How to Get Deals with the Pipedrive API in Python
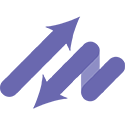
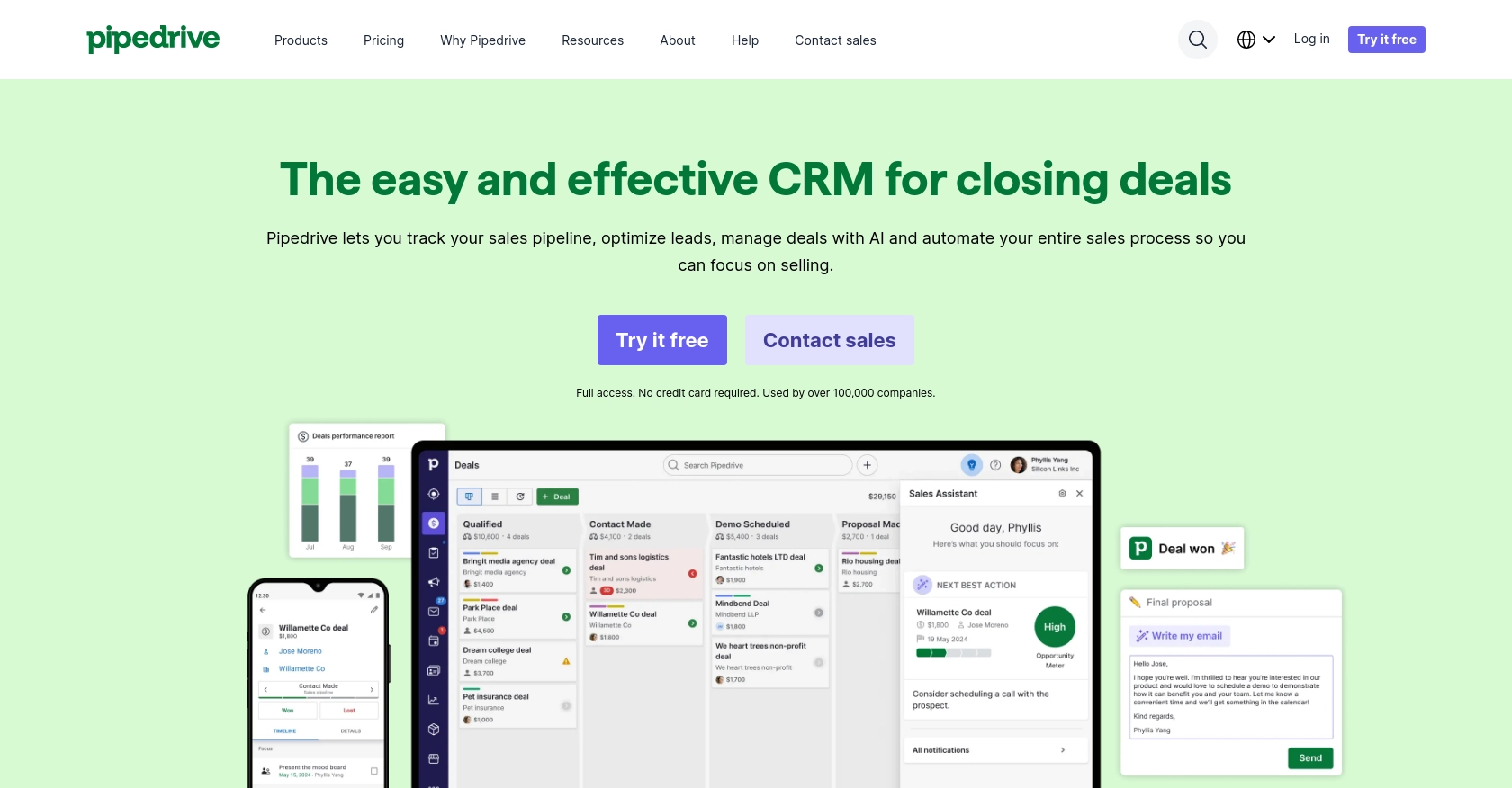
Introduction to Pipedrive CRM and API Integration
Pipedrive is a powerful sales CRM platform designed to help businesses manage their sales processes more effectively. With its intuitive interface and robust features, Pipedrive enables sales teams to track deals, manage contacts, and automate workflows, making it a popular choice for organizations aiming to enhance their sales efficiency.
Integrating with the Pipedrive API allows developers to access and manipulate sales data programmatically, providing opportunities to automate tasks and streamline operations. For example, a developer might use the Pipedrive API to retrieve deal information and integrate it with other business tools, enabling seamless data flow and enhanced sales insights.
This article will guide you through the process of using Python to interact with the Pipedrive API, specifically focusing on retrieving deals. By the end of this tutorial, you'll be equipped to efficiently access and manage deal data within Pipedrive using Python.
Setting Up Your Pipedrive Developer Sandbox Account
Before you can start interacting with the Pipedrive API, it's essential to set up a developer sandbox account. This environment allows you to test and develop your integrations without affecting live data, providing a risk-free space to experiment with the API.
Creating a Pipedrive Developer Sandbox Account
To begin, you'll need to create a developer sandbox account with Pipedrive. Follow these steps to get started:
- Visit the Pipedrive Developer Sandbox page.
- Fill out the necessary form to request access to a sandbox account.
- Once your request is approved, you'll receive access to a sandbox account, which mimics a regular Pipedrive company account but is limited to five seats.
Generating OAuth Credentials for Pipedrive API Access
Since the Pipedrive API uses OAuth 2.0 for authentication, you'll need to create an app to obtain the necessary credentials. Here's how:
- Log in to your Pipedrive sandbox account and navigate to the Developer Hub.
- Register your app by providing the required details, such as the app name and description.
- Once registered, you'll receive a client ID and client secret. These credentials are crucial for implementing the OAuth flow.
- Ensure your app has the appropriate scopes and permissions to access deal data.
For more detailed instructions, refer to the Pipedrive app creation guide.
Importing Sample Data into Your Pipedrive Sandbox
To effectively test your integration, you may want to import sample data into your sandbox account. Follow these steps:
- Download the template spreadsheets with sample data from the Pipedrive documentation.
- In your sandbox account, go to the "More" menu and select "Import data" followed by "From a spreadsheet."
- Upload the sample data spreadsheets to populate your sandbox with test data.
This setup will allow you to simulate real-world scenarios and ensure your integration functions as expected.
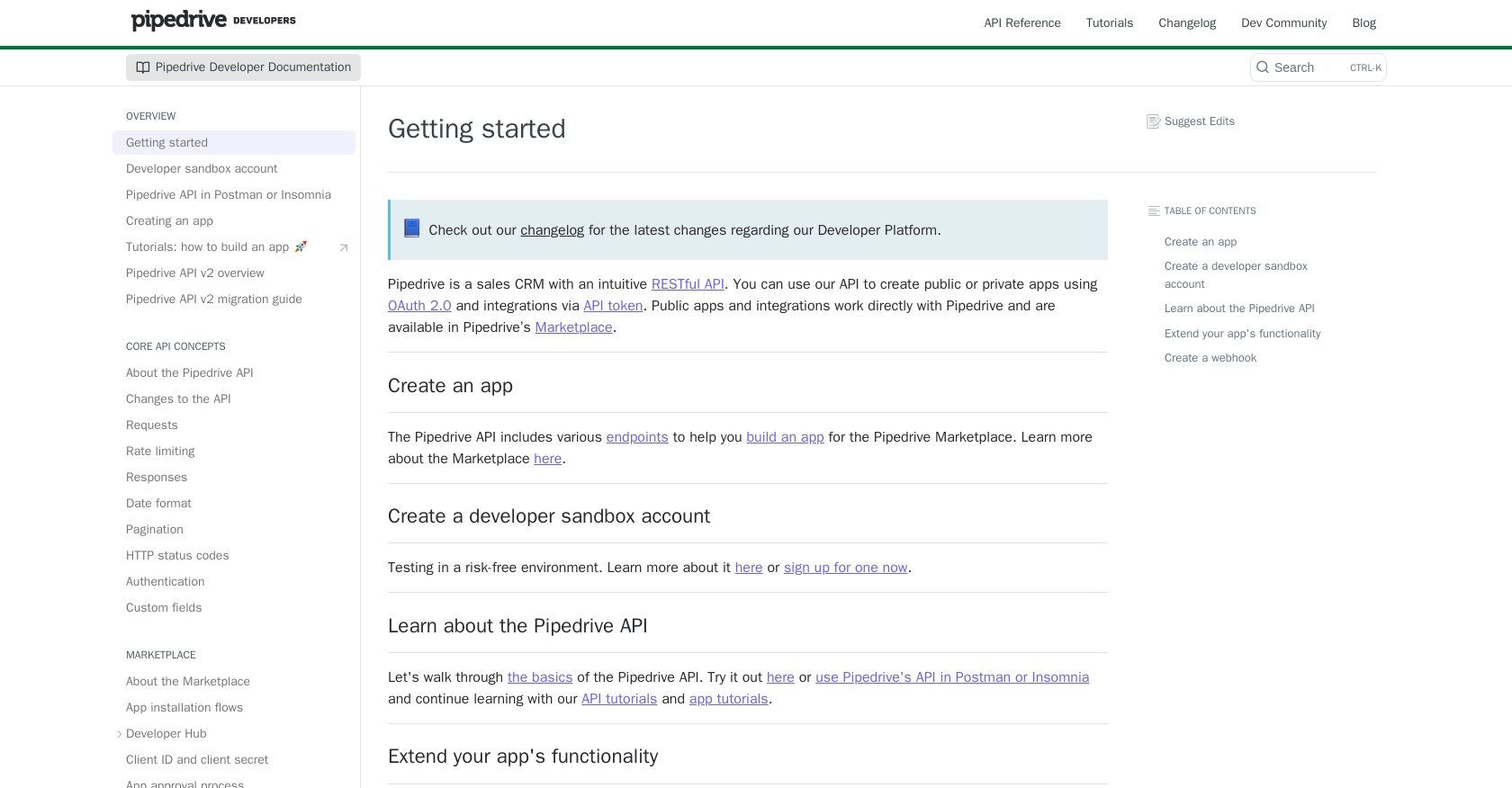
sbb-itb-96038d7
How to Make an API Call to Retrieve Deals from Pipedrive Using Python
To interact with the Pipedrive API and retrieve deal information, you'll need to set up your Python environment and make the necessary API calls. This section will guide you through the process, including setting up your Python environment, writing the code to fetch deals, and handling potential errors.
Setting Up Your Python Environment for Pipedrive API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Once you have these installed, open your terminal or command prompt and install the requests
library, which will be used to make HTTP requests:
pip install requests
Writing Python Code to Retrieve Deals from Pipedrive
Now that your environment is set up, you can write the Python code to interact with the Pipedrive API. Create a file named get_pipedrive_deals.py
and add the following code:
import requests
# Define the API endpoint and headers
endpoint = "https://api.pipedrive.com/v1/deals"
headers = {
"Authorization": "Bearer Your_Access_Token"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
deals = response.json().get("data", [])
for deal in deals:
print(f"Deal ID: {deal['id']}, Title: {deal['title']}")
else:
print(f"Failed to retrieve deals: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the access token obtained during the OAuth setup process. This code makes a GET request to the Pipedrive API to fetch all deals and prints their IDs and titles.
Verifying Successful API Requests and Handling Errors
After running the script, you should see a list of deals printed in your terminal. To verify that the request succeeded, you can log into your Pipedrive sandbox account and check the deals section to ensure the data matches.
If the request fails, the script will print an error message with the status code and response text. Common HTTP status codes include:
- 200 OK: The request was successful.
- 401 Unauthorized: Invalid access token.
- 429 Too Many Requests: Rate limit exceeded.
For more detailed information on error codes, refer to the Pipedrive HTTP status codes documentation.
By following these steps, you can efficiently retrieve deal data from Pipedrive using Python, enabling you to integrate this information into your applications and workflows.
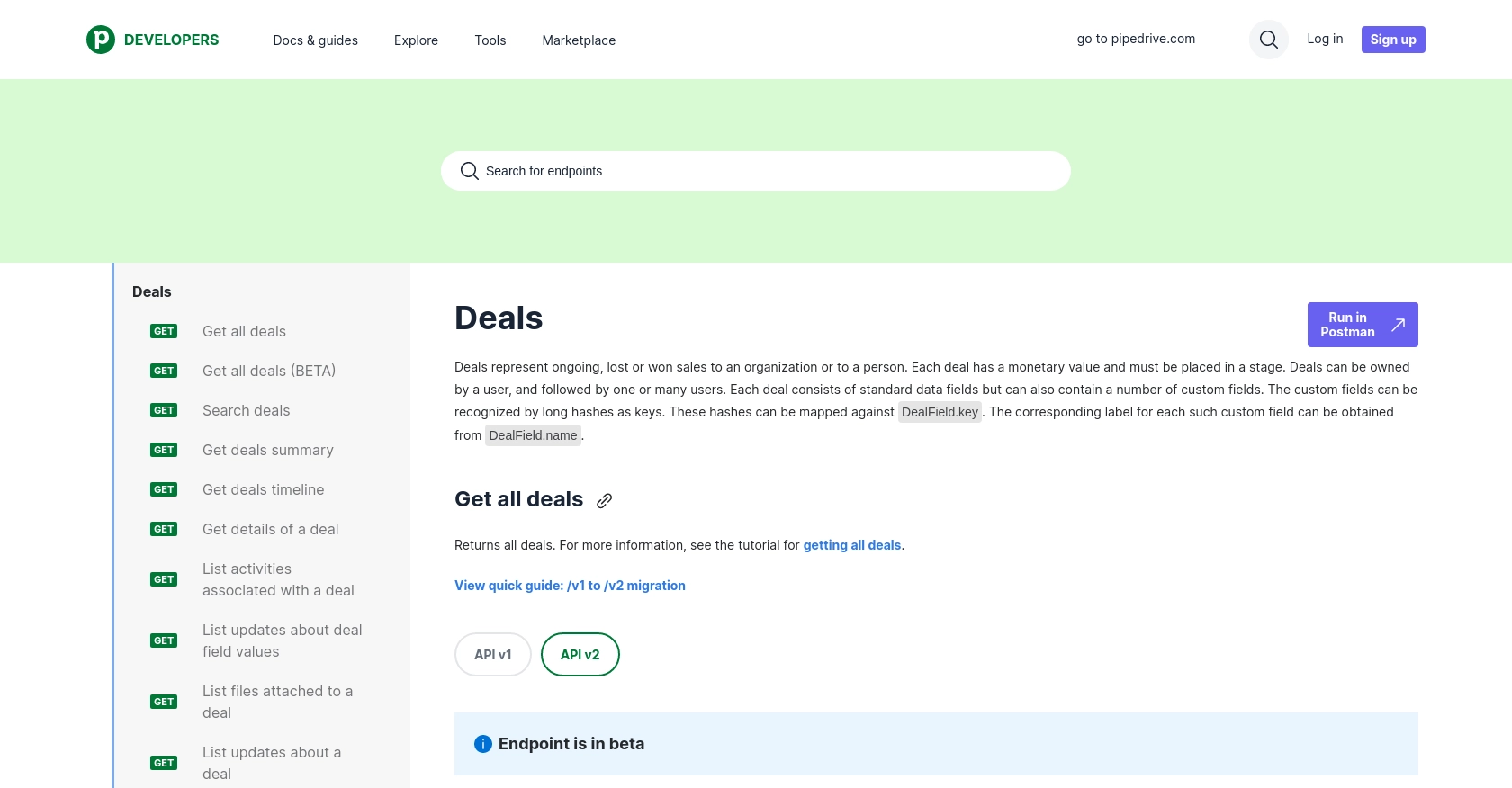
Conclusion and Best Practices for Pipedrive API Integration Using Python
Integrating with the Pipedrive API using Python provides a powerful way to automate and enhance your sales processes. By following the steps outlined in this guide, you can efficiently retrieve and manage deal data, enabling seamless integration with your existing business tools.
Best Practices for Secure and Efficient Pipedrive API Usage
- Securely Store Credentials: Always store your OAuth credentials, such as client ID and client secret, securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of Pipedrive's rate limits, which allow up to 80 requests per 2 seconds for OAuth apps. Implement error handling for HTTP 429 status codes and consider using exponential backoff strategies.
- Optimize Data Handling: Use pagination to efficiently handle large datasets. Pipedrive supports cursor-based pagination, which is ideal for traversing large amounts of data.
- Standardize Data Fields: When integrating with multiple systems, ensure that data fields are standardized to maintain consistency across platforms.
Leverage Endgrate for Streamlined Integration Solutions
While building custom integrations can be rewarding, it can also be time-consuming and complex. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product. With Endgrate, you can build once for each use case and connect to multiple platforms, including Pipedrive, effortlessly.
Explore how Endgrate can enhance your integration experience by visiting Endgrate's website and discover how it can save you time and resources.
Read More
- https://endgrate.com/provider/pipedrive
- https://pipedrive.readme.io/docs/getting-started
- https://pipedrive.readme.io/docs/developer-sandbox-account
- https://pipedrive.readme.io/docs/marketplace-creating-a-proper-app
- https://pipedrive.readme.io/docs/core-api-concepts-rate-limiting
- https://pipedrive.readme.io/docs/core-api-concepts-pagination
- https://pipedrive.readme.io/docs/core-api-concepts-http-status-codes
- https://pipedrive.readme.io/docs/core-api-concepts-custom-fields
- https://developers.pipedrive.com/docs/api/v1/Deals
- https://developers.pipedrive.com/docs/api/v1/DealFields
Ready to get started?