Using the Google Meet API to Get Meeting Analytics in Javascript
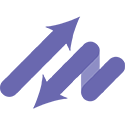
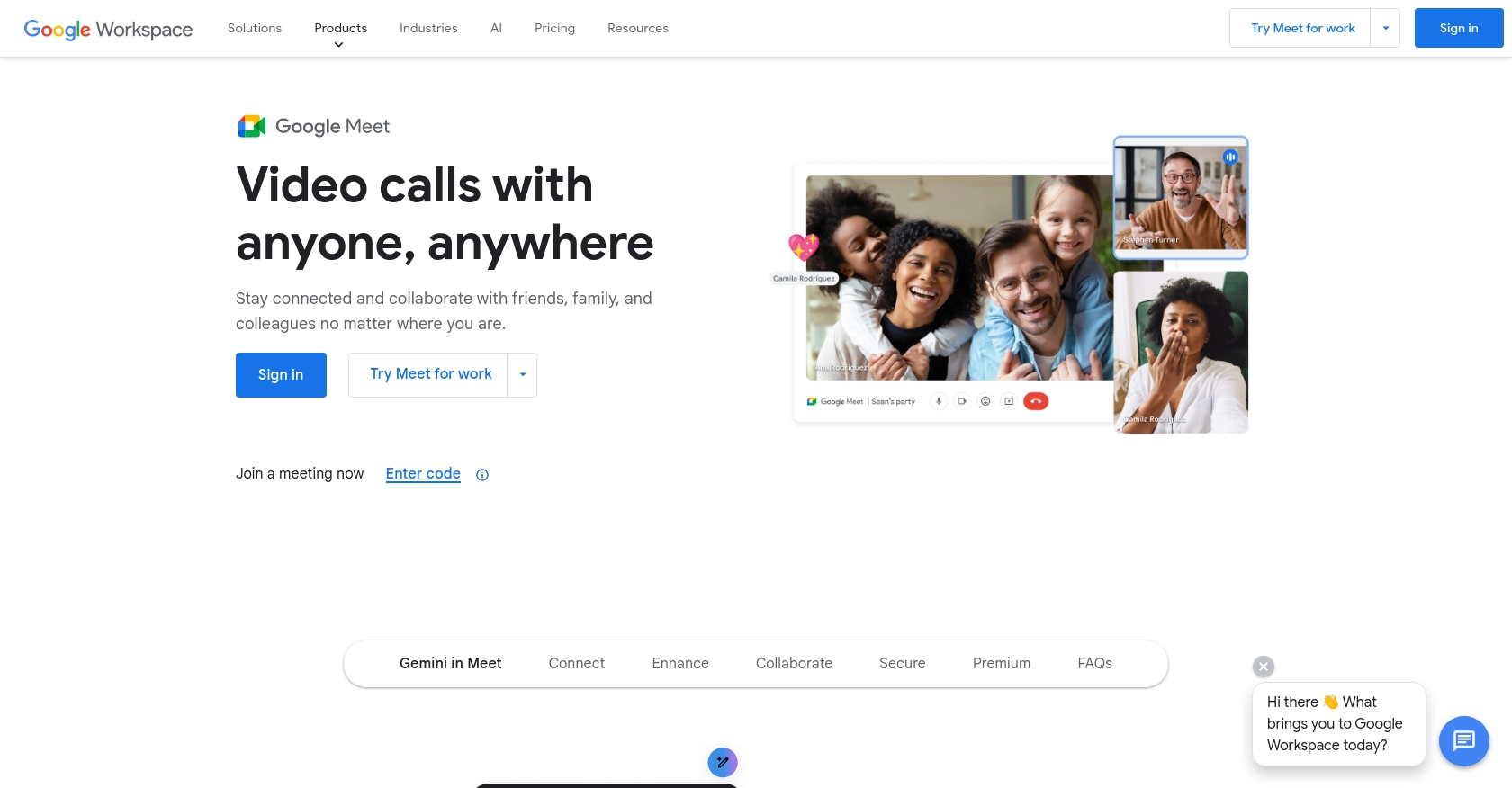
Introduction to Google Meet API
Google Meet is a robust video conferencing platform that is part of the Google Workspace suite. It offers seamless integration with other Google services, making it a popular choice for businesses and educational institutions looking to facilitate virtual meetings and collaborations.
Developers may want to integrate with the Google Meet API to access detailed meeting analytics, which can be invaluable for tracking participation and engagement. For example, a developer could use the Google Meet API to gather data on meeting attendance and duration, enabling organizations to analyze meeting effectiveness and optimize scheduling.
Setting Up Your Google Meet API Test Environment
Before you can start using the Google Meet API to gather meeting analytics, you'll need to set up a Google Cloud project and configure OAuth 2.0 authentication. This process will allow you to access the API securely and perform operations on behalf of users.
Create a Google Cloud Project for Google Meet API
- Go to the Google Cloud Console.
- Navigate to Menu > IAM & Admin > Create a Project.
- Enter a descriptive name for your project and click Create.
Enable Google Meet API in Your Project
- In the Google Cloud Console, go to Menu > APIs & Services > Library.
- Search for Google Meet API and click on it.
- Click Enable to activate the API for your project.
Configure OAuth Consent Screen for Google Meet API Access
- In the Google Cloud Console, navigate to APIs & Services > OAuth consent screen.
- Select the user type for your app and click Create.
- Fill out the app registration form and click Save and Continue.
- Add any necessary scopes, such as
https://www.googleapis.com/auth/meetings.space.readonly
, and click Save and Continue.
Create OAuth 2.0 Credentials for Google Meet API
- Go to APIs & Services > Credentials.
- Click Create Credentials > OAuth client ID.
- Select Web application as the application type.
- Enter a name for your OAuth client and add your authorized redirect URIs.
- Click Create to generate your client ID and client secret.
Once you have completed these steps, you will have a Google Cloud project set up with the necessary credentials to access the Google Meet API. Keep your client ID and client secret secure, as they are essential for authenticating API requests.
For more detailed instructions, refer to the official Google documentation: Create a Google Cloud Project, Enable Google Workspace APIs, Configure OAuth Consent, and Create Access Credentials.
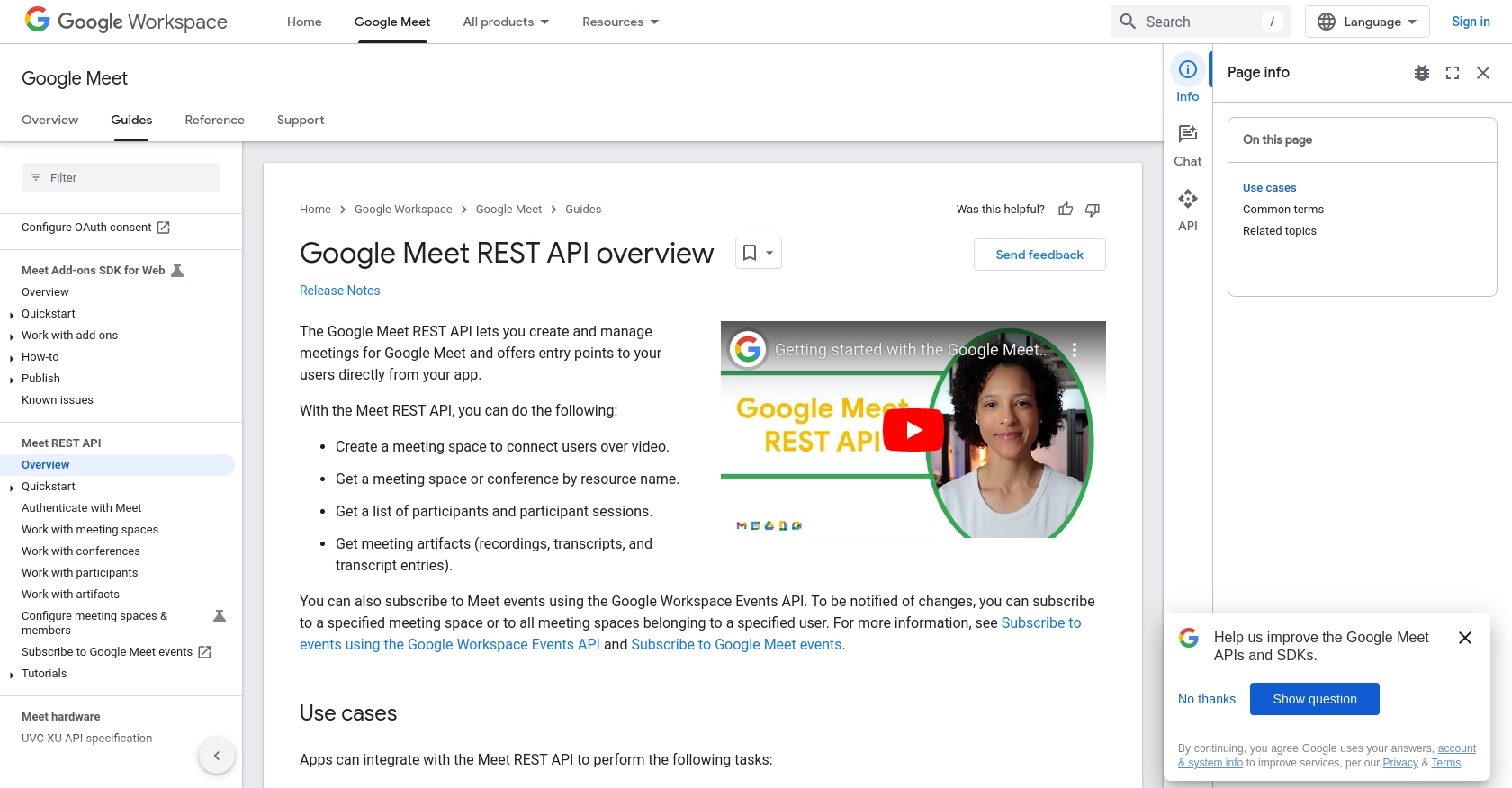
sbb-itb-96038d7
How to Make Google Meet API Calls Using JavaScript
To interact with the Google Meet API using JavaScript, you'll need to ensure you have the right environment set up and understand the necessary dependencies. This section will guide you through making API calls to retrieve meeting analytics, such as participant data and meeting duration.
Setting Up Your JavaScript Environment for Google Meet API
Before making API calls, ensure you have Node.js installed on your machine. You can download it from the official Node.js website. Additionally, you'll need the googleapis
package, which provides access to Google APIs.
npm install googleapis
Authenticating with Google Meet API Using OAuth 2.0
To authenticate your requests, use the OAuth 2.0 credentials you created earlier. The following example demonstrates how to set up authentication and make a request to the Google Meet API.
const { google } = require('googleapis');
const OAuth2 = google.auth.OAuth2;
// Replace with your client ID and client secret
const oauth2Client = new OAuth2(
'YOUR_CLIENT_ID',
'YOUR_CLIENT_SECRET',
'YOUR_REDIRECT_URI'
);
// Set the credentials
oauth2Client.setCredentials({
access_token: 'YOUR_ACCESS_TOKEN',
refresh_token: 'YOUR_REFRESH_TOKEN',
scope: 'https://www.googleapis.com/auth/meetings.space.readonly',
token_type: 'Bearer',
expiry_date: 1234567890
});
// Initialize the Google Meet API
const meet = google.meet({ version: 'v1', auth: oauth2Client });
// Function to get meeting analytics
async function getMeetingAnalytics() {
try {
const response = await meet.spaces.list({
pageSize: 10,
});
console.log('Meeting Analytics:', response.data);
} catch (error) {
console.error('Error fetching meeting analytics:', error);
}
}
getMeetingAnalytics();
Understanding the Google Meet API Response
When you make a successful API call, you can expect a response containing meeting analytics data. This data may include details about participants, meeting duration, and other relevant metrics. Verify the data by checking your Google Cloud project or Google Meet dashboard.
Handling Errors and Google Meet API Error Codes
It's crucial to handle potential errors when making API calls. Common errors include invalid credentials, insufficient permissions, or exceeding rate limits. Refer to the Google Meet API documentation for detailed error code explanations and troubleshooting tips.
By following these steps, you can effectively interact with the Google Meet API using JavaScript to gather valuable meeting analytics. Ensure you handle authentication securely and manage API responses and errors appropriately.
Conclusion and Best Practices for Using Google Meet API with JavaScript
Integrating with the Google Meet API using JavaScript provides developers with powerful tools to access and analyze meeting data. By following the steps outlined in this guide, you can effectively gather valuable insights into meeting participation and engagement, enhancing your organization's ability to optimize virtual collaboration.
Best Practices for Secure and Efficient Google Meet API Integration
- Securely Store Credentials: Ensure that your OAuth 2.0 credentials, such as client ID and client secret, are stored securely and not exposed in your codebase.
- Handle Rate Limiting: Be aware of Google Meet API rate limits and implement strategies to handle them gracefully. For example, if the API allows 100 requests per minute, ensure your application does not exceed this limit to avoid disruptions.
- Transform and Standardize Data: When processing meeting analytics data, consider transforming and standardizing fields to match your organization's data models, making it easier to integrate with other systems.
- Monitor API Usage: Regularly monitor your API usage and performance metrics in the Google Cloud Console to ensure optimal operation and identify any potential issues early.
Streamline Your Integration Process with Endgrate
While building integrations with the Google Meet API can be rewarding, it can also be time-consuming and complex. Endgrate offers a solution to simplify this process by providing a unified API endpoint that connects to multiple platforms, including Google Meet. By using Endgrate, you can save time and resources, allowing you to focus on your core product development while ensuring a seamless integration experience for your customers.
Explore how Endgrate can enhance your integration capabilities by visiting Endgrate today.
Read More
- https://endgrate.com/provider/googlemeet
- https://developers.google.com/meet/api/guides/overview
- https://developers.google.com/meet/api/guides/authenticate-authorize
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
Ready to get started?