How to Export Data as CSV with the CSV API in Javascript
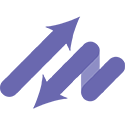
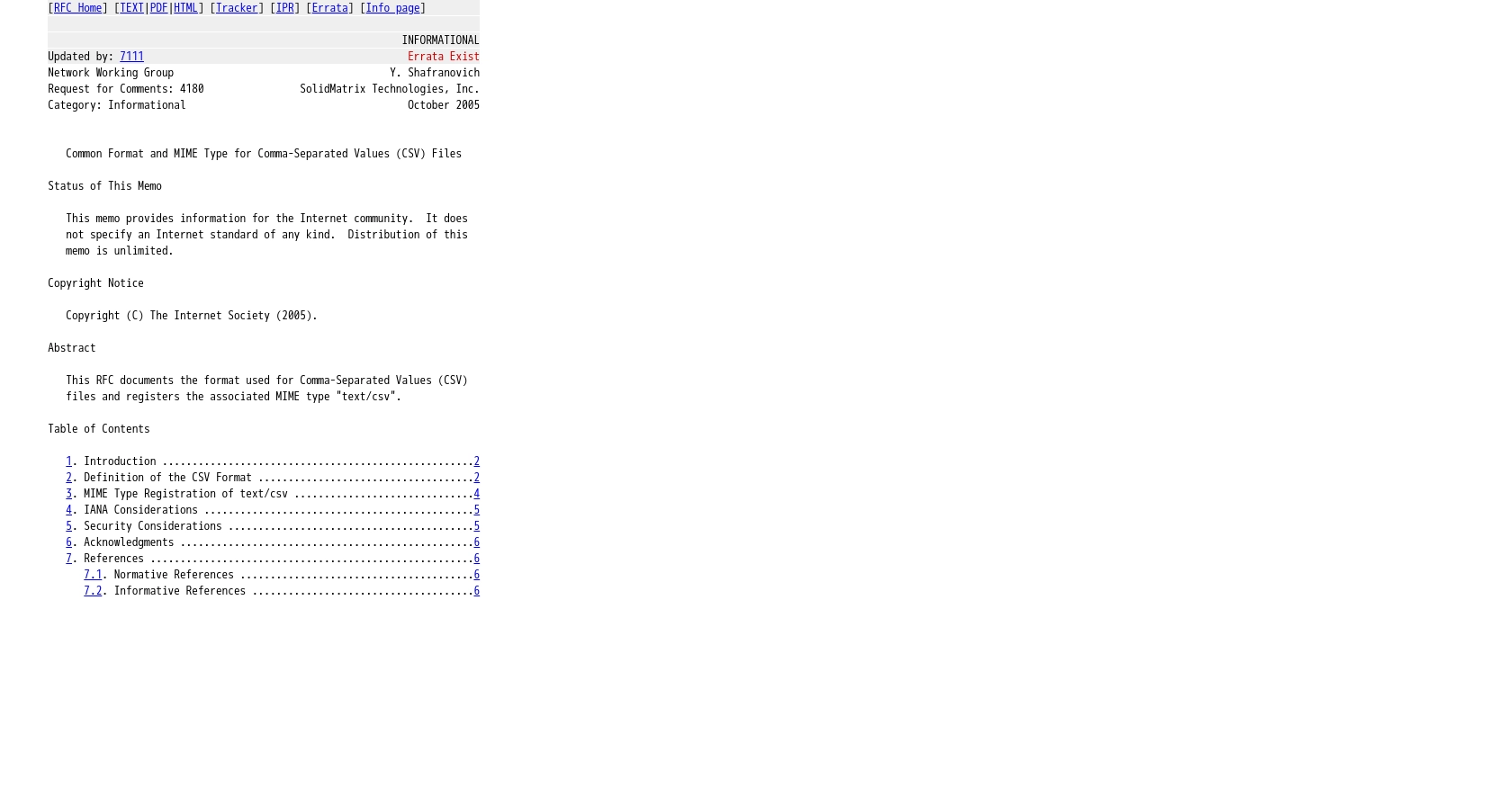
Introduction to CSV Integration
The CSV format is a widely used method for storing and exchanging data, especially in business and technical environments. It allows for easy manipulation of data in a tabular format, making it a popular choice for data export and import tasks.
For developers working with B2B SaaS products, integrating CSV functionality can streamline data handling processes. By using a CSV API, developers can automate the export of data into CSV files, which can then be used for reporting, data analysis, or migration to other systems.
For example, a developer might want to export user data from a SaaS application to a CSV file for further analysis in a spreadsheet program. This article will guide you through the process of exporting data as CSV using the CSV API in JavaScript, providing a seamless way to handle data exports in your applications.
Setting Up Your CSV API Test Environment
Before you can start exporting data as CSV using the CSV API in JavaScript, you'll need to set up a test environment. This involves creating a sandbox account and configuring the necessary settings to interact with the CSV API effectively.
Creating a Sandbox Account for CSV Integration
To begin, you'll need to create a sandbox account that allows you to test the CSV integration without affecting live data. This is crucial for ensuring that your integration works as expected before deploying it to a production environment.
- Visit the CSV API provider's website and sign up for a free trial or sandbox account.
- Follow the registration process, providing the necessary information to create your account.
- Once registered, log in to your sandbox account to access the CSV API dashboard.
Configuring CSV API Authentication
Since the CSV integration uses a custom authentication method, you'll need to configure it to allow your application to interact with the API securely. Follow these steps to set up authentication:
- Navigate to the API settings section within your sandbox account dashboard.
- Locate the authentication settings and generate the necessary credentials, such as API keys or tokens, if applicable.
- Ensure that these credentials are stored securely, as they will be used to authenticate your API requests.
Uploading and Downloading CSV Files
The CSV API requires a custom frontend to handle CSV file uploads and downloads. Here's how you can set this up:
- Use CSV libraries to load CSV data into your database, allowing for seamless data manipulation.
- Implement a frontend interface that enables users to upload CSV files in chunks, ensuring efficient data processing.
- Configure the frontend to allow CSV files to be downloaded, providing users with easy access to exported data.
With your sandbox account and authentication configured, you're ready to start exporting data as CSV using the CSV API in JavaScript. This setup ensures a smooth integration process, allowing you to focus on building robust data export functionalities in your application.
Executing CSV API Calls with JavaScript
To effectively export data as CSV using the CSV API in JavaScript, you'll need to understand the language's capabilities and ensure your environment is properly set up. JavaScript is a versatile language widely used for web development, making it an excellent choice for handling CSV data operations.
Setting Up Your JavaScript Environment for CSV API Integration
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine, as it provides the runtime environment for executing JavaScript outside the browser.
- npm (Node Package Manager) to manage dependencies and libraries.
Once you have Node.js and npm installed, you can proceed to install necessary libraries for handling HTTP requests and CSV data.
Installing Required JavaScript Libraries
To interact with the CSV API, you'll need to install libraries that facilitate HTTP requests and CSV data manipulation. Use the following command to install Axios and a CSV parser library:
npm install axios csv-parser
Making a CSV API Call to Export Data
With your environment set up, you can now write the JavaScript code to make an API call to export data as CSV. Create a file named exportCsv.js
and add the following code:
const axios = require('axios');
const fs = require('fs');
// Define the API endpoint and headers
const endpoint = 'https://api.example.com/export';
const headers = {
'Authorization': 'Bearer Your_Token',
'Content-Type': 'application/json'
};
// Function to export data as CSV
async function exportDataAsCsv() {
try {
const response = await axios.get(endpoint, { headers });
if (response.status === 200) {
// Write the CSV data to a file
fs.writeFileSync('exported_data.csv', response.data);
console.log('Data exported successfully as CSV.');
} else {
console.error('Failed to export data:', response.status, response.statusText);
}
} catch (error) {
console.error('Error making API call:', error.message);
}
}
exportDataAsCsv();
Replace Your_Token
with the actual token obtained from your CSV API authentication setup.
Verifying Successful CSV Data Export
After running the script, check the directory for the exported_data.csv
file. Open it to verify that the data has been exported correctly. If the file contains the expected data, your API call was successful.
Handling Errors and Debugging CSV API Calls
When making API calls, it's crucial to handle potential errors gracefully. The code above includes basic error handling by checking the response status and catching exceptions. For more detailed error information, refer to the CSV API documentation.
By following these steps, you can efficiently export data as CSV using the CSV API in JavaScript, streamlining your data handling processes.
Conclusion and Best Practices for CSV API Integration in JavaScript
Successfully exporting data as CSV using the CSV API in JavaScript can significantly enhance your application's data handling capabilities. By following the steps outlined in this guide, you can ensure a smooth and efficient integration process.
Best Practices for Secure and Efficient CSV API Integration
- Secure Storage of Credentials: Always store your API credentials securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the CSV API provider. Implement retry logic and exponential backoff strategies to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that the data exported to CSV is properly formatted and standardized. This will facilitate easier data analysis and integration with other systems.
- Error Handling: Implement comprehensive error handling to manage unexpected API responses or network issues. This will improve the reliability of your data export processes.
Streamlining Integrations with Endgrate
While integrating with the CSV API in JavaScript offers powerful data export capabilities, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including CSV APIs.
With Endgrate, you can save time and resources by building once for each use case instead of multiple times for different integrations. This allows you to focus on your core product while offering an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover the benefits of a streamlined, efficient integration process.
Read More
Ready to get started?