, it's essential to follow best practices to ensure secure and efficient interactions. Here are some recommendations:
- Securely Store Credentials: Always store your OAuth tokens securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Zoho CRM imposes API rate limits. Monitor your API usage and implement retry logic with exponential backoff to handle rate limit errors gracefully. For more details, refer to the Zoho CRM API Limits Documentation.
- Data Standardization: Ensure that data fields are standardized across your application and Zoho CRM to maintain consistency and avoid data discrepancies.
- Error Handling: Implement robust error handling to manage API errors effectively. Log errors for debugging and provide user-friendly messages where applicable.
Streamlining Integration with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Zoho CRM.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the integration complexities.
- Build Once, Use Everywhere: Develop integration logic once and apply it across multiple platforms, reducing redundancy.
- Enhance User Experience: Offer your customers a seamless and intuitive integration experience.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/search-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/insert-records.html
- https://www.zoho.com/crm/developer/docs/api/v3/update-records.html
Ready to get started?