How to Get Contacts with the Brevo API in Python
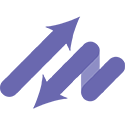
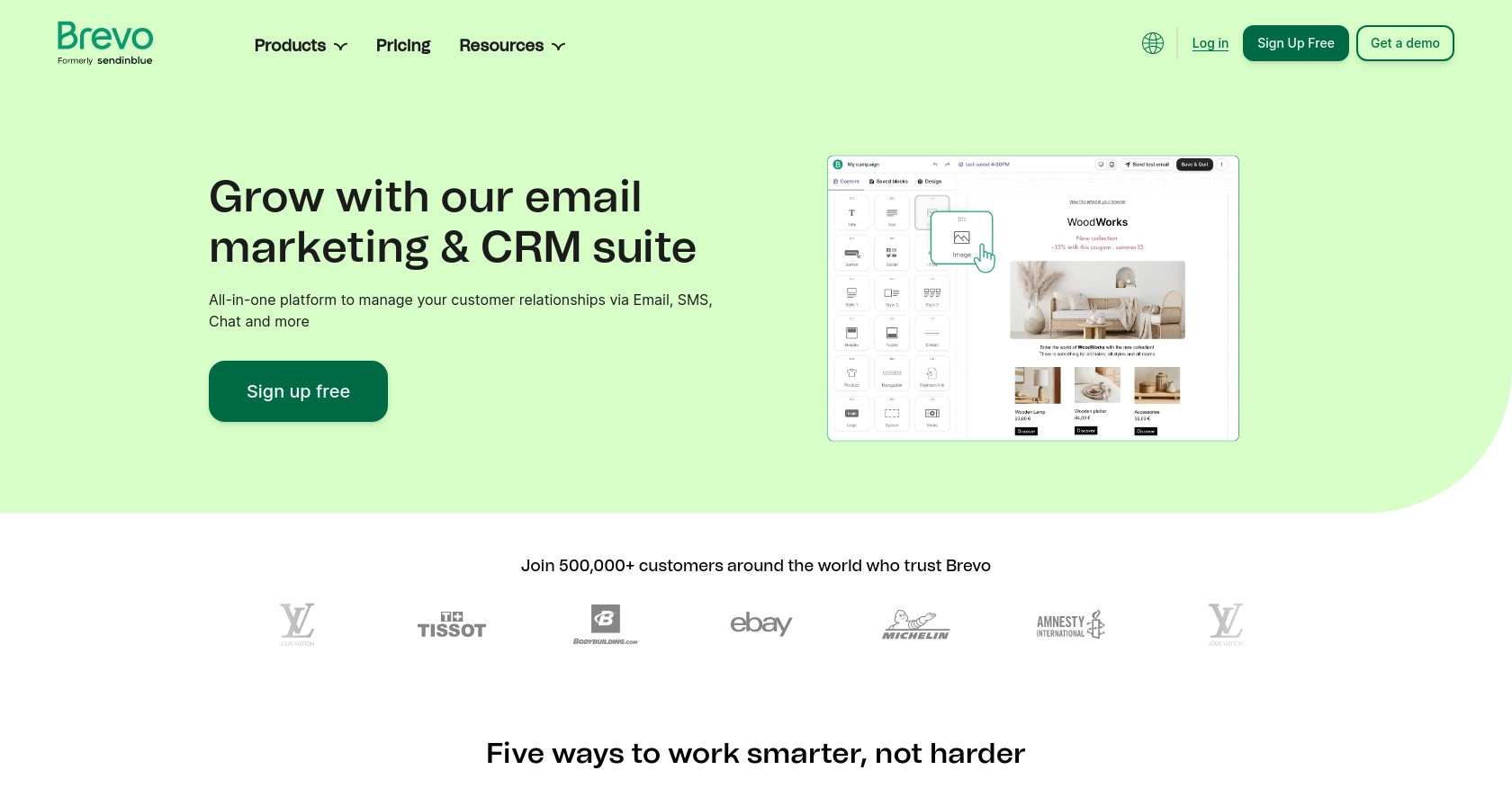
Introduction to Brevo API
Brevo is a powerful platform designed to enhance communication and marketing efforts through its comprehensive suite of tools. It offers solutions for email marketing, SMS campaigns, and contact management, making it an essential tool for businesses looking to streamline their marketing strategies.
Integrating with Brevo's API allows developers to efficiently manage and access contact data, enabling personalized communication and targeted marketing campaigns. For example, a developer might use the Brevo API to retrieve contact information and segment users based on their engagement levels, facilitating more effective email marketing campaigns.
Setting Up Your Brevo Test Account
Before you can start interacting with the Brevo API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Create a Brevo Account
If you don't already have a Brevo account, you can sign up for free on the Brevo website. Follow the instructions to complete your registration and verify your email address.
Generate Your Brevo API Key
Brevo uses API key authentication to authorize requests. Here's how you can generate your API key:
- Log in to your Brevo account.
- Click on your profile name in the top-right corner and select SMTP & API.
- Navigate to the API keys tab and click on Generate a new API key.
- Name your API key for easy identification and click Generate.
- Copy the generated API key and store it securely, as you'll need it for making API requests.
For more detailed instructions, refer to the Brevo documentation.
Verify Your API Key
Once you have your API key, you can verify it by making a simple API call to ensure it's working correctly. This step is crucial to confirm that your setup is complete and ready for further API interactions.
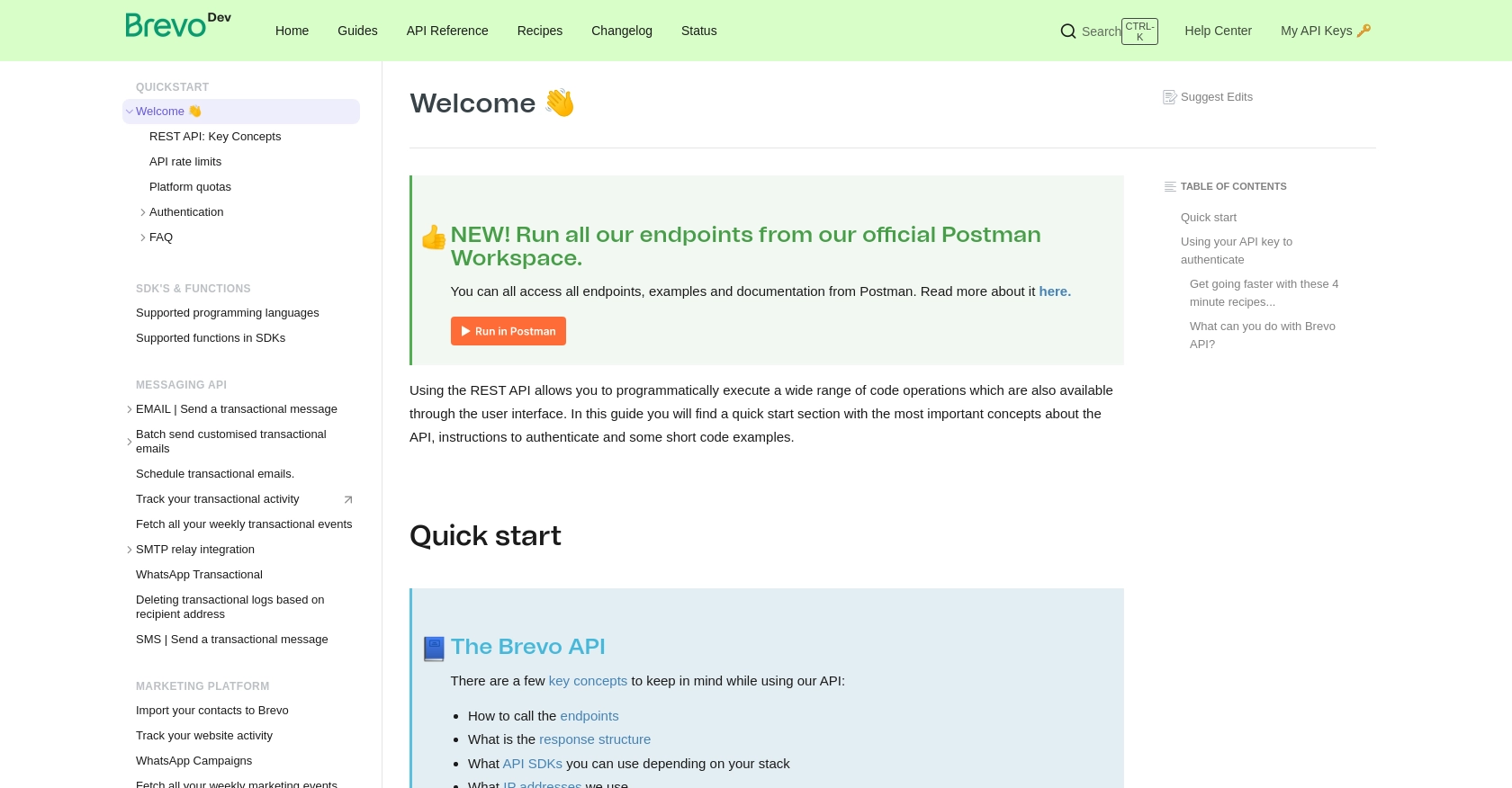
sbb-itb-96038d7
Making API Calls to Retrieve Contacts with Brevo API in Python
To interact with the Brevo API and retrieve contact information, you'll need to use Python. This section will guide you through the process of setting up your environment, writing the necessary code, and executing API calls to fetch contacts from Brevo.
Setting Up Your Python Environment for Brevo API Integration
Before you begin, ensure that you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need to install the sib-api-v3-sdk
package, which provides the necessary tools to interact with the Brevo API.
pip install sib-api-v3-sdk
Writing the Python Code to Fetch Contacts from Brevo
Once your environment is set up, you can write a Python script to make an API call to Brevo and retrieve contacts. Create a file named get_brevo_contacts.py
and add the following code:
from __future__ import print_function
import sib_api_v3_sdk
from sib_api_v3_sdk.rest import ApiException
from pprint import pprint
# Configure API key authorization
configuration = sib_api_v3_sdk.Configuration()
configuration.api_key['api-key'] = 'YOUR_API_KEY'
# Create an instance of the API class
api_instance = sib_api_v3_sdk.ContactsApi(sib_api_v3_sdk.ApiClient(configuration))
limit = 50 # Number of contacts per page
offset = 0 # Starting point for the list of contacts
try:
# Fetch contacts
api_response = api_instance.get_contacts(limit=limit, offset=offset)
pprint(api_response)
except ApiException as e:
print("Exception when calling ContactsApi->get_contacts: %s\n" % e)
Replace 'YOUR_API_KEY'
with the API key you generated earlier. This script sets up the API client, specifies the number of contacts to retrieve, and makes the API call to fetch the contacts.
Running the Python Script and Verifying the Output
To execute the script, run the following command in your terminal:
python get_brevo_contacts.py
If successful, the script will output a list of contacts from your Brevo account. You can verify the retrieved data by comparing it with the contacts listed in your Brevo dashboard.
Handling Errors and Understanding Brevo API Response Codes
While making API calls, you might encounter errors. The Brevo API provides specific error codes to help you diagnose issues:
- 400 Bad Request: Indicates a malformed request. Check your parameters and syntax.
- 429 Too Many Requests: You've exceeded the rate limit. Consider implementing a retry mechanism.
For more detailed error handling, refer to the Brevo API documentation.
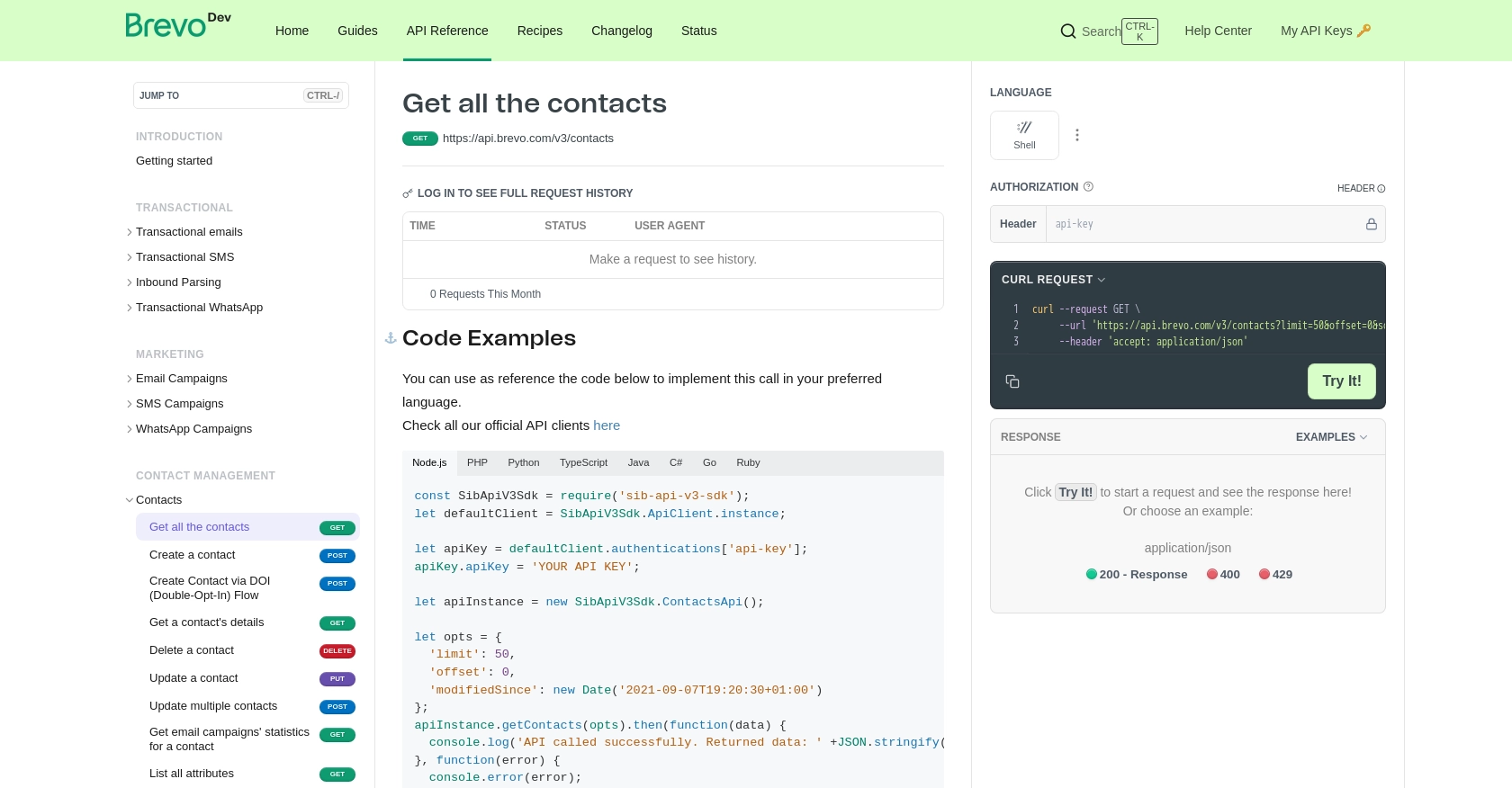
Conclusion and Best Practices for Using Brevo API in Python
Integrating with the Brevo API using Python provides a robust solution for managing contact data and enhancing your marketing strategies. By following the steps outlined in this guide, you can efficiently retrieve and manage contacts, enabling personalized and targeted communication efforts.
Best Practices for Secure and Efficient Brevo API Integration
- Secure API Key Storage: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of Brevo's rate limits to avoid disruptions. Implement a retry mechanism with exponential backoff to handle
429 Too Many Requests
errors gracefully. - Data Standardization: Ensure that the data retrieved from Brevo is standardized and transformed as needed to fit your application's requirements.
Enhance Your Integration Experience with Endgrate
While integrating with Brevo is straightforward, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint for all your integration needs. With Endgrate, you can:
- Save time and resources by outsourcing integration management.
- Build once for each use case, rather than multiple times for different platforms.
- Offer an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website.
Read More
Ready to get started?