Using the Sugar Market API to Create Or Update Contacts in Javascript
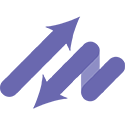
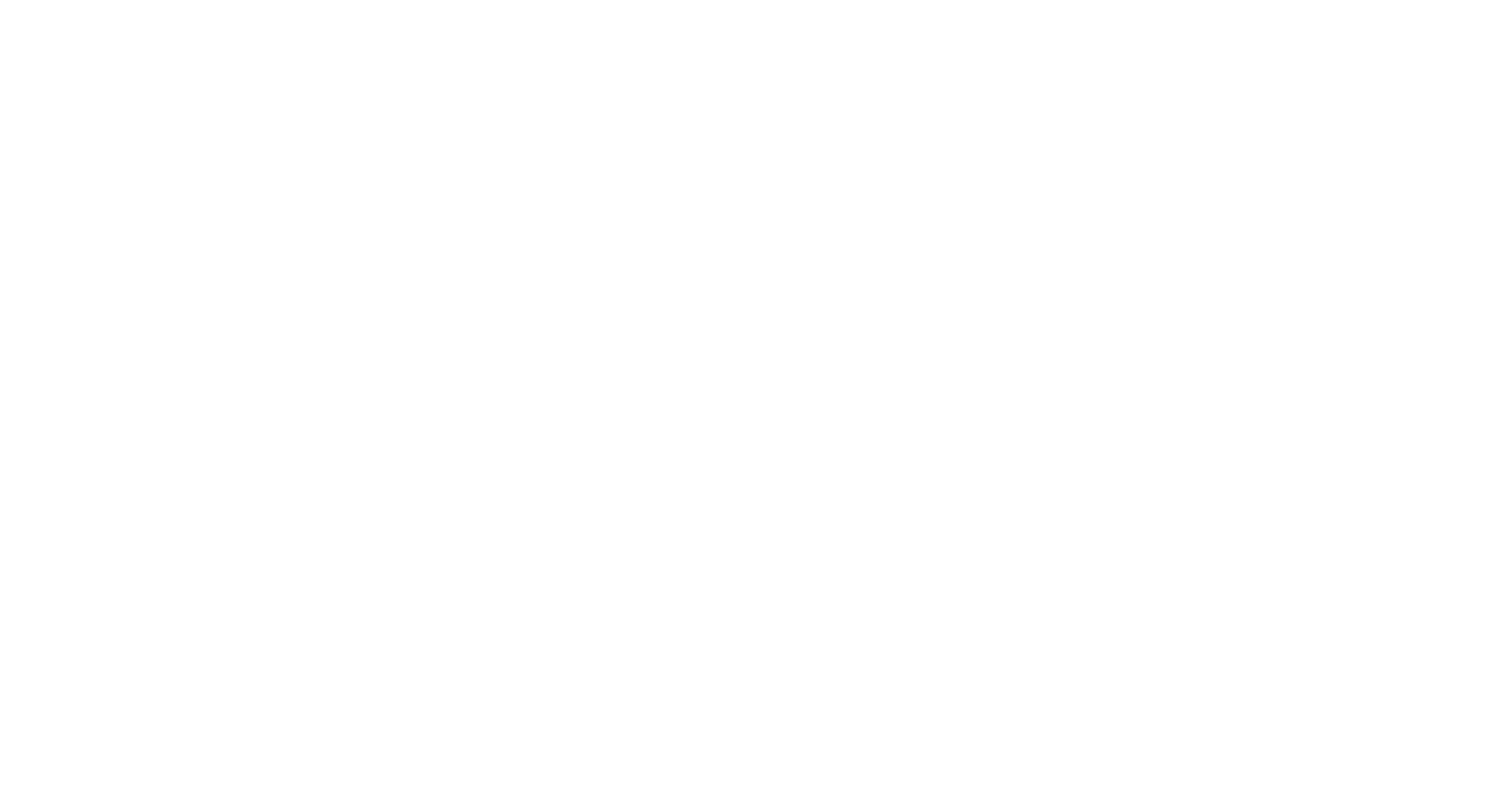
Introduction to Sugar Market API
Sugar Market is a robust marketing automation platform designed to enhance marketing efforts for B2B organizations. It offers a comprehensive suite of tools for email marketing, lead nurturing, and campaign management, enabling businesses to streamline their marketing processes and drive growth.
Integrating with the Sugar Market API allows developers to efficiently manage and update contact information, facilitating seamless marketing operations. For example, you can use the API to automatically update contact details from a CRM system, ensuring that your marketing campaigns are always targeting the right audience.
Setting Up Your Sugar Market Test Account
Before you can start using the Sugar Market API to create or update contacts, you'll need to set up a test or sandbox account. This will allow you to safely experiment with the API without affecting your live data.
Creating a Sugar Market Sandbox Account
If you don't already have a Sugar Market account, you can sign up for a free trial or request access to a sandbox environment. Visit the Sugar Market website to get started.
Follow the instructions to create your account. Once your account is set up, you'll have access to the sandbox environment where you can test API calls.
Generating API Credentials for Sugar Market
To interact with the Sugar Market API, you'll need to generate API credentials. These credentials will include a client ID and client secret, which are necessary for authenticating your API requests.
- Log in to your Sugar Market account.
- Navigate to the API settings section, typically found under the integrations or developer tools menu.
- Create a new API application by providing the necessary details, such as the application name and description.
- Once the application is created, you'll receive a client ID and client secret. Make sure to store these securely, as you'll need them to authenticate your API requests.
Authenticating with Sugar Market API
Sugar Market uses a custom authentication method. To authenticate your API requests, you'll need to include the client ID and client secret in your request headers.
// Example of setting headers for authentication
const headers = {
"Content-Type": "application/json",
"Client-ID": "your_client_id",
"Client-Secret": "your_client_secret"
};
Replace your_client_id
and your_client_secret
with the credentials you obtained earlier.
With your sandbox account set up and your API credentials ready, you're now prepared to start making API calls to create or update contacts in Sugar Market using JavaScript.
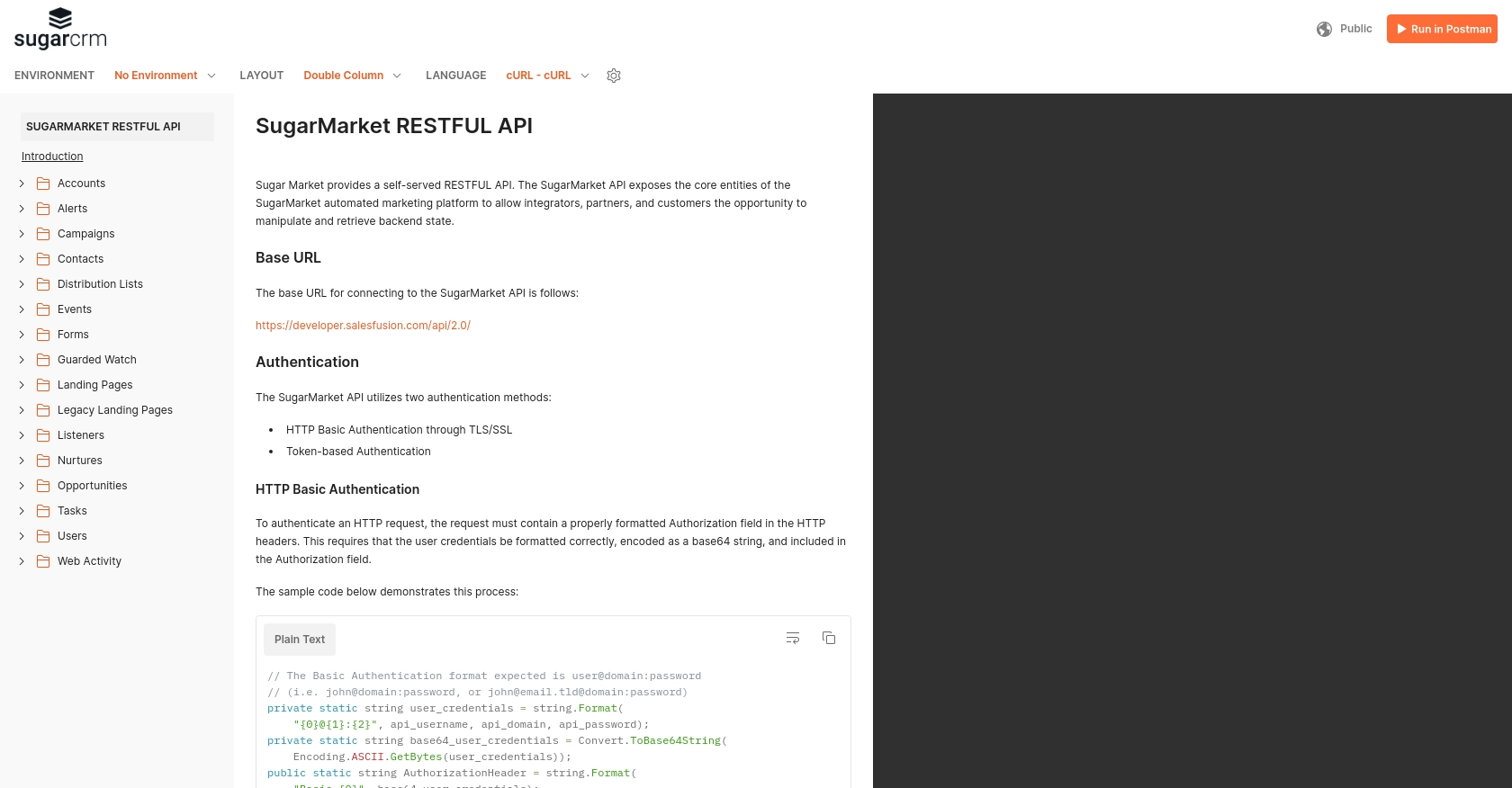
sbb-itb-96038d7
Making API Calls to Create or Update Contacts in Sugar Market Using JavaScript
To interact with the Sugar Market API for creating or updating contacts, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the necessary code, and handling responses effectively.
Setting Up Your JavaScript Environment for Sugar Market API
Before you begin, ensure you have a modern JavaScript environment set up. You can use Node.js or a browser-based environment to execute your code. Make sure you have access to the fetch
API or any HTTP client library like Axios for making HTTP requests.
Installing Necessary Dependencies
If you're using Node.js, you might want to install Axios for easier HTTP requests:
npm install axios
Writing JavaScript Code to Create or Update Contacts in Sugar Market
Below is an example of how you can use JavaScript to create or update contacts in Sugar Market:
const axios = require('axios');
// Define the API endpoint
const apiUrl = 'https://api.sugarmarket.com/v1/contacts';
// Set up the request headers with authentication
const headers = {
'Content-Type': 'application/json',
'Client-ID': 'your_client_id',
'Client-Secret': 'your_client_secret'
};
// Define the contact data to be created or updated
const contactData = {
email: 'example@sugarmarket.com',
firstName: 'John',
lastName: 'Doe'
};
// Function to create or update a contact
async function createOrUpdateContact() {
try {
const response = await axios.post(apiUrl, contactData, { headers });
console.log('Contact created or updated successfully:', response.data);
} catch (error) {
console.error('Error creating or updating contact:', error.response ? error.response.data : error.message);
}
}
// Execute the function
createOrUpdateContact();
Replace your_client_id
and your_client_secret
with the credentials you obtained from your Sugar Market account. The contactData
object should contain the contact information you wish to create or update.
Verifying API Call Success in Sugar Market Sandbox
After executing the code, you can verify the success of your API call by checking the Sugar Market sandbox environment. The contact should appear in the list of contacts, reflecting the data you provided.
Handling Errors and Error Codes
It's crucial to handle errors gracefully. The example code above logs errors to the console, but you might want to implement more robust error handling depending on your application's needs. Check the Sugar Market API documentation for specific error codes and their meanings.
Conclusion and Best Practices for Using Sugar Market API with JavaScript
Integrating with the Sugar Market API using JavaScript provides a powerful way to automate and enhance your marketing processes. By following the steps outlined in this guide, you can efficiently create or update contacts, ensuring your marketing campaigns are always targeting the right audience.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your client ID and client secret securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the Sugar Market API. Implement retry logic or backoff strategies to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that the data fields you send to the API are standardized and validated to prevent errors and maintain data integrity.
- Error Handling: Implement robust error handling to manage API response errors effectively. Refer to the Sugar Market API documentation for specific error codes and their meanings.
Streamline Your Integrations with Endgrate
While integrating with the Sugar Market API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Sugar Market.
By using Endgrate, you can save time and resources, allowing you to focus on your core product development. With a single API endpoint, you can manage multiple integrations efficiently, providing an intuitive experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?