Using the Sap Business One API to Create or Update Vendors in Python
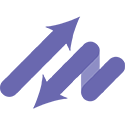
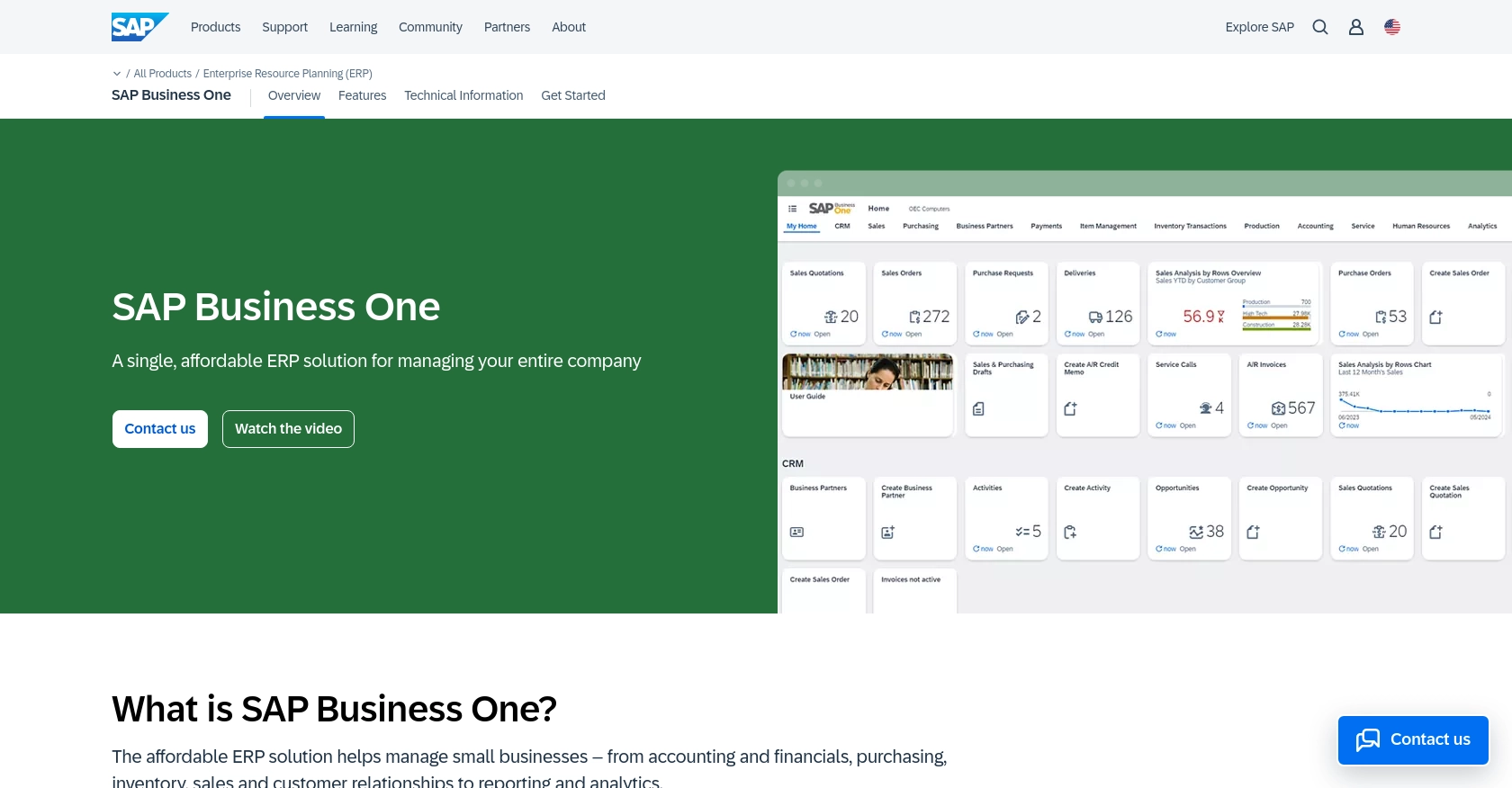
Introduction to SAP Business One API
SAP Business One is a comprehensive enterprise resource planning (ERP) solution tailored for small to medium-sized businesses. It offers a wide range of functionalities, including financial management, sales, customer relationship management, and supply chain operations, all integrated into a single platform.
Developers often seek to integrate with the SAP Business One API to streamline business processes and enhance operational efficiency. By connecting with this API, developers can automate tasks such as creating or updating vendor records, which is crucial for maintaining accurate supplier information and ensuring smooth procurement operations.
For example, a developer might use the SAP Business One API to automatically update vendor details when changes occur in an external supplier database, ensuring that the ERP system always reflects the most current information.
Setting Up Your SAP Business One Test or Sandbox Account
Before you can start integrating with the SAP Business One API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your live data.
Creating a SAP Business One Sandbox Account
To begin, you'll need access to a SAP Business One sandbox environment. If your organization already uses SAP Business One, you can request access to a sandbox from your system administrator. If not, consider reaching out to SAP or a certified partner to inquire about trial or demo environments.
Configuring OAuth-Based Authentication for SAP Business One API
SAP Business One uses a custom authentication method. Follow these steps to configure your authentication:
- Log in to your SAP Business One sandbox account.
- Navigate to the Service Layer settings. This is where you'll manage API access.
- Create a new application to generate your Client ID and Client Secret. These credentials are essential for authenticating API requests.
- Ensure that your application has the necessary permissions to create or update vendor records.
For more detailed instructions, refer to the SAP Business One Service Layer documentation.
Generating API Keys for SAP Business One
In addition to OAuth credentials, you may need an API key for certain operations. Follow these steps to generate an API key:
- Access the API Management section in your SAP Business One sandbox.
- Generate a new API key and associate it with your application.
- Store the API key securely, as it will be used to authenticate your API requests.
With your sandbox account and authentication credentials set up, you're ready to start making API calls to create or update vendors in SAP Business One using Python.
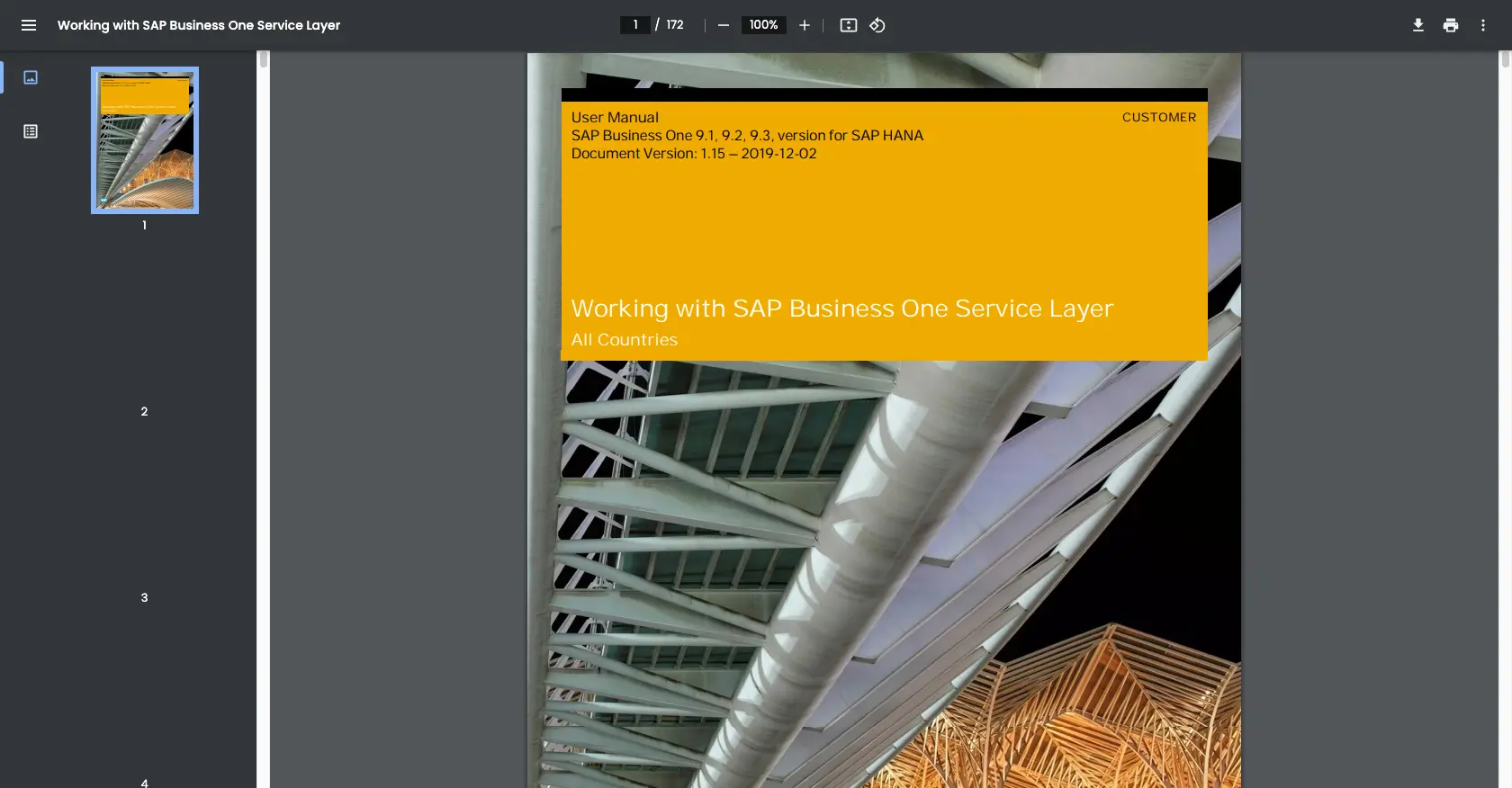
sbb-itb-96038d7
How to Make API Calls to Create or Update Vendors in SAP Business One Using Python
To interact with the SAP Business One API for creating or updating vendor records, you'll need to use Python. This section will guide you through the necessary steps, including setting up your Python environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for SAP Business One API Integration
Before you begin coding, ensure that you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Once you have these installed, open your terminal or command prompt and install the requests
library, which will be used to make HTTP requests:
pip install requests
Writing Python Code to Create or Update Vendors in SAP Business One
Now that your environment is ready, you can write the Python code to interact with the SAP Business One API. Create a file named sap_b1_vendor.py
and add the following code:
import requests
# Define the API endpoint and headers
endpoint = "https://your-sap-b1-instance.com/b1s/v1/Vendors"
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Token"
}
# Define the vendor data
vendor_data = {
"CardCode": "V001",
"CardName": "New Vendor",
"CardType": "Supplier"
}
# Make a POST request to create a new vendor
response = requests.post(endpoint, json=vendor_data, headers=headers)
# Check if the request was successful
if response.status_code == 201:
print("Vendor created successfully.")
else:
print("Failed to create vendor:", response.json())
Replace Your_Token
with the token you obtained during the authentication setup. The above code sends a POST request to create a new vendor. If the vendor already exists and you want to update it, you can use the PUT method instead.
Verifying API Request Success in SAP Business One Sandbox
After running your script, you should verify the success of your API request by checking the SAP Business One sandbox environment. Navigate to the vendor section and confirm that the new or updated vendor appears as expected.
Handling Errors and Error Codes in SAP Business One API
When making API calls, it's crucial to handle potential errors gracefully. The SAP Business One API may return various error codes, such as:
- 400 Bad Request: The request was invalid. Check your data format and required fields.
- 401 Unauthorized: Authentication failed. Verify your token and permissions.
- 500 Internal Server Error: An error occurred on the server. Try again later or contact support.
In your code, you can handle these errors by checking the response status code and implementing appropriate error-handling logic.
Conclusion and Best Practices for Integrating with SAP Business One API
Integrating with the SAP Business One API to create or update vendor records can significantly enhance your business operations by automating and streamlining supplier management processes. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate your requests, and handle potential errors.
Best Practices for SAP Business One API Integration
- Securely Store Credentials: Always store your API credentials, such as tokens and API keys, securely. Consider using environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the SAP Business One API. Implement logic to handle retries or backoff strategies to avoid exceeding these limits.
- Standardize Data Fields: Ensure that your data fields are consistent and standardized across your systems to prevent data discrepancies and improve data quality.
- Monitor API Usage: Regularly monitor your API usage and logs to identify any anomalies or issues that may arise during integration.
Streamline Your Integration Process with Endgrate
While integrating with SAP Business One API can be a powerful way to enhance your business processes, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Save time and resources by outsourcing integrations and ensuring a seamless connection with platforms like SAP Business One.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?